Mastering Concurrency and Multithreading: A Comprehensive Guide for Technical Interviews
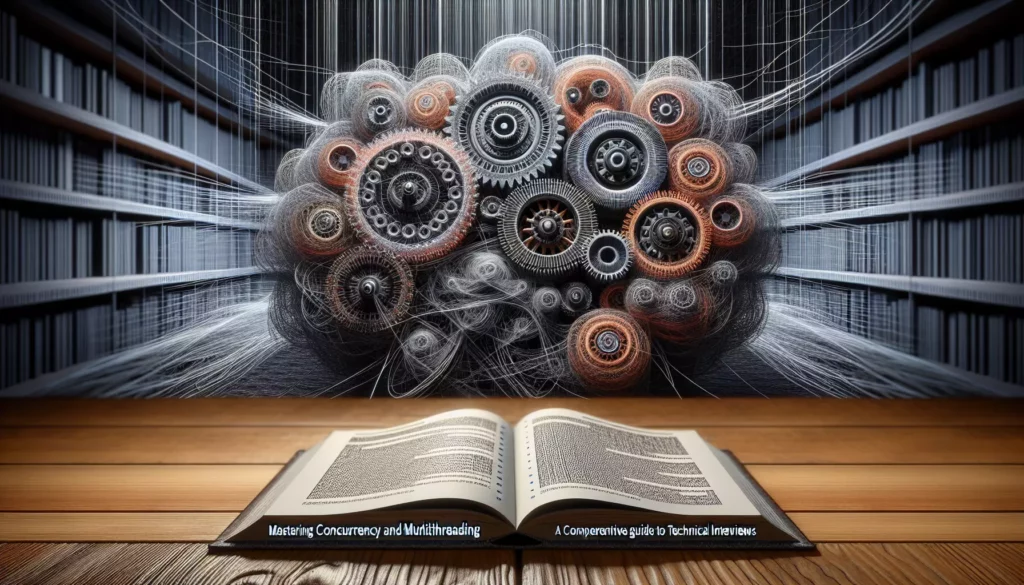
In the world of modern software development, concurrency and multithreading have become essential concepts that every programmer should master. As applications grow in complexity and scale, the ability to efficiently utilize system resources and handle multiple tasks simultaneously has become crucial. This is particularly true when preparing for technical interviews at top tech companies, where these topics are frequently explored to assess a candidate’s understanding of advanced programming concepts.
In this comprehensive guide, we’ll dive deep into the world of concurrency and multithreading, exploring key concepts, common challenges, and effective strategies for tackling related interview questions. Whether you’re a beginner looking to expand your knowledge or an experienced developer preparing for a big interview, this article will equip you with the tools and insights you need to succeed.
Understanding Concurrency and Multithreading
Before we delve into specific interview strategies, let’s start by clarifying the fundamental concepts of concurrency and multithreading:
Concurrency
Concurrency refers to the ability of a system to handle multiple tasks or processes simultaneously. In a concurrent system, multiple computations can make progress at the same time, even if they’re not necessarily executed in parallel. Concurrency is about dealing with multiple things at once, but not necessarily doing them at the exact same moment.
Multithreading
Multithreading is a programming concept where a single process can have multiple threads of execution running concurrently. Each thread represents an independent path of execution within the same program, sharing the same memory space but having its own stack and program counter. Multithreading allows for parallel execution of code, potentially improving performance and responsiveness in applications.
While concurrency and multithreading are related concepts, they’re not exactly the same. Concurrency is a broader term that encompasses various approaches to managing multiple tasks, while multithreading is a specific implementation of concurrency using threads within a single process.
Common Concurrency and Multithreading Concepts
To excel in technical interviews, it’s crucial to have a solid grasp of the following concepts:
1. Threads and Processes
Understanding the difference between threads and processes is fundamental:
- Process: An independent program in execution with its own memory space.
- Thread: A lightweight unit of execution within a process, sharing the same memory space with other threads in the same process.
2. Synchronization
Synchronization mechanisms are used to coordinate access to shared resources and prevent race conditions. Key synchronization primitives include:
- Mutex (Mutual Exclusion): Ensures that only one thread can access a shared resource at a time.
- Semaphore: Controls access to a shared resource by maintaining a set of permits.
- Monitor: A synchronization construct that combines mutex and condition variables.
3. Deadlocks
A deadlock occurs when two or more threads are unable to proceed because each is waiting for the other to release a resource. Understanding how to detect, prevent, and resolve deadlocks is crucial.
