Mastering AI and Machine Learning Interview Questions: A Comprehensive Guide
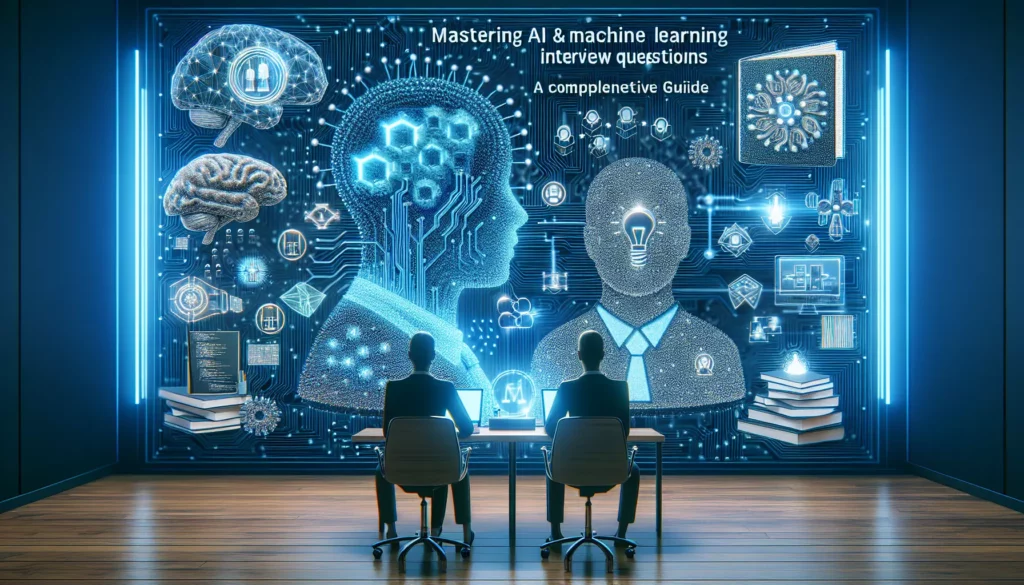
As the fields of Artificial Intelligence (AI) and Machine Learning (ML) continue to evolve and expand, more and more companies are seeking talented professionals with expertise in these areas. If you’re preparing for an AI or ML interview, it’s crucial to be well-versed in the fundamental concepts, algorithms, and practical applications of these technologies. In this comprehensive guide, we’ll explore how to approach AI and machine learning questions in interviews, providing you with valuable insights and strategies to help you succeed.
Understanding the Landscape of AI and ML Interviews
Before diving into specific strategies, it’s essential to understand the types of questions you might encounter in an AI or ML interview. These can typically be categorized into several areas:
- Theoretical concepts and foundations
- Algorithm implementation and optimization
- Data preprocessing and feature engineering
- Model selection and evaluation
- Practical problem-solving scenarios
- Ethical considerations and bias in AI
- Industry trends and recent advancements
With this framework in mind, let’s explore how to approach each of these areas effectively.
1. Mastering Theoretical Concepts and Foundations
A solid understanding of the theoretical foundations of AI and ML is crucial for any aspiring professional in the field. Interviewers often begin by assessing your grasp of fundamental concepts. Here are some key areas to focus on:
Machine Learning Paradigms
Be prepared to discuss and differentiate between the three main types of machine learning:
- Supervised Learning: Explain how algorithms learn from labeled data and provide examples of classification and regression problems.
- Unsupervised Learning: Discuss clustering, dimensionality reduction, and other techniques for finding patterns in unlabeled data.
- Reinforcement Learning: Describe how agents learn to make decisions through interaction with an environment.
Deep Learning and Neural Networks
Demonstrate your knowledge of neural network architectures and deep learning concepts:
- Explain the basic structure of a neural network, including input layers, hidden layers, and output layers.
- Discuss activation functions (e.g., ReLU, sigmoid, tanh) and their purposes.
- Describe different types of neural networks, such as Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs).
