Mastering Anagram Problems: Techniques and Strategies for Efficient Solutions
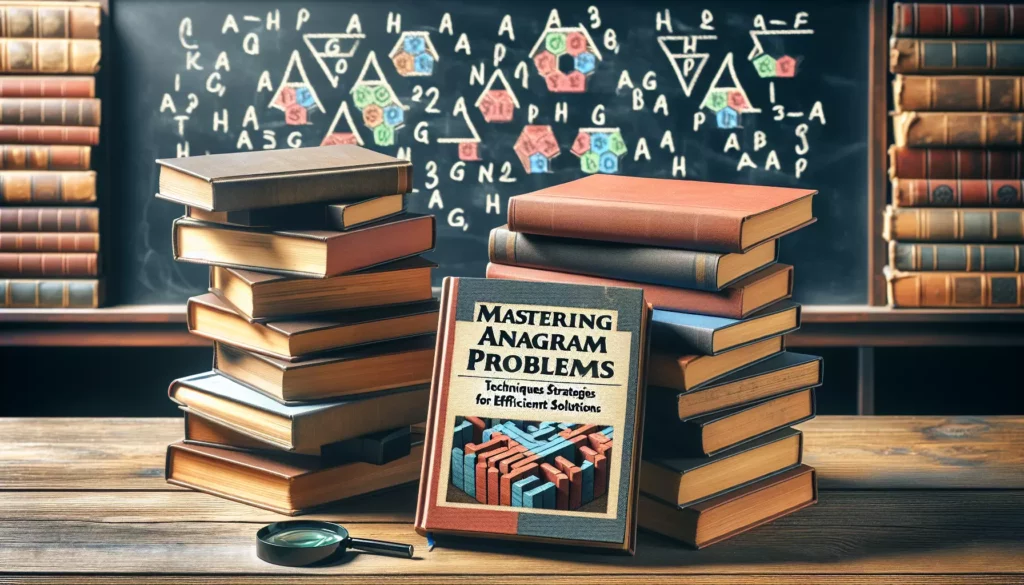
Anagram problems are a common challenge in coding interviews and algorithmic competitions. These problems test a programmer’s ability to manipulate strings, work with data structures, and optimize for efficiency. In this comprehensive guide, we’ll explore various techniques for solving anagram problems, ranging from basic approaches to advanced algorithms. Whether you’re preparing for a technical interview at a FAANG company or simply looking to improve your coding skills, mastering these techniques will prove invaluable.
What is an Anagram?
Before diving into solution techniques, let’s first define what an anagram is. An anagram is a word or phrase formed by rearranging the letters of another word or phrase, typically using all the original letters exactly once. For example:
- “listen” is an anagram of “silent”
- “debit card” is an anagram of “bad credit”
- “astronomer” is an anagram of “moon starer”
In programming contexts, anagram problems often involve determining whether two strings are anagrams of each other, finding all anagrams of a given word in a dictionary, or grouping anagrams from a list of words.
Basic Technique: Sorting
One of the simplest approaches to solve anagram problems is by sorting the characters of the strings and then comparing them. If two strings are anagrams, they will have the same characters in the same quantities, just in a different order. By sorting both strings, we bring them into a common order for easy comparison.
Algorithm:
- Convert both strings to lowercase (if case-insensitive comparison is required)
- Sort the characters of both strings
- Compare the sorted strings
Python Implementation:
def are_anagrams(str1, str2):
# Convert to lowercase and remove non-alphanumeric characters
str1 = ''.join(e.lower() for e in str1 if e.isalnum())
str2 = ''.join(e.lower() for e in str2 if e.isalnum())
# Sort the strings and compare
return sorted(str1) == sorted(str2)
# Example usage
print(are_anagrams("listen", "silent")) # Output: True
print(are_anagrams("hello", "world")) # Output: False
This approach is straightforward and easy to implement. However, it has a time complexity of O(n log n) due to the sorting operation, where n is the length of the string. For small strings, this method is perfectly acceptable, but for larger strings or when dealing with a large number of comparisons, more efficient methods may be necessary.
Intermediate Technique: Character Count
A more efficient approach is to count the occurrences of each character in both strings and compare the counts. This method avoids the need for sorting and can be implemented with a single pass through each string.