4. Race Conditions
Race conditions happen when the behavior of a program depends on the relative timing of events, particularly when multiple threads access shared data concurrently. Identifying and mitigating race conditions is a key skill.
5. Thread Safety
Thread-safe code can be safely called from multiple threads without causing unintended behavior or data corruption. Implementing thread-safe data structures and algorithms is an important aspect of concurrent programming.
6. Parallel Programming Models
Familiarity with different parallel programming models can be beneficial:
- Fork-Join: A model where a task is divided into smaller subtasks that are executed in parallel and then joined.
- Producer-Consumer: A pattern where one or more producer threads generate data consumed by one or more consumer threads.
- Reader-Writer: A model that allows concurrent read access to shared data but exclusive write access.
Strategies for Approaching Concurrency and Multithreading Questions
When faced with concurrency and multithreading questions in technical interviews, consider the following strategies:
1. Identify the Core Problem
Start by clearly understanding the problem at hand. Is it a synchronization issue? A resource allocation problem? Or perhaps a performance optimization challenge? Identifying the core problem will guide your approach to the solution.
2. Consider Thread Safety
Always evaluate whether the given scenario requires thread-safe implementations. If multiple threads are accessing shared resources, think about how to ensure data integrity and prevent race conditions.
3. Choose Appropriate Synchronization Mechanisms
Based on the problem requirements, select the most suitable synchronization primitives. Consider factors such as the number of threads, the nature of the shared resource, and the desired level of concurrency.
4. Be Mindful of Deadlocks
When designing solutions involving multiple locks or resources, always consider the possibility of deadlocks. Implement strategies to prevent or detect deadlocks, such as using a consistent order for acquiring locks.
5. Optimize for Performance
While ensuring correctness is paramount, also consider the performance implications of your solution. Look for opportunities to minimize contention and maximize parallelism where appropriate.
6. Use Higher-Level Abstractions When Appropriate
Many modern programming languages provide high-level concurrency constructs (e.g., Java’s ExecutorService or Python’s asyncio). Don’t hesitate to leverage these when they simplify the solution without sacrificing performance.
7. Consider Edge Cases
Think about potential edge cases, such as what happens when a thread is interrupted, how the system behaves under high load, or how it recovers from failures.
8. Explain Your Reasoning
As you develop your solution, clearly articulate your thought process. Explain why you chose certain synchronization mechanisms or design patterns, and discuss any trade-offs you considered.
Common Types of Concurrency and Multithreading Interview Questions
To help you prepare, let’s explore some common types of questions you might encounter in technical interviews:
1. Implementing Classic Concurrency Problems
Interviewers often ask candidates to implement solutions to well-known concurrency problems. Some examples include:
- The Dining Philosophers Problem
- The Producer-Consumer Problem
- The Readers-Writers Problem
For these questions, focus on demonstrating your understanding of synchronization primitives and your ability to prevent deadlocks and race conditions.
2. Designing Thread-Safe Data Structures
You might be asked to implement thread-safe versions of common data structures, such as:
- A thread-safe queue
- A concurrent hash map
- A lock-free stack
When tackling these problems, consider the trade-offs between different synchronization mechanisms and their impact on performance.
3. Parallelizing Algorithms
Some questions may involve parallelizing existing algorithms or designing parallel versions of common operations. Examples include:
- Implementing a parallel merge sort
- Designing a concurrent web crawler
- Parallelizing matrix multiplication
For these questions, focus on how to divide the problem into independent subtasks and how to efficiently combine the results.
4. Debugging Concurrency Issues
Interviewers might present you with code snippets containing concurrency bugs and ask you to identify and fix the issues. Common problems include:
- Race conditions
- Deadlocks
- Livelock situations
When approaching these questions, systematically analyze the code, identify potential points of contention, and propose solutions to resolve the issues.
5. Scaling and Performance Optimization
Some questions may focus on optimizing concurrent systems for performance and scalability. You might be asked to:
- Design a highly concurrent caching system
- Optimize a database connection pool
- Implement a load balancing algorithm
For these questions, consider factors such as thread pool sizing, resource utilization, and strategies for minimizing contention.
Sample Interview Question and Solution
Let’s walk through a sample interview question and a possible solution to illustrate how to apply these strategies in practice.
Question: Implement a Thread-Safe Bounded Buffer
Implement a thread-safe bounded buffer that can be used by multiple producer and consumer threads. The buffer should have a fixed capacity and support the following operations:
put(item)
: Add an item to the buffer. If the buffer is full, the thread should wait until space becomes available.get()
: Remove and return an item from the buffer. If the buffer is empty, the thread should wait until an item becomes available.