Statistical Foundations
Show your understanding of the statistical principles underlying machine learning:
- Probability theory and Bayes’ theorem
- Descriptive and inferential statistics
- Hypothesis testing and confidence intervals
2. Algorithm Implementation and Optimization
Interviewers often assess your ability to implement and optimize machine learning algorithms. Be prepared to discuss and potentially code the following:
Classic Machine Learning Algorithms
- Linear Regression and Logistic Regression
- Decision Trees and Random Forests
- Support Vector Machines (SVM)
- K-Means Clustering
- Principal Component Analysis (PCA)
Optimization Techniques
Demonstrate your knowledge of various optimization algorithms used in machine learning:
- Gradient Descent and its variants (e.g., Stochastic Gradient Descent, Mini-batch Gradient Descent)
- Adam, RMSprop, and other adaptive learning rate methods
- Regularization techniques (L1, L2, Elastic Net)
Coding Example: Implementing Gradient Descent
Here’s a simple example of implementing gradient descent for linear regression in Python:
import numpy as np
def gradient_descent(X, y, learning_rate=0.01, num_iterations=1000):
m, n = X.shape
theta = np.zeros(n)
for _ in range(num_iterations):
h = np.dot(X, theta)
gradient = (1/m) * np.dot(X.T, (h - y))
theta -= learning_rate * gradient
return theta
# Example usage
X = np.array([[1, 1], [1, 2], [1, 3]])
y = np.array([1, 2, 3])
theta = gradient_descent(X, y)
print("Optimized theta:", theta)
Be prepared to explain the code, discuss its time and space complexity, and suggest potential optimizations.
3. Data Preprocessing and Feature Engineering
Effective data preprocessing and feature engineering are crucial steps in any machine learning pipeline. Interviewers may ask you about various techniques and their importance:
Data Cleaning and Handling Missing Values
- Discuss methods for identifying and handling outliers
- Explain different approaches to dealing with missing data (e.g., imputation, deletion)
- Describe techniques for handling imbalanced datasets
Feature Scaling and Normalization
Be prepared to explain and implement various scaling techniques:
- Min-Max Scaling
- Standardization (Z-score normalization)
- Robust Scaling
Feature Selection and Dimensionality Reduction
Demonstrate your understanding of methods to select relevant features and reduce dimensionality:
- Filter methods (e.g., correlation-based feature selection)
- Wrapper methods (e.g., Recursive Feature Elimination)
- Embedded methods (e.g., LASSO regularization)
- Principal Component Analysis (PCA) for dimensionality reduction
Coding Example: Feature Scaling
Here’s an example of implementing Min-Max scaling in Python:
import numpy as np
def min_max_scale(X):
min_vals = np.min(X, axis=0)
max_vals = np.max(X, axis=0)
scaled_X = (X - min_vals) / (max_vals - min_vals)
return scaled_X
# Example usage
X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
scaled_X = min_max_scale(X)
print("Scaled features:")
print(scaled_X)
Be ready to discuss the advantages and potential drawbacks of different scaling methods.
4. Model Selection and Evaluation
Choosing the right model and effectively evaluating its performance are critical skills for any AI or ML professional. Be prepared to discuss the following topics:
Model Selection Techniques
- Cross-validation methods (k-fold, stratified k-fold, leave-one-out)
- Hyperparameter tuning (grid search, random search, Bayesian optimization)
- Ensemble methods (bagging, boosting, stacking)
Evaluation Metrics
Demonstrate your understanding of various performance metrics and when to use them:
- Classification metrics: Accuracy, Precision, Recall, F1-score, ROC-AUC
- Regression metrics: Mean Squared Error (MSE), Root Mean Squared Error (RMSE), Mean Absolute Error (MAE), R-squared
- Clustering metrics: Silhouette score, Calinski-Harabasz index
Overfitting and Underfitting
Be prepared to discuss strategies for identifying and addressing overfitting and underfitting:
- Regularization techniques (L1, L2, Elastic Net)
- Early stopping in neural networks
- Dropout and other regularization methods for deep learning
Coding Example: K-Fold Cross-Validation
Here’s an example of implementing k-fold cross-validation in Python:
from sklearn.model_selection import KFold
from sklearn.metrics import mean_squared_error
import numpy as np
def k_fold_cross_validation(X, y, model, k=5):
kf = KFold(n_splits=k, shuffle=True, random_state=42)
mse_scores = []
for train_index, test_index in kf.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
mse_scores.append(mse)
return np.mean(mse_scores), np.std(mse_scores)
# Example usage (assuming you have a model and data)
# mean_mse, std_mse = k_fold_cross_validation(X, y, model)
# print(f"Mean MSE: {mean_mse:.4f} (+/- {std_mse:.4f})")
Be prepared to explain the benefits of cross-validation and how it helps in assessing model performance.
