Mastering Binary Search Tree Problems: Essential Techniques for Coding Interviews
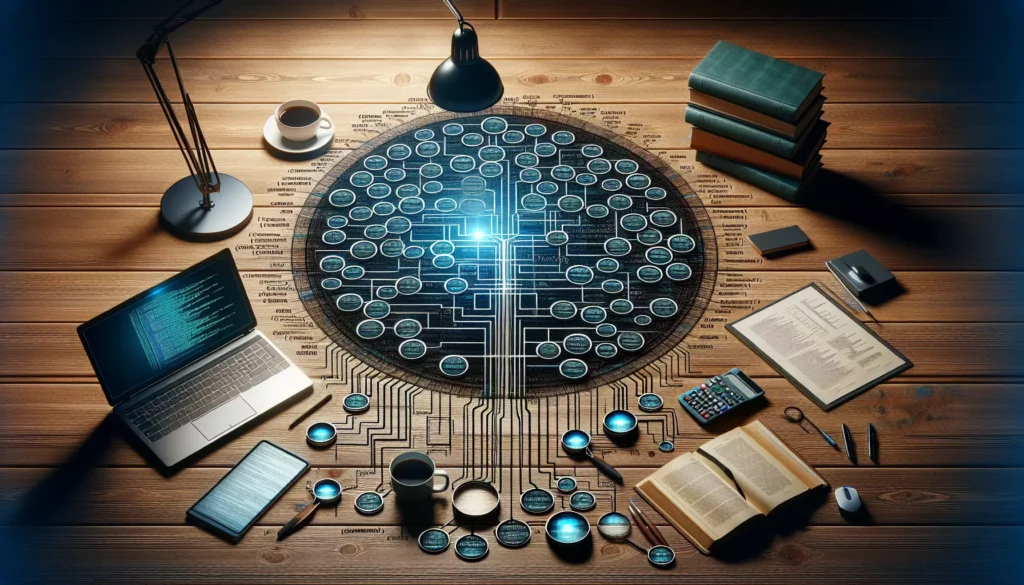
Binary Search Trees (BSTs) are fundamental data structures in computer science, often featured prominently in coding interviews, especially those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). As aspiring software engineers and developers prepare for these challenging interviews, understanding and mastering BST problems becomes crucial. In this comprehensive guide, we’ll explore various techniques for solving Binary Search Tree problems, providing you with the tools and knowledge to excel in your coding interviews and enhance your overall programming skills.
Understanding Binary Search Trees
Before diving into problem-solving techniques, let’s quickly review what a Binary Search Tree is and its key properties:
- A BST is a binary tree where each node has at most two children.
- For each node, all elements in its left subtree are smaller than the node, and all elements in its right subtree are larger.
- This property holds true for every node in the tree.
- BSTs allow for efficient searching, insertion, and deletion operations, typically with an average time complexity of O(log n).
With this foundation, let’s explore the techniques that will help you tackle BST problems effectively.
1. Recursive Traversal
Recursive traversal is one of the most fundamental techniques for working with BSTs. It leverages the tree’s recursive structure to perform operations on each node. There are three main types of recursive traversals:
a) In-order Traversal
In-order traversal visits the left subtree, then the current node, and finally the right subtree. This traversal gives nodes in ascending order for a BST.
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val)
inorder_traversal(root.right)
b) Pre-order Traversal
Pre-order traversal visits the current node before its children. This is useful for creating a copy of the tree or generating a prefix expression.
def preorder_traversal(root):
if root:
print(root.val)
preorder_traversal(root.left)
preorder_traversal(root.right)
c) Post-order Traversal
Post-order traversal visits the children before the current node. This is often used when deleting nodes or evaluating expressions.
def postorder_traversal(root):
if root:
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.val)
These traversal techniques form the basis for many BST operations and are essential to master for coding interviews.
2. Iterative Traversal
While recursive traversals are intuitive, iterative approaches can be more efficient in terms of space complexity. Iterative traversals use an explicit stack or queue to keep track of nodes.
Iterative In-order Traversal
def iterative_inorder(root):
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
print(current.val)
current = current.right
This iterative approach mimics the recursive in-order traversal but uses a stack to keep track of nodes to visit.
