Mastering C++ Input and Output: A Comprehensive Guide to cout and cin
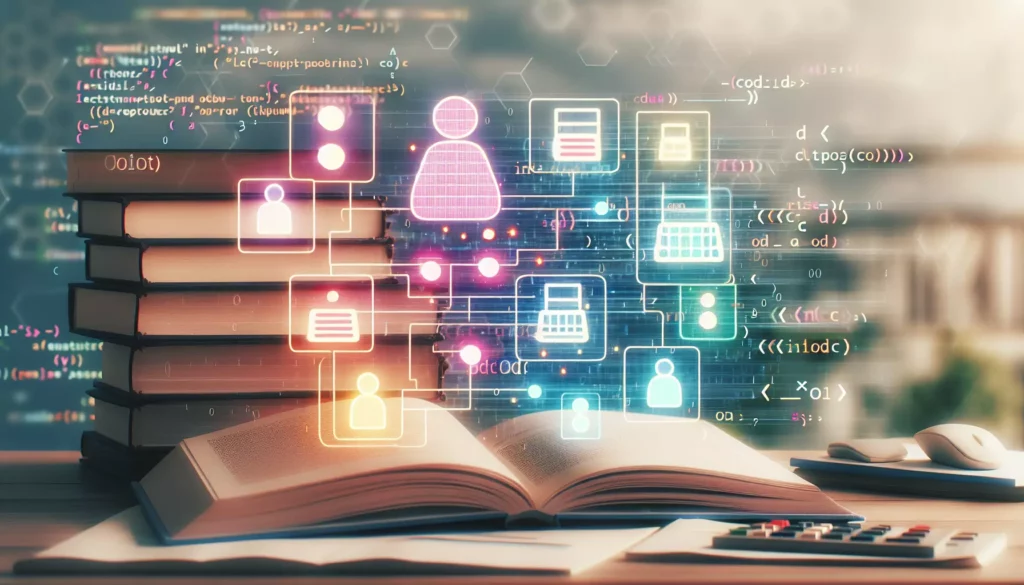
C++ is a powerful programming language that offers robust input and output capabilities. Two of the most fundamental and widely used features for handling input and output in C++ are cout
and cin
. These objects, part of the iostream library, allow programmers to interact with users, display information, and receive data from various sources. In this comprehensive guide, we’ll explore the ins and outs of cout
and cin
, providing you with the knowledge and skills to effectively utilize these essential tools in your C++ programs.
Table of Contents
- Introduction to C++ Input and Output
- Understanding cout: C++’s Output Stream
- Mastering cin: C++’s Input Stream
- Formatting Output with cout
- Advanced Input Techniques with cin
- Common Pitfalls and How to Avoid Them
- Best Practices for Using cout and cin
- Alternative Input/Output Methods in C++
- Practical Examples and Use Cases
- Conclusion
1. Introduction to C++ Input and Output
Before diving into the specifics of cout
and cin
, it’s essential to understand the broader context of input and output in C++. Input/Output (I/O) operations are fundamental to almost every program, allowing software to communicate with users, read from files, and write data to various destinations.
In C++, I/O operations are primarily handled through streams. A stream is an abstraction that represents a device on which input and output operations are performed. The iostream library provides several pre-defined stream objects:
cout
: Standard output stream (usually the console)cin
: Standard input stream (usually the keyboard)cerr
: Standard error stream (unbuffered)clog
: Standard error stream (buffered)
In this guide, we’ll focus on cout
and cin
, as they are the most commonly used for basic input and output operations.
2. Understanding cout: C++’s Output Stream
cout
is an object of the ostream
class and is used to display output to the standard output device, which is typically the console. Here’s a basic example of how to use cout
:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this example, we use the insertion operator (<<
) to send the string “Hello, World!” to the cout
object, which then displays it on the console.
Key features of cout
:
- No need to specify data types:
cout
automatically detects the type of data being output. - Chainable: You can output multiple items in a single statement.
- Supports various data types: integers, floating-point numbers, strings, and more.
