Mastering Contextual Computing: Building Adaptive Applications for Enhanced User Experience
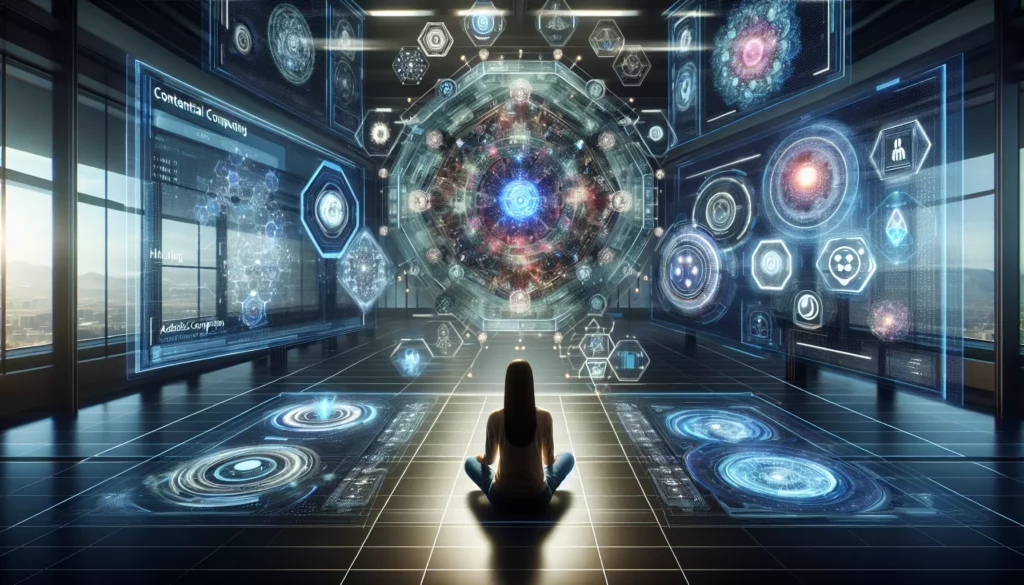
In today’s rapidly evolving technological landscape, the ability to create applications that adapt to user context has become increasingly crucial. This concept, known as contextual computing, is revolutionizing the way we interact with digital systems and enhancing user experiences across various domains. In this comprehensive guide, we’ll delve deep into the world of contextual computing, exploring its importance, key principles, and practical implementation strategies.
What is Contextual Computing?
Contextual computing refers to the development of applications and systems that can understand and respond to the user’s current context. This context can include various factors such as:
- Location
- Time of day
- User preferences
- Device capabilities
- Environmental conditions
- User behavior patterns
By taking these contextual elements into account, applications can provide more personalized, relevant, and efficient experiences for users. This adaptability is becoming increasingly important in a world where users expect seamless interactions across multiple devices and environments.
The Importance of Contextual Computing in Modern Applications
As we progress further into the digital age, the significance of contextual computing continues to grow. Here are some key reasons why developers and businesses should prioritize contextual computing in their applications:
- Enhanced User Experience: By adapting to the user’s context, applications can provide more intuitive and personalized interactions, leading to increased user satisfaction and engagement.
- Improved Efficiency: Contextual awareness allows applications to anticipate user needs and streamline processes, saving time and reducing cognitive load for users.
- Increased Relevance: By delivering content and functionality that is appropriate to the user’s current situation, applications can maintain their relevance and utility across various contexts.
- Better Resource Utilization: Context-aware applications can optimize resource usage by adjusting their behavior based on device capabilities and network conditions.
- Competitive Advantage: As users become accustomed to context-aware experiences, applications that fail to adapt may struggle to compete in the market.
Key Principles of Contextual Computing
To effectively implement contextual computing in your applications, it’s essential to understand and apply the following key principles:
1. Context Sensing
The foundation of contextual computing lies in the ability to gather and interpret contextual information. This involves leveraging various sensors and data sources to collect relevant data about the user’s environment and situation. Some common context sensing techniques include:
- GPS for location tracking
- Accelerometers for motion detection
- Light sensors for ambient light measurement
- Microphones for audio input
- Cameras for visual input
- Network connectivity status
- Time and date information
2. Context Interpretation
Once contextual data is collected, it needs to be interpreted to derive meaningful insights. This often involves combining multiple data points and applying machine learning algorithms to recognize patterns and infer higher-level context. For example, combining GPS data with time information might help determine if a user is at work, home, or commuting.
3. Adaptive Behavior
Based on the interpreted context, applications should be able to adapt their behavior, user interface, or content to provide the most appropriate experience. This might involve:
- Adjusting the UI layout for different screen sizes and orientations
- Modifying app functionality based on the user’s location
- Personalizing content recommendations based on user preferences and behavior
- Optimizing performance based on device capabilities and network conditions
4. Privacy and Security
As contextual computing often involves collecting and processing sensitive user data, it’s crucial to prioritize privacy and security. This includes implementing robust data protection measures, obtaining user consent for data collection, and providing transparent controls for users to manage their privacy settings.
