Mastering Coding Logic and Reasoning: A Comprehensive Guide to Leveling Up Your Programming Skills
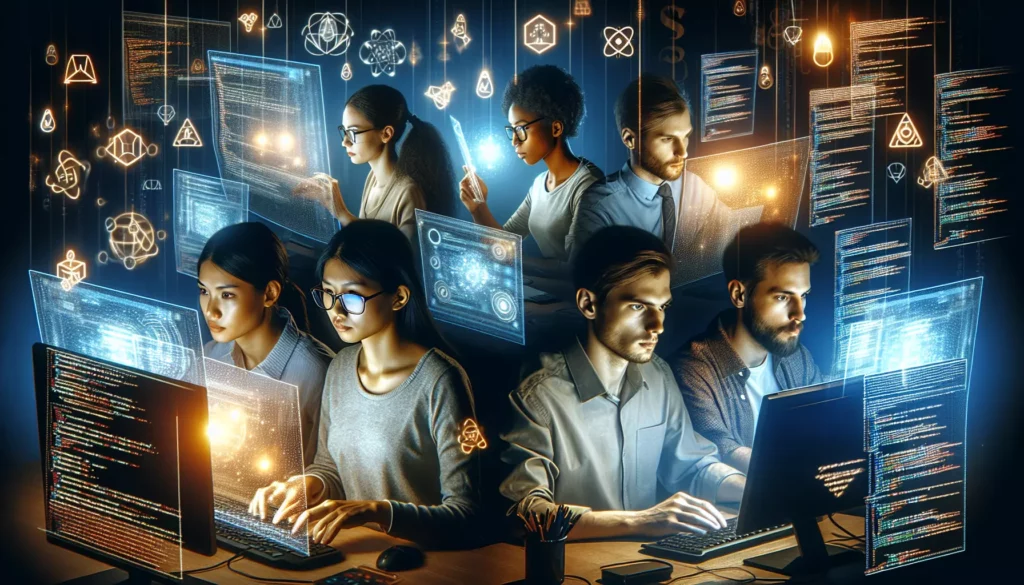
In the ever-evolving world of technology, coding has become an essential skill for many professionals. Whether you’re a beginner just starting your journey or an experienced developer looking to sharpen your skills, improving your coding logic and reasoning abilities is crucial for success. This comprehensive guide will explore various strategies, techniques, and resources to help you enhance your problem-solving capabilities and become a more efficient programmer.
Understanding the Importance of Coding Logic and Reasoning
Before diving into the methods for improvement, it’s essential to understand why coding logic and reasoning skills are so vital in programming. These skills form the foundation of your ability to:
- Analyze complex problems and break them down into manageable components
- Develop efficient algorithms and data structures
- Debug and troubleshoot code effectively
- Write clean, maintainable, and scalable code
- Adapt to new programming languages and technologies quickly
- Excel in technical interviews and coding challenges
By focusing on enhancing these skills, you’ll not only become a better programmer but also increase your value in the job market, especially when targeting positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
Strategies to Improve Your Coding Logic and Reasoning Skills
1. Practice, Practice, Practice
The old adage “practice makes perfect” holds true for coding as well. Regular practice is one of the most effective ways to improve your coding logic and reasoning skills. Here are some ways to incorporate practice into your routine:
- Solve coding challenges: Platforms like LeetCode, HackerRank, and CodeWars offer a wide range of programming problems to solve, from easy to advanced levels.
- Participate in coding competitions: Websites like Codeforces and TopCoder host regular coding contests that can help you improve your problem-solving skills under time pressure.
- Work on personal projects: Building your own applications or tools can help you apply your skills in real-world scenarios and learn from hands-on experience.
- Contribute to open-source projects: Collaborating with other developers on open-source projects can expose you to different coding styles and problem-solving approaches.
2. Study Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for developing strong coding logic and reasoning skills. These fundamental concepts form the building blocks of efficient problem-solving in programming. Here’s how you can approach this study:
- Learn the basics: Start with fundamental data structures like arrays, linked lists, stacks, queues, and trees. Understand their properties, use cases, and implementation details.
- Master common algorithms: Study sorting algorithms (e.g., bubble sort, merge sort, quicksort), searching algorithms (e.g., binary search), and graph algorithms (e.g., breadth-first search, depth-first search).