Here’s an example demonstrating these features:
#include <iostream>
int main() {
int age = 30;
double height = 1.75;
std::string name = "John Doe";
std::cout << "Name: " << name << "\nAge: " << age << "\nHeight: " << height << " meters";
return 0;
}
This code will output:
Name: John Doe
Age: 30
Height: 1.75 meters
3. Mastering cin: C++’s Input Stream
cin
is an object of the istream
class and is used to read input from the standard input device, typically the keyboard. It uses the extraction operator (>>
) to read data into variables. Here’s a basic example:
#include <iostream>
#include <string>
int main() {
std::string name;
int age;
std::cout << "Enter your name: ";
std::cin >> name;
std::cout << "Enter your age: ";
std::cin >> age;
std::cout << "Hello, " << name << "! You are " << age << " years old.";
return 0;
}
Key features of cin
:
- Automatically converts input to the appropriate data type.
- Skips whitespace (spaces, tabs, newlines) by default.
- Can be chained to read multiple inputs in a single statement.
It’s important to note that cin
stops reading at the first whitespace character it encounters. This can lead to unexpected behavior when reading strings with spaces. We’ll address this issue in the “Advanced Input Techniques” section.
4. Formatting Output with cout
While cout
is straightforward to use, you often need more control over how your output is formatted. C++ provides several ways to format output:
4.1 Manipulators
Manipulators are special functions that can be inserted into the output stream to modify its behavior. Some common manipulators include:
endl
: Inserts a newline character and flushes the stream.setw(int n)
: Sets the width of the next output field.setprecision(int n)
: Sets the decimal precision for floating-point numbers.fixed
: Uses fixed-point notation for floating-point numbers.scientific
: Uses scientific notation for floating-point numbers.
Here’s an example using some of these manipulators:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159265358979323846;
std::cout << "Default: " << pi << std::endl;
std::cout << "Fixed: " << std::fixed << pi << std::endl;
std::cout << "Scientific: " << std::scientific << pi << std::endl;
std::cout << "Precision 10: " << std::setprecision(10) << pi << std::endl;
std::cout << "Width 20: " << std::setw(20) << pi << "|" << std::endl;
return 0;
}
4.2 Format Flags
You can also use format flags to control various aspects of output formatting. These flags are set using the setf()
function of the ios
class. Some common flags include:
ios::left
: Left-justifies output within its field.ios::right
: Right-justifies output within its field.ios::showpos
: Shows the + sign for positive numbers.ios::uppercase
: Uses uppercase letters in scientific notation.
Here’s an example using format flags:
#include <iostream>
#include <iomanip>
int main() {
int num = 42;
std::cout.setf(std::ios::showpos);
std::cout << "With showpos: " << num << std::endl;
std::cout.setf(std::ios::left, std::ios::adjustfield);
std::cout << std::setw(20) << "Left-justified:" << num << "|" << std::endl;
std::cout.setf(std::ios::right, std::ios::adjustfield);
std::cout << std::setw(20) << "Right-justified:" << num << "|" << std::endl;
return 0;
}
5. Advanced Input Techniques with cin
While cin
is suitable for basic input operations, there are situations where you need more control over how input is read. Let’s explore some advanced techniques:
5.1 Reading Entire Lines
To read an entire line of input, including spaces, you can use the getline()
function:
#include <iostream>
#include <string>
int main() {
std::string fullName;
std::cout << "Enter your full name: ";
std::getline(std::cin, fullName);
std::cout << "Hello, " << fullName << "!";
return 0;
}
5.2 Handling Input Failures
When cin
fails to read input (e.g., when trying to read an integer but the user enters a string), it enters a fail state. You can check for and handle these failures:
#include <iostream>
#include <limits>
int main() {
int age;
while (true) {
std::cout << "Enter your age: ";
if (std::cin >> age) {
break;
} else {
std::cout << "Invalid input. Please enter a number." << std::endl;
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
}
std::cout << "Your age is: " << age;
return 0;
}
5.3 Reading Until a Specific Character
You can use cin.get()
to read characters one at a time until a specific character is encountered:
#include <iostream>
#include <string>
int main() {
std::string input;
char ch;
std::cout << "Enter text (end with #): ";
while (std::cin.get(ch) && ch != '#') {
input += ch;
}
std::cout << "You entered: " << input;
return 0;
}
6. Common Pitfalls and How to Avoid Them
When working with cout
and cin
, there are several common pitfalls that programmers often encounter. Being aware of these issues can help you write more robust and error-free code.