3. Level-order Traversal (BFS)
Level-order traversal, also known as Breadth-First Search (BFS), visits nodes level by level from top to bottom. This technique is useful for problems that require processing nodes based on their depth or finding the shortest path.
from collections import deque
def level_order_traversal(root):
if not root:
return
queue = deque([root])
while queue:
level_size = len(queue)
for _ in range(level_size):
node = queue.popleft()
print(node.val, end=' ')
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
print() # New line for each level
This approach uses a queue to process nodes in a first-in-first-out (FIFO) order, ensuring that nodes are visited level by level.
4. Binary Search Technique
The binary search technique leverages the BST property to efficiently search, insert, or delete nodes. This technique is crucial for maintaining the BST structure and achieving O(log n) time complexity for these operations.
Searching in a BST
def search_bst(root, val):
if not root or root.val == val:
return root
if val < root.val:
return search_bst(root.left, val)
return search_bst(root.right, val)
Inserting into a BST
def insert_bst(root, val):
if not root:
return TreeNode(val)
if val < root.val:
root.left = insert_bst(root.left, val)
else:
root.right = insert_bst(root.right, val)
return root
These operations demonstrate how the binary search property of BSTs can be used to efficiently navigate and modify the tree structure.
5. Tree Construction Techniques
Constructing a BST from given data is a common problem in coding interviews. Two primary approaches are often used:
a) Insertion-based Construction
This method involves inserting nodes one by one into an initially empty tree.
def construct_bst(values):
root = None
for val in values:
root = insert_bst(root, val)
return root
b) Sorted Array to BST
When given a sorted array, we can construct a balanced BST by recursively choosing the middle element as the root.
def sorted_array_to_bst(nums):
if not nums:
return None
mid = len(nums) // 2
root = TreeNode(nums[mid])
root.left = sorted_array_to_bst(nums[:mid])
root.right = sorted_array_to_bst(nums[mid+1:])
return root
Understanding these construction techniques is crucial for problems involving BST creation or conversion from other data structures.
6. Tree Balancing Techniques
Maintaining balance in a BST is crucial for ensuring optimal performance. While perfect balance is not always achievable, several techniques can help keep the tree relatively balanced:
a) AVL Trees
AVL trees are self-balancing BSTs that maintain a balance factor (difference in height between left and right subtrees) of at most 1 for every node. When this balance is violated, rotations are performed to restore balance.
class AVLNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
self.height = 1
def insert_avl(root, key):
if not root:
return AVLNode(key)
if key < root.val:
root.left = insert_avl(root.left, key)
else:
root.right = insert_avl(root.right, key)
root.height = 1 + max(get_height(root.left), get_height(root.right))
balance = get_balance(root)
# Left Left Case
if balance > 1 and key < root.left.val:
return right_rotate(root)
# Right Right Case
if balance < -1 and key > root.right.val:
return left_rotate(root)
# Left Right Case
if balance > 1 and key > root.left.val:
root.left = left_rotate(root.left)
return right_rotate(root)
# Right Left Case
if balance < -1 and key < root.right.val:
root.right = right_rotate(root.right)
return left_rotate(root)
return root
def get_height(node):
if not node:
return 0
return node.height
def get_balance(node):
if not node:
return 0
return get_height(node.left) - get_height(node.right)
def left_rotate(z):
y = z.right
T2 = y.left
y.left = z
z.right = T2
z.height = 1 + max(get_height(z.left), get_height(z.right))
y.height = 1 + max(get_height(y.left), get_height(y.right))
return y
def right_rotate(y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
y.height = 1 + max(get_height(y.left), get_height(y.right))
x.height = 1 + max(get_height(x.left), get_height(x.right))
return x
b) Red-Black Trees
Red-Black trees are another type of self-balancing BST that use color properties and rotations to maintain balance. While more complex than AVL trees, they often provide better performance for insertion and deletion operations.
Understanding these balancing techniques is important for advanced BST problems and for optimizing BST-based data structures in real-world applications.