- Analyze time and space complexity: Learn to evaluate the efficiency of your code using Big O notation and understand the trade-offs between different approaches.
- Implement from scratch: Try implementing data structures and algorithms from scratch to deepen your understanding of their inner workings.
Resources like “Introduction to Algorithms” by Cormen et al. and online courses on platforms like Coursera and edX can provide structured learning paths for these topics.
3. Develop a Problem-Solving Mindset
Improving your coding logic and reasoning skills isn’t just about writing code; it’s about developing a systematic approach to problem-solving. Here are some techniques to cultivate this mindset:
- Break down problems: When faced with a complex problem, break it down into smaller, more manageable sub-problems. This approach, known as “divide and conquer,” can make seemingly insurmountable challenges more approachable.
- Plan before coding: Before jumping into writing code, take the time to plan your approach. Sketch out flowcharts, write pseudocode, or use comments to outline your solution strategy.
- Consider multiple solutions: Don’t settle for the first solution that comes to mind. Try to think of alternative approaches and evaluate their pros and cons before implementing.
- Learn from others: After solving a problem, look at other people’s solutions. This can expose you to different thinking patterns and more efficient algorithms.
4. Enhance Your Analytical Skills
Strong analytical skills are crucial for effective coding and problem-solving. Here are some ways to sharpen your analytical abilities:
- Practice logical reasoning: Solve logic puzzles, riddles, and brain teasers to improve your ability to think critically and analytically.
- Study discrete mathematics: Concepts from discrete math, such as set theory, combinatorics, and graph theory, can significantly enhance your problem-solving capabilities in programming.
- Analyze existing code: Read and analyze well-written code from open-source projects or experienced developers. Try to understand the reasoning behind their design decisions and implementation choices.
- Debug systematically: When debugging, approach the process systematically by forming hypotheses, testing them, and analyzing the results. This structured approach can improve your analytical thinking.
5. Learn Multiple Programming Paradigms
Exposing yourself to different programming paradigms can broaden your perspective and improve your problem-solving skills. Each paradigm offers unique approaches to structuring and organizing code. Consider exploring:
- Object-Oriented Programming (OOP): Learn to design and implement programs using objects and classes.
- Functional Programming: Understand the principles of immutability and pure functions, and how they can lead to more predictable and maintainable code.
- Procedural Programming: Master the basics of writing code as a sequence of instructions and function calls.
- Declarative Programming: Explore languages and frameworks that allow you to describe what you want to achieve rather than specifying exact steps.
By understanding these different paradigms, you’ll be better equipped to choose the most appropriate approach for solving various programming problems.
6. Utilize Interactive Learning Platforms
Interactive learning platforms can provide structured paths to improve your coding skills while offering immediate feedback and guidance. AlgoCademy, for instance, offers a range of features designed to enhance your coding logic and reasoning:
- Step-by-step tutorials: Learn complex concepts through bite-sized, interactive lessons.
- AI-powered assistance: Get personalized help and explanations as you work through coding challenges.
- Practice problems: Solve a variety of coding problems designed to improve your algorithmic thinking and problem-solving skills.
- Progress tracking: Monitor your improvement over time and identify areas that need more focus.
Other platforms like Codecademy, freeCodeCamp, and Educative.io also offer interactive coding environments and structured courses to help you improve your skills.
7. Implement Code Reviews and Pair Programming
Collaborating with other developers can significantly enhance your coding logic and reasoning skills. Two effective methods for this are:
- Code reviews: Regularly participate in code reviews, both as a reviewer and as someone whose code is being reviewed. This process exposes you to different coding styles and problem-solving approaches while also helping you learn to critically evaluate code quality and efficiency.
- Pair programming: Work alongside another developer in real-time, taking turns to write code and provide feedback. This collaborative approach can help you learn new techniques, catch errors early, and improve your ability to explain your thought process.
8. Study Design Patterns and Best Practices
Familiarizing yourself with common design patterns and industry best practices can elevate your coding skills and help you write more efficient, maintainable code. Consider the following:
- Design patterns: Learn about common patterns like Singleton, Factory, Observer, and Strategy. Understand when and how to apply them in your code.
- SOLID principles: Study and apply the SOLID principles of object-oriented design to create more flexible and maintainable software.
- Clean Code practices: Read books like “Clean Code” by Robert C. Martin to learn techniques for writing readable, understandable, and maintainable code.
