Mastering Binary Search: A Comprehensive Guide to Solving Complex Problems
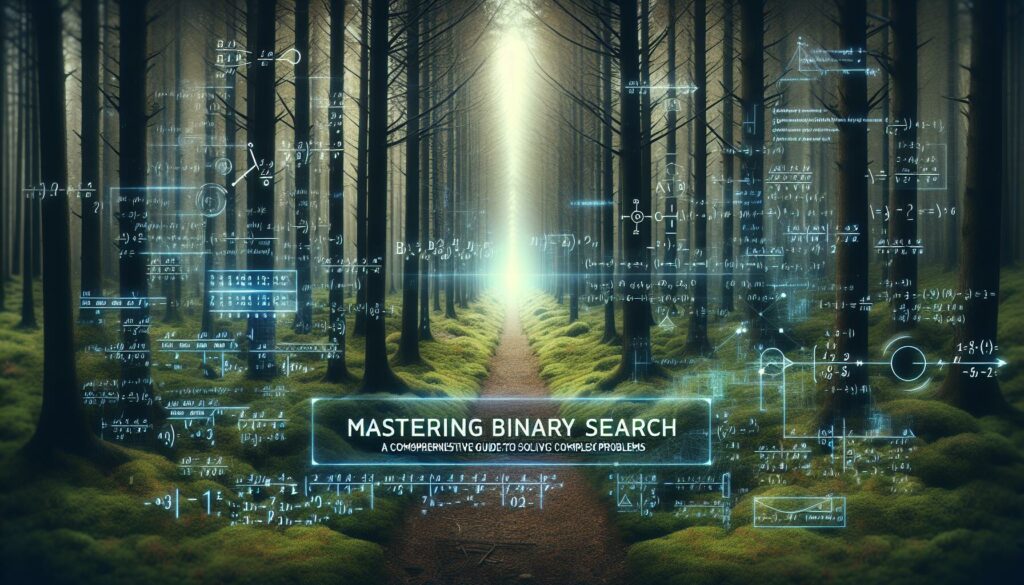
Binary search is a fundamental algorithm in computer science that extends far beyond its basic application of finding an element in a sorted array. This powerful technique can be applied to a wide range of problems, making it an essential tool in a programmer’s toolkit. In this comprehensive guide, we’ll explore the versatility of binary search and how it can be used to solve various complex problems efficiently.
1. Introduction to Binary Search
Before diving into advanced applications, let’s quickly review the basics of binary search. At its core, binary search is a divide-and-conquer algorithm that works on sorted data. It repeatedly divides the search interval in half, significantly reducing the search space with each iteration.
1.1 The Classic Binary Search
The most common application of binary search is finding an element in a sorted array. Here’s a simple implementation in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Element not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
This implementation has a time complexity of O(log n), making it extremely efficient for large datasets.
2. Advanced Binary Search Applications
Now, let’s explore some of the more advanced and less obvious applications of binary search.
2.1 Search in an Infinite Sorted Array
Imagine you have a sorted array so large that its size is unknown or practically infinite. How would you search for an element in such an array?
The solution involves two steps:
- Find a range where the element might exist
- Perform a binary search within that range
Here’s an implementation:
def search_infinite_array(arr, target):
# Step 1: Find a range for binary search
left, right = 0, 1
while arr[right] < target:
left = right
right *= 2
# Step 2: Perform binary search
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Element not found
# Example usage (assume arr is a very large sorted array)
result = search_infinite_array(arr, 100)
print(f"Element found at index: {result}")
This approach efficiently handles arrays of unknown or infinite size by exponentially increasing the search range until a suitable window is found.
2.2 Finding the First or Last Occurrence
When dealing with sorted arrays containing duplicates, you might need to find the first or last occurrence of a specific element. Binary search can be modified to handle this scenario efficiently.
def find_first_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
result = mid
right = mid - 1 # Continue searching towards left
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return result
# Example usage
sorted_array_with_duplicates = [1, 2, 2, 2, 3, 4, 5]
first_occurrence = find_first_occurrence(sorted_array_with_duplicates, 2)
print(f"First occurrence of 2 is at index: {first_occurrence}")
To find the last occurrence, you would modify the algorithm to continue searching towards the right when a match is found.
2.3 Search on a Monotonic Function
Binary search can be applied to monotonic functions, which are functions that are either entirely non-increasing or non-decreasing. This is particularly useful in optimization problems.
Consider finding the square root of a number up to a certain precision:
def square_root(n, precision=1e-6):
if n < 0:
return None # Square root of negative numbers is not real
left, right = 0, max(1, n)
while right - left > precision:
mid = (left + right) / 2
if mid * mid > n:
right = mid
else:
left = mid
return (left + right) / 2
# Example usage
result = square_root(16)
print(f"Square root of 16 is approximately: {result}")
This algorithm efficiently narrows down the range where the square root lies, providing a result within the specified precision.
2.4 Finding Peak in a Bitonic Array
A bitonic array is one that first increases and then decreases. Finding the peak element in such an array can be done efficiently using binary search.
def find_peak_bitonic(arr):
left, right = 0, len(arr) - 1
while left < right:
mid = (left + right) // 2
if arr[mid] < arr[mid + 1]:
left = mid + 1
else:
right = mid
return left # Index of the peak element
# Example usage
bitonic_array = [1, 3, 8, 12, 4, 2]
peak_index = find_peak_bitonic(bitonic_array)
print(f"Peak element {bitonic_array[peak_index]} found at index: {peak_index}")
This algorithm efficiently locates the peak by comparing middle elements with their neighbors and adjusting the search range accordingly.
3. Binary Search in Problem-Solving
Binary search is not just limited to searching in arrays. It’s a powerful problem-solving technique that can be applied to various scenarios where the search space can be efficiently divided.
