Mastering Algorithm-Based Coding Questions: A Comprehensive Guide
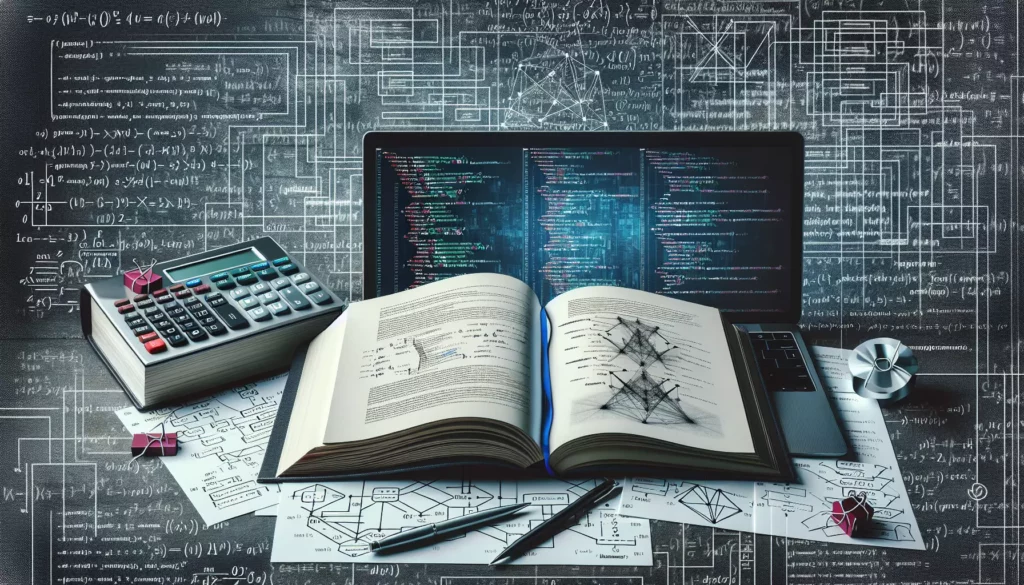
In the world of software development and computer science, algorithm-based coding questions are a crucial aspect of technical interviews, coding competitions, and overall programming proficiency. Whether you’re a beginner looking to improve your problem-solving skills or an experienced developer preparing for a job interview at a major tech company, understanding how to approach these questions is essential. In this comprehensive guide, we’ll explore effective strategies, common pitfalls, and practical tips to help you tackle algorithm-based coding questions with confidence.
Understanding Algorithm-Based Coding Questions
Before diving into the strategies, it’s important to understand what algorithm-based coding questions are and why they’re so prevalent in the tech industry.
What Are Algorithm-Based Coding Questions?
Algorithm-based coding questions are problems that require you to design and implement efficient algorithms to solve specific computational tasks. These questions typically focus on fundamental data structures (like arrays, linked lists, trees, and graphs) and algorithmic techniques (such as sorting, searching, dynamic programming, and graph traversal).
Why Are They Important?
There are several reasons why these questions are crucial:
- Problem-Solving Skills: They test your ability to analyze problems and develop efficient solutions.
- Algorithmic Thinking: They evaluate your understanding of core computer science concepts and data structures.
- Code Quality: They assess your ability to write clean, maintainable, and efficient code.
- Performance Optimization: They challenge you to consider time and space complexity, pushing you to optimize your solutions.
Strategies for Approaching Algorithm-Based Coding Questions
Now that we understand the importance of these questions, let’s explore strategies to tackle them effectively.
1. Read and Understand the Problem
The first step in solving any coding question is to thoroughly read and understand the problem statement. This may seem obvious, but it’s a step that many candidates rush through, leading to misunderstandings and incorrect solutions.
- Read the problem statement multiple times if necessary.
- Identify the input and expected output clearly.
- Look for any constraints or special conditions mentioned in the problem.
- If provided, analyze the sample inputs and outputs to understand the expected behavior.
2. Ask Clarifying Questions
Don’t hesitate to ask questions if anything is unclear. In a real interview setting, this shows that you’re thorough and communicative. Some questions you might ask include:
- What are the input ranges?
