Mastering Computational Complexity Theory: A Comprehensive Guide for Aspiring Programmers
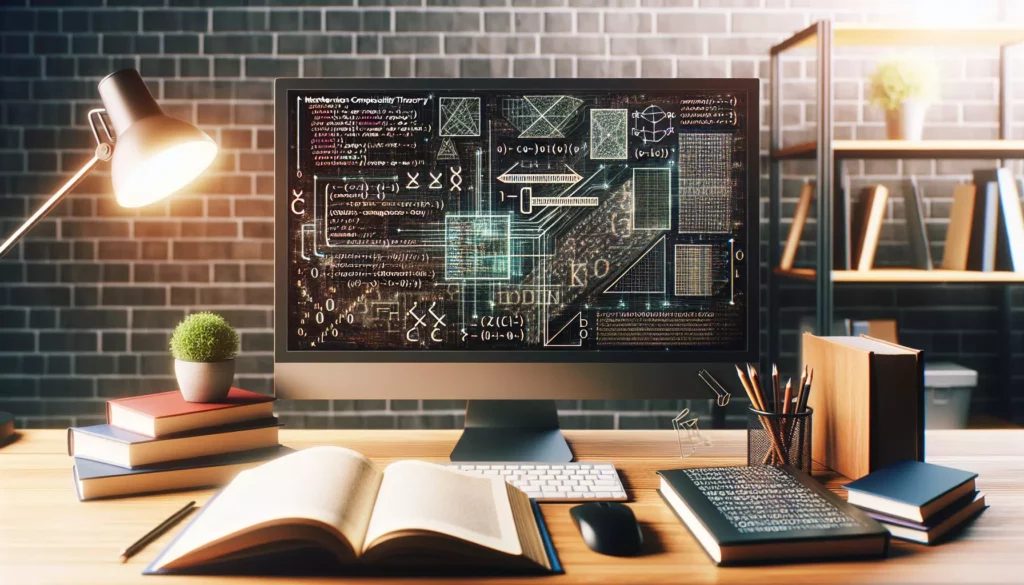
In the world of computer science and software engineering, understanding algorithms is crucial. However, equally important is the ability to analyze and evaluate these algorithms in terms of their efficiency and resource requirements. This is where Computational Complexity Theory comes into play. As an essential topic in AlgoCademy’s curriculum, mastering this concept will significantly enhance your coding skills and prepare you for technical interviews at top tech companies.
In this comprehensive guide, we’ll dive deep into Computational Complexity Theory, exploring its fundamental concepts, practical applications, and why it’s a critical skill for any aspiring programmer. Whether you’re a beginner just starting your coding journey or an experienced developer looking to sharpen your skills for FAANG interviews, this article will provide valuable insights and practical knowledge.
What is Computational Complexity Theory?
Computational Complexity Theory is a branch of computer science that focuses on classifying computational problems according to their inherent difficulty. It provides a framework for understanding how the resources required by an algorithm (such as time and space) scale with the size of the input.
The primary goals of Computational Complexity Theory are:
- To categorize problems based on their computational difficulty
- To establish relationships between different problem classes
- To determine the limits of what can be efficiently computed
By studying Computational Complexity Theory, programmers can make informed decisions about algorithm selection, optimize code performance, and understand the fundamental limitations of computational systems.
Key Concepts in Computational Complexity Theory
1. Time Complexity
Time complexity is a measure of how the running time of an algorithm increases with the size of the input. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s time requirement.
Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Here’s a simple example of an O(n) algorithm in Python:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
2. Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s often expressed using Big O notation.
Common space complexities include:
- O(1) – Constant space
- O(n) – Linear space
- O(n^2) – Quadratic space
Here’s an example of an algorithm with O(n) space complexity:
def create_square_array(n):
return [x**2 for x in range(n)]
3. Asymptotic Analysis
Asymptotic analysis is a method used to describe the running time or space requirements of an algorithm as the input size approaches infinity. This approach allows us to focus on the algorithm’s behavior for large inputs, ignoring constant factors and lower-order terms that become less significant as the input size grows.
