Mastering Common Prefix Problems: Techniques and Strategies for Efficient Solutions
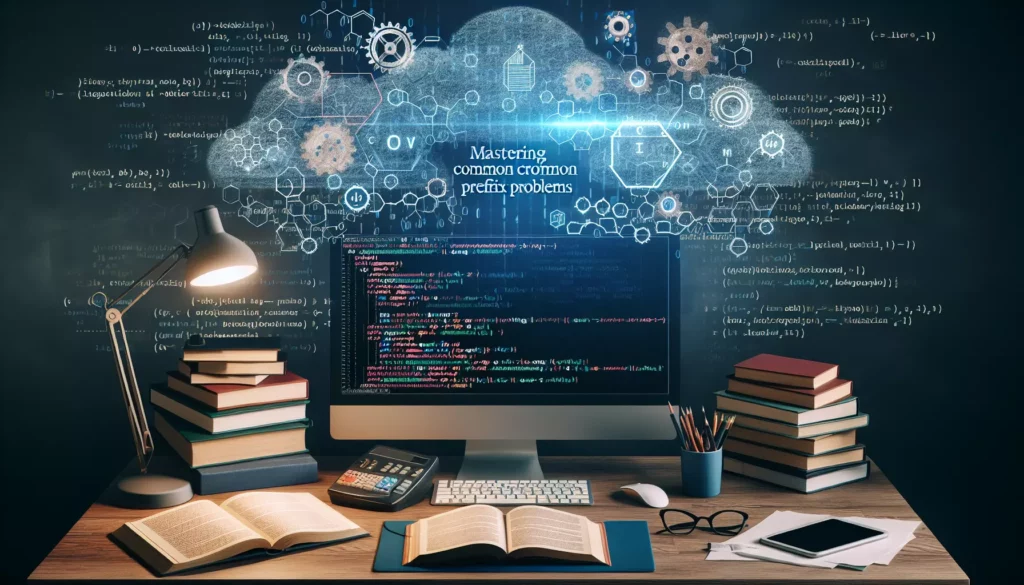
In the world of coding interviews and algorithmic problem-solving, common prefix problems are a frequent occurrence. These problems involve finding the longest common prefix among a set of strings, which can be crucial in various applications such as autocomplete features, spell checkers, and data compression algorithms. In this comprehensive guide, we’ll explore various techniques for solving common prefix problems, providing you with the tools and knowledge to tackle these challenges effectively.
Understanding Common Prefix Problems
Before diving into the techniques, let’s first understand what common prefix problems are and why they’re important:
- Definition: A common prefix is the longest string of characters that appears at the beginning of all strings in a given set.
- Example: For the strings [“flower”, “flow”, “flight”], the longest common prefix is “fl”.
- Importance: Solving these problems efficiently is crucial for optimizing various string-based algorithms and applications.
Technique 1: Horizontal Scanning
Horizontal scanning is a straightforward approach to solving common prefix problems. Here’s how it works:
- Take the first string as the initial prefix.
- Iterate through the remaining strings, comparing them with the current prefix.
- Update the prefix by removing characters from the end that don’t match.
- Repeat until all strings are processed or the prefix becomes empty.
Let’s implement this technique in Python:
def longest_common_prefix(strs):
if not strs:
return ""
prefix = strs[0]
for i in range(1, len(strs)):
while strs[i].find(prefix) != 0:
prefix = prefix[:-1]
if not prefix:
return ""
return prefix
# Example usage
strings = ["flower", "flow", "flight"]
result = longest_common_prefix(strings)
print(f"The longest common prefix is: {result}")
This approach has a time complexity of O(S), where S is the sum of all characters in the array. In the worst case, all n strings are the same.
Technique 2: Vertical Scanning
Vertical scanning is another effective method for solving common prefix problems. Here’s the process:
- Compare characters from each string at the same index.
- Start with the first character of each string and move right.
- Stop when a mismatch is found or when the end of a string is reached.
Here’s a Python implementation of the vertical scanning technique:
def longest_common_prefix(strs):
if not strs:
return ""
for i in range(len(strs[0])):
char = strs[0][i]
for j in range(1, len(strs)):
if i == len(strs[j]) or strs[j][i] != char:
return strs[0][:i]
return strs[0]
# Example usage
strings = ["flower", "flow", "flight"]
result = longest_common_prefix(strings)
print(f"The longest common prefix is: {result}")
The time complexity of this approach is O(S), where S is the sum of all characters in the array. In the worst case, the algorithm compares all characters of all strings.
Technique 3: Divide and Conquer
The divide and conquer approach is a more advanced technique that can be particularly useful for large datasets. Here’s how it works:
- Divide the array of strings into two halves.
- Recursively find the common prefix for each half.
- Find the common prefix of the results from step 2.
Let’s implement this technique in Python:
def longest_common_prefix(strs):
if not strs:
return ""
def common_prefix(left, right):
min_len = min(len(left), len(right))
for i in range(min_len):
if left[i] != right[i]:
return left[:i]
return left[:min_len]
def divide_and_conquer(low, high):
if low == high:
return strs[low]
mid = (low + high) // 2
left_prefix = divide_and_conquer(low, mid)
right_prefix = divide_and_conquer(mid + 1, high)
return common_prefix(left_prefix, right_prefix)
return divide_and_conquer(0, len(strs) - 1)
# Example usage
strings = ["flower", "flow", "flight"]
result = longest_common_prefix(strings)
print(f"The longest common prefix is: {result}")
The time complexity of this approach is O(S * log(n)), where S is the sum of all characters in the array, and n is the number of strings. This can be more efficient for large datasets compared to the previous methods.