Algorithm:
- Create a dictionary or array to store character counts
- Iterate through the first string, incrementing counts for each character
- Iterate through the second string, decrementing counts for each character
- Check if all counts in the dictionary/array are zero
Python Implementation:
from collections import defaultdict
def are_anagrams(str1, str2):
# Convert to lowercase and remove non-alphanumeric characters
str1 = ''.join(e.lower() for e in str1 if e.isalnum())
str2 = ''.join(e.lower() for e in str2 if e.isalnum())
# Check if lengths are different
if len(str1) != len(str2):
return False
# Count characters
char_count = defaultdict(int)
for char in str1:
char_count[char] += 1
for char in str2:
char_count[char] -= 1
if char_count[char] < 0:
return False
return all(count == 0 for count in char_count.values())
# Example usage
print(are_anagrams("debit card", "bad credit")) # Output: True
print(are_anagrams("hello", "world")) # Output: False
This method has a time complexity of O(n), where n is the length of the string. It’s more efficient than the sorting method, especially for longer strings. The space complexity is O(k), where k is the number of unique characters in the string (which is typically constant and small, e.g., 26 for lowercase English letters).
Advanced Technique: Prime Number Product
An interesting and less common approach to solving anagram problems involves using prime numbers. This method assigns a unique prime number to each character and computes the product of these prime numbers for each string. If two strings are anagrams, their products will be identical.
Algorithm:
- Assign a unique prime number to each character (e.g., a=2, b=3, c=5, …)
- Compute the product of prime numbers for each character in the string
- Compare the products of both strings
Python Implementation:
def are_anagrams(str1, str2):
# Convert to lowercase and remove non-alphanumeric characters
str1 = ''.join(e.lower() for e in str1 if e.isalnum())
str2 = ''.join(e.lower() for e in str2 if e.isalnum())
# Check if lengths are different
if len(str1) != len(str2):
return False
# Prime numbers for each lowercase letter
primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101]
# Compute products
product1 = 1
product2 = 1
for char in str1:
product1 *= primes[ord(char) - ord('a')]
for char in str2:
product2 *= primes[ord(char) - ord('a')]
return product1 == product2
# Example usage
print(are_anagrams("astronomer", "moon starer")) # Output: True
print(are_anagrams("hello", "world")) # Output: False
This method has a time complexity of O(n) and a space complexity of O(1). It’s very efficient for short to medium-length strings. However, for very long strings, the product might exceed the maximum integer value supported by the programming language, leading to overflow issues. In such cases, using modular arithmetic or switching to a big integer implementation might be necessary.
Optimizing for Large Datasets: Anagram Grouping
When dealing with large datasets, such as grouping anagrams from a list of thousands of words, efficiency becomes crucial. Here’s an approach that can handle such scenarios effectively:
Algorithm:
- Create a hash map where the key is a sorted version of the word, and the value is a list of anagrams
- Iterate through the list of words
- For each word, sort its characters and use it as a key in the hash map
- Append the original word to the list associated with that key
- Return the values of the hash map, which are the grouped anagrams
Python Implementation:
from collections import defaultdict
def group_anagrams(words):
anagram_groups = defaultdict(list)
for word in words:
# Sort the characters of the word to use as a key
sorted_word = ''.join(sorted(word.lower()))
anagram_groups[sorted_word].append(word)
return list(anagram_groups.values())
# Example usage
word_list = ["eat", "tea", "tan", "ate", "nat", "bat"]
grouped_anagrams = group_anagrams(word_list)
print(grouped_anagrams)
# Output: [['eat', 'tea', 'ate'], ['tan', 'nat'], ['bat']]
This method has a time complexity of O(n * k log k), where n is the number of words and k is the maximum length of a word. The space complexity is O(n * k) to store all the words in the hash map. This approach is particularly useful when you need to group a large number of words by their anagrams efficiently.
Handling Special Cases: Unicode and Multi-byte Characters
When working with anagrams, it’s important to consider special cases such as Unicode characters and multi-byte encodings. Most of the techniques we’ve discussed so far work well with ASCII characters, but may need modifications to handle more complex character sets.
