Mastering Binary Search: Strategies for Tackling Algorithm Questions
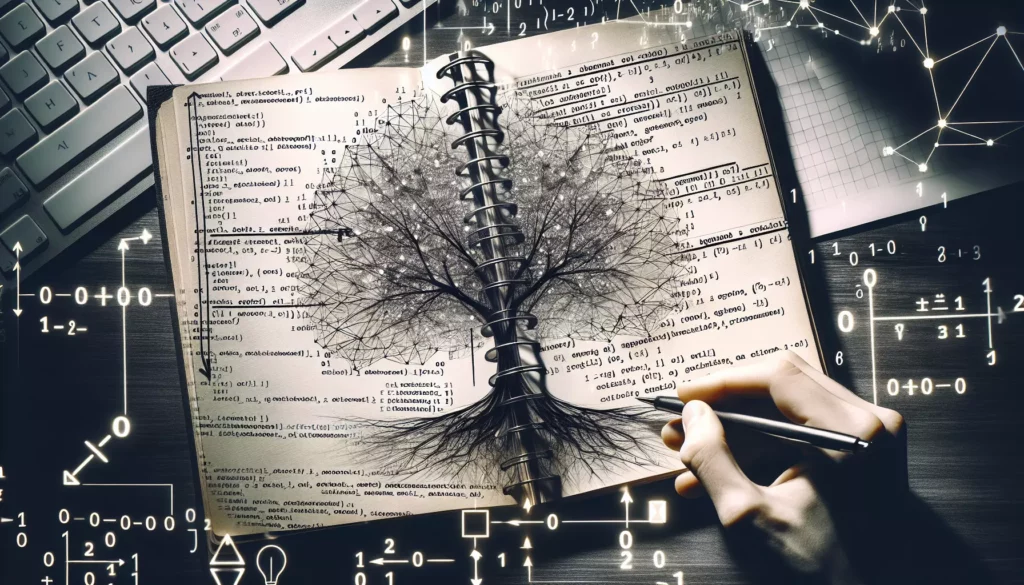
In the world of coding interviews and competitive programming, the binary search algorithm stands out as a powerful tool for efficiently solving a wide range of problems. Whether you’re preparing for technical interviews at top tech companies or honing your algorithmic skills, understanding and mastering binary search is crucial. In this comprehensive guide, we’ll explore various strategies for approaching binary search algorithm questions, providing you with the knowledge and techniques to excel in your coding journey.
Understanding Binary Search
Before diving into specific strategies, let’s briefly review what binary search is and why it’s so important in the realm of algorithms and data structures.
Binary search is a divide-and-conquer algorithm used to efficiently locate a target value within a sorted array. Its time complexity is O(log n), making it significantly faster than linear search for large datasets. The basic idea is to repeatedly divide the search interval in half, narrowing down the possible locations of the target value until it’s found or determined to be absent.
The Classic Binary Search Algorithm
Here’s a simple implementation of the classic binary search algorithm in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
This implementation returns the index of the target value if found, or -1 if the target is not present in the array.
Strategy 1: Identify the Search Space
One of the most crucial aspects of solving binary search problems is correctly identifying the search space. The search space is the range of possible values or indices where the solution might exist. Here are some tips for identifying and working with the search space:
- Determine the boundaries: Clearly define the left and right boundaries of your search space. In a sorted array, this is typically 0 and len(arr) – 1.
- Consider implicit search spaces: Sometimes, the search space isn’t explicitly given as an array. For example, in problems involving finding a square root or determining a minimum value that satisfies a condition, you need to identify the range of possible answers.
- Handle edge cases: Always consider the extremes of your search space. What happens at the boundaries? Are there any special cases to handle?
Example: Square Root Problem
Let’s look at an example where identifying the search space is crucial:
def mySqrt(x):
if x < 2:
return x
left, right = 2, x // 2
while left <= right:
mid = left + (right - left) // 2
square = mid * mid
if square == x:
return mid
elif square < x:
left = mid + 1
else:
right = mid - 1
return right # Return the floor of the square root
# Example usage
x = 16
result = mySqrt(x)
print(f"The square root of {x} is: {result}")
In this problem, we’re finding the floor of the square root of a given number. The search space is from 2 to x//2, as the square root can’t be larger than half of the number itself (for numbers greater than 2).