Technique 4: Binary Search
Binary search can be applied to common prefix problems by leveraging the fact that the common prefix must be a prefix of the shortest string in the array. Here’s how it works:
- Find the minimum length string in the array.
- Perform binary search on the length of this string.
- For each mid-point, check if all strings have the same prefix of that length.
- Adjust the search range based on the result.
Here’s a Python implementation of the binary search approach:
def longest_common_prefix(strs):
if not strs:
return ""
def is_common_prefix(length):
prefix = strs[0][:length]
return all(s.startswith(prefix) for s in strs)
min_len = min(len(s) for s in strs)
low, high = 0, min_len
while low <= high:
mid = (low + high) // 2
if is_common_prefix(mid):
low = mid + 1
else:
high = mid - 1
return strs[0][:high]
# Example usage
strings = ["flower", "flow", "flight"]
result = longest_common_prefix(strings)
print(f"The longest common prefix is: {result}")
The time complexity of this approach is O(S * log(m)), where S is the sum of all characters in the array, and m is the length of the shortest string. This can be more efficient when dealing with very long strings.
Technique 5: Trie Data Structure
Using a Trie (prefix tree) is an advanced technique that can be very efficient for solving common prefix problems, especially when dealing with a large number of strings or when you need to perform multiple prefix queries. Here’s how it works:
- Build a Trie from all the strings in the array.
- Traverse the Trie from the root, following the common path.
- Stop when a node has more than one child or when we reach the end of a string.
Let’s implement this technique using Python:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def longest_common_prefix(self):
node = self.root
prefix = []
while len(node.children) == 1 and not node.is_end:
char = next(iter(node.children))
prefix.append(char)
node = node.children[char]
return ''.join(prefix)
def longest_common_prefix(strs):
if not strs:
return ""
trie = Trie()
for s in strs:
trie.insert(s)
return trie.longest_common_prefix()
# Example usage
strings = ["flower", "flow", "flight"]
result = longest_common_prefix(strings)
print(f"The longest common prefix is: {result}")
The time complexity for building the Trie is O(S), where S is the sum of all characters in the array. Finding the longest common prefix takes O(m) time, where m is the length of the longest common prefix. This approach can be very efficient, especially when you need to perform multiple prefix queries on the same set of strings.
Optimizing Your Approach
When solving common prefix problems, consider the following optimizations:
- Early termination: Stop processing as soon as you find a mismatch or reach the end of the shortest string.
- Sorting: For some approaches, sorting the strings lexicographically first can lead to optimizations.
- Caching: If you’re performing multiple queries on the same dataset, consider caching results.
- Parallel processing: For very large datasets, consider parallelizing the comparison process.
Common Variations and Extensions
As you progress in your coding journey, you may encounter variations of the common prefix problem. Here are some examples:
- Longest Common Suffix: Find the longest common ending among a set of strings.
- Longest Common Substring: Find the longest string that is a substring of all strings in the set.
- K-Common Prefix: Find the longest prefix that is common to at least K strings in the set.
- Dynamic Common Prefix: Maintain the common prefix as strings are added or removed from the set.
These variations often require adapting the techniques we’ve discussed or combining them with other algorithms.
Real-World Applications
Understanding and efficiently solving common prefix problems has numerous practical applications:
- Autocomplete systems: Suggesting completions for partially typed words or phrases.
- File systems: Organizing and searching for files with similar names or paths.
- DNA sequence analysis: Finding common sequences in genetic data.
- IP routing: Optimizing network routing tables based on common address prefixes.
- Data compression: Identifying repeating patterns for efficient data storage.
Practice and Improvement
To master common prefix problems and related techniques, consider the following steps:
- Practice regularly: Solve a variety of common prefix problems on coding platforms like LeetCode, HackerRank, or CodeSignal.
- Analyze time and space complexity: For each solution you develop, assess its efficiency and consider ways to optimize it.
- Implement multiple approaches: Try solving the same problem using different techniques to understand their trade-offs.
- Review and learn from others: Study efficient solutions proposed by others and understand their reasoning.
- Apply to real-world scenarios: Try to identify and solve common prefix problems in your own projects or work.
Conclusion
Common prefix problems are a fundamental concept in string manipulation and algorithm design. By mastering the techniques we’ve explored – horizontal scanning, vertical scanning, divide and conquer, binary search, and using Trie data structures – you’ll be well-equipped to tackle a wide range of string-related challenges in coding interviews and real-world applications.
Remember that the best approach often depends on the specific problem constraints, input characteristics, and performance requirements. As you practice and gain experience, you’ll develop the intuition to choose the most appropriate technique for each situation.
Keep honing your skills, exploring new variations, and applying these concepts to diverse problems. With dedication and practice, you’ll not only excel at solving common prefix problems but also enhance your overall problem-solving abilities in the world of algorithms and data structures.