Mastering Coding Interviews: Proven Strategies to Ace Algorithm Questions
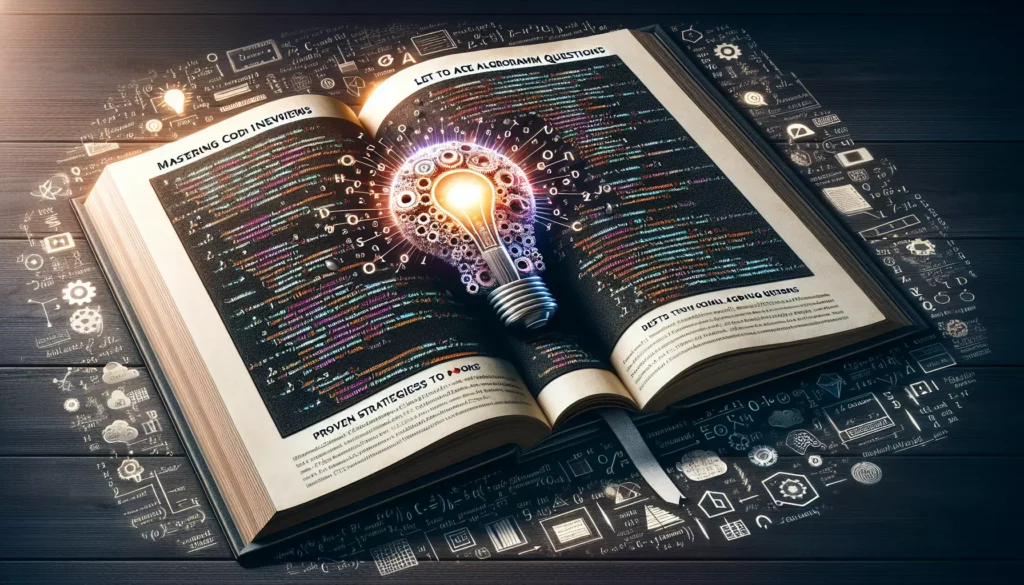
In the competitive world of tech, coding interviews have become a crucial gateway to landing your dream job. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, mastering algorithms is essential. This comprehensive guide will walk you through effective strategies to improve your coding interview performance, with a special focus on tackling algorithm questions.
Understanding the Importance of Algorithms in Coding Interviews
Before diving into improvement strategies, it’s crucial to understand why algorithms play such a significant role in coding interviews. Algorithms are the building blocks of efficient problem-solving in computer science. They demonstrate your ability to think logically, optimize solutions, and handle complex scenarios. Companies use algorithm questions to assess your:
- Problem-solving skills
- Ability to think critically under pressure
- Understanding of fundamental computer science concepts
- Code optimization capabilities
- Communication skills when explaining your thought process
Now that we’ve established the importance of algorithms, let’s explore strategies to enhance your performance in coding interviews.
1. Build a Strong Foundation in Data Structures
A solid understanding of data structures is crucial for solving algorithm problems efficiently. Familiarize yourself with the following key data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
For each data structure, make sure you understand:
- Its basic implementation
- Time and space complexity for common operations
- Typical use cases and scenarios where it excels
- Common algorithms associated with it
Resources like AlgoCademy offer interactive tutorials and exercises to help you master these fundamental data structures.
2. Practice, Practice, Practice
There’s no substitute for consistent practice when it comes to improving your algorithm skills. Here are some effective ways to practice:
a) Solve Problems Regularly
Set aside time each day to solve coding problems. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of algorithm questions, often categorized by difficulty and topic.
b) Participate in Coding Contests
Join coding competitions on platforms like Codeforces or TopCoder. These contests simulate the pressure of real interviews and expose you to diverse problem types.
c) Implement Algorithms from Scratch
Try implementing common algorithms like sorting algorithms (QuickSort, MergeSort), graph algorithms (BFS, DFS), and dynamic programming solutions without referring to existing code. This deepens your understanding of how these algorithms work.
d) Use Spaced Repetition
Revisit problems you’ve solved after a few days or weeks. This reinforces your learning and helps identify areas where you might need more practice.
3. Master Common Algorithm Techniques
Certain algorithm techniques appear frequently in coding interviews. Familiarize yourself with these approaches:
a) Two Pointers
This technique is often used for array or string problems. It involves using two pointers to traverse the data structure, usually moving in opposite directions or at different speeds.
Example problem: Reverse a string in-place.
def reverse_string(s):
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
# Example usage
print(reverse_string(list("hello"))) # Output: ['o', 'l', 'l', 'e', 'h']
b) Sliding Window
This technique is useful for problems involving subarrays or substrings. It maintains a “window” that slides through the data, expanding or contracting as needed.
