Mastering Computer Algebra Systems: A Deep Dive into Mathematica and SageMath
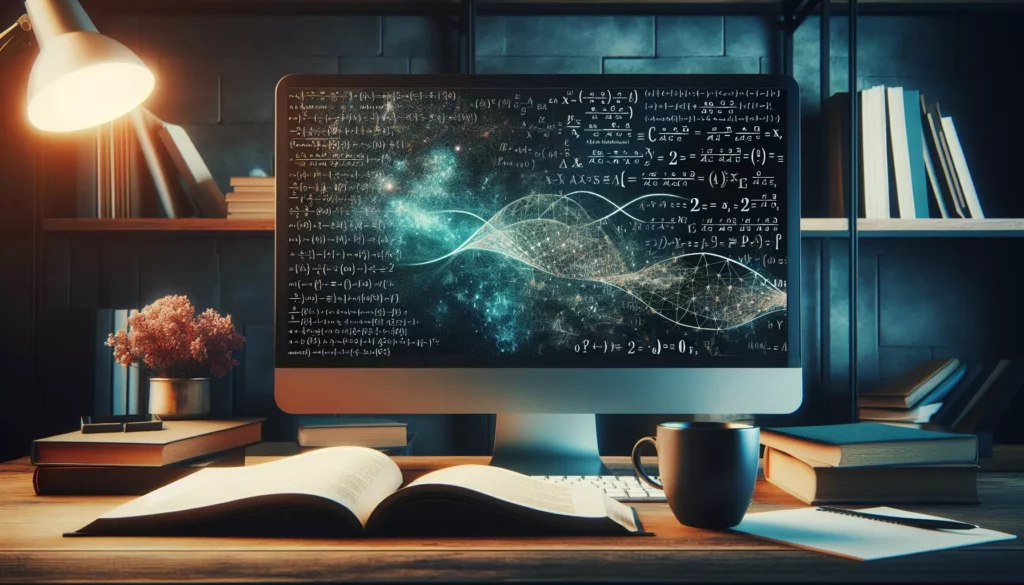
In the ever-evolving landscape of coding education and programming skills development, one area that often gets overlooked but holds immense potential is the use of Computer Algebra Systems (CAS). These powerful software tools can significantly enhance your problem-solving abilities and deepen your understanding of complex mathematical concepts. In this comprehensive guide, we’ll explore two of the most popular CAS: Mathematica and SageMath. We’ll discuss how these tools can be integrated into your coding journey, from beginner-level coding to preparing for technical interviews at major tech companies.
Understanding Computer Algebra Systems
Before we dive into the specifics of Mathematica and SageMath, let’s first understand what Computer Algebra Systems are and why they’re important in the context of coding education and algorithmic thinking.
A Computer Algebra System is a software program that facilitates symbolic mathematics. Unlike traditional calculators or programming languages that primarily deal with numerical computations, CAS can manipulate mathematical expressions in their symbolic form. This means they can perform operations like:
- Algebraic simplification
- Solving equations symbolically
- Differentiation and integration
- Matrix operations
- Series expansions
- And much more
The power of CAS lies in their ability to handle these operations with precision, often providing exact solutions rather than numerical approximations. This makes them invaluable tools for both theoretical exploration and practical problem-solving in fields ranging from pure mathematics to physics, engineering, and computer science.
Mathematica: The Powerhouse of Symbolic Computation
Mathematica, developed by Wolfram Research, is one of the most comprehensive and powerful CAS available. It’s widely used in academia, research, and industry for its robust capabilities and user-friendly interface.
Key Features of Mathematica
- Symbolic Computation: Mathematica excels at manipulating mathematical expressions symbolically, allowing for exact solutions to complex problems.
- Numerical Computation: Despite its strength in symbolic math, Mathematica is also highly capable of performing numerical calculations with high precision.
- Data Analysis and Visualization: With built-in functions for data manipulation and a wide range of plotting options, Mathematica is a powerful tool for data science and visualization.
- Programming Language: Mathematica comes with its own programming language, which is particularly well-suited for mathematical and scientific computing.
- Machine Learning and AI: Recent versions of Mathematica include robust machine learning and artificial intelligence capabilities, making it a versatile tool for cutting-edge research and development.
Using Mathematica in Your Coding Journey
For those on the path of coding education, Mathematica can be an invaluable asset. Here’s how you can incorporate it into your learning process:
- Algorithm Visualization: Use Mathematica to visualize algorithms, helping you understand complex concepts more intuitively. For example, you can easily visualize sorting algorithms or graph traversal techniques.
- Problem Solving: When tackling complex mathematical problems in your coding challenges, Mathematica can help you verify your solutions or provide insights into the problem structure.
- Code Optimization: By implementing algorithms in Mathematica and comparing them with your own code, you can gain insights into optimization techniques and efficiency improvements.
- Data Structure Exploration: Mathematica’s powerful list manipulation capabilities make it an excellent tool for exploring and understanding various data structures.