5. Continuous Learning and Improvement
Contextual computing systems should be designed to learn and improve over time. This involves collecting feedback on the effectiveness of adaptive behaviors and refining the context interpretation and adaptation strategies accordingly.
Implementing Contextual Computing in Your Applications
Now that we’ve covered the key principles, let’s explore how to implement contextual computing in your applications. Here’s a step-by-step guide to get you started:
Step 1: Identify Relevant Contextual Factors
Begin by determining which contextual factors are most relevant to your application. Consider the following questions:
- What type of information would help your app provide a better user experience?
- Which sensors or data sources are available on your target devices?
- What contextual information can be inferred from user behavior within your app?
Step 2: Implement Context Sensing
Based on the identified contextual factors, implement the necessary code to collect relevant data. Here’s an example of how to access the device’s location using JavaScript:
if ("geolocation" in navigator) {
navigator.geolocation.getCurrentPosition(function(position) {
var latitude = position.coords.latitude;
var longitude = position.coords.longitude;
console.log("Latitude: " + latitude + ", Longitude: " + longitude);
});
} else {
console.log("Geolocation is not supported by this browser.");
}
Step 3: Develop Context Interpretation Logic
Create algorithms or utilize machine learning models to interpret the collected contextual data. This might involve combining multiple data points to infer higher-level context. For example, you could use a simple rule-based system to determine the user’s activity based on location and time:
function determineUserActivity(latitude, longitude, time) {
const workLocation = { lat: 37.7749, lon: -122.4194 }; // Example coordinates
const homeLocation = { lat: 37.7858, lon: -122.4064 }; // Example coordinates
const distanceToWork = calculateDistance(latitude, longitude, workLocation.lat, workLocation.lon);
const distanceToHome = calculateDistance(latitude, longitude, homeLocation.lat, homeLocation.lon);
const hour = new Date(time).getHours();
if (distanceToWork < 0.1 && hour >= 9 && hour < 17) {
return "At work";
} else if (distanceToHome < 0.1 && (hour < 9 || hour >= 17)) {
return "At home";
} else {
return "Out and about";
}
}
function calculateDistance(lat1, lon1, lat2, lon2) {
// Implementation of distance calculation (e.g., using Haversine formula)
// ...
}
Step 4: Implement Adaptive Behaviors
Based on the interpreted context, implement adaptive behaviors in your application. This could involve adjusting the UI, modifying functionality, or personalizing content. Here’s an example of how you might adapt the UI based on the user’s activity:
function adaptUIToUserActivity(activity) {
switch (activity) {
case "At work":
document.body.classList.add("work-mode");
showProductivityFeatures();
break;
case "At home":
document.body.classList.add("home-mode");
showEntertainmentFeatures();
break;
case "Out and about":
document.body.classList.add("mobile-mode");
showLocationBasedFeatures();
break;
}
}
function showProductivityFeatures() {
// Implement logic to display productivity-focused features
}
function showEntertainmentFeatures() {
// Implement logic to display entertainment-focused features
}
function showLocationBasedFeatures() {
// Implement logic to display location-relevant features
}
Step 5: Implement Privacy Controls
Ensure that your application respects user privacy by implementing appropriate controls and obtaining necessary permissions. Here’s an example of how to request permission for location access:
function requestLocationPermission() {
if ("geolocation" in navigator) {
navigator.permissions.query({ name: "geolocation" }).then(function(result) {
if (result.state === "granted") {
console.log("Location permission already granted");
} else if (result.state === "prompt") {
console.log("Location permission will be requested");
navigator.geolocation.getCurrentPosition(function() {
console.log("Permission granted");
}, function() {
console.log("Permission denied");
});
} else if (result.state === "denied") {
console.log("Location permission denied");
}
});
} else {
console.log("Geolocation is not supported by this browser");
}
}
Step 6: Implement Feedback Mechanisms
To continuously improve your contextual computing system, implement mechanisms to collect feedback on the effectiveness of your adaptive behaviors. This could involve explicit user feedback or implicit measures such as user engagement metrics. Here’s a simple example of collecting explicit feedback:
function collectAdaptationFeedback(adaptationType, isHelpful) {
// Send feedback to server or store locally
const feedback = {
adaptationType: adaptationType,
isHelpful: isHelpful,
timestamp: new Date().toISOString()
};
// Example: Sending feedback to a server
fetch("/api/feedback", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(feedback)
})
.then(response => response.json())
.then(data => console.log("Feedback sent successfully", data))
.catch(error => console.error("Error sending feedback", error));
}
// Usage
document.getElementById("feedbackYes").addEventListener("click", function() {
collectAdaptationFeedback("workModeUI", true);
});
document.getElementById("feedbackNo").addEventListener("click", function() {
collectAdaptationFeedback("workModeUI", false);
});
Advanced Contextual Computing Techniques
As you become more comfortable with the basics of contextual computing, you can explore more advanced techniques to enhance your applications:
1. Machine Learning for Context Prediction
Implement machine learning models to predict user context based on historical data. This can help your application anticipate user needs and proactively adapt. For example, you could use a neural network to predict the likelihood of a user needing certain features based on their past behavior and current context.
