Mastering Combination Problems: Strategies for Efficient Problem-Solving
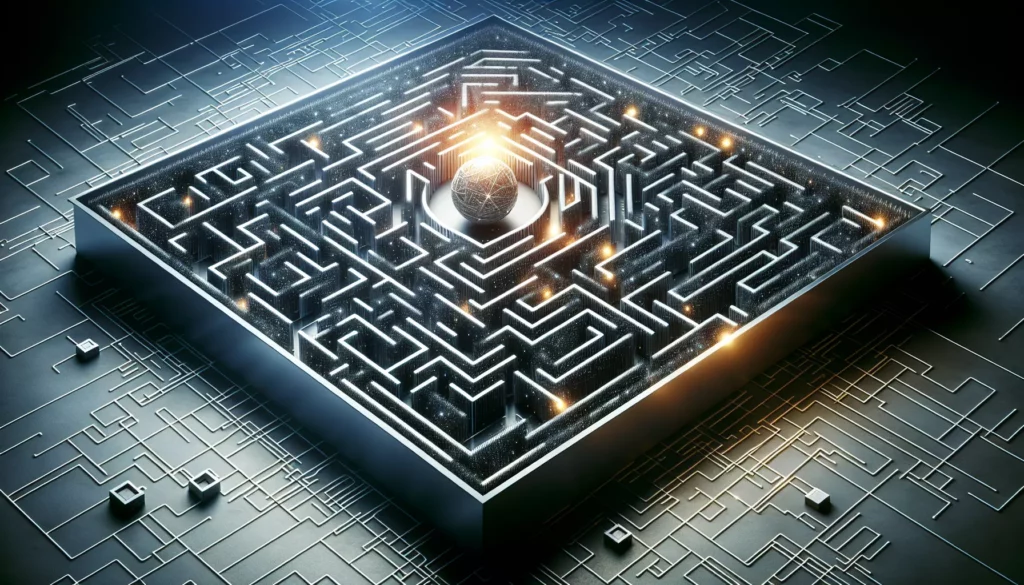
In the realm of coding interviews and algorithmic problem-solving, combination problems stand out as a crucial topic that often challenges even seasoned programmers. These problems, which involve selecting or arranging elements from a set in various ways, are not only common in technical interviews but also fundamental to many real-world applications. In this comprehensive guide, we’ll explore effective strategies for tackling combination problems, providing you with the tools you need to approach these challenges with confidence.
Understanding Combination Problems
Before diving into strategies, it’s essential to understand what combination problems are and why they’re important in the world of programming and computer science.
What are Combination Problems?
Combination problems involve selecting a subset of items from a larger set, where the order of selection doesn’t matter. For example, choosing 3 items from a set of 5 is a combination problem. These problems are distinct from permutation problems, where the order does matter.
Why are They Important?
Combination problems are crucial for several reasons:
- They form the basis of many advanced algorithms and data structures
- They’re frequently used in optimization problems
- Understanding combinations is essential for probability and statistics in computer science
- They’re a common type of question in coding interviews, especially for tech giants like FAANG companies
Fundamental Concepts
Before we delve into specific strategies, let’s review some fundamental concepts that will be crucial in solving combination problems.
The Combination Formula
The number of ways to choose k items from a set of n items is given by the combination formula:
C(n,k) = n! / (k! * (n-k)!)
Where n! represents the factorial of n.
Pascal’s Triangle
Pascal’s Triangle is a triangular array of the binomial coefficients that arises in probability theory, combinatorics, and algebra. Each number is the sum of the two numbers directly above it.
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
This triangle is incredibly useful for solving combination problems, as each number represents C(n,k) where n is the row number (starting from 0) and k is the position in the row (also starting from 0).
Strategy 1: Recursive Approach
One of the most intuitive ways to solve combination problems is through recursion. This approach breaks down the problem into smaller subproblems, solving them recursively until reaching a base case.
The Recursive Formula
The recursive formula for combinations is:
C(n,k) = C(n-1,k-1) + C(n-1,k)
This formula is based on the idea that for any element, we have two choices: either include it in our combination or exclude it.