5. Practical Problem-Solving Scenarios
Interviewers often present real-world scenarios to assess your ability to apply AI and ML concepts to solve practical problems. Here are some tips for approaching these questions:
Problem Framing
- Clearly define the problem and its objectives
- Identify the type of machine learning task (classification, regression, clustering, etc.)
- Discuss potential constraints and limitations
Data Considerations
- Describe the type and amount of data needed
- Discuss potential data collection methods
- Address data quality and preprocessing requirements
Model Selection and Development
- Propose suitable algorithms or models for the problem
- Discuss feature engineering and selection strategies
- Outline the training and evaluation process
Deployment and Monitoring
- Describe how you would deploy the model in a production environment
- Discuss strategies for monitoring model performance over time
- Address potential challenges in scaling the solution
Example Scenario: Customer Churn Prediction
Suppose you’re asked to develop a machine learning solution to predict customer churn for a subscription-based service. Here’s how you might approach this problem:
- Problem Framing: Define churn prediction as a binary classification problem, where the goal is to predict whether a customer will cancel their subscription within a specific timeframe.
- Data Considerations: Discuss the need for historical customer data, including demographics, usage patterns, customer support interactions, and past churn events. Address potential data privacy concerns and the need for anonymization.
- Feature Engineering: Propose relevant features such as customer tenure, frequency of product usage, recent changes in usage patterns, and customer satisfaction scores. Discuss the importance of creating time-based features to capture trends.
- Model Selection: Suggest using ensemble methods like Random Forest or Gradient Boosting Machines due to their ability to handle complex relationships and provide feature importance. Discuss the need for handling class imbalance, as churn events are typically rare.
- Evaluation: Propose using metrics such as ROC-AUC and precision-recall curves, given the likely class imbalance. Discuss the importance of choosing an appropriate threshold based on the business’s tolerance for false positives vs. false negatives.
- Deployment: Describe how you would integrate the model into the company’s existing systems, potentially using a microservices architecture for scalability. Discuss the need for real-time or batch predictions based on the use case.
- Monitoring: Propose a system for monitoring model performance over time, including tracking of key metrics and implementing automated retraining when performance degrades.
By systematically addressing each aspect of the problem, you demonstrate your ability to apply AI and ML concepts to real-world scenarios.
6. Ethical Considerations and Bias in AI
As AI and ML systems become more prevalent in society, it’s crucial to address ethical considerations and potential biases. Be prepared to discuss the following topics:
Types of Bias in AI
- Data bias: Discuss how biased training data can lead to biased model outputs
- Algorithmic bias: Explain how certain algorithms may inadvertently discriminate against protected groups
- Interaction bias: Describe how the way users interact with AI systems can reinforce existing biases
Fairness in Machine Learning
Be prepared to discuss various definitions of fairness and their trade-offs:
- Demographic parity
- Equal opportunity
- Equalized odds
Strategies for Mitigating Bias
- Diverse and representative training data
- Careful feature selection and engineering
- Regular audits of model performance across different demographic groups
- Implementing fairness constraints in model training
Transparency and Explainability
Discuss the importance of model interpretability and techniques for achieving it:
- LIME (Local Interpretable Model-agnostic Explanations)
- SHAP (SHapley Additive exPlanations) values
- Feature importance analysis
Example Discussion: Addressing Bias in a Hiring Algorithm
Suppose you’re asked how you would address potential bias in a machine learning model used for resume screening in a hiring process. Here’s how you might approach this discussion:
- Identify Potential Sources of Bias: Discuss how historical hiring data may reflect past discriminatory practices, potentially leading to biased predictions.
- Data Preprocessing: Propose techniques for removing or obfuscating sensitive attributes (e.g., name, gender, age) from the training data to reduce direct discrimination.
- Feature Engineering: Suggest focusing on job-relevant features and skills rather than demographic information or proxies for protected attributes.
- Model Selection: Recommend using interpretable models (e.g., decision trees) or techniques that allow for easy auditing of decision-making processes.
