Mastering Coding Challenges: Your Ultimate Guide to Competitive Programming
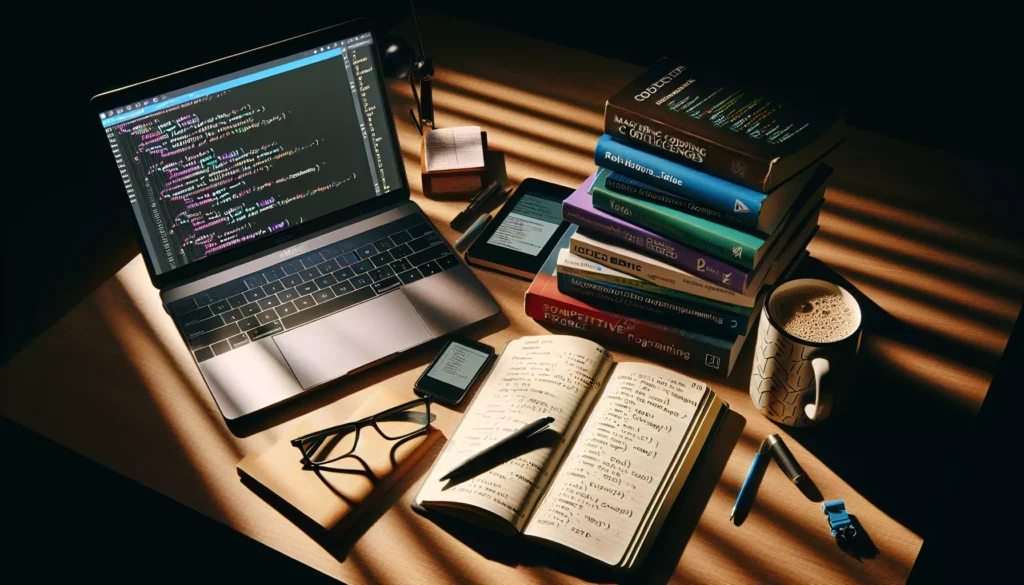
In the rapidly evolving world of technology, coding skills have become more crucial than ever. Whether you’re a budding programmer or an experienced developer looking to sharpen your skills, participating in coding challenges and competitions can be an excellent way to enhance your abilities and stand out in the competitive job market. This comprehensive guide will explore the world of coding challenges, their benefits, and how you can leverage platforms like HackerRank, CodeChef, and Topcoder to boost your programming prowess.
The Importance of Coding Challenges in Skill Development
Coding challenges are more than just fun exercises; they play a vital role in developing and honing your programming skills. Here’s why they’re essential:
- Problem-solving skills: Challenges force you to think creatively and analytically to solve complex problems.
- Time management: Many competitions have time constraints, helping you learn to code efficiently under pressure.
- Exposure to new concepts: You’ll encounter a wide variety of problems, exposing you to different algorithms and data structures.
- Practical application: Challenges often simulate real-world scenarios, bridging the gap between theory and practice.
- Competitive edge: Regular participation can give you an advantage in technical interviews and job applications.
Popular Coding Challenge Platforms
Let’s dive into some of the most popular platforms for coding challenges and competitions:
1. HackerRank
HackerRank is a technology company that focuses on competitive programming challenges for both consumers and businesses. It’s an excellent platform for beginners and experienced coders alike.
Key Features:
- Wide range of programming languages supported
- Skill-based challenges and certifications
- Interview preparation kits
- Company-sponsored coding contests
How to Get Started:
- Create a free account on HackerRank.com
- Choose your preferred programming language
- Start with easy challenges and progress to more difficult ones
- Participate in contests to test your skills against others
2. CodeChef
CodeChef is a competitive programming platform that hosts monthly coding competitions and provides a vast problem archive for practice.
Key Features:
- Long and short coding contests
- Discussion forums for problem-solving
- Practice problems with various difficulty levels
- CodeChef Certified Data Structure & Algorithms Programme
How to Get Started:
- Sign up for a free account on CodeChef.com
- Explore the practice section and attempt problems
- Join the monthly Long Challenge to improve your skills
- Participate in Cook-Off and Lunchtime competitions for quick challenges
3. Topcoder
Topcoder is one of the oldest competitive programming platforms, known for its high-quality challenges and strong community.
