Managing Pressure in Programming: Strategies for Success Under Stress
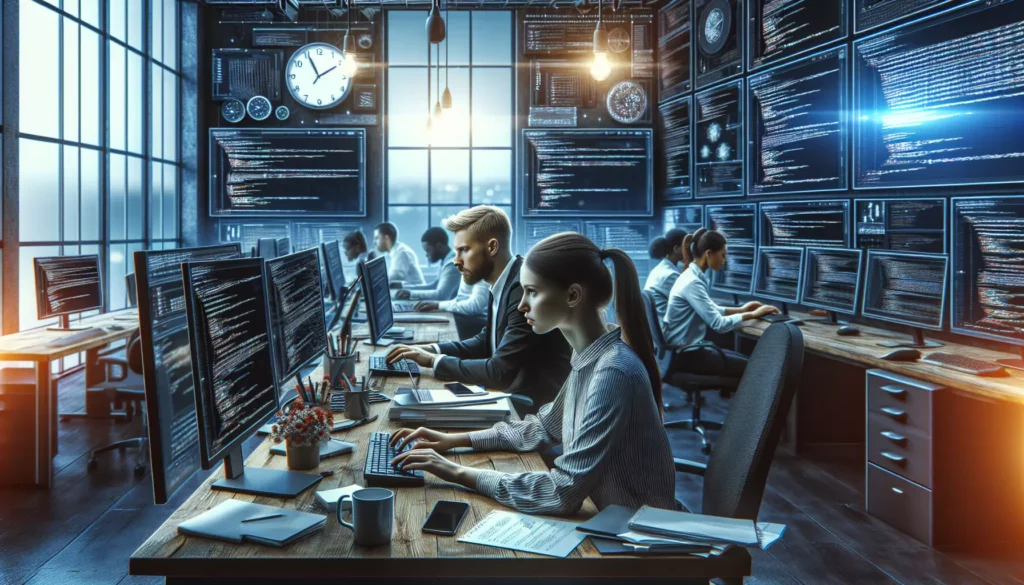
Programming is often depicted as a career of innovation and creativity, but the reality can be quite different. Behind the sleek interfaces and elegant code lies a world of tight deadlines, complex problems, and high expectations. For many developers, pressure is a constant companion throughout their careers.
The ability to manage pressure effectively isn’t just a nice to have skill; it’s essential for long term success and wellbeing in the programming field. This article explores the unique pressures programmers face and provides practical strategies to not only survive but thrive under pressure.
Understanding the Pressure Points in Programming
Before addressing solutions, it’s important to recognize the common sources of pressure in programming:
Tight Deadlines
Perhaps the most universal pressure point for programmers is the deadline. Whether it’s shipping a new feature, fixing a critical bug, or completing a client project, time constraints create significant stress. The challenge intensifies when deadlines are unrealistic or constantly shifting.
Complex Problem Solving
Programming inherently involves solving complex problems. When faced with particularly challenging issues or bugs that resist resolution, the mental strain can be immense. This cognitive load is often invisible to non technical stakeholders who may not understand why something “simple” is taking so long.
Evolving Technologies
The rapid pace of technological change means programmers must constantly learn new languages, frameworks, and tools. This creates pressure to stay relevant while simultaneously delivering on current responsibilities.
High Stakes Environments
In many contexts, programming errors can have serious consequences. Financial systems, healthcare applications, or security critical software carry additional pressure due to the potential impact of mistakes.
Unclear Requirements
Working with vague, changing, or contradictory requirements is a common frustration. This ambiguity creates pressure as programmers attempt to deliver solutions without a clear target.
The Impact of Unmanaged Pressure
When not properly managed, pressure can lead to several negative outcomes:
Burnout
Chronic, unrelenting pressure is a primary contributor to burnout, characterized by emotional exhaustion, cynicism, and reduced professional efficacy. In programming, burnout can manifest as an inability to focus, decreased problem solving abilities, and loss of passion for the work.
Decreased Code Quality
Under extreme pressure, programmers may take shortcuts, skip testing, or accumulate technical debt. These compromises might meet immediate deadlines but create larger problems down the road.
Impaired Decision Making
Pressure affects cognitive function, particularly the ability to make complex decisions. In programming, where decisions have long lasting implications, this can be particularly problematic.
Increased Error Rates
Studies consistently show that pressure increases error rates. For programmers, this means more bugs, security vulnerabilities, and system failures.
Practical Strategies for Managing Pressure
Now that we understand the challenges, let’s explore effective strategies for managing pressure in programming contexts:
Technical Approaches
Break Down Complex Problems
When facing overwhelming complexity, break problems into smaller, manageable components. This divide and conquer approach makes progress visible and reduces cognitive load.
// Instead of tackling an entire feature at once
// Break it down into smaller functions
// Example: User authentication system
function validateUserCredentials() {
// Focus just on credential validation
}
function generateAuthToken() {
// Handle token generation separately
}
function setupUserSession() {
// Manage session initialization
}
Implement Test Driven Development
TDD provides a structured approach to development that can reduce pressure by creating clear, incremental goals. Writing tests first helps define success criteria and provides confidence in your solutions.
// Example of Test Driven Development
// 1. Write a failing test
test('should calculate correct total with tax', () => {
const calculator = new PriceCalculator();
expect(calculator.calculateTotal(100, 0.1)).toBe(110);
});
// 2. Implement the minimal code to pass
class PriceCalculator {
calculateTotal(price, taxRate) {
return price + (price * taxRate);
}
}
// 3. Refactor while keeping tests passing
Leverage Automation
Identify repetitive tasks that can be automated. This might include testing, deployment, code formatting, or documentation generation. Automation reduces cognitive load and frees mental resources for more complex challenges.