Solution:
Here’s a possible implementation of a thread-safe bounded buffer in Java:
import java.util.LinkedList;
import java.util.Queue;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class BoundedBuffer<T> {
private final Queue<T> buffer;
private final int capacity;
private final Lock lock;
private final Condition notFull;
private final Condition notEmpty;
public BoundedBuffer(int capacity) {
this.capacity = capacity;
this.buffer = new LinkedList<>();
this.lock = new ReentrantLock();
this.notFull = lock.newCondition();
this.notEmpty = lock.newCondition();
}
public void put(T item) throws InterruptedException {
lock.lock();
try {
while (buffer.size() == capacity) {
notFull.await();
}
buffer.add(item);
notEmpty.signal();
} finally {
lock.unlock();
}
}
public T get() throws InterruptedException {
lock.lock();
try {
while (buffer.isEmpty()) {
notEmpty.await();
}
T item = buffer.remove();
notFull.signal();
return item;
} finally {
lock.unlock();
}
}
}
Let’s break down this solution and explain the key concepts:
- Data Structure: We use a
Queue
to store the items in the buffer. TheLinkedList
implementation provides efficient add and remove operations. - Synchronization: We use a
ReentrantLock
for mutual exclusion and twoCondition
variables (notFull
andnotEmpty
) for signaling between threads. - Capacity Limit: We maintain a fixed capacity and check it before adding items to the buffer.
- Blocking Operations: Both
put()
andget()
methods block when the buffer is full or empty, respectively, using the condition variables. - Thread Safety: All operations on the shared buffer are performed within the lock, ensuring thread safety.
This implementation addresses several key aspects of concurrent programming:
- It prevents race conditions by using a lock to ensure mutual exclusion when accessing the shared buffer.
- It avoids busy-waiting by using condition variables to efficiently suspend and resume threads.
- It handles the producer-consumer problem by coordinating between threads that add items (producers) and threads that remove items (consumers).
- It respects the bounded nature of the buffer, preventing overflow and underflow conditions.
Advanced Topics and Further Considerations
As you progress in your understanding of concurrency and multithreading, consider exploring these advanced topics:
1. Memory Models and Cache Coherence
Understanding how modern processors handle memory and maintain cache coherence is crucial for writing high-performance concurrent code. Familiarize yourself with concepts like memory barriers, volatile variables, and the happens-before relationship.
2. Lock-Free and Wait-Free Algorithms
Lock-free and wait-free algorithms aim to improve concurrency by avoiding traditional locking mechanisms. These techniques can significantly enhance performance in highly concurrent systems but require a deep understanding of memory ordering and atomic operations.
3. Concurrent Data Structures
Study advanced concurrent data structures like concurrent skip lists, lock-free queues, and concurrent hash maps. Understanding the design principles behind these structures can help you create efficient solutions for complex concurrency problems.
4. Actor Model and Message Passing
The actor model is an alternative approach to concurrency that focuses on message passing between independent actors. Familiarize yourself with frameworks like Akka for Java/Scala or Erlang’s built-in actor support.
5. Parallel Programming Patterns
Learn about common parallel programming patterns such as map-reduce, pipeline parallelism, and speculative execution. Understanding these patterns can help you design scalable solutions for a wide range of problems.
6. Concurrency in Distributed Systems
Explore how concurrency concepts apply to distributed systems, including topics like distributed locking, consensus algorithms (e.g., Paxos, Raft), and eventual consistency.
Conclusion
Mastering concurrency and multithreading is a challenging but rewarding journey that can significantly enhance your capabilities as a software developer. As you prepare for technical interviews, remember that practice is key. Implement various concurrent algorithms, experiment with different synchronization techniques, and analyze real-world concurrent systems to deepen your understanding.
Keep in mind that while theoretical knowledge is important, interviewers are often more interested in your problem-solving approach and your ability to reason about concurrent systems. Be prepared to discuss trade-offs, explain your design decisions, and demonstrate an awareness of potential pitfalls.
By following the strategies outlined in this guide and continually expanding your knowledge of concurrency and multithreading, you’ll be well-equipped to tackle even the most challenging interview questions in this domain. Remember, the goal is not just to pass the interview but to develop skills that will serve you throughout your career as a software engineer.
Happy coding, and best of luck in your technical interviews!