Key Features:
- Algorithm competitions
- Development and design challenges
- Real-world problem-solving opportunities
- Potential to earn money through competitions
How to Get Started:
- Create an account on Topcoder.com
- Start with practice rounds to familiarize yourself with the platform
- Participate in Single Round Matches (SRMs) for algorithmic challenges
- Explore Marathon Matches for longer, optimization-based problems
Strategies for Success in Coding Challenges
To make the most of your coding challenge experience, consider the following strategies:
1. Start with the Basics
Before diving into complex challenges, ensure you have a solid foundation in programming fundamentals. This includes:
- Basic data structures (arrays, linked lists, stacks, queues)
- Fundamental algorithms (sorting, searching, recursion)
- Time and space complexity analysis
Here’s a simple example of implementing a stack in Python:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
def peek(self):
if not self.is_empty():
return self.items[-1]
def size(self):
return len(self.items)
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
2. Practice Regularly
Consistency is key when it comes to improving your coding skills. Set aside time each day or week to solve problems and participate in challenges. Even 30 minutes a day can make a significant difference over time.
3. Learn from Others
After completing a challenge, take the time to review other participants’ solutions. This can expose you to different approaches and potentially more efficient algorithms. Many platforms provide discussion forums where you can engage with other coders and learn from their experiences.
4. Focus on Problem-Solving Skills
While knowing various algorithms is important, the ability to break down and solve complex problems is crucial. Practice the following problem-solving steps:
- Understand the problem thoroughly
- Break the problem into smaller, manageable parts
- Identify the appropriate algorithms or data structures
- Implement your solution
- Test and optimize your code
5. Time Management
Many coding competitions have time limits, so it’s essential to manage your time effectively. Here are some tips:
- Read all problems before starting to solve any
- Start with the problems you’re most confident about
- If you’re stuck on a problem, move on and come back to it later
- Leave time for testing and debugging
6. Learn and Implement Efficient Algorithms
As you progress, focus on learning and implementing more advanced algorithms and data structures. Some important ones to master include:
- Dynamic Programming
- Graph Algorithms (BFS, DFS, Dijkstra’s, etc.)
- Advanced Data Structures (Segment Trees, Fenwick Trees, etc.)
- String Algorithms (KMP, Z-algorithm, etc.)
Here’s an example of implementing the Knuth-Morris-Pratt (KMP) string matching algorithm in C++:
#include <iostream>
#include <vector>
#include <string>
std::vector<int> computeLPS(const std::string& pattern) {
int m = pattern.length();
std::vector<int> lps(m, 0);
int len = 0;
int i = 1;
while (i < m) {
if (pattern[i] == pattern[len]) {
len++;
lps[i] = len;
i++;
} else {
if (len != 0) {
len = lps[len - 1];
} else {
lps[i] = 0;
i++;
}
}
}
return lps;
}
void KMPSearch(const std::string& text, const std::string& pattern) {
int n = text.length();
int m = pattern.length();
std::vector<int> lps = computeLPS(pattern);
int i = 0, j = 0;
while (i < n) {
if (pattern[j] == text[i]) {
j++;
i++;
}
if (j == m) {
std::cout << "Pattern found at index " << i - j << std::endl;
j = lps[j - 1];
} else if (i < n && pattern[j] != text[i]) {
if (j != 0) {
j = lps[j - 1];
} else {
i++;
}
}
}
}
int main() {
std::string text = "ABABDABACDABABCABAB";
std::string pattern = "ABABCABAB";
KMPSearch(text, pattern);
return 0;
}
Leveraging Coding Challenges for Career Growth
Participating in coding challenges can significantly boost your career prospects. Here’s how:
1. Building a Strong Portfolio
Your performance in coding challenges can serve as a portfolio of your skills. Many platforms provide profiles that showcase your achievements, which can be shared with potential employers.
2. Preparing for Technical Interviews
The problems you encounter in coding challenges are often similar to those asked in technical interviews, especially for positions at major tech companies like Google, Facebook, Amazon, Apple, and Netflix (often referred to as FAANG).
3. Networking Opportunities
Participating in coding competitions can connect you with like-minded individuals and potential employers. Many companies sponsor coding contests to identify talented programmers.
4. Continuous Learning
The tech industry is constantly evolving, and coding challenges help you stay updated with the latest algorithms and problem-solving techniques.