Strategy 2: Choosing the Right Comparison
The heart of the binary search algorithm lies in the comparison made at each step. Choosing the right comparison is crucial for the algorithm’s correctness and efficiency. Here are some tips for selecting and implementing the right comparison:
- Understand the problem requirements: Clearly identify what you’re searching for and how it relates to the elements in your search space.
- Use appropriate comparison operators: Depending on the problem, you might need to use <, <=, >, or >= in your comparisons.
- Handle equality cases: Decide how to handle cases where the middle element is equal to your target. Sometimes you might want to continue searching in one direction even if you’ve found a match.
- Consider complex comparisons: In some problems, the comparison might involve calling a function or performing a calculation rather than a simple numeric comparison.
Example: Search in Rotated Sorted Array
Here’s an example where choosing the right comparison is crucial:
def search(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
# Check if the left half is sorted
if nums[left] <= nums[mid]:
if nums[left] <= target < nums[mid]:
right = mid - 1
else:
left = mid + 1
# Right half must be sorted
else:
if nums[mid] < target <= nums[right]:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
rotated_array = [4, 5, 6, 7, 0, 1, 2]
target = 0
result = search(rotated_array, target)
print(f"Target {target} found at index: {result}")
In this problem, we’re searching for a target in a rotated sorted array. The comparison logic is more complex because we need to determine which half of the array is sorted and whether the target lies within that sorted half.
Strategy 3: Handling Duplicates
Many binary search problems become more challenging when the input contains duplicate elements. Here are some strategies for dealing with duplicates:
- Decide on the desired behavior: Determine whether you want to find the first occurrence, last occurrence, or any occurrence of the target value.
- Modify the comparison logic: Adjust your comparisons to handle duplicate values correctly.
- Use additional checks: Sometimes you may need to perform extra checks or move the pointers in a specific way to handle duplicates.
- Consider using two binary searches: For problems like finding the range of a target value, you might need to perform two separate binary searches (one for the left bound and one for the right bound).
Example: Find First and Last Position of Element in Sorted Array
Here’s an example of handling duplicates in a binary search problem:
def searchRange(nums, target):
def findBound(nums, target, isFirst):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
if isFirst:
if mid == left or nums[mid-1] < target:
return mid
right = mid - 1
else:
if mid == right or nums[mid+1] > target:
return mid
left = mid + 1
elif nums[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
first = findBound(nums, target, True)
if first == -1:
return [-1, -1]
last = findBound(nums, target, False)
return [first, last]
# Example usage
sorted_array = [5,7,7,8,8,10]
target = 8
result = searchRange(sorted_array, target)
print(f"Range of target {target}: {result}")
This solution uses two separate binary searches to find the first and last occurrences of the target value in a sorted array with possible duplicates.
Strategy 4: Binary Search on Answer
Sometimes, binary search can be applied not to the input array itself, but to the range of possible answers. This technique is often called “binary search on answer” or “guess and check.” Here’s how to approach such problems:
- Identify the range of possible answers: Determine the minimum and maximum possible values for your answer.
- Implement a check function: Create a function that can verify whether a given value is a valid answer.
- Apply binary search on the answer range: Use binary search to narrow down the correct answer by repeatedly checking the middle value.
- Optimize the check function: Ensure your check function is efficient, as it will be called multiple times during the binary search.
Example: Koko Eating Bananas
Here’s an example of applying binary search on the answer space:
from math import ceil
def minEatingSpeed(piles, h):
def canEatAll(speed):
return sum(ceil(pile / speed) for pile in piles) <= h
left, right = 1, max(piles)
while left < right:
mid = (left + right) // 2
if canEatAll(mid):
right = mid
else:
left = mid + 1
return left
# Example usage
piles = [3,6,7,11]
h = 8
result = minEatingSpeed(piles, h)
print(f"Minimum eating speed: {result}")
In this problem, we’re finding the minimum eating speed at which Koko can eat all the bananas within h hours. We apply binary search on the range of possible eating speeds (1 to max(piles)) and use a check function to determine if a given speed is sufficient.
