Mastering Breadth-First Search Techniques: A Comprehensive Guide
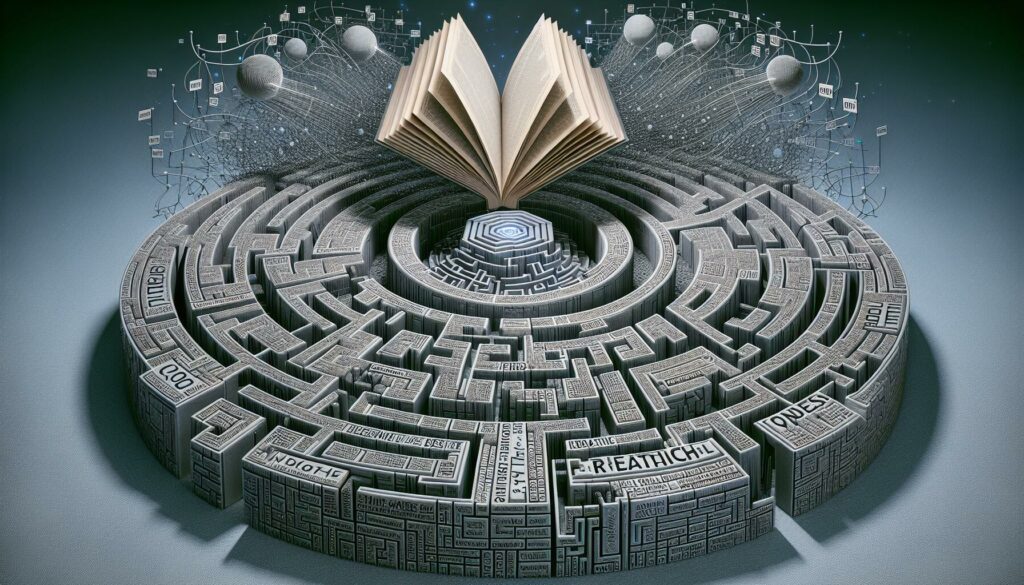
In the world of algorithms and data structures, Breadth-First Search (BFS) stands out as a fundamental technique for exploring and analyzing graphs. Whether you’re a beginner programmer or preparing for technical interviews at top tech companies, understanding BFS is crucial for solving a wide range of problems efficiently. In this comprehensive guide, we’ll dive deep into the intricacies of BFS, explore its applications, and provide practical examples to help you master this essential algorithm.
Table of Contents
- Introduction to Breadth-First Search
- How BFS Works
- Implementing BFS in Code
- Applications of BFS
- Time and Space Complexity
- BFS vs. DFS: When to Use Each
- Optimizing BFS
- Common Interview Questions and Solutions
- Advanced BFS Techniques
- Conclusion
1. Introduction to Breadth-First Search
Breadth-First Search is a graph traversal algorithm that explores all the vertices of a graph or tree in breadth-first order. In simpler terms, it visits all the neighbors at the present depth before moving to the vertices at the next depth level. This approach ensures that the algorithm explores the graph level by level, making it particularly useful for finding the shortest path between two vertices in an unweighted graph.
BFS was first developed by Konrad Zuse in 1945 and later independently by Edward F. Moore in 1959. Since then, it has become an indispensable tool in computer science, with applications ranging from social network analysis to puzzle-solving and pathfinding in artificial intelligence.
2. How BFS Works
To understand how BFS works, let’s break down the algorithm into steps:
- Start at the root node (or any arbitrary node for a graph) and mark it as visited.
- Add the starting node to a queue.
- While the queue is not empty:
- Remove the first node from the queue.
- Process the node (e.g., print it, check if it’s the goal, etc.).
- Add all unvisited neighbors of the current node to the queue and mark them as visited.
- Repeat step 3 until the queue is empty or the goal is reached.
This process ensures that all nodes at a given depth are explored before moving to the next level, hence the name “breadth-first.” The use of a queue data structure is crucial for maintaining the correct order of exploration.
3. Implementing BFS in Code
Let’s implement BFS in Python to solidify our understanding. We’ll use a simple graph represented as an adjacency list:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal starting from vertex 'A':")
bfs(graph, 'A')
This implementation uses Python’s deque
class for efficient queue operations. The algorithm starts at the specified node, marks it as visited, and adds it to the queue. It then enters a loop, processing each node in the queue and adding its unvisited neighbors to the queue.
Running this code will output the BFS traversal of the graph starting from vertex ‘A’:
BFS traversal starting from vertex 'A':
A B C D E F
4. Applications of BFS
BFS has numerous practical applications across various domains of computer science and beyond. Some notable applications include:
- Shortest Path in Unweighted Graphs: BFS can find the shortest path between two nodes in an unweighted graph, making it useful for navigation systems and network routing protocols.