Example: Implementing Quicksort in Mathematica
Here’s a simple implementation of the Quicksort algorithm in Mathematica:
quickSort[{}] := {}
quickSort[{pivot_, rest___}] :=
Join[quickSort[Select[{rest}, # <= pivot &]],
{pivot},
quickSort[Select[{rest}, # > pivot &]]]
list = RandomInteger[100, 20];
Print["Original list: ", list]
Print["Sorted list: ", quickSort[list]]
This implementation showcases Mathematica’s concise syntax and powerful list manipulation capabilities. You can easily visualize the sorting process by adding print statements or using Mathematica’s dynamic visualization features.
SageMath: The Open-Source Alternative
SageMath, often simply called Sage, is a free, open-source mathematics software system. It builds on top of many existing open-source packages, combining them into a common Python-based interface.
Key Features of SageMath
- Open-Source: Being free and open-source makes SageMath accessible to everyone, from students to researchers.
- Python-Based: SageMath uses Python as its primary programming language, making it easier for those already familiar with Python to get started.
- Comprehensive Library: It includes a vast library of mathematical functions, covering algebra, calculus, number theory, cryptography, and more.
- Integration with Other Tools: SageMath can interface with other mathematical software like Mathematica, Maple, and MATLAB.
- Web-Based Interface: SageMath provides a notebook interface similar to Jupyter notebooks, making it easy to create interactive mathematical documents.
Using SageMath in Your Coding Education
SageMath can be a powerful ally in your coding education journey. Here are some ways to leverage it:
- Learning Python: Since SageMath is based on Python, it’s an excellent way to learn Python while exploring mathematical concepts.
- Exploring Algorithms: Implement and visualize various algorithms in SageMath to deepen your understanding.
- Number Theory and Cryptography: SageMath’s strong capabilities in these areas make it an excellent tool for exploring concepts related to computer security and encryption.
- Graph Theory: Use SageMath’s graph theory functions to visualize and manipulate graphs, which is crucial for many computer science algorithms.
Example: Implementing a Simple Graph Algorithm in SageMath
Here’s an example of how to create a graph and find its shortest paths using SageMath:
# Create a graph
G = Graph({0:[1,4,5], 1:[2,3,4], 2:[3], 3:[4], 4:[5]})
G.show() # Visualize the graph
# Find shortest paths
start = 0
for end in range(1, 6):
print(f"Shortest path from {start} to {end}: {G.shortest_path(start, end)}")
This example demonstrates how easily you can work with graphs in SageMath, which is particularly useful when studying algorithms related to graph theory.
Integrating CAS into Your Coding Interview Preparation
As you progress in your coding education and begin preparing for technical interviews, particularly for major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), Computer Algebra Systems can be powerful allies. Here’s how you can leverage Mathematica and SageMath in your interview preparation:
1. Algorithm Analysis
Use CAS to analyze the time and space complexity of algorithms. For example, you can use Mathematica or SageMath to plot the growth of different complexity classes (O(n), O(n log n), O(n^2), etc.) and visualize how they compare. This can help you develop a strong intuition for algorithm efficiency.
2. Problem Solving Practice
Many coding interview questions involve mathematical concepts. Use CAS to explore these concepts deeply. For instance, if you’re working on a problem involving prime numbers, you can use SageMath’s number theory functions to generate prime numbers, factorize large numbers, or explore properties of modular arithmetic.
3. Visualizing Data Structures
Both Mathematica and SageMath excel at visualization. Use them to create visual representations of complex data structures like trees, graphs, or multi-dimensional arrays. This can help you better understand how these structures work and how to manipulate them efficiently.
4. Verifying Solutions
After implementing an algorithm, use CAS to verify your solution for various inputs. This is particularly useful for problems involving mathematical computations or optimizations.
5. Exploring Edge Cases
CAS can help you generate and explore edge cases for your algorithms. For example, you can use Mathematica to generate large random datasets or specific types of input that might stress-test your algorithm.
Advanced Topics: Leveraging CAS for Specific Interview Topics
Let’s dive deeper into how you can use Computer Algebra Systems to prepare for specific topics that often come up in coding interviews:
1. Dynamic Programming
Dynamic Programming (DP) problems often involve recognizing and exploiting mathematical patterns. Use CAS to:
- Visualize the recurrence relations in DP problems
- Generate and analyze sequences that form the basis of many DP problems
- Explore the time and space complexity of different DP approaches
For example, in Mathematica, you could visualize the Fibonacci sequence and its growth:
fibSequence = Table[Fibonacci[n], {n, 0, 20}];
ListPlot[fibSequence, Joined -> True,
PlotLabel -> "Growth of Fibonacci Sequence",
AxesLabel -> {"n", "Fibonacci(n)"}]
2. Graph Algorithms
Both Mathematica and SageMath have robust graph theory capabilities. Use them to:
- Visualize different types of graphs (directed, undirected, weighted)
- Implement and test graph algorithms like DFS, BFS, Dijkstra’s algorithm
- Analyze the properties of graphs (connectivity, cycles, etc.)