7. Path-based Techniques
Many BST problems involve finding or manipulating paths within the tree. These techniques are crucial for solving problems related to tree properties, node relationships, and tree transformations.
a) Path Sum
Finding paths that sum to a given value is a common problem. This can be solved using recursion and backtracking.
def has_path_sum(root, target_sum):
if not root:
return False
if not root.left and not root.right:
return root.val == target_sum
return has_path_sum(root.left, target_sum - root.val) or \
has_path_sum(root.right, target_sum - root.val)
b) Lowest Common Ancestor (LCA)
Finding the lowest common ancestor of two nodes is another frequently asked question in interviews.
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
c) Maximum Path Sum
Finding the maximum path sum in a binary tree is a more challenging problem that requires careful consideration of negative values.
class Solution:
def max_path_sum(self, root):
self.max_sum = float('-inf')
def max_gain(node):
if not node:
return 0
left_gain = max(max_gain(node.left), 0)
right_gain = max(max_gain(node.right), 0)
path_sum = node.val + left_gain + right_gain
self.max_sum = max(self.max_sum, path_sum)
return node.val + max(left_gain, right_gain)
max_gain(root)
return self.max_sum
These path-based techniques are powerful tools for solving a wide range of BST problems and are often key to cracking difficult interview questions.
8. Tree Modification Techniques
Modifying BSTs while maintaining their properties is a crucial skill. These techniques involve careful manipulation of node connections and often require a deep understanding of BST properties.
a) Flattening a BST to a Linked List
This problem involves converting a BST into a right-skewed tree (essentially a linked list).
class Solution:
def flatten(self, root):
if not root:
return None
self.flatten(root.left)
self.flatten(root.right)
if root.left:
current = root.left
while current.right:
current = current.right
current.right = root.right
root.right = root.left
root.left = None
b) Converting BST to Greater Tree
This technique involves modifying each node’s value to be the sum of its original value and all greater values in the BST.
class Solution:
def convert_bst(self, root):
self.sum = 0
def traverse(node):
if not node:
return
traverse(node.right)
self.sum += node.val
node.val = self.sum
traverse(node.left)
traverse(root)
return root
c) Pruning a BST
Pruning involves removing nodes from a BST based on certain conditions while maintaining the BST property.
def prune_bst(root, low, high):
if not root:
return None
if root.val > high:
return prune_bst(root.left, low, high)
if root.val < low:
return prune_bst(root.right, low, high)
root.left = prune_bst(root.left, low, high)
root.right = prune_bst(root.right, low, high)
return root
These modification techniques demonstrate the flexibility of BSTs and are often used to transform trees for specific use cases or to optimize certain operations.
9. BST Property Verification
Verifying whether a given binary tree is a valid BST is a common interview question. This technique requires careful consideration of node values and their relationships.
def is_valid_bst(root):
def validate(node, low=float('-inf'), high=float('inf')):
if not node:
return True
if node.val <= low or node.val >= high:
return False
return validate(node.left, low, node.val) and validate(node.right, node.val, high)
return validate(root)
This recursive approach checks each node against a valid range, which is updated as we traverse down the tree. Understanding this technique is crucial for problems involving BST validation or modification.
10. Serialization and Deserialization
Serialization (converting a BST to a string) and deserialization (reconstructing a BST from a string) are advanced techniques often encountered in system design questions or when dealing with data persistence.
class Codec:
def serialize(self, root):
if not root:
return 'null'
return f"{root.val},{self.serialize(root.left)},{self.serialize(root.right)}"
def deserialize(self, data):
def dfs():
val = next(values)
if val == 'null':
return None
node = TreeNode(int(val))
node.left = dfs()
node.right = dfs()
return node
values = iter(data.split(','))
return dfs()
This technique allows for efficient storage and transmission of BSTs, which is particularly useful in distributed systems or when working with large-scale data structures.
Conclusion
Mastering these techniques for solving Binary Search Tree problems is essential for success in coding interviews, particularly those conducted by major tech companies. These methods not only prepare you for specific BST-related questions but also enhance your overall problem-solving skills and understanding of tree-based data structures.
Remember, the key to excelling in BST problems lies in practice and understanding the underlying principles. As you work through different problems, try to identify which techniques are most applicable and how they can be combined or adapted to solve more complex challenges.
By incorporating these techniques into your problem-solving toolkit, you’ll be well-equipped to tackle a wide range of BST problems in your coding interviews and beyond. Keep practicing, stay curious, and don’t hesitate to explore variations and optimizations of these techniques as you encounter new and challenging problems.