- Web Crawling: Search engines use BFS to discover and index web pages by following links level by level.
- Social Network Analysis: BFS can be used to find the degrees of separation between users in a social network.
- Puzzle Solving: Many puzzle-solving algorithms, such as solving the Rubik’s Cube or the 15-puzzle, use BFS to find the optimal solution.
- Garbage Collection: Some garbage collection algorithms in programming languages use BFS to traverse object graphs and identify unreachable objects.
- Broadcasting in Network: BFS is used in broadcasting algorithms to efficiently disseminate information across a network.
- Finding Connected Components: BFS can be used to identify all connected components in an undirected graph.
- Testing Graph Bipartiteness: BFS can determine if a graph is bipartite by coloring vertices alternately as it traverses the graph.
5. Time and Space Complexity
Understanding the time and space complexity of BFS is crucial for assessing its efficiency in different scenarios:
Time Complexity
The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because:
- Each vertex is enqueued and dequeued exactly once, taking O(V) time.
- Each edge is considered exactly once when we iterate through the neighbors of all vertices, taking O(E) time.
Space Complexity
The space complexity of BFS is O(V), where V is the number of vertices. This space is used for:
- The queue, which in the worst case might contain all vertices of the graph.
- The visited set, which stores all vertices to avoid revisiting them.
It’s worth noting that for dense graphs (where E is close to V^2), the time complexity approaches O(V^2). For sparse graphs, where E is much smaller than V^2, the time complexity is closer to O(V).
6. BFS vs. DFS: When to Use Each
While both Breadth-First Search (BFS) and Depth-First Search (DFS) are graph traversal algorithms, they have different characteristics that make them suitable for different scenarios:
Use BFS when:
- Finding the shortest path in an unweighted graph
- Exploring nodes level by level
- The solution is not far from the root of the tree
- The tree is very deep and solutions are rare
Use DFS when:
- Exploring as far as possible along each branch before backtracking
- The tree is very wide
- Solutions are frequent but located deep in the tree
- Implementing game or puzzle solvers
Here’s a quick comparison table:
Aspect | BFS | DFS |
---|---|---|
Data Structure | Queue | Stack |
Space Complexity | O(V) – can be high for wide graphs | O(h) where h is the height of the tree |
Completeness | Complete (finds a solution if one exists) | Not complete for infinite-depth trees |
Optimality | Optimal for unweighted graphs | Not optimal |
7. Optimizing BFS
While the basic BFS algorithm is efficient for many applications, there are several optimizations and variations that can improve its performance in specific scenarios:
1. Bidirectional BFS
When searching for a path between two nodes, running BFS simultaneously from both the start and end nodes can significantly reduce the search space. The search terminates when the two frontiers meet.
2. Early Termination
If you’re searching for a specific node or condition, you can terminate the BFS as soon as the goal is found, rather than exploring the entire graph.
3. Using a Bit Vector for Visited Nodes
For graphs with a known, finite number of nodes, using a bit vector instead of a set or hash table to mark visited nodes can be more memory-efficient.
4. Level-Aware BFS
If you need to keep track of the level (or distance from the start node), you can modify the BFS to process nodes level by level explicitly:
from collections import deque
def level_aware_bfs(graph, start):
visited = set([start])
queue = deque([(start, 0)]) # (node, level)
while queue:
vertex, level = queue.popleft()
print(f"Node {vertex} at level {level}")
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append((neighbor, level + 1))
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
level_aware_bfs(graph, 'A')
5. Memory-Efficient BFS for Large Graphs
For extremely large graphs that don’t fit in memory, you can implement an external memory BFS algorithm that uses disk storage to handle the frontier and visited set.
8. Common Interview Questions and Solutions
BFS is a popular topic in technical interviews, especially for roles at major tech companies. Here are some common BFS-related questions you might encounter, along with solution approaches:
1. Word Ladder Problem
Question: Given two words (beginWord and endWord), and a dictionary’s word list, find the length of shortest transformation sequence from beginWord to endWord, such that:
- Only one letter can be changed at a time.
- Each transformed word must exist in the word list.
Solution Approach: This problem can be solved using BFS, where each word is a node, and an edge exists between two words if they differ by exactly one letter. Here’s a Python implementation:
from collections import deque
def ladderLength(beginWord, endWord, wordList):
word_set = set(wordList)
if endWord not in word_set:
return 0
queue = deque([(beginWord, 1)])
visited = set([beginWord])
while queue:
word, length = queue.popleft()
if word == endWord:
return length
for i in range(len(word)):
for c in 'abcdefghijklmnopqrstuvwxyz':
next_word = word[:i] + c + word[i+1:]
if next_word in word_set and next_word not in visited:
visited.add(next_word)
queue.append((next_word, length + 1))
return 0
# Example usage
beginWord = "hit"
endWord = "cog"
wordList = ["hot","dot","dog","lot","log","cog"]
print(ladderLength(beginWord, endWord, wordList)) # Output: 5
2. Binary Tree Level Order Traversal
Question: Given a binary tree, return the level order traversal of its nodes’ values. (i.e., from left to right, level by level).