- How should edge cases be handled?
- Are there any performance requirements or constraints?
- Can I make any assumptions about the input data?
3. Break Down the Problem
Complex problems can be overwhelming at first glance. Break them down into smaller, manageable sub-problems. This approach, known as “divide and conquer,” can make seemingly difficult problems much more approachable.
- Identify the main components or steps needed to solve the problem.
- Consider solving each sub-problem independently.
- Think about how these sub-solutions can be combined to solve the overall problem.
4. Think About Data Structures
Choosing the right data structure is often key to developing an efficient solution. Consider which data structures might be most appropriate for the problem at hand.
- Arrays or Lists: For sequential data or when you need constant-time access to elements.
- Hash Tables: For quick lookups, counting, or when you need to store key-value pairs.
- Stacks or Queues: For problems involving last-in-first-out (LIFO) or first-in-first-out (FIFO) operations.
- Trees or Graphs: For hierarchical data or problems involving connections between entities.
- Heaps: For problems involving finding the minimum or maximum element frequently.
5. Consider Multiple Approaches
Don’t settle for the first solution that comes to mind. Try to think of multiple approaches to solve the problem. This can help you identify the most efficient solution and demonstrates your problem-solving versatility.
- Brute Force: Start with the simplest, most straightforward solution.
- Optimization: Think about how you can improve the brute force approach.
- Alternative Algorithms: Consider if there are standard algorithms that can be applied to the problem.
6. Analyze Time and Space Complexity
Efficiency is a crucial aspect of algorithm design. Always consider the time and space complexity of your solution.
- Estimate the time complexity using Big O notation.
- Consider the space requirements of your algorithm.
- Think about trade-offs between time and space complexity.
7. Start with Pseudocode
Before diving into coding, it can be helpful to write pseudocode. This allows you to outline your approach without getting bogged down in syntax details.
- Write high-level steps of your algorithm.
- Focus on the logic and flow of your solution.
- Use this as a roadmap for your actual code implementation.
8. Implement Your Solution
Once you have a clear plan, start implementing your solution. Remember to:
- Write clean, readable code.
- Use meaningful variable and function names.
- Break your code into functions or methods for better organization.
- Add comments to explain complex parts of your code.
9. Test Your Code
After implementation, thoroughly test your code. Don’t rely solely on the provided test cases.
- Start with the given sample inputs.
- Test edge cases (empty inputs, single-element inputs, very large inputs).
- Consider corner cases specific to the problem.
- Use a debugger if available to step through your code.
10. Optimize and Refine
If time allows, look for ways to optimize your solution further.
- Can you reduce the time or space complexity?
- Is there any redundant code that can be removed?
- Can you make your code more readable or efficient?
Common Algorithmic Techniques
Familiarizing yourself with common algorithmic techniques can greatly enhance your problem-solving abilities. Here are some key techniques to master:
1. Two Pointers
The two-pointer technique involves using two pointers to traverse a data structure, often moving in tandem or in opposite directions. This technique is particularly useful for problems involving arrays or linked lists.
Example problem: Finding a pair of elements in a sorted array that sum to a target value.
def find_pair_with_sum(arr, target):
left = 0
right = len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return None # No pair found
2. Sliding Window
The sliding window technique is used to perform operations on a specific window of elements in an array or string. It’s particularly useful for problems involving subarrays or substrings.
Example problem: Finding the maximum sum subarray of a fixed size k.
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
3. Binary Search
Binary search is an efficient algorithm for searching a sorted array by repeatedly dividing the search interval in half. It has a time complexity of O(log n).
Example problem: Finding the position of a target value in a sorted array.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
4. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s often used for optimization problems.
Example problem: Computing the nth Fibonacci number.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
5. Depth-First Search (DFS) and Breadth-First Search (BFS)
DFS and BFS are graph traversal algorithms that are fundamental to many graph-related problems.
