Mastering CI/CD: A Comprehensive Guide to Continuous Integration and Continuous Deployment
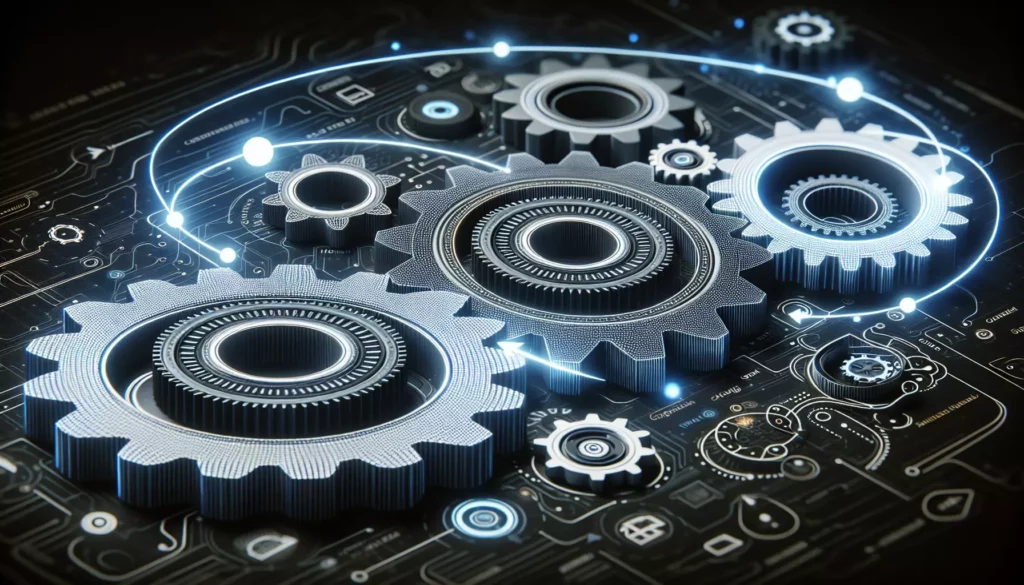
In today’s fast-paced software development world, the ability to deliver high-quality code quickly and efficiently is crucial. This is where Continuous Integration and Continuous Deployment (CI/CD) come into play. As an essential part of modern software engineering practices, CI/CD has revolutionized the way teams build, test, and deploy applications. In this comprehensive guide, we’ll dive deep into the world of CI/CD, exploring its concepts, benefits, and popular tools that can help streamline your software delivery process.
Understanding CI/CD
Before we delve into the specifics, let’s break down what CI/CD actually means:
Continuous Integration (CI)
Continuous Integration is the practice of frequently merging code changes into a central repository. This process involves automatically building and testing the code to ensure that new changes don’t break the existing codebase. The primary goal of CI is to detect and address integration issues early in the development cycle.
Continuous Deployment (CD)
Continuous Deployment takes CI a step further by automatically deploying all code changes to a testing or production environment after the build stage. This means that every change that passes all automated tests is released to users without manual intervention.
It’s worth noting that some organizations use the term “Continuous Delivery” instead of “Continuous Deployment.” The main difference is that Continuous Delivery involves a manual approval step before changes are deployed to production, while Continuous Deployment is fully automated.
The Benefits of Implementing CI/CD
Adopting CI/CD practices can bring numerous advantages to your development process:
- Faster Time-to-Market: By automating the build, test, and deployment processes, CI/CD significantly reduces the time it takes to get new features and bug fixes to users.
- Improved Code Quality: Frequent integration and automated testing help catch bugs early, leading to higher overall code quality.
- Reduced Risk: Smaller, more frequent releases minimize the risk associated with big bang releases and make it easier to identify and fix issues.
- Increased Collaboration: CI/CD encourages developers to commit code more frequently, fostering better communication and collaboration within teams.
- Enhanced Productivity: Automation of repetitive tasks frees up developers to focus on writing code and solving problems.
- Better Visibility: CI/CD pipelines provide clear insights into the state of your application at each stage of development.
Key Components of a CI/CD Pipeline
A typical CI/CD pipeline consists of several stages:
- Source Control: This is where developers commit their code changes. Popular options include Git, SVN, and Mercurial.
- Build: The process of compiling source code into executable code.
- Test: Automated tests are run to ensure code quality and functionality.
- Deploy: The application is deployed to a staging or production environment.
- Monitor: The deployed application is monitored for performance and errors.
Popular CI/CD Tools
Now that we understand the basics of CI/CD, let’s explore some of the most popular tools used to implement these practices:
Jenkins
Jenkins is one of the most widely used open-source automation servers. It offers a vast ecosystem of plugins and integrations, making it highly customizable for various development needs.
Key Features of Jenkins:
- Extensible through a wide range of plugins
- Supports distributed builds across multiple machines
- Easy configuration via web interface
- Integrates with virtually any tool in the software development lifecycle
Setting up a Basic Jenkins Pipeline
Here’s a simple example of a Jenkinsfile that defines a basic CI/CD pipeline:
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building the application...'
// Add your build commands here
}
}
stage('Test') {
steps {
echo 'Running tests...'
// Add your test commands here
}
}
stage('Deploy') {
steps {
echo 'Deploying the application...'
// Add your deployment commands here
}
}
}
}
This pipeline defines three stages: Build, Test, and Deploy. Each stage contains steps that would typically include commands to build your application, run tests, and deploy to your target environment.