Implementing the Recursive Approach
Here’s a Python implementation of the recursive approach:
def combination(n, k):
if k == 0 or k == n:
return 1
return combination(n-1, k-1) + combination(n-1, k)
# Example usage
print(combination(5, 2)) # Output: 10
Pros and Cons
Pros:
- Intuitive and easy to understand
- Directly reflects the mathematical definition
Cons:
- Can be inefficient for large values of n and k due to repeated calculations
- May lead to stack overflow for very large inputs
Strategy 2: Dynamic Programming
Dynamic Programming (DP) is a powerful technique that can significantly improve the efficiency of our solution, especially for larger inputs.
The DP Approach
The idea is to store the results of subproblems in a table (usually a 2D array) to avoid redundant calculations. This approach is also known as memoization.
Implementing the DP Approach
Here’s a Python implementation using dynamic programming:
def combination_dp(n, k):
dp = [[0 for _ in range(k+1)] for _ in range(n+1)]
for i in range(n+1):
for j in range(min(i, k)+1):
if j == 0 or j == i:
dp[i][j] = 1
else:
dp[i][j] = dp[i-1][j-1] + dp[i-1][j]
return dp[n][k]
# Example usage
print(combination_dp(5, 2)) # Output: 10
Pros and Cons
Pros:
- Much more efficient than the recursive approach for larger inputs
- Avoids stack overflow issues
Cons:
- Requires additional space for the DP table
- May be overkill for small inputs
Strategy 3: Iterative Approach
An iterative approach can sometimes be the most straightforward and efficient way to solve combination problems, especially when we only need to calculate a single combination value.
The Iterative Formula
We can calculate C(n,k) iteratively using the following formula:
C(n,k) = (n * (n-1) * ... * (n-k+1)) / (k * (k-1) * ... * 1)
Implementing the Iterative Approach
Here’s a Python implementation of the iterative approach:
def combination_iterative(n, k):
if k > n - k:
k = n - k
result = 1
for i in range(k):
result *= (n - i)
result //= (i + 1)
return result
# Example usage
print(combination_iterative(5, 2)) # Output: 10
Pros and Cons
Pros:
- Very efficient in terms of both time and space complexity
- Easy to implement and understand
Cons:
- May encounter overflow issues for very large inputs
- Not as flexible as the DP approach for calculating multiple combinations
Strategy 4: Using Built-in Libraries
Many programming languages provide built-in libraries or modules that include functions for calculating combinations. While it’s crucial to understand the underlying concepts, using these libraries can be very convenient and efficient in practice.
Python’s math.comb()
In Python 3.8 and later, you can use the math.comb()
function:
import math
# Example usage
print(math.comb(5, 2)) # Output: 10
Other Languages
Other languages have similar functions:
- C++:
std::binomial_coefficient
(since C++17) - Java: No built-in function, but libraries like Apache Commons Math provide this functionality
- JavaScript: No built-in function, but libraries like mathjs provide combination calculations
Pros and Cons
Pros:
- Extremely convenient and usually very efficient
- Handles edge cases and large inputs well
Cons:
- May not be available in all programming languages or versions
- Doesn’t provide insight into the underlying algorithm, which may be important in interview settings
Advanced Techniques
While the strategies we’ve discussed so far cover most combination problems you’re likely to encounter, there are some advanced techniques that can be useful in specific scenarios or for optimizing solutions further.
Lucas’ Theorem
Lucas’ Theorem is particularly useful when dealing with very large numbers and when working with modular arithmetic. It states that for prime p:
C(n,k) ≡ ΠC(ni,ki) (mod p)
Where ni and ki are the digits of n and k in base p representation.
Generating Combinations
Sometimes, instead of just calculating the number of combinations, you need to generate all possible combinations. Here’s a Python function that generates all combinations of k elements from a list:
def generate_combinations(elements, k):
if k == 0:
yield []
elif k > len(elements):
return
else:
for i in range(len(elements)):
for combo in generate_combinations(elements[i+1:], k-1):
yield [elements[i]] + combo
# Example usage
elements = [1, 2, 3, 4, 5]
k = 3
for combo in generate_combinations(elements, k):
print(combo)
Bit Manipulation
Bit manipulation can be an efficient way to generate combinations, especially when working with small sets. Here’s an example of how to generate all combinations using bit manipulation:
def bit_combinations(n, k):
# Generate all numbers from 0 to 2^n - 1
for i in range(1 << n):
# If the number of set bits is k, it's a valid combination
if bin(i).count('1') == k:
combination = []
for j in range(n):
if i & (1 << j):
combination.append(j)
yield combination
# Example usage
n, k = 5, 3
for combo in bit_combinations(n, k):
print(combo)
Common Pitfalls and How to Avoid Them
When solving combination problems, there are several common pitfalls that programmers often encounter. Being aware of these can help you avoid errors and optimize your solutions.