Solution Approach: This is a classic application of BFS. We can use a queue to keep track of nodes at each level:
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def levelOrder(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
level = []
for _ in range(level_size):
node = queue.popleft()
level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(level)
return result
# Example usage
root = TreeNode(3)
root.left = TreeNode(9)
root.right = TreeNode(20)
root.right.left = TreeNode(15)
root.right.right = TreeNode(7)
print(levelOrder(root)) # Output: [[3], [9, 20], [15, 7]]
3. Shortest Path in a Binary Matrix
Question: In an N by N square grid, each cell is either empty (0) or blocked (1). A clear path from top-left to bottom-right has length k if and only if it is composed of cells C_1, C_2, …, C_k where adjacent cells C_i and C_{i+1} are connected 8-directionally (i.e., they are different and share an edge or corner). Return the length of the shortest such clear path from top-left to bottom-right. If such a path does not exist, return -1.
Solution Approach: This problem can be solved using BFS, treating the matrix as a graph where each cell is a node, and adjacent cells are connected:
from collections import deque
def shortestPathBinaryMatrix(grid):
if grid[0][0] == 1 or grid[-1][-1] == 1:
return -1
n = len(grid)
directions = [
(-1,-1), (-1,0), (-1,1),
(0,-1), (0,1),
(1,-1), (1,0), (1,1)
]
queue = deque([(0, 0, 1)]) # (row, col, distance)
visited = set([(0, 0)])
while queue:
row, col, dist = queue.popleft()
if row == n - 1 and col == n - 1:
return dist
for dr, dc in directions:
r, c = row + dr, col + dc
if 0 <= r < n and 0 <= c < n and grid[r][c] == 0 and (r, c) not in visited:
visited.add((r, c))
queue.append((r, c, dist + 1))
return -1
# Example usage
grid = [
[0,0,0],
[1,1,0],
[1,1,0]
]
print(shortestPathBinaryMatrix(grid)) # Output: 4
9. Advanced BFS Techniques
As you become more proficient with BFS, you can explore advanced techniques that extend its capabilities or combine it with other algorithms:
1. A* Search Algorithm
A* is an informed search algorithm that combines elements of BFS with heuristics. It’s particularly useful for pathfinding and graph traversal. A* uses a best-first search and finds a least-cost path from a given initial node to one goal node (out of one or more possible goals).
2. Iterative Deepening BFS
This technique combines the space-efficiency of DFS with the level-wise exploration of BFS. It repeatedly applies BFS with increasing depth limits until the goal is found.
3. Multi-Source BFS
In some problems, you might need to start BFS from multiple source nodes simultaneously. This can be achieved by initializing the queue with all source nodes.
4. BFS on Implicit Graphs
Sometimes, the graph is not explicitly given but is implied by the problem constraints. For example, in a chess knight’s tour problem, the graph of possible moves is generated on-the-fly during the BFS.
5. Parallel BFS
For very large graphs, you can implement a parallel version of BFS that distributes the workload across multiple processors or machines.
6. BFS with Priority Queue
In some scenarios, you might want to prioritize certain nodes over others during the traversal. Using a priority queue instead of a regular queue can achieve this effect.
Example: BFS with Priority Queue
import heapq
def bfs_with_priority(graph, start, priority_func):
visited = set()
pq = [(priority_func(start), 0, start)] # (priority, step, node)
visited.add(start)
while pq:
_, step, node = heapq.heappop(pq)
print(f"Visiting {node} at step {step}")
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
priority = priority_func(neighbor)
heapq.heappush(pq, (priority, step + 1, neighbor))
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
# Priority function (lower values have higher priority)
def priority_func(node):
return ord(node) - ord('A')
bfs_with_priority(graph, 'A', priority_func)
This implementation uses a priority queue to determine the order in which nodes are visited. The priority function can be customized based on the specific requirements of the problem.
10. Conclusion
Mastering Breadth-First Search is an essential skill for any programmer or computer scientist. Its versatility and efficiency make it a go-to algorithm for a wide range of problems, from pathfinding to social network analysis. By understanding the core principles of BFS, its implementations, optimizations, and advanced techniques, you’ll be well-equipped to tackle complex graph problems and excel in technical interviews.
Remember that practice is key to truly mastering BFS. Try implementing the algorithm from scratch, solve various problems that utilize BFS, and experiment with different optimizations and variations. As you gain more experience, you’ll develop an intuition for when and how to apply BFS most effectively.
Keep exploring, keep coding, and don’t hesitate to dive deeper into the fascinating world of graph algorithms. Happy coding!