3.1 Allocating Minimum Pages
Consider a problem where you need to allocate a number of books to students such that the maximum number of pages assigned to a student is minimized. This is a classic example of using binary search on the answer space.
def can_allocate(arr, n, m, max_pages):
students = 1
pages = 0
for pages_in_book in arr:
if pages_in_book > max_pages:
return False
if pages + pages_in_book > max_pages:
students += 1
pages = pages_in_book
if students > m:
return False
else:
pages += pages_in_book
return True
def allocate_minimum_pages(arr, m):
if len(arr) < m:
return -1 # Not enough books for all students
left = max(arr)
right = sum(arr)
result = -1
while left <= right:
mid = (left + right) // 2
if can_allocate(arr, len(arr), m, mid):
result = mid
right = mid - 1
else:
left = mid + 1
return result
# Example usage
books = [12, 34, 67, 90]
students = 2
min_pages = allocate_minimum_pages(books, students)
print(f"Minimum number of maximum pages: {min_pages}")
This problem demonstrates how binary search can be applied to optimization problems by searching over the possible answer space.
3.2 Search in a 2D Sorted Matrix
Binary search can be extended to two-dimensional structures like sorted matrices. Here’s an efficient approach to search in a matrix where each row and column is sorted:
def search_2d_matrix(matrix, target):
if not matrix or not matrix[0]:
return False
rows, cols = len(matrix), len(matrix[0])
left, right = 0, rows * cols - 1
while left <= right:
mid = (left + right) // 2
mid_value = matrix[mid // cols][mid % cols]
if mid_value == target:
return True
elif mid_value < target:
left = mid + 1
else:
right = mid - 1
return False
# Example usage
matrix = [
[1, 3, 5, 7],
[10, 11, 16, 20],
[23, 30, 34, 50]
]
target = 3
result = search_2d_matrix(matrix, target)
print(f"Target {target} found: {result}")
This approach treats the 2D matrix as a flattened sorted array, allowing for an efficient binary search implementation.
4. Binary Search in Real-World Applications
The applications of binary search extend far beyond academic problems. Let’s explore some real-world scenarios where binary search plays a crucial role.
4.1 Database Indexing
In database systems, binary search is fundamental to the implementation of indexing structures like B-trees. These structures allow for efficient searching, insertion, and deletion of records in large datasets.
For instance, when you perform a query on an indexed column in a database, the database engine often uses a variation of binary search to quickly locate the relevant records.
4.2 IP Routing Tables
In computer networking, routers use binary search (or its variations) to quickly look up the next hop for an IP packet in their routing tables. This is crucial for maintaining high-speed internet connectivity.
4.3 Version Control Systems
Git, a popular version control system, uses binary search in its “git bisect” feature. This feature helps developers find the commit that introduced a bug by systematically checking the middle point between a known good commit and a known bad commit.
4.4 Machine Learning
In machine learning, binary search is used in various optimization algorithms. For example, in hyperparameter tuning, binary search can be employed to efficiently find optimal values for model parameters.
5. Optimizing Binary Search
While binary search is already efficient, there are ways to optimize it further in certain scenarios.
5.1 Avoiding Integer Overflow
In languages prone to integer overflow, the calculation of the midpoint can be problematic. Instead of (left + right) // 2
, you can use:
mid = left + (right - left) // 2
This formulation avoids potential overflow issues when left + right
exceeds the maximum integer value.
5.2 Interpolation Search
For uniformly distributed sorted arrays, interpolation search can be more efficient than binary search. It estimates the likely position of the target value based on the distribution of values in the array.
def interpolation_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right and arr[left] <= target <= arr[right]:
if left == right:
if arr[left] == target:
return left
return -1
pos = left + ((target - arr[left]) * (right - left)) // (arr[right] - arr[left])
if arr[pos] == target:
return pos
elif arr[pos] < target:
left = pos + 1
else:
right = pos - 1
return -1
# Example usage
sorted_array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = interpolation_search(sorted_array, 7)
print(f"Element found at index: {result}")
Interpolation search can achieve O(log log n) time complexity for uniformly distributed data, compared to O(log n) for standard binary search.
6. Common Pitfalls and Best Practices
When implementing binary search, be aware of these common pitfalls and best practices:
6.1 Off-by-One Errors
One of the most common errors in binary search implementations is the off-by-one error. Always double-check your boundary conditions, especially when updating left
and right
pointers.
6.2 Infinite Loops
Ensure that your termination condition is correct and that the search space is actually reducing in each iteration to avoid infinite loops.
6.3 Handling Empty Arrays
Always check for empty arrays or invalid input before starting the binary search algorithm.
6.4 Testing Edge Cases
Test your binary search implementation with various edge cases, including:
- Arrays with a single element
- Target element at the beginning or end of the array
- Target element not present in the array
- Arrays with duplicate elements
7. Conclusion
Binary search is a powerful algorithm that extends far beyond its basic application of searching in sorted arrays. Its efficiency and versatility make it an indispensable tool in a programmer’s arsenal. From solving complex algorithmic problems to optimizing real-world applications, binary search continues to play a crucial role in computer science and software engineering.
As you progress in your coding journey, mastering binary search and understanding its various applications will significantly enhance your problem-solving skills. Whether you’re preparing for technical interviews, optimizing database queries, or developing efficient algorithms, the principles of binary search will serve you well.
Remember, the key to mastering binary search lies not just in understanding its basic implementation, but in recognizing the patterns and problems where it can be applied creatively. Practice implementing binary search in various scenarios, and you’ll find it becoming an intuitive part of your problem-solving approach.
Happy coding, and may your searches always be efficient!