1. Integer Overflow
When calculating combinations with large numbers, integer overflow can occur. To avoid this:
- Use languages or data types that support arbitrary-precision arithmetic (like Python’s built-in integers)
- Implement modular arithmetic if working with a specific modulus
- Use logarithms and exponentiation for very large numbers
2. Confusing Combinations and Permutations
Remember that combinations don’t care about order, while permutations do. Make sure you’re using the correct formula for your problem.
3. Inefficient Implementations
Naive recursive implementations can be very slow for large inputs. Always consider using dynamic programming or iterative approaches for better efficiency.
4. Off-by-One Errors
Be careful with your loop bounds and base cases. Off-by-one errors are common in combination problems, especially when dealing with zero-based indexing.
5. Not Considering Edge Cases
Always consider edge cases like n = k, k = 0, or n = 0. These often have simple answers (1, 1, and 0 respectively for C(n,k)) but can cause errors if not handled properly.
Real-World Applications
Understanding and being able to solve combination problems isn’t just about passing technical interviews. These concepts have numerous real-world applications across various domains of computer science and beyond.
1. Cryptography
Combination problems play a crucial role in cryptography, particularly in areas like:
- Key generation: The strength of many cryptographic systems relies on the large number of possible combinations for keys
- Cryptanalysis: Understanding combinations is crucial for analyzing the security of cryptographic systems
2. Network Design
In network design and analysis, combinations are used for:
- Calculating the number of possible connections in a network
- Analyzing network reliability and redundancy
3. Machine Learning and Data Science
Combination problems arise frequently in machine learning and data science:
- Feature selection: Choosing the best subset of features from a larger set
- Ensemble methods: Combining multiple models or datasets
- Cross-validation: Selecting subsets of data for training and testing
4. Bioinformatics
In bioinformatics, combinations are used for:
- Analyzing genetic sequences
- Protein folding predictions
- Studying biodiversity and species interactions
5. Operations Research
Combination problems are fundamental to many optimization problems in operations research, such as:
- Resource allocation
- Scheduling problems
- Portfolio optimization in finance
Practice Problems
To reinforce your understanding and skills in solving combination problems, here are some practice problems of varying difficulty:
Easy
- Calculate C(10,3)
- How many ways are there to choose 2 cards from a standard 52-card deck?
- Implement a function to calculate C(n,k) using the recursive formula
Medium
- Implement a function to generate all combinations of k elements from a list of n elements
- Calculate the sum of all elements in the 10th row of Pascal’s Triangle
- Find the number of ways to select 3 distinct numbers from the range 1 to 20 such that their sum is even
Hard
- Implement Lucas’ Theorem to calculate C(n,k) mod p, where p is prime
- Given an array of integers and an integer k, find the number of subarrays with exactly k odd numbers
- Calculate C(10^18, 10^9) mod (10^9 + 7)
Conclusion
Mastering combination problems is a crucial skill for any programmer or computer scientist. These problems not only frequently appear in technical interviews, especially for prestigious tech companies, but also have wide-ranging applications in various fields of computer science and beyond.
We’ve explored several strategies for solving combination problems, from basic recursive approaches to more advanced techniques like dynamic programming and bit manipulation. We’ve also discussed common pitfalls to avoid and provided examples of real-world applications.
Remember, the key to mastering these problems is practice. Start with simpler problems and gradually work your way up to more complex ones. Don’t be discouraged if you find some problems challenging at first – with persistence and practice, you’ll develop the skills and intuition needed to tackle even the most difficult combination problems.
As you continue your journey in programming and computer science, keep in mind that understanding combinations is just one piece of the puzzle. It often interplays with other important concepts like recursion, dynamic programming, and algorithmic complexity. By building a strong foundation in these fundamental areas, you’ll be well-equipped to tackle a wide range of problems in your studies, interviews, and professional career.
Happy coding, and may the combinations be ever in your favor!