- Fairness Constraints: Discuss implementing fairness constraints during model training to ensure equal selection rates across different demographic groups.
- Regular Audits: Propose a system for regularly auditing the model’s performance across different demographic groups to identify and address any emerging biases.
- Human Oversight: Emphasize the importance of human review in the hiring process and using the ML model as a decision support tool rather than an autonomous decision-maker.
- Transparency: Suggest providing explanations for the model’s recommendations to both hiring managers and candidates to increase transparency and trust in the process.
By addressing these ethical considerations, you demonstrate awareness of the broader implications of AI and ML systems in society.
7. Industry Trends and Recent Advancements
Staying up-to-date with the latest developments in AI and ML is crucial for any professional in the field. Be prepared to discuss recent trends and advancements, such as:
Transformer Models and Natural Language Processing
- Discuss the impact of models like BERT, GPT-3, and their variants on NLP tasks
- Explain the concept of transfer learning in the context of large language models
- Address the challenges and opportunities presented by few-shot and zero-shot learning
AutoML and Neural Architecture Search
- Describe how AutoML tools are changing the landscape of model development
- Discuss the potential benefits and limitations of automated feature engineering and model selection
- Explain the concept of Neural Architecture Search and its applications
Federated Learning and Privacy-Preserving AI
- Explain the principles of federated learning and its applications in privacy-sensitive domains
- Discuss other privacy-preserving techniques such as differential privacy and homomorphic encryption
- Address the trade-offs between model performance and privacy preservation
AI in Edge Computing
- Discuss the challenges and opportunities of deploying AI models on edge devices
- Explain techniques for model compression and quantization
- Address the potential impact of edge AI on various industries (e.g., IoT, autonomous vehicles)
Example Discussion: The Impact of Large Language Models
If asked about the impact of large language models like GPT-3 on the AI landscape, you might structure your response as follows:
- Advancements in Natural Language Understanding: Discuss how these models have significantly improved performance on a wide range of NLP tasks, from text generation to question-answering and summarization.
- Few-shot and Zero-shot Learning: Explain how these models can perform tasks with minimal or no task-specific training data, potentially reducing the need for large labeled datasets in some applications.
- Transfer Learning: Discuss how pre-trained language models can be fine-tuned for specific tasks, significantly reducing the time and resources required for model development.
- Ethical Considerations: Address concerns about bias in large language models and the potential for misuse in generating misleading or harmful content.
- Computational Requirements: Discuss the significant computational resources required to train and deploy these models, and the associated environmental impact.
- Future Directions: Speculate on potential future developments, such as multimodal models that combine language understanding with vision or other sensory inputs.
By demonstrating your awareness of current trends and their implications, you show that you’re engaged with the field and capable of thinking critically about its future directions.
Conclusion: Putting It All Together
Successfully navigating AI and machine learning interview questions requires a combination of technical knowledge, problem-solving skills, and the ability to communicate complex ideas clearly. As you prepare for your interviews, keep these key points in mind:
- Build a Strong Foundation: Ensure you have a solid understanding of the fundamental concepts and algorithms in AI and ML.
- Practice Implementation: Be prepared to write code and implement algorithms, focusing on efficiency and best practices.
- Develop a Problem-Solving Framework: Create a systematic approach to tackling real-world ML problems, from problem framing to model deployment.
- Stay Current: Keep up with the latest trends and advancements in the field, and be prepared to discuss their implications.
- Consider the Bigger Picture: Be ready to address ethical considerations and the broader impact of AI on society.
- Communicate Clearly: Practice explaining complex concepts in simple terms, using analogies and examples where appropriate.
- Engage in Continuous Learning: The field of AI and ML is rapidly evolving, so commit to ongoing learning and skill development.
Remember, interviews are not just about showcasing your knowledge but also demonstrating your passion for the field and your ability to contribute to a team. By thoroughly preparing and approaching interviews with confidence and enthusiasm, you’ll be well-positioned to succeed in your AI and ML career journey.
As you continue to develop your skills and prepare for interviews, consider leveraging resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you master the algorithms and problem-solving techniques essential for success in the field of AI and machine learning. With dedication, practice, and a strategic approach to interview preparation, you’ll be well-equipped to tackle even the most challenging AI and ML interview questions and launch a successful career in this exciting and rapidly evolving field.