Strategy 5: Template Method for Binary Search
Developing a template for binary search can help you approach a wide variety of problems more systematically. Here’s a general template that can be adapted for many binary search scenarios:
def binary_search_template(array):
def condition(value) -> bool:
# Implement the condition here
pass
left, right = min(search_space), max(search_space)
while left < right:
mid = left + (right - left) // 2
if condition(mid):
right = mid
else:
left = mid + 1
return left
# Example usage
result = binary_search_template(some_array)
print(f"Result: {result}")
This template uses a few key concepts:
- The
condition
function encapsulates the logic for determining whether the current value satisfies the problem requirements. - The search continues while
left < right
, ensuring that the loop terminates when left and right converge. - The mid-point calculation
left + (right - left) // 2
avoids potential integer overflow. - The template is designed to find the leftmost value that satisfies the condition.
By adapting this template to specific problems, you can tackle a wide range of binary search questions more efficiently.
Strategy 6: Optimizing for Edge Cases
Edge cases can often be the downfall of an otherwise correct binary search implementation. Here are some strategies for handling edge cases effectively:
- Consider empty or single-element arrays: Always test your algorithm with these minimal inputs.
- Handle out-of-bounds targets: Ensure your algorithm behaves correctly when the target is smaller than the smallest element or larger than the largest element in the array.
- Test with repeated elements: If your problem allows for duplicates, test thoroughly with arrays containing multiple occurrences of the same value.
- Check boundary conditions: Pay special attention to what happens at the edges of your search space, especially when the target is at the very beginning or end of the array.
Example: Search Insert Position
Here’s an example that demonstrates handling various edge cases:
def searchInsert(nums, target):
left, right = 0, len(nums)
while left < right:
mid = (left + right) // 2
if nums[mid] < target:
left = mid + 1
else:
right = mid
return left
# Example usage
sorted_array = [1,3,5,6]
targets = [5, 2, 7, 0]
for target in targets:
result = searchInsert(sorted_array, target)
print(f"Insert position for target {target}: {result}")
This implementation correctly handles cases where the target is not in the array, including when it should be inserted at the beginning or end of the array.
Strategy 7: Applying Binary Search to Complex Problems
Binary search isn’t limited to simple sorted arrays. It can be applied to more complex problems and data structures. Here are some tips for recognizing and applying binary search in non-standard scenarios:
- Look for monotonic properties: Binary search can be applied whenever there’s a monotonically increasing or decreasing property in the problem space.
- Consider binary search on derived values: Sometimes, you need to apply binary search not on the input directly, but on some function of the input.
- Combine with other algorithms: Binary search can often be used as part of a larger algorithm, perhaps in combination with dynamic programming or graph algorithms.
- Think about binary decisions: Even if the problem doesn’t involve searching an array, consider if you can frame it as a series of binary decisions.
Example: Find Minimum in Rotated Sorted Array
Here’s an example of applying binary search to a more complex scenario:
def findMin(nums):
left, right = 0, len(nums) - 1
while left < right:
mid = (left + right) // 2
if nums[mid] > nums[right]:
left = mid + 1
else:
right = mid
return nums[left]
# Example usage
rotated_array = [4,5,6,7,0,1,2]
result = findMin(rotated_array)
print(f"Minimum element: {result}")
This problem applies binary search to find the minimum element in a rotated sorted array, demonstrating how binary search can be used in scenarios beyond simple sorted array searches.
Conclusion
Mastering binary search is a crucial skill for any programmer preparing for technical interviews or competitive programming. By understanding and applying these strategies, you’ll be well-equipped to tackle a wide range of binary search problems:
- Identify the search space
- Choose the right comparison
- Handle duplicates effectively
- Apply binary search on the answer space
- Use a template method for consistency
- Optimize for edge cases
- Recognize and apply binary search in complex scenarios
Remember, practice is key to internalizing these strategies. Work through a variety of binary search problems, applying these techniques, and you’ll soon find yourself confidently solving even the most challenging binary search questions in your coding interviews and competitions.
Keep honing your skills, and don’t hesitate to revisit these strategies as you encounter new and more complex binary search problems. With persistence and practice, you’ll master the art of binary search and significantly enhance your problem-solving toolkit.