Travis CI
Travis CI is a popular cloud-based CI/CD service that integrates well with GitHub repositories. It’s known for its ease of use and is particularly popular among open-source projects.
Key Features of Travis CI:
- Easy setup with GitHub integration
- Supports a wide range of programming languages and frameworks
- Provides both Linux and macOS build environments
- Offers parallel build execution
Example Travis CI Configuration
Here’s a basic .travis.yml file for a Node.js project:
language: node_js
node_js:
- "14"
cache:
directories:
- node_modules
script:
- npm run build
- npm test
deploy:
provider: heroku
api_key: $HEROKU_API_KEY
app: my-app-name
This configuration specifies the language (Node.js), the version to use, caches the node_modules directory for faster builds, defines the build and test scripts to run, and sets up automatic deployment to Heroku.
CircleCI
CircleCI is another cloud-based CI/CD platform that offers a flexible and powerful pipeline configuration system. It’s known for its speed and efficiency, particularly for projects that require complex workflows.
Key Features of CircleCI:
- Supports Docker out of the box
- Offers parallel execution of tests
- Provides detailed insights and analytics
- Allows for complex workflow orchestration
Example CircleCI Configuration
Here’s a sample config.yml file for a CircleCI pipeline:
version: 2.1
jobs:
build:
docker:
- image: circleci/node:14
steps:
- checkout
- run: npm install
- run: npm run build
test:
docker:
- image: circleci/node:14
steps:
- checkout
- run: npm install
- run: npm test
deploy:
docker:
- image: circleci/node:14
steps:
- checkout
- run: npm install
- run: npm run deploy
workflows:
version: 2
build-test-deploy:
jobs:
- build
- test:
requires:
- build
- deploy:
requires:
- test
filters:
branches:
only: main
This configuration defines three jobs (build, test, and deploy) and orchestrates them in a workflow. The deploy job only runs on the main branch after the build and test jobs have completed successfully.
Best Practices for Implementing CI/CD
To get the most out of your CI/CD implementation, consider the following best practices:
- Commit Often: Encourage developers to make small, frequent commits to catch integration issues early.
- Maintain a Comprehensive Test Suite: Ensure your automated tests cover a wide range of scenarios to catch potential issues.
- Keep the Build Fast: Aim for quick feedback by optimizing your build and test processes.
- Use Feature Flags: Implement feature flags to decouple deployment from release, allowing for more controlled rollouts.
- Monitor and Iterate: Continuously monitor your pipeline and application performance, and make improvements based on feedback and metrics.
- Secure Your Pipeline: Implement security best practices throughout your CI/CD pipeline to protect sensitive information and prevent unauthorized access.
- Document Your Process: Maintain clear documentation of your CI/CD setup to help onboard new team members and troubleshoot issues.
Challenges in Implementing CI/CD
While CI/CD offers numerous benefits, it’s not without its challenges. Here are some common hurdles you might face:
- Cultural Resistance: Some team members may be resistant to changing established workflows.
- Initial Setup Complexity: Setting up a CI/CD pipeline can be complex, especially for large, existing projects.
- Maintaining Test Quality: As the codebase grows, maintaining a comprehensive and efficient test suite can become challenging.
- Managing Environment Parity: Ensuring consistency across development, testing, and production environments can be difficult.
- Handling Database Changes: Managing database schema changes and data migrations in a CI/CD context requires careful planning.
CI/CD in the Context of Different Development Methodologies
CI/CD practices can be adapted to fit various development methodologies:
Agile Development
CI/CD aligns well with Agile methodologies, supporting the principles of frequent delivery and responding to change. It enables teams to deliver working software at the end of each sprint or even more frequently.
DevOps
CI/CD is a cornerstone of DevOps practices, bridging the gap between development and operations by automating the software delivery process. It supports the DevOps goals of increased collaboration, faster delivery, and improved quality.
Microservices Architecture
In a microservices environment, CI/CD becomes even more crucial. It allows teams to independently build, test, and deploy individual services, supporting the decoupled nature of microservices architecture.
Future Trends in CI/CD
As technology evolves, so do CI/CD practices. Here are some emerging trends to watch:
- AI-Powered CI/CD: Machine learning algorithms are being used to optimize build processes, predict test outcomes, and even suggest code improvements.
- GitOps: This approach uses Git as the single source of truth for declarative infrastructure and applications.
- Serverless CI/CD: Serverless computing is being leveraged to create more scalable and cost-effective CI/CD pipelines.
- Progressive Delivery: This extends continuous delivery by gradually rolling out changes to a subset of users before a full release.
Conclusion
Continuous Integration and Continuous Deployment have become integral parts of modern software development. By automating the build, test, and deployment processes, CI/CD enables teams to deliver high-quality software faster and more reliably.
Whether you choose Jenkins, Travis CI, CircleCI, or another tool, implementing CI/CD can significantly improve your development workflow. Remember that CI/CD is not just about tools, but also about fostering a culture of collaboration, automation, and continuous improvement.
As you embark on your CI/CD journey, start small, focus on automating one part of your process at a time, and gradually build up to a full CI/CD pipeline. With patience and persistence, you’ll soon reap the benefits of faster, more reliable software delivery.
The world of CI/CD is vast and ever-evolving. As you continue to learn and implement these practices, you’ll discover new tools, techniques, and best practices that can further enhance your development process. Embrace the journey of continuous learning and improvement – after all, that’s what CI/CD is all about!