6.1 Buffer Flushing
One common issue is forgetting to flush the output buffer, especially when debugging or writing interactive programs. The output might not appear immediately, leading to confusion. To address this, you can either use endl
(which flushes the buffer) or explicitly call cout.flush()
:
#include <iostream>
int main() {
std::cout << "Enter a number: ";
std::cout.flush(); // Explicitly flush the buffer
int number;
std::cin >> number;
std::cout << "You entered: " << number << std::endl; // endl flushes the buffer
return 0;
}
6.2 Mixing cin and getline()
When using cin
followed by getline()
, you might encounter unexpected behavior due to the newline character left in the input buffer. To avoid this, you can clear the buffer before calling getline()
:
#include <iostream>
#include <string>
int main() {
int age;
std::string name;
std::cout << "Enter your age: ";
std::cin >> age;
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Clear the buffer
std::cout << "Enter your full name: ";
std::getline(std::cin, name);
std::cout << "Age: " << age << ", Name: " << name;
return 0;
}
6.3 Precision Loss in Floating-Point Output
When outputting floating-point numbers, you might experience precision loss. To control this, use the setprecision
manipulator:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159265358979323846;
std::cout << "Default precision: " << pi << std::endl;
std::cout << "High precision: " << std::setprecision(15) << pi << std::endl;
return 0;
}
7. Best Practices for Using cout and cin
To make the most of cout
and cin
, consider following these best practices:
7.1 Use Meaningful Prompts
When requesting input, provide clear and concise prompts to guide the user:
#include <iostream>
#include <string>
int main() {
std::string name;
int age;
std::cout << "Please enter your name: ";
std::getline(std::cin, name);
std::cout << "Please enter your age: ";
std::cin >> age;
std::cout << "Hello, " << name << "! You are " << age << " years old.";
return 0;
}
7.2 Validate Input
Always validate user input to ensure it meets your program’s requirements:
#include <iostream>
#include <limits>
int main() {
int age;
do {
std::cout << "Enter your age (0-120): ";
std::cin >> age;
if (std::cin.fail() || age < 0 || age > 120) {
std::cout << "Invalid input. Please try again." << std::endl;
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
} else {
break;
}
} while (true);
std::cout << "Your age is: " << age;
return 0;
}
7.3 Use Appropriate Data Types
Choose the most appropriate data type for your variables to ensure accurate input and output:
#include <iostream>
#include <iomanip>
int main() {
double price;
int quantity;
std::cout << "Enter the price of the item: $";
std::cin >> price;
std::cout << "Enter the quantity: ";
std::cin >> quantity;
double total = price * quantity;
std::cout << std::fixed << std::setprecision(2);
std::cout << "Total cost: $" << total;
return 0;
}
8. Alternative Input/Output Methods in C++
While cout
and cin
are the most commonly used I/O methods in C++, there are alternative approaches that can be useful in certain situations:
8.1 printf() and scanf()
These functions, inherited from C, can still be used in C++. They offer more control over formatting but are less type-safe:
#include <cstdio>
int main() {
int age;
char name[50];
printf("Enter your name: ");
scanf("%49s", name);
printf("Enter your age: ");
scanf("%d", &age);
printf("Hello, %s! You are %d years old.\n", name, age);
return 0;
}
8.2 File I/O
C++ provides ifstream
and ofstream
classes for file input and output:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ofstream outFile("example.txt");
outFile << "This is a line of text." << std::endl;
outFile.close();
std::ifstream inFile("example.txt");
std::string line;
std::getline(inFile, line);
std::cout << "Read from file: " << line;
inFile.close();
return 0;
}
8.3 Stringstream
stringstream
allows you to treat strings as streams, which can be useful for parsing or formatting:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string input = "42 3.14 Hello";
std::istringstream iss(input);
int number;
double pi;
std::string word;
iss >> number >> pi >> word;
std::cout << "Parsed values: " << number << ", " << pi << ", " << word;
