Mastering Continuous Integration: A Comprehensive Guide for Modern Developers
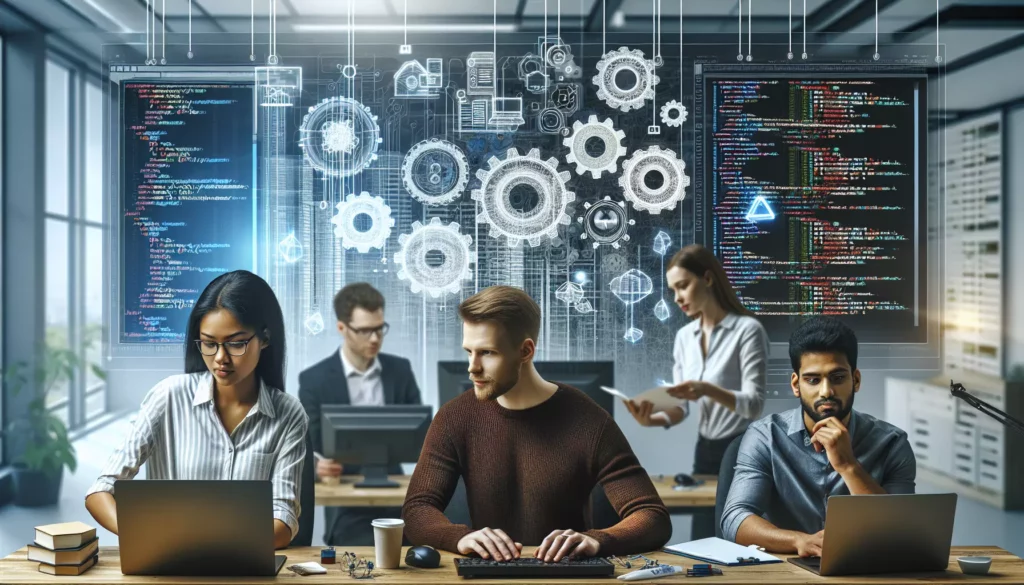
In the fast-paced world of software development, efficiency and quality are paramount. As projects grow in complexity and team sizes increase, the need for streamlined processes becomes crucial. This is where Continuous Integration (CI) comes into play, revolutionizing the way developers work and collaborate. In this comprehensive guide, we’ll dive deep into the world of Continuous Integration, exploring its benefits, best practices, and how it fits into the broader landscape of modern software development.
What is Continuous Integration?
Continuous Integration is a software development practice where members of a team integrate their work frequently, usually multiple times a day. Each integration is verified by an automated build and automated tests. By integrating regularly, you can detect errors quickly, and locate them more easily.
The main goal of CI is to prevent integration problems, also known as “integration hell,” where code works on a developer’s local machine but fails when integrated into the main branch. CI aims to provide rapid feedback so that if a defect is introduced into the code base, it can be identified and corrected as soon as possible.
The Benefits of Continuous Integration
Implementing Continuous Integration in your development workflow offers numerous advantages:
- Early Bug Detection: By integrating and testing code frequently, bugs are caught early in the development process when they’re easier and less expensive to fix.
- Improved Code Quality: Regular integration and automated testing encourage developers to write better, more modular code.
- Faster Release Cycles: CI facilitates quicker and more reliable releases by automating the build and test processes.
- Increased Visibility: The entire team has insight into the state of the codebase and can quickly identify and address issues.
- Reduced Risk: Frequent integrations reduce the risk associated with big-bang integrations at the end of a development cycle.
- Better Collaboration: CI promotes a culture of shared responsibility and encourages developers to communicate and collaborate more effectively.
Key Components of a CI Pipeline
A typical Continuous Integration pipeline consists of several key components:
1. Version Control System
At the heart of any CI setup is a Version Control System (VCS) like Git. It allows developers to manage and track changes to the codebase over time. A VCS is crucial for CI as it provides a central repository where all code changes are pushed and from which the CI system can pull the latest code for building and testing.
2. CI Server
The CI server is the engine that drives the Continuous Integration process. Popular CI servers include Jenkins, Travis CI, CircleCI, and GitLab CI. The CI server monitors the version control system for changes and triggers the build process when new code is pushed.