- Refactoring techniques: Learn how to improve the structure of existing code without changing its external behavior.
9. Engage in Coding Challenges and Competitions
Participating in coding challenges and competitions can be an exciting way to improve your skills while testing yourself against others. These events often present unique, complex problems that require creative thinking and efficient problem-solving. Some popular platforms and events include:
- Google Code Jam: An annual coding competition hosted by Google, featuring multiple rounds of algorithmic problems.
- Facebook Hacker Cup: A global programming competition organized by Facebook, focusing on solving algorithmic challenges.
- ACM International Collegiate Programming Contest (ICPC): A team-based algorithmic programming contest for college students.
- Advent of Code: An annual set of Christmas-themed programming puzzles that can be solved in any programming language.
These competitions not only help you improve your coding skills but also expose you to the types of problems often asked in technical interviews at major tech companies.
10. Teach and Explain Concepts to Others
One of the most effective ways to solidify your understanding of coding concepts and improve your ability to reason about them is to teach others. This approach, often referred to as the “Feynman Technique,” involves explaining complex ideas in simple terms. You can do this by:
- Writing blog posts or tutorials: Start a coding blog where you explain concepts you’ve learned or solutions to problems you’ve solved.
- Creating video tutorials: Make YouTube videos or screencasts demonstrating coding techniques or explaining algorithms.
- Mentoring junior developers: Offer to mentor less experienced programmers, either in your workplace or through online communities.
- Answering questions on forums: Participate in Q&A sites like Stack Overflow or Reddit’s programming subreddits to help others with their coding problems.
By teaching others, you’ll often find gaps in your own understanding, which can motivate you to learn more deeply and improve your ability to articulate complex ideas.
Practical Exercises to Enhance Your Coding Logic
To help you get started on improving your coding logic and reasoning skills, here are some practical exercises you can try:
1. Implement a Sorting Algorithm
Try implementing a sorting algorithm from scratch, such as quicksort or merge sort. This exercise will help you understand how these fundamental algorithms work and improve your ability to manipulate data structures.
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Test the function
unsorted_list = [3, 6, 8, 10, 1, 2, 1]
print(quick_sort(unsorted_list)) # Output: [1, 1, 2, 3, 6, 8, 10]
2. Solve a Dynamic Programming Problem
Dynamic programming is a powerful technique for solving complex problems by breaking them down into simpler subproblems. Try solving a classic dynamic programming problem like the Fibonacci sequence or the Longest Common Subsequence.
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Test the function
print(fibonacci(10)) # Output: 55
3. Implement a Data Structure
Choose a data structure you’re less familiar with, such as a binary search tree or a hash table, and implement it from scratch. This exercise will deepen your understanding of how these fundamental structures work and when to use them.
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, key):
self.root = self._insert_recursive(self.root, key)
def _insert_recursive(self, root, key):
if root is None:
return Node(key)
if key < root.val:
root.left = self._insert_recursive(root.left, key)
else:
root.right = self._insert_recursive(root.right, key)
return root
def inorder_traversal(self):
self._inorder_recursive(self.root)
def _inorder_recursive(self, root):
if root:
self._inorder_recursive(root.left)
print(root.val, end=" ")
self._inorder_recursive(root.right)
# Test the BST
bst = BinarySearchTree()
bst.insert(50)
bst.insert(30)
bst.insert(20)
bst.insert(40)
bst.insert(70)
bst.insert(60)
bst.insert(80)
print("Inorder traversal of the BST:")
bst.inorder_traversal() # Output: 20 30 40 50 60 70 80
Conclusion
Improving your coding logic and reasoning skills is a continuous journey that requires dedication, practice, and a willingness to learn. By incorporating the strategies and exercises outlined in this guide, you can significantly enhance your problem-solving abilities and become a more proficient programmer.
Remember that everyone’s learning path is unique, so don’t be discouraged if you find certain concepts challenging at first. Persistence and consistent effort are key to mastering these skills. As you progress, you’ll find that your improved coding logic and reasoning abilities not only make you a better programmer but also open up new opportunities in your career, especially when targeting positions at major tech companies.
Keep challenging yourself, stay curious, and never stop learning. With time and practice, you’ll develop the strong coding logic and reasoning skills that are highly valued in the tech industry. Happy coding!