Example: Implementing DFS for a graph represented as an adjacency list.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
0: [1, 2],
1: [0, 3, 4],
2: [0, 4],
3: [1, 4],
4: [1, 2, 3]
}
dfs(graph, 0)
Common Pitfalls to Avoid
When tackling algorithm-based coding questions, be aware of these common pitfalls:
1. Rushing to Code
One of the biggest mistakes is jumping into coding without fully understanding the problem or planning your approach. Take the time to analyze the problem and outline your solution before writing any code.
2. Ignoring Edge Cases
Always consider edge cases such as empty inputs, single-element inputs, or inputs at the extreme ends of the allowed range. These cases often reveal flaws in your algorithm.
3. Overlooking Time and Space Complexity
Don’t focus solely on correctness. An inefficient solution, even if correct, may not be acceptable. Always consider the time and space complexity of your algorithm and look for ways to optimize.
4. Not Testing Your Code
After implementing your solution, thoroughly test it. Don’t assume it works correctly just because it compiles or runs without errors. Test with various inputs, including edge cases.
5. Overcomplicating the Solution
Sometimes, the simplest solution is the best. Don’t overcomplicate your approach if a straightforward solution exists. Clarity and simplicity are often preferred over overly complex implementations.
6. Poor Code Organization
Write clean, well-organized code. Use meaningful variable names, break your code into functions, and add comments where necessary. This not only makes your code more readable but also helps you catch errors more easily.
7. Not Communicating Your Thought Process
In an interview setting, it’s crucial to communicate your thought process. Explain your approach, discuss trade-offs, and think out loud. This gives the interviewer insight into your problem-solving skills.
Practice and Preparation Tips
Improving your skills in solving algorithm-based coding questions requires consistent practice and preparation. Here are some tips to help you in your journey:
1. Solve Problems Regularly
Consistency is key. Set aside time each day or week to solve coding problems. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of problems to practice with.
2. Study Core Data Structures and Algorithms
Ensure you have a solid understanding of fundamental data structures (arrays, linked lists, trees, graphs, etc.) and algorithms (sorting, searching, dynamic programming, etc.). Review these concepts regularly.
3. Analyze Multiple Solutions
After solving a problem, don’t stop there. Look at other solutions, especially those that are more efficient or use different approaches. This broadens your problem-solving toolkit.
4. Time Yourself
Practice solving problems under time constraints. This helps you get comfortable with the pressure of coding interviews and improves your ability to think and code quickly.
5. Mock Interviews
Participate in mock interviews, either with friends or through platforms that offer this service. This helps you get comfortable with explaining your thought process while coding.
6. Learn from Your Mistakes
Keep track of the problems you struggle with and the mistakes you make. Review these regularly and practice similar problems to strengthen your weak areas.
7. Implement Algorithms from Scratch
Try implementing common algorithms (like binary search, merge sort, or BFS) from scratch. This deepens your understanding of how these algorithms work.
8. Read Code
Reading well-written code can improve your own coding skills. Study open-source projects or solutions to coding problems to learn different coding styles and techniques.
9. Participate in Coding Competitions
Platforms like Codeforces, TopCoder, and Google’s Coding Competitions offer challenging problems and the opportunity to compete with others. This can be a great way to push your skills to the next level.
10. Use Visualization Tools
Tools that visualize algorithms can help you understand how they work. Websites like VisuAlgo offer interactive visualizations of various data structures and algorithms.
Conclusion
Mastering algorithm-based coding questions is a journey that requires patience, practice, and persistence. By following the strategies outlined in this guide, avoiding common pitfalls, and consistently practicing, you can significantly improve your problem-solving skills and confidence in tackling these challenges.
Remember, the goal is not just to solve the problem, but to develop a systematic approach to problem-solving that you can apply to any coding challenge you encounter. Whether you’re preparing for technical interviews, coding competitions, or simply aiming to become a better programmer, the skills you develop in tackling algorithm-based questions will serve you well throughout your career in software development.
Keep learning, stay curious, and embrace the challenge of each new problem as an opportunity to grow. With time and dedication, you’ll find yourself approaching even the most complex algorithm-based coding questions with confidence and skill.