Example problem: Find the maximum sum of a subarray of size k.
def max_subarray_sum(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
print(max_subarray_sum([1, 4, 2, 10, 23, 3, 1, 0, 20], 4)) # Output: 39
c) Binary Search
Binary search is an efficient algorithm for searching sorted arrays. It’s also useful in various other scenarios where the search space can be divided.
Example problem: Find the first occurrence of a target number in a sorted array.
def binary_search_first_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
result = mid
right = mid - 1 # Continue searching on the left side
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return result
# Example usage
print(binary_search_first_occurrence([1, 2, 2, 2, 3, 4, 5], 2)) # Output: 1
d) Depth-First Search (DFS) and Breadth-First Search (BFS)
These graph traversal algorithms are fundamental for solving problems involving trees, graphs, and matrices.
Example of DFS on a binary tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def dfs_inorder(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(dfs_inorder(root)) # Output: [4, 2, 5, 1, 3]
e) Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into simpler subproblems.
Example problem: Calculate the nth Fibonacci number.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
print(fibonacci(10)) # Output: 55
4. Analyze Time and Space Complexity
Understanding the time and space complexity of your solutions is crucial. Interviewers often ask about the efficiency of your code and expect you to optimize it if possible.
- Learn to analyze time complexity using Big O notation.
- Practice identifying the bottlenecks in your code.
- Be prepared to discuss trade-offs between time and space complexity.
Example: Analyzing the time complexity of a simple loop.
def find_max(arr):
if not arr:
return None
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
# Time Complexity: O(n), where n is the length of the array
# Space Complexity: O(1), as we only use a constant amount of extra space
5. Develop a Problem-Solving Approach
Having a structured approach to problem-solving can significantly improve your performance in coding interviews. Follow these steps:
- Understand the problem: Clarify any ambiguities and ask questions if needed.
- Analyze the constraints: Consider the input size, time/space limitations, and edge cases.
- Brainstorm solutions: Think of multiple approaches before settling on one.
- Plan your approach: Outline your solution before coding.
- Implement the solution: Write clean, readable code.
- Test your code: Use example inputs and edge cases to verify your solution.
- Optimize if necessary: Look for ways to improve time or space complexity.
6. Mock Interviews and Peer Programming
Simulating real interview conditions can help you become more comfortable and confident. Consider these options:
- Use platforms like Pramp or InterviewBit for mock interviews with peers.
- Ask friends or colleagues to conduct mock interviews for you.
- Join coding interview study groups or communities.
- Practice explaining your thought process out loud as you solve problems.
7. Learn from Your Mistakes
Every mistake is an opportunity to learn and improve. After each practice session or mock interview:
- Review the problems you struggled with.
- Analyze why you made certain mistakes.
- Study alternative solutions and more efficient approaches.
- Keep a log of common mistakes and areas for improvement.
8. Stay Updated with Industry Trends
The tech industry is constantly evolving, and so are coding interview practices. Stay informed about:
- New types of questions being asked in interviews.
- Emerging technologies and their impact on algorithmic problem-solving.
- Best practices in software development and clean coding.
9. Utilize Online Resources and Courses
Take advantage of the wealth of online resources available for algorithm practice and interview preparation:
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms.
- Coursera and edX: Provide in-depth computer science and algorithm courses from top universities.
- YouTube channels: Channels like “Back to Back SWE” and “Tushar Roy – Coding Made Simple” offer excellent algorithm explanations.
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell and “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein are invaluable resources.
10. Manage Interview Anxiety
Even with extensive preparation, interview anxiety can impact your performance. Here are some tips to manage stress:
- Practice relaxation techniques like deep breathing or meditation.
- Maintain a positive mindset and focus on your preparation.
- Get enough sleep and eat well before the interview.
- Remember that it’s okay to ask for clarification or take a moment to think during the interview.
Conclusion: Continuous Improvement is Key
Improving your coding interview performance on algorithms is a journey that requires dedication, consistent practice, and a willingness to learn from both successes and failures. By following the strategies outlined in this guide and leveraging resources like AlgoCademy, you can significantly enhance your problem-solving skills and increase your chances of success in technical interviews.
Remember, the goal is not just to memorize solutions but to develop a deep understanding of algorithmic thinking. This skill will not only help you in interviews but will also make you a better software engineer in the long run.
As you continue your preparation, stay motivated, track your progress, and celebrate small victories along the way. With persistence and the right approach, you’ll be well-equipped to tackle even the most challenging algorithm questions in your next coding interview.
Good luck, and happy coding!