3. Build Script
The build script is a set of instructions that tell the CI server how to compile the code, run tests, and package the application. This script is typically written in a format specific to the CI tool being used, such as a Jenkinsfile for Jenkins or a .travis.yml file for Travis CI.
4. Automated Tests
Automated tests are a critical component of CI. These tests can include unit tests, integration tests, and end-to-end tests. They ensure that new code changes don’t break existing functionality and meet the required quality standards.
5. Artifact Repository
After a successful build, the resulting artifacts (such as compiled binaries or container images) are often stored in an artifact repository. This allows for easy deployment and rollback if necessary.
6. Notification System
A notification system keeps the team informed about the status of builds and tests. This can include email notifications, Slack messages, or dashboard updates.
Setting Up a Basic CI Pipeline
Let’s walk through the process of setting up a basic CI pipeline using GitHub Actions, a popular CI/CD platform integrated with GitHub repositories.
Step 1: Create a GitHub Repository
If you don’t already have one, create a new repository on GitHub for your project.
Step 2: Add a Workflow File
In your repository, create a new file at .github/workflows/ci.yml
. This YAML file will define your CI workflow.
Step 3: Define the Workflow
Here’s a basic example of a CI workflow for a Python project:
name: CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
python -m unittest discover tests
This workflow does the following:
- Triggers on pushes to the main branch and pull requests targeting the main branch
- Sets up a Python 3.8 environment
- Installs project dependencies
- Runs the project’s test suite
Step 4: Commit and Push
Commit the workflow file and push it to your GitHub repository. GitHub Actions will automatically detect the workflow and start running it on future pushes and pull requests.
Best Practices for Continuous Integration
To get the most out of Continuous Integration, consider adopting these best practices:
1. Maintain a Single Source Repository
Keep all your code and resources in a single repository that’s accessible to all team members. This ensures everyone is working with the same codebase and makes it easier to manage dependencies.
2. Automate the Build
Your build process should be fully automated, requiring no manual intervention. This includes compiling code, running tests, and packaging the application.
3. Make Your Build Self-Testing
Include automated tests in your build process. These tests should cover as much of your codebase as possible and should run quickly to provide fast feedback.
4. Keep the Build Fast
Aim for builds that complete in less than 10 minutes. If builds take too long, developers may be tempted to bypass the CI process or integrate less frequently.
5. Test in a Clone of the Production Environment
Your test environment should mirror your production environment as closely as possible. This helps catch environment-specific issues early.
6. Make it Easy for Anyone to Get the Latest Executable
The output of your CI process should be easily accessible to all team members. This could be in the form of a downloadable artifact or a deployed staging environment.
7. Everyone Can See What’s Happening
Use dashboards or notification systems to keep the entire team informed about the state of the build and any issues that arise.
8. Automate Deployment
While not strictly part of CI, automating your deployment process (Continuous Deployment) is a natural next step that can further streamline your development workflow.
Common Challenges and Solutions
While Continuous Integration offers numerous benefits, it’s not without its challenges. Here are some common issues you might encounter and how to address them:
1. Slow Builds
Challenge: As your project grows, build times can increase, slowing down the feedback loop.
Solution: Optimize your build process by parallelizing tests, using incremental builds, and regularly profiling and optimizing your test suite.
2. Flaky Tests
Challenge: Tests that pass and fail intermittently can erode trust in the CI process.
Solution: Identify and fix flaky tests promptly. Consider using retry mechanisms for inherently unstable tests (like those dependent on external services).
3. Managing Dependencies
Challenge: Keeping track of and updating dependencies can be complex, especially in large projects.
Solution: Use dependency management tools and regularly update dependencies. Consider using tools like Dependabot to automate dependency updates.
4. Resource Constraints
Challenge: CI processes can be resource-intensive, especially for larger projects or teams.
Solution: Optimize resource usage by cleaning up old builds, using caching mechanisms, and considering cloud-based CI solutions that can scale with your needs.
5. Maintaining Test Coverage
Challenge: As the codebase grows, it can be challenging to maintain comprehensive test coverage.