Here’s an example of creating and visualizing a graph in SageMath:
G = graphs.PetersenGraph()
G.show(edge_labels=True, graph_border=True)
print(f"Diameter of the graph: {G.diameter()}")
print(f"Is the graph connected? {G.is_connected()}")
3. Bit Manipulation
Bit manipulation questions are common in coding interviews. Use CAS to:
- Visualize binary representations of numbers
- Explore bitwise operations and their properties
- Analyze patterns in binary representations
In Mathematica, you could create a function to visualize the binary representation of numbers:
visualizeBinary[n_Integer] :=
Grid[Partition[Reverse@IntegerDigits[n, 2, 8], 4],
Frame -> All,
Background -> {None, None, {White, LightGray}}]
Table[{n, visualizeBinary[n]}, {n, 0, 15}] // TableForm
4. Number Theory
Number theory problems often appear in cryptography-related questions. Use CAS to:
- Generate prime numbers and explore their properties
- Implement and test algorithms like the Sieve of Eratosthenes
- Explore modular arithmetic and its applications
Here’s an example of implementing the Sieve of Eratosthenes in SageMath:
def sieve_of_eratosthenes(n):
primes = [True] * (n + 1)
primes[0] = primes[1] = False
for i in range(2, int(n**0.5) + 1):
if primes[i]:
for j in range(i*i, n+1, i):
primes[j] = False
return [i for i in range(n+1) if primes[i]]
primes = sieve_of_eratosthenes(100)
print(f"Primes up to 100: {primes}")
Practical Tips for Incorporating CAS into Your Study Routine
Now that we’ve explored the capabilities of Computer Algebra Systems and how they can be applied to coding interview preparation, let’s discuss some practical tips for incorporating these tools into your study routine:
1. Start with Simple Problems
Begin by using CAS to solve simple mathematical problems or implement basic algorithms. This will help you get familiar with the syntax and capabilities of the system you’re using.
2. Parallel Implementation
When working on a coding problem, try implementing the solution both in your primary programming language (e.g., Python or Java) and in a CAS. Compare the implementations and runtime performance. This can provide insights into the strengths and weaknesses of different approaches.
3. Use CAS for Verification
After solving a problem, use CAS to verify your solution. This is particularly useful for problems involving complex mathematical calculations or large datasets.
4. Explore Visualizations
Take advantage of the visualization capabilities of CAS. Create visual representations of algorithms, data structures, or mathematical concepts. This can help reinforce your understanding and provide new insights.
5. Dive Deep into Mathematical Concepts
Use CAS to explore the mathematical concepts underlying algorithms and data structures. For example, you could use Mathematica or SageMath to explore the properties of different hash functions or the distribution of prime numbers.
6. Create a CAS Notebook for Each Topic
As you study different topics, create a notebook (in Mathematica) or a worksheet (in SageMath) for each major topic. Use these to collect relevant functions, visualizations, and examples. This can serve as a valuable reference during your interview preparation.
7. Collaborate and Share
Many CAS have features that allow you to share your work. Collaborate with other learners, share your insights, and learn from others’ approaches. This can broaden your perspective and expose you to new problem-solving techniques.
Conclusion: Elevating Your Coding Skills with Computer Algebra Systems
Incorporating Computer Algebra Systems like Mathematica and SageMath into your coding education and interview preparation can significantly enhance your problem-solving skills and deepen your understanding of complex algorithms and data structures. These powerful tools offer a unique blend of symbolic computation, visualization capabilities, and programming flexibility that can complement your traditional coding practice.
By leveraging CAS, you can:
- Gain deeper insights into mathematical concepts underlying algorithms
- Visualize complex data structures and algorithm behavior
- Verify and optimize your solutions to coding problems
- Explore advanced topics in computer science more effectively
- Develop a more intuitive understanding of algorithm efficiency and complexity
As you progress in your journey from beginner-level coding to preparing for technical interviews at major tech companies, remember that tools like Mathematica and SageMath are powerful allies. They can help you not only in solving specific problems but also in developing the kind of analytical and creative thinking that is highly valued in the tech industry.
Whether you’re exploring the intricacies of graph algorithms, diving into the world of cryptography, or tackling complex optimization problems, Computer Algebra Systems can provide you with insights and capabilities that go beyond traditional programming environments. By integrating these tools into your study routine, you’re not just preparing for interviews – you’re developing a deeper, more comprehensive understanding of computer science that will serve you well throughout your career.
So, as you continue your coding education journey, don’t hesitate to explore the world of Computer Algebra Systems. Experiment with Mathematica and SageMath, leverage their unique capabilities, and watch as they open up new dimensions in your understanding of algorithms and problem-solving. With these powerful tools at your disposal, you’ll be well-equipped to tackle even the most challenging coding interviews and thrive in the ever-evolving landscape of technology.