return 0;
}
9. Practical Examples and Use Cases
Let’s explore some practical examples that demonstrate the power and flexibility of cout
and cin
in real-world scenarios:
9.1 Simple Calculator
#include <iostream>
int main() {
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter operation (+, -, *, /): ";
std::cin >> operation;
std::cout << "Enter second number: ";
std::cin >> num2;
double result;
switch (operation) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
} else {
std::cout << "Error: Division by zero!";
return 1;
}
break;
default:
std::cout << "Invalid operation!";
return 1;
}
std::cout << "Result: " << result;
return 0;
}
9.2 Temperature Converter
#include <iostream>
#include <iomanip>
double celsiusToFahrenheit(double celsius) {
return (celsius * 9.0 / 5.0) + 32.0;
}
double fahrenheitToCelsius(double fahrenheit) {
return (fahrenheit - 32.0) * 5.0 / 9.0;
}
int main() {
char choice;
double temperature;
std::cout << "Temperature Converter" << std::endl;
std::cout << "C - Convert Celsius to Fahrenheit" << std::endl;
std::cout << "F - Convert Fahrenheit to Celsius" << std::endl;
std::cout << "Enter your choice (C/F): ";
std::cin >> choice;
std::cout << "Enter temperature: ";
std::cin >> temperature;
double result;
if (toupper(choice) == 'C') {
result = celsiusToFahrenheit(temperature);
std::cout << temperature << " Celsius is " << std::fixed << std::setprecision(2) << result << " Fahrenheit";
} else if (toupper(choice) == 'F') {
result = fahrenheitToCelsius(temperature);
std::cout << temperature << " Fahrenheit is " << std::fixed << std::setprecision(2) << result << " Celsius";
} else {
std::cout << "Invalid choice!";
return 1;
}
return 0;
}
9.3 Simple Address Book
#include <iostream>
#include <string>
#include <vector>
#include <limits>
struct Contact {
std::string name;
std::string phone;
std::string email;
};
void addContact(std::vector<Contact>& addressBook) {
Contact newContact;
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
std::cout << "Enter name: ";
std::getline(std::cin, newContact.name);
std::cout << "Enter phone number: ";
std::getline(std::cin, newContact.phone);
std::cout << "Enter email: ";
std::getline(std::cin, newContact.email);
addressBook.push_back(newContact);
std::cout << "Contact added successfully!" << std::endl;
}
void displayContacts(const std::vector<Contact>& addressBook) {
if (addressBook.empty()) {
std::cout << "Address book is empty." << std::endl;
return;
}
for (size_t i = 0; i < addressBook.size(); ++i) {
std::cout << "Contact " << i + 1 << ":" << std::endl;
std::cout << "Name: " << addressBook[i].name << std::endl;
std::cout << "Phone: " << addressBook[i].phone << std::endl;
std::cout << "Email: " << addressBook[i].email << std::endl;
std::cout << std::endl;
}
}
int main() {
std::vector<Contact> addressBook;
int choice;
do {
std::cout << "Address Book Menu:" << std::endl;
std::cout << "1. Add Contact" << std::endl;
std::cout << "2. Display Contacts" << std::endl;
std::cout << "3. Exit" << std::endl;
std::cout << "Enter your choice: ";
std::cin >> choice;
switch (choice) {
case 1:
addContact(addressBook);
break;
case 2:
displayContacts(addressBook);
break;
case 3:
std::cout << "Goodbye!" << std::endl;
break;
default:
std::cout << "Invalid choice. Please try again." << std::endl;
}
} while (choice != 3);
return 0;
}
10. Conclusion
In this comprehensive guide, we’ve explored the intricacies of cout
and cin
, the primary input and output streams in C++. We’ve covered their basic usage, advanced techniques, common pitfalls, and best practices. By mastering these fundamental I/O tools, you’ll be well-equipped to create interactive and user-friendly C++ programs.
Remember that while cout
and cin
are powerful and versatile, they’re just the beginning of C++’s I/O capabilities. As you continue to develop your C++ skills, you’ll encounter more advanced I/O techniques and libraries that can further enhance your programs.
Practice using cout
and cin
in various scenarios, and don’t hesitate to experiment with different formatting options and input validation techniques. With time and experience, you’ll become proficient in handling input and output in C++, allowing you to create more robust and user-friendly applications.