Mastering Coding Interviews: A Comprehensive Guide
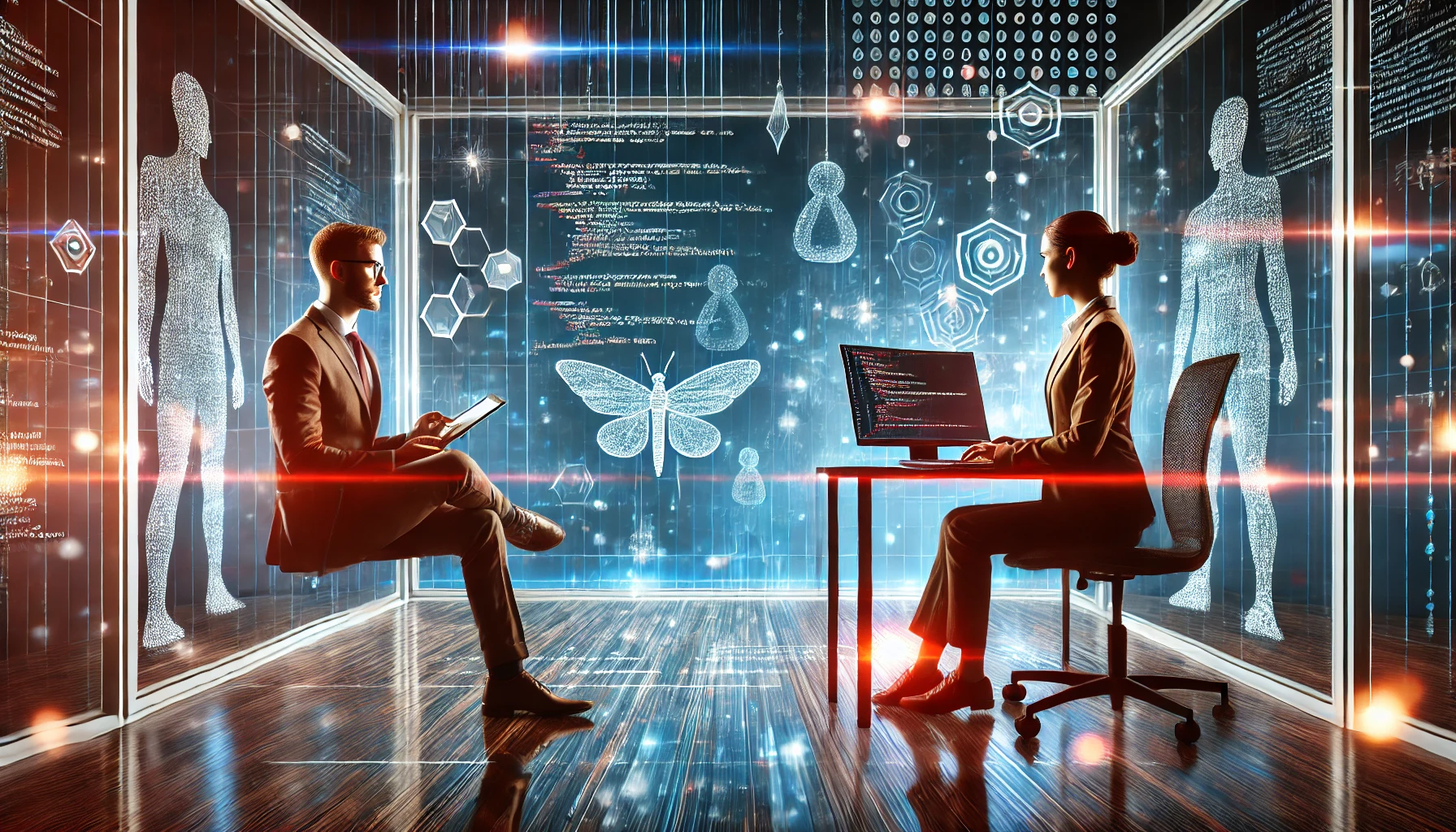
In today’s competitive tech landscape, coding interviews have become the gateway to landing your dream job at companies like Google or Facebook. The good news? You don’t necessarily need a college degree. The challenge? These interviews are notoriously difficult and complex. But fear not! This comprehensive guide will walk you through the essential stages of building a solid programming foundation, mastering algorithms and data structures, and ultimately landing your first developer job or moving to a better company.
Why Coding Interviews Matter
Coding interviews are the bread and butter of tech company hiring processes. They involve logic-based challenges that test your ability to:
- Solve problems efficiently
- Communicate your thoughts clearly
- Write high-quality code
These skills are crucial because modern tech companies, especially the big ones with disruptive products, need developers who can come up with optimal, original solutions to complex problems. Coding interviews help them identify candidates with these abilities.
The Interview Process
Typically, the interview process follows these stages:
- Online assessment: Solve 1-2 coding challenges
- Phone screen: Tackle 1-2 more coding challenges
- On-site interview: Face several coding challenges in person
To excel at each stage, you need to become a great problem solver. But how do you get there?
The Right Mindset for Success
Before diving into the technical aspects, it’s crucial to adopt the right mindset:
- Learn things in the right order: Don’t jump straight into advanced algorithms. Master the basics first.
- Choose quality over quantity: Spend at least 30 minutes on each question, trying to develop your own optimal solution.