2. Multi-modal Context Sensing
Combine data from multiple sensors and sources to create a more comprehensive understanding of user context. This might involve fusing data from GPS, accelerometers, and calendar information to infer complex contexts like “in a meeting” or “commuting to work.”
3. Edge Computing for Context Processing
Utilize edge computing techniques to process contextual data locally on the user’s device, reducing latency and improving privacy. This is particularly useful for applications that need to respond quickly to changes in context or handle sensitive contextual information.
4. Contextual Group Awareness
Extend contextual computing beyond individual users to consider group contexts. This can be valuable for collaborative applications or social platforms where the context of multiple users needs to be considered simultaneously.
5. Cross-platform Context Sharing
Implement mechanisms to share contextual information across different devices and platforms, allowing for seamless user experiences as they move between devices. This might involve cloud-based context storage or direct device-to-device communication.
Challenges and Considerations in Contextual Computing
While contextual computing offers numerous benefits, it also comes with its own set of challenges that developers need to be aware of:
1. Privacy Concerns
Collecting and processing contextual data can raise significant privacy concerns. It’s crucial to be transparent about data collection practices, obtain user consent, and provide robust privacy controls. Consider implementing data minimization techniques and local processing where possible to reduce privacy risks.
2. Battery and Resource Consumption
Continuous context sensing and processing can be resource-intensive, potentially impacting device battery life and performance. Optimize your context sensing algorithms and consider implementing adaptive sensing strategies that adjust based on the importance of the context and available resources.
3. Accuracy and Reliability
Interpreting context accurately can be challenging, especially when dealing with noisy or incomplete data. Implement robust error handling and fallback mechanisms to ensure your application degrades gracefully when contextual information is unreliable or unavailable.
4. Over-adaptation
While adaptation can enhance user experience, excessive or unpredictable changes can lead to user confusion and frustration. Strike a balance between adaptivity and consistency, and consider providing users with options to customize or disable certain adaptive behaviors.
5. Cross-cultural Considerations
Context interpretation may vary across different cultures and regions. Ensure your contextual computing system is designed with cultural sensitivity and can be localized for different markets.
Future Trends in Contextual Computing
As technology continues to evolve, several emerging trends are shaping the future of contextual computing:
1. Integration with Artificial Intelligence
The convergence of contextual computing with advanced AI technologies like deep learning and natural language processing will enable more sophisticated context interpretation and adaptive behaviors.
2. Internet of Things (IoT) Integration
As IoT devices become more prevalent, contextual computing systems will be able to leverage a wider array of sensors and data sources, enabling more comprehensive context awareness.
3. Augmented Reality (AR) and Context
Contextual computing will play a crucial role in enhancing AR experiences, enabling more seamless integration of digital information with the physical world based on user context.
4. Emotional and Physiological Context
Advancements in affective computing and biometric sensors will allow applications to consider emotional and physiological factors as part of the user’s context, enabling more empathetic and responsive systems.
5. Federated Learning for Privacy-Preserving Context Models
To address privacy concerns, federated learning techniques may be employed to train contextual models across multiple devices without centralizing sensitive user data.
Conclusion
Contextual computing represents a powerful paradigm shift in application development, enabling the creation of more intelligent, responsive, and user-centric experiences. By understanding and implementing the principles of contextual computing, developers can create applications that truly adapt to user needs and environmental factors.
As we’ve explored in this comprehensive guide, the journey to mastering contextual computing involves careful consideration of context sensing, interpretation, adaptive behaviors, and privacy concerns. By following the step-by-step implementation guide and keeping abreast of advanced techniques and future trends, you’ll be well-equipped to harness the power of contextual computing in your applications.
Remember that contextual computing is an evolving field, and staying curious and experimental will be key to pushing the boundaries of what’s possible. As you implement these concepts in your projects, always keep the user experience at the forefront, ensuring that your adaptive applications truly enhance and simplify the lives of your users.
By embracing contextual computing, you’re not just building applications; you’re creating intelligent companions that understand and respond to the ever-changing contexts of our digital lives. So go forth, experiment, and shape the future of adaptive computing!