4. Best, Average, and Worst-Case Complexity
When analyzing algorithms, we often consider three scenarios:
- Best-case: The minimum time/space required on any input of size n
- Average-case: The average time/space required over all inputs of size n
- Worst-case: The maximum time/space required on any input of size n
Understanding these different scenarios helps in making informed decisions about algorithm selection based on the expected input characteristics.
Complexity Classes
Computational Complexity Theory classifies problems into different complexity classes based on the resources required to solve them. Some important complexity classes include:
1. P (Polynomial Time)
The class P contains all decision problems that can be solved by a deterministic Turing machine in polynomial time. These are generally considered “efficiently solvable” problems.
Example: Determining if a number is prime (using the AKS primality test).
2. NP (Nondeterministic Polynomial Time)
NP includes all decision problems for which a solution can be verified in polynomial time. All problems in P are also in NP, but it’s unknown whether P = NP (one of the most famous open problems in computer science).
Example: The Boolean Satisfiability Problem (SAT).
3. NP-Complete
NP-Complete problems are the hardest problems in NP. If any NP-Complete problem could be solved in polynomial time, all problems in NP could be solved in polynomial time.
Example: The Traveling Salesman Problem.
4. NP-Hard
NP-Hard problems are at least as hard as the hardest problems in NP. They may or may not be in NP themselves.
Example: The Halting Problem.
Practical Applications of Computational Complexity Theory
Understanding Computational Complexity Theory has numerous practical applications in software development and problem-solving:
1. Algorithm Selection and Optimization
By analyzing the time and space complexity of different algorithms, developers can choose the most efficient solution for a given problem. This is particularly important when dealing with large datasets or resource-constrained environments.
For example, consider the problem of sorting an array. While bubble sort is simple to implement, it has a worst-case time complexity of O(n^2). For large arrays, a more efficient algorithm like quicksort, with an average-case complexity of O(n log n), would be a better choice.
2. Scalability Analysis
Complexity analysis helps in predicting how an algorithm or system will perform as the input size or user base grows. This is crucial for designing scalable applications and services.
For instance, if you’re developing a social media platform, you need to consider how your algorithms for generating news feeds or suggesting friends will scale as the number of users increases.
3. Resource Allocation
Understanding the space and time requirements of algorithms allows for better resource allocation in systems design. This is particularly important in cloud computing environments where resources are directly tied to costs.
4. Cryptography and Security
Many cryptographic systems rely on the assumed hardness of certain computational problems. For example, the security of RSA encryption is based on the difficulty of factoring large numbers, a problem believed to be computationally hard.
5. Machine Learning and AI
In the field of artificial intelligence and machine learning, complexity theory helps in understanding the limitations and capabilities of learning algorithms. It provides insights into questions like: How much data and computation time is needed to learn a particular concept?
Techniques for Analyzing Algorithm Complexity
To effectively apply Computational Complexity Theory, it’s essential to master various techniques for analyzing algorithm complexity. Here are some key approaches:
1. Counting Primitive Operations
This technique involves counting the number of basic operations (like arithmetic operations, comparisons, and assignments) performed by an algorithm. While it provides a detailed analysis, it can be time-consuming for complex algorithms.
Example:
def sum_array(arr):
total = 0 # 1 operation
for i in range(len(arr)): # n + 1 operations (n iterations + 1 final check)
total += arr[i] # 2 operations per iteration (addition and assignment)
return total # 1 operation
# Total: 1 + (n + 1) + 2n + 1 = 3n + 3 operations
# Time complexity: O(n)
2. Analyzing Loops
For algorithms with loops, we often focus on the loop structure to determine complexity. The basic rules are:
- Simple loops: O(n) where n is the number of iterations
- Nested loops: Multiply the complexities of each loop
- Consecutive loops: Add the complexities
Example of nested loops:
def print_pairs(arr):
for i in range(len(arr)): # O(n)
for j in range(len(arr)): # O(n)
print(arr[i], arr[j])
# Time complexity: O(n) * O(n) = O(n^2)
3. Recurrence Relations
For recursive algorithms, we often use recurrence relations to describe the complexity. These can then be solved using techniques like the Master Theorem or recursion trees.