- Trust the process: Skill development takes time. Be patient and persistent.
The Comprehensive Study Plan
Now, let’s break down the study plan into manageable stages:
Stage 1: Fundamental Concepts
Start with the basics:
- Variables: Understand data types and mathematical operations.
- Conditional statements: Learn to control program flow with if-else statements.
- Loops: Master for and while loops for repetitive tasks.
- Functions: Organize your code efficiently.
Practice these concepts with simple exercises before moving on.
Stage 2: Advanced Concepts
Build on your foundation:
- Time and space complexity: Learn to measure and optimize your algorithms.
- Arrays: Master creating, accessing, and manipulating arrays.
- Strings: Understand string operations and built-in functions.
- Multi-dimensional arrays: Get comfortable with 2D arrays.
- Nested loops: Practice problems requiring multiple levels of iteration.
- Classes: Learn the basics of object-oriented programming.
Stage 3: Deep Dive into Arrays and Strings
Arrays and strings are fundamental to many coding challenges:
- Array techniques: Practice finding min/max elements, searching, and reordering.
- String manipulation: Learn to reverse strings, check for palindromes, and find substrings.
- Nested loops: Solve more complex problems like Two Sum.
Stage 4: Sorting and Searching
Sorting is a crucial skill:
- Sorting algorithms: Understand bubble sort, insertion sort, and selection sort.
- Built-in sort functions: Learn how to use and customize them.
- Comparison functions: Create custom sorting criteria.
- Binary search: Master this efficient searching algorithm and its variations.
Stage 5: Hash Tables
Hash tables are powerful data structures:
- HashMap: Use for quick key-value lookups.
- HashSet: Employ for storing unique elements.
- Practice: Solve problems involving counting occurrences or finding unique elements.
Stage 6: Recursion and Backtracking
Recursion is key to solving complex problems:
- Recursive functions: Understand how they work and practice simple problems.
- Memoization: Learn to optimize recursive solutions.
- Backtracking: Generate all possible configurations for a problem.
Stage 7: Divide and Conquer
This technique breaks down complex problems:
- Concept: Understand how to divide problems into smaller subproblems.
- Practice: Implement merge sort and quicksort algorithms.
Stage 8: Linked Lists
Linked lists are common in interviews:
- Singly linked lists: Master traversal, insertion, and deletion.
- Doubly linked lists: Understand the added complexity of backward links.
- Pointer manipulation: Practice reversing linked lists and detecting cycles.
Stage 9: Binary Trees and Binary Search Trees
Trees are hierarchical data structures:
- Binary trees: Understand the structure and basic operations.
- Binary search trees: Learn the ordering property and its applications.
- Depth-first search (DFS): Master in-order, post-order, and pre-order traversals.
Stage 10: Heaps and Priority Queues
These data structures efficiently manage collections:
- Min heaps and max heaps: Understand their properties and uses.
- Priority queues: Learn when and how to apply them in problem-solving.
Stage 11: Advanced Array Techniques
Some problems require specialized array techniques:
- Partial sums: Understand prefix sum arrays.
- Kadane’s algorithm: Learn to find maximum sum subarrays.
- Sliding window: Master this technique for substring problems.
- Two pointers: Apply this method to array manipulation problems.
Stage 12: Stacks and Queues
These data structures manage data in specific orders:
- Stacks: Understand LIFO (Last In, First Out) operations.
- Queues: Learn FIFO (First In, First Out) principles.
- Applications: Practice using stacks and queues in tree and graph problems.
Stage 13: Graphs
Graphs model complex relationships:
- Graph representation: Learn adjacency lists and matrices.
- Depth-first search (DFS): Understand applications like connected components and topological sort.
- Breadth-first search (BFS): Master shortest path algorithms in unweighted graphs.
- Dijkstra’s algorithm: Learn to find shortest paths in weighted graphs.
Stage 14: Dynamic Programming
Dynamic programming optimizes recursive solutions:
- Concept: Understand how to break down problems into subproblems.
- Memoization vs. Tabulation: Learn both approaches to DP.
- Practice: Start with 1D problems and progress to 2D and 3D DP problems.
Practical Tips for Interview Success
- Simulate real interviews: Practice solving 2-3 coding questions optimally within an hour.
- Improve communication: Explain your thought process clearly while coding.
- Write quality code: Focus on readability and efficiency.
- Handle edge cases: Always consider and test for edge cases in your solutions.
- Time management: Learn to balance thinking, coding, and testing within the interview timeframe.
Beyond Technical Skills
While technical prowess is crucial, don’t neglect these aspects:
- Soft skills: Develop your communication and teamwork abilities.
- Problem-solving mindset: Cultivate a systematic approach to breaking down complex problems.
- Continuous learning: Stay updated with new technologies and best practices.
- Projects: Build personal projects to showcase your skills and passion.
Conclusion
Mastering coding interviews is a journey that requires dedication, patience, and consistent practice. By following this comprehensive guide and study plan, you’ll be well-equipped to tackle even the most challenging coding interviews. Remember, the goal isn’t just to pass the interview but to become a skilled problem solver and high-quality code writer.
As you progress through your preparation, don’t hesitate to seek help when needed. Consider joining coding communities, participating in mock interviews, or even enrolling in mentorship programs for personalized guidance.
With perseverance and the right approach, you’ll be well on your way to landing your dream job in the tech industry. Good luck, and happy coding!