Considerations for Unicode:
- Case sensitivity: Some Unicode characters may have multiple case forms
- Normalization: Different Unicode representations of the same character
- Combining characters: Characters that modify other characters
Python Implementation for Unicode-aware Anagram Check:
import unicodedata
def are_unicode_anagrams(str1, str2):
# Normalize and convert to lowercase
str1 = unicodedata.normalize('NFKD', str1.lower())
str2 = unicodedata.normalize('NFKD', str2.lower())
# Remove non-alphanumeric characters and combining characters
str1 = ''.join(c for c in str1 if not unicodedata.combining(c) and c.isalnum())
str2 = ''.join(c for c in str2 if not unicodedata.combining(c) and c.isalnum())
# Use character count method
if len(str1) != len(str2):
return False
char_count = {}
for char in str1:
char_count[char] = char_count.get(char, 0) + 1
for char in str2:
if char not in char_count:
return False
char_count[char] -= 1
if char_count[char] == 0:
del char_count[char]
return len(char_count) == 0
# Example usage
print(are_unicode_anagrams("café", "face")) # Output: True
print(are_unicode_anagrams("résumé", "summer")) # Output: False
This implementation takes into account Unicode normalization and handles combining characters. It’s more robust for dealing with a wide range of text inputs, including non-English languages and special characters.
Performance Optimization: Bit Manipulation
For scenarios where maximum performance is required and we’re dealing with a known, limited character set (e.g., lowercase English letters), we can use bit manipulation techniques. This approach is extremely fast and memory-efficient.
Algorithm:
- Use an integer to represent the presence of characters (each bit represents a letter)
- For each character in the string, set the corresponding bit
- Compare the final integer values for both strings
Python Implementation:
def are_anagrams_bitwise(str1, str2):
# Convert to lowercase and remove non-alphabetic characters
str1 = ''.join(c.lower() for c in str1 if c.isalpha())
str2 = ''.join(c.lower() for c in str2 if c.isalpha())
# Check if lengths are different
if len(str1) != len(str2):
return False
# Use bitwise operations
bit_vector1 = 0
bit_vector2 = 0
for char in str1:
bit_vector1 |= (1 << (ord(char) - ord('a')))
for char in str2:
bit_vector2 |= (1 << (ord(char) - ord('a')))
return bit_vector1 == bit_vector2
# Example usage
print(are_anagrams_bitwise("listen", "silent")) # Output: True
print(are_anagrams_bitwise("hello", "world")) # Output: False
This method has a time complexity of O(n) and a space complexity of O(1). It’s incredibly fast for short strings composed of lowercase English letters. However, it doesn’t account for character frequency, so it would consider “aab” and “aba” as anagrams, which may or may not be desired depending on the specific problem requirements.
Practical Applications and Interview Strategies
Understanding these techniques for solving anagram problems is crucial for coding interviews, especially when applying to top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Here are some strategies to keep in mind:
- Clarify the Problem: Always start by clarifying the requirements. Ask about case sensitivity, handling of spaces and punctuation, and whether character frequency matters.
- Consider the Constraints: Think about the size of the input. Is it a pair of strings, or are you dealing with a large dataset? This will help you choose the most appropriate algorithm.
- Start Simple: Begin with a straightforward solution like the sorting method. It’s easy to explain and implement, which is valuable in an interview setting.
- Optimize: After implementing a basic solution, discuss potential optimizations. This shows your ability to think critically about efficiency.
- Handle Edge Cases: Don’t forget to consider empty strings, single-character strings, and strings with special characters or Unicode.
- Explain Trade-offs: Discuss the pros and cons of different approaches, demonstrating your understanding of time and space complexity.
Conclusion
Mastering techniques for solving anagram problems is an essential skill for any programmer, particularly those aiming for positions at top tech companies. From basic sorting methods to advanced bit manipulation techniques, each approach has its strengths and ideal use cases. By understanding these various techniques and their trade-offs, you’ll be well-equipped to tackle anagram-related challenges in coding interviews and real-world applications.
Remember, the key to success in algorithmic problem-solving is not just knowing these techniques, but understanding when and how to apply them. Practice implementing these solutions, analyze their performance characteristics, and be prepared to discuss their pros and cons. With this knowledge and practice, you’ll be well-prepared to face anagram problems and similar string manipulation challenges in your coding journey.
Keep coding, keep learning, and embrace the challenge of continually improving your algorithmic thinking skills!