Example: Analyzing the time complexity of the Merge Sort algorithm
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
# Recurrence relation: T(n) = 2T(n/2) + O(n)
# Solving this using the Master Theorem gives us O(n log n)
4. Amortized Analysis
Amortized analysis is used when the worst-case time complexity of a single operation is not representative of the algorithm’s overall performance. It considers the average cost per operation over a sequence of operations.
A classic example is the dynamic array (like ArrayList in Java or list in Python). While a single append operation might occasionally require O(n) time to resize the array, the amortized time complexity of append is O(1).
Common Pitfalls in Complexity Analysis
While analyzing algorithm complexity, there are several common mistakes to avoid:
1. Ignoring Constants and Lower-Order Terms
While Big O notation allows us to ignore constants and lower-order terms, it’s important to remember that these can still have significant impacts in practice, especially for smaller input sizes.
2. Focusing Only on Time Complexity
While time complexity is often the primary concern, space complexity can be equally important, especially in memory-constrained environments.
3. Overlooking Average-Case Analysis
Many algorithms have different best-case, average-case, and worst-case complexities. Focusing solely on the worst-case scenario might lead to suboptimal choices in practice.
4. Neglecting the Impact of Data Structures
The choice of data structure can significantly impact an algorithm’s complexity. For example, using a hash table instead of an array can often reduce search operations from O(n) to O(1).
Advanced Topics in Computational Complexity Theory
As you progress in your understanding of Computational Complexity Theory, you may encounter more advanced topics:
1. Approximation Algorithms
For NP-hard problems, where finding an optimal solution in polynomial time is believed to be impossible, approximation algorithms aim to find near-optimal solutions efficiently.
2. Randomized Algorithms
These algorithms use random numbers to guide their execution. They can often solve problems more efficiently than deterministic algorithms, but introduce a small probability of error.
3. Parallel and Distributed Algorithms
With the rise of multi-core processors and distributed systems, understanding how algorithms perform in parallel or distributed environments is increasingly important.
4. Quantum Complexity Theory
This field studies the computational complexity of problems that can be solved by quantum computers, potentially offering exponential speedups for certain problems.
Preparing for Technical Interviews
Understanding Computational Complexity Theory is crucial for technical interviews, especially at top tech companies. Here are some tips to help you prepare:
1. Practice Analyzing Various Algorithms
Regularly practice analyzing the time and space complexity of different algorithms. Start with simple algorithms and gradually move to more complex ones.
2. Solve Problems with Efficiency in Mind
When solving coding problems, don’t just focus on correctness. Always consider the efficiency of your solution and try to optimize it.
3. Understand Trade-offs
Be prepared to discuss trade-offs between time and space complexity. Sometimes, you can achieve better time complexity at the cost of increased space usage, or vice versa.
4. Learn to Explain Your Analysis
Practice explaining your complexity analysis clearly and concisely. Interviewers often want to understand your thought process, not just the final answer.
5. Study Common Algorithms and Data Structures
Familiarize yourself with the complexity of common algorithms (sorting, searching, graph algorithms) and data structures (arrays, linked lists, trees, hash tables).
Conclusion
Computational Complexity Theory is a fundamental concept in computer science that plays a crucial role in algorithm design, system optimization, and problem-solving. By mastering this topic, you’ll be better equipped to develop efficient solutions, make informed decisions about algorithm selection, and excel in technical interviews.
Remember, understanding complexity is not just about memorizing Big O notations. It’s about developing a intuition for how algorithms scale and behave with different inputs. As you continue your journey with AlgoCademy, make sure to apply these concepts in your coding practice and problem-solving endeavors.
Keep challenging yourself with increasingly complex problems, and don’t hesitate to dive into the more advanced topics as you progress. With dedication and practice, you’ll develop the skills necessary to tackle even the most challenging computational problems and shine in technical interviews at top tech companies.
Happy coding, and may your algorithms always be efficient!