Advanced Topics in Competitive Programming
As you progress in your competitive programming journey, you’ll encounter more advanced topics. Here are some areas to explore:
1. Advanced Data Structures
Familiarize yourself with complex data structures such as:
- Segment Trees
- Fenwick Trees (Binary Indexed Trees)
- Disjoint Set Union (DSU)
- Trie (Prefix Tree)
Here’s an example of a Trie implementation in Java:
class TrieNode {
TrieNode[] children;
boolean isEndOfWord;
TrieNode() {
children = new TrieNode[26];
isEndOfWord = false;
}
}
class Trie {
private TrieNode root;
public Trie() {
root = new TrieNode();
}
public void insert(String word) {
TrieNode current = root;
for (char ch : word.toCharArray()) {
int index = ch - 'a';
if (current.children[index] == null) {
current.children[index] = new TrieNode();
}
current = current.children[index];
}
current.isEndOfWord = true;
}
public boolean search(String word) {
TrieNode node = searchPrefix(word);
return node != null && node.isEndOfWord;
}
public boolean startsWith(String prefix) {
return searchPrefix(prefix) != null;
}
private TrieNode searchPrefix(String word) {
TrieNode current = root;
for (char ch : word.toCharArray()) {
int index = ch - 'a';
if (current.children[index] == null) {
return null;
}
current = current.children[index];
}
return current;
}
}
// Usage
Trie trie = new Trie();
trie.insert("apple");
System.out.println(trie.search("apple")); // returns true
System.out.println(trie.search("app")); // returns false
System.out.println(trie.startsWith("app")); // returns true
2. Graph Algorithms
Master various graph algorithms, including:
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Dijkstra’s Algorithm for shortest paths
- Floyd-Warshall Algorithm for all-pairs shortest paths
- Minimum Spanning Tree algorithms (Kruskal’s and Prim’s)
- Topological Sorting
- Strongly Connected Components
3. Dynamic Programming
Dynamic Programming (DP) is a powerful technique for solving optimization problems. Key concepts include:
- Memoization and Tabulation
- State transitions
- Subproblem optimization
- Common DP patterns (e.g., Knapsack, Longest Common Subsequence)
4. Number Theory and Combinatorics
These mathematical concepts often appear in advanced coding challenges:
- Prime number algorithms (Sieve of Eratosthenes)
- Modular arithmetic
- Combinatorial calculations
- Fermat’s Little Theorem
5. String Algorithms
Advanced string manipulation techniques include:
- Knuth-Morris-Pratt (KMP) Algorithm
- Z-Algorithm
- Suffix Arrays and LCP Arrays
- Aho-Corasick Algorithm for pattern matching
Balancing Competitive Programming with Real-World Development
While competitive programming can significantly enhance your problem-solving skills, it’s important to balance this with real-world software development experience. Here are some tips to maintain this balance:
1. Apply Competitive Programming Skills to Projects
Use the algorithms and optimization techniques you learn from coding challenges in your personal or professional projects. This helps bridge the gap between competitive programming and practical software development.
2. Focus on Code Readability and Maintainability
In competitive programming, the focus is often on solving problems quickly. However, in real-world scenarios, code readability and maintainability are crucial. Practice writing clean, well-documented code even when solving challenges.
3. Explore Software Design Patterns
While not typically emphasized in coding competitions, design patterns are essential for building scalable and maintainable software systems. Familiarize yourself with common patterns like Singleton, Factory, Observer, and Strategy.
4. Learn Version Control Systems
Version control is a fundamental skill for any software developer. Get comfortable with Git and platforms like GitHub or GitLab.
5. Contribute to Open Source Projects
Contributing to open source projects can help you apply your skills in a collaborative environment and expose you to large-scale codebases.
Conclusion
Participating in coding challenges and competitions is an excellent way to enhance your programming skills, prepare for technical interviews, and stay current with the latest algorithms and problem-solving techniques. Platforms like HackerRank, CodeChef, and Topcoder offer a wealth of opportunities to test your abilities and learn from a global community of developers.
Remember, the key to success in competitive programming is consistent practice, a willingness to learn from others, and the ability to apply your knowledge to solve complex problems efficiently. As you progress, don’t forget to balance your competitive programming skills with real-world software development experience to become a well-rounded and highly sought-after programmer.
Whether you’re a beginner looking to build a strong foundation or an experienced developer aiming to sharpen your skills, the world of coding challenges has something to offer. So, pick a platform, start solving problems, and watch your programming prowess grow. Happy coding!