Solution: Use code coverage tools and set coverage thresholds in your CI pipeline. Make writing tests a standard part of your development process.
Continuous Integration in the Context of DevOps
Continuous Integration is a key practice within the broader DevOps movement. DevOps aims to break down silos between development and operations teams, fostering a culture of collaboration and shared responsibility. CI plays a crucial role in this by:
- Enabling faster, more frequent releases
- Improving communication between development and operations teams
- Reducing the time between writing code and deploying it to production
- Increasing the overall reliability and stability of software systems
When combined with Continuous Delivery (CD) and Continuous Deployment, CI forms part of a comprehensive CI/CD pipeline that can significantly streamline the software development lifecycle.
Tools for Continuous Integration
There are numerous tools available to help implement Continuous Integration. Here’s an overview of some popular options:
1. Jenkins
An open-source automation server that supports building, deploying, and automating any project. Jenkins offers a wide range of plugins and can be customized to fit almost any CI/CD need.
2. Travis CI
A hosted CI service that is deeply integrated with GitHub. It’s known for its ease of use and is popular among open-source projects.
3. CircleCI
A cloud-based CI/CD platform that supports rapid software development and deployment. It offers features like parallelism and caching to speed up builds.
4. GitLab CI
Part of the GitLab platform, GitLab CI is deeply integrated with GitLab’s version control and project management features.
5. GitHub Actions
GitHub’s built-in CI/CD solution, offering tight integration with GitHub repositories and a marketplace of pre-built actions.
6. TeamCity
A powerful and user-friendly CI server by JetBrains. It offers a rich set of features out of the box and integrates well with JetBrains IDEs.
Measuring the Success of Your CI Implementation
To ensure your CI implementation is effective, it’s important to track key metrics. Here are some metrics you should consider:
1. Build Duration
The time it takes for a build to complete. Shorter build times generally indicate a more efficient CI process.
2. Build Success Rate
The percentage of builds that pass successfully. A high success rate indicates a stable codebase and effective CI practices.
3. Time to Fix Broken Builds
How long it takes to fix a build after it fails. Quick fixes indicate that the team is responsive to CI feedback.
4. Code Coverage
The percentage of code covered by automated tests. Higher coverage generally indicates more thorough testing.
5. Deployment Frequency
How often code is deployed to production. More frequent deployments often indicate a more agile development process.
6. Mean Time to Recovery (MTTR)
The average time it takes to recover from a failure in production. A lower MTTR suggests that issues are being caught and fixed quickly.
Future Trends in Continuous Integration
As software development practices continue to evolve, so too does Continuous Integration. Here are some trends to watch:
1. AI and Machine Learning in CI
AI and ML are being integrated into CI processes to predict build failures, optimize test selection, and even automatically fix certain types of bugs.
2. Shift-Left Security
Security testing is being integrated earlier in the CI pipeline, catching vulnerabilities before they make it to production.
3. Containerization and Microservices
CI processes are adapting to handle containerized applications and microservices architectures, with tools focusing on container-specific build and test processes.
4. GitOps
The practice of using Git as the single source of truth for declarative infrastructure and applications is gaining traction, influencing how CI pipelines are structured.
5. Serverless CI
Serverless computing is being applied to CI, allowing for more scalable and cost-effective build processes.
Conclusion
Continuous Integration has become an indispensable practice in modern software development. By automating the integration and testing of code changes, CI helps teams deliver high-quality software more rapidly and reliably. While implementing CI can be challenging, the benefits in terms of improved code quality, faster release cycles, and better team collaboration are well worth the effort.
As you embark on your CI journey, remember that it’s not just about tools and processes—it’s also about fostering a culture of continuous improvement and shared responsibility within your team. Start small, measure your progress, and continuously refine your approach. With time and practice, Continuous Integration will become an integral part of your development workflow, enabling you to deliver better software, faster.
Whether you’re a seasoned developer looking to optimize your CI practices or a newcomer taking your first steps into automated builds and testing, the world of Continuous Integration offers endless opportunities for learning and improvement. Embrace the challenge, stay curious, and happy coding!