Maintain a Knowledge Base
Create personal documentation for solutions to problems you’ve solved. This reduces pressure when similar issues arise in the future and serves as a reference during high stress periods.
Planning and Time Management
Realistic Estimation
Improving estimation skills is crucial for managing pressure. When providing time estimates:
- Consider your historical performance on similar tasks
- Account for unknown factors by adding buffer time
- Break down tasks to estimate more accurately
- Learn to communicate constraints and uncertainties
Prioritization Techniques
Not all tasks are created equal. Use techniques like the Eisenhower Matrix (urgent/important classification) or MoSCoW method (Must have, Should have, Could have, Won’t have) to identify what truly needs your attention first.
Time Boxing
Allocate fixed time periods for specific tasks. This creates boundaries and prevents perfectionism from consuming unlimited time. The Pomodoro Technique (25 minutes of focused work followed by a 5 minute break) is particularly effective for programming tasks.
Strategic Procrastination
Sometimes stepping away from a problem is the most productive approach. This “incubation period” allows your subconscious to work on the problem while you focus elsewhere. Many programmers report breakthroughs after temporarily abandoning difficult problems.
Communication Strategies
Setting Boundaries
Clear communication about capacity and limitations is essential. Learning to say “no” or “not now” to additional work when already at capacity is a critical skill for sustainable performance.
Expectation Management
Proactively communicate progress, challenges, and revised timelines. When stakeholders understand the reality of the situation, pressure often decreases. Regular updates prevent last minute surprises and build trust.
Asking for Help
Many programmers struggle with this, but asking for help is a sign of strength, not weakness. Whether it’s technical assistance, additional resources, or deadline adjustments, clear requests can significantly reduce pressure.
Psychological Approaches
Reframing Pressure
Research shows that how we interpret pressure affects our response to it. Try viewing pressure as a challenge rather than a threat. This “challenge mindset” improves performance under pressure compared to viewing the same situation as threatening.
Mindfulness Practices
Techniques like meditation, deep breathing, or even simple awareness exercises can reduce the physiological stress response. Even brief mindfulness practices (5 minutes) can improve focus and reduce reactivity to pressure.
// A "mindful debugging" approach
// When stuck on a difficult bug:
1. Take three deep breaths
2. Notice any physical tension and consciously relax
3. Observe thoughts without judgment ("I notice I'm thinking this is impossible")
4. Return focus to the specific problem, not catastrophizing about consequences
5. Approach the problem with curiosity rather than frustration
Maintaining Perspective
In the heat of a programming crisis, perspective can be lost. Regularly step back and ask: “How important will this seem in a week, a month, or a year?” This temporal distancing reduces immediate pressure and improves decision making.
Environmental and Physical Factors
Workspace Optimization
Your physical environment significantly impacts your ability to handle pressure. Consider:
- Noise management (noise cancelling headphones or ambient sound)
- Ergonomic setup to reduce physical strain
- Lighting that reduces eye fatigue
- Organization systems that reduce cognitive load
Physical Well Being
The mind body connection is powerful. Basic physical maintenance dramatically improves pressure tolerance:
- Adequate sleep (sleep deprivation severely impairs problem solving)
- Regular movement (even short walks improve cognitive function)
- Hydration and nutrition (the brain is particularly sensitive to these factors)
- Limiting stimulants when already under pressure
Team and Organizational Approaches
Creating Supportive Team Cultures
Individual strategies are more effective within supportive teams. Consider advocating for:
- Blameless postmortems that focus on learning rather than fault finding
- Pair programming during high pressure periods
- Code review processes that distribute knowledge and responsibility
- Psychological safety that allows honest communication about capacity
Organizational Practices
Some pressure sources require organizational solutions:
- Realistic project planning that includes developer input
- Buffer time for unexpected challenges
- Clear prioritization from leadership
- Recognition that sustainable pace outperforms constant crunch time
Learning from High Pressure Experiences
Every high pressure situation contains valuable lessons. After navigating a particularly stressful period:
Conduct Personal Retrospectives
Ask yourself:
- What created the pressure in this situation?
- Which strategies helped me manage effectively?
- What would I do differently next time?
- Were there early warning signs I missed?
Document Lessons Learned
Create personal playbooks for handling similar situations in the future. These might include technical approaches, communication templates, or self care reminders.
Build Pressure Tolerance
Like other skills, handling pressure improves with practice. Deliberately seeking moderate challenges expands your capacity to perform under pressure over time.
When Pressure Becomes Too Much
Sometimes pressure exceeds what can be reasonably managed with the strategies above. Recognize the signs that additional intervention may be needed:
- Persistent sleep disturbances
- Inability to disconnect from work thoughts
- Physical symptoms like headaches or digestive issues
- Loss of interest in activities previously enjoyed
- Increased irritability or emotional reactivity
In these cases, consider:
- Taking time off to genuinely disconnect
- Seeking support from mental health professionals
- Having direct conversations about workload adjustments
- Exploring whether your current role or organization is a good fit
Conclusion
Pressure is an inevitable part of programming careers, but it doesn’t have to lead to burnout or diminished performance. By understanding the unique pressures of the field and implementing targeted strategies, programmers can not only survive high pressure situations but use them as opportunities for growth and mastery.
The most successful programmers aren’t those who never feel pressure, but those who have developed systems to manage it effectively. By incorporating technical approaches, planning strategies, communication skills, and psychological techniques, you can build your capacity to perform well even when the stakes are high.
Remember that pressure management is highly individual. Experiment with different approaches to discover what works best for your unique circumstances and working style. With practice and intention, you can transform pressure from an obstacle to an opportunity for your best work.