Manacher’s Algorithm: Mastering the Art of Finding the Longest Palindromic Substring
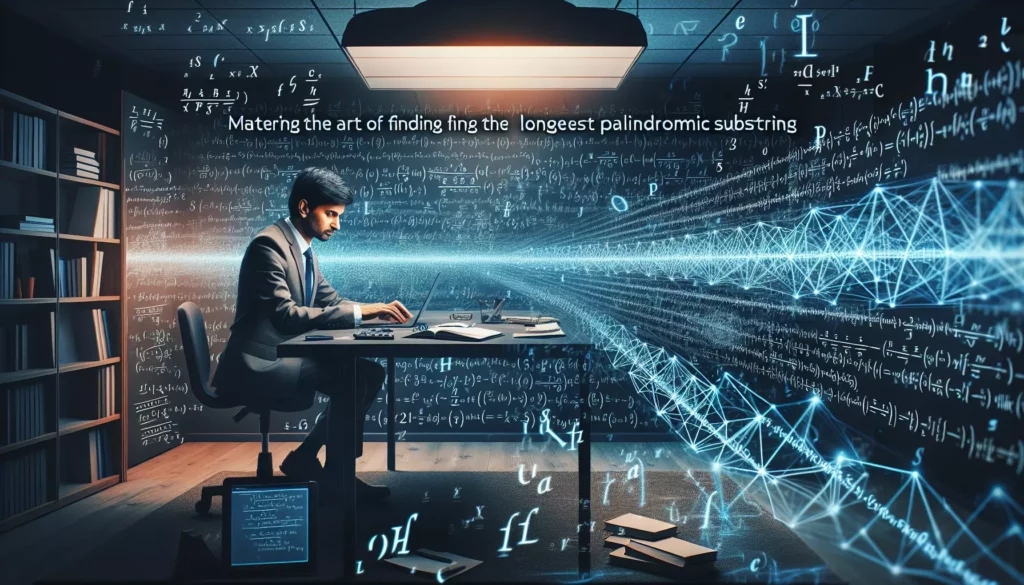
In the vast realm of algorithmic problem-solving, few challenges are as intriguing and practical as finding the longest palindromic substring within a given string. This problem has applications ranging from computational biology to natural language processing, and mastering its solution is a testament to one’s programming prowess. Enter Manacher’s Algorithm – an elegant and efficient approach that revolutionizes how we tackle this classic problem.
In this comprehensive guide, we’ll dive deep into Manacher’s Algorithm, unraveling its intricacies and demonstrating why it’s a game-changer in the world of string manipulation. Whether you’re a coding enthusiast looking to expand your algorithmic toolkit or a seasoned developer preparing for technical interviews at top tech companies, understanding Manacher’s Algorithm is sure to elevate your problem-solving skills.
Understanding Palindromes and the Problem at Hand
Before we delve into the algorithm itself, let’s refresh our understanding of palindromes and the problem we’re trying to solve.
What is a Palindrome?
A palindrome is a sequence of characters that reads the same forward and backward. For example:
- “racecar” is a palindrome
- “level” is a palindrome
- “A man, a plan, a canal: Panama” is a palindrome (ignoring spaces and punctuation)
The Longest Palindromic Substring Problem
Given a string, the task is to find the longest substring within it that is a palindrome. For instance:
- In the string “babad”, the longest palindromic substring is “bab” or “aba” (both are valid answers)
- In “cbbd”, the longest palindromic substring is “bb”
This problem has numerous applications, including:
- DNA sequence analysis in bioinformatics
- Text processing and pattern matching
- Data compression algorithms
- Cryptography and secure communication
The Naive Approach: Brute Force
Before we introduce Manacher’s Algorithm, let’s consider the straightforward brute force approach to solving this problem. This method involves checking every possible substring to determine if it’s a palindrome and keeping track of the longest one found.
Here’s a simple implementation in Python:
def longest_palindrome_brute_force(s):
n = len(s)
longest = ""
for i in range(n):
for j in range(i, n):
substring = s[i:j+1]
if substring == substring[::-1] and len(substring) > len(longest):
longest = substring
return longest
# Example usage
print(longest_palindrome_brute_force("babad")) # Output: "bab" or "aba"
While this approach works, it has a time complexity of O(n³), where n is the length of the string. This makes it impractical for longer strings or in scenarios where performance is crucial.
Enter Manacher’s Algorithm
Manacher’s Algorithm, introduced by Glenn K. Manacher in 1975, provides a linear-time solution to the longest palindromic substring problem. It’s an ingenious approach that leverages the symmetry of palindromes to drastically reduce the number of comparisons needed.
Key Concepts of Manacher’s Algorithm
- Preprocessing the String: The algorithm first transforms the input string by inserting special characters (usually ‘#’) between each character and at the beginning and end. This ensures that all palindromes have odd length, simplifying the algorithm.
- Center Expansion: It expands around each character as a potential center of a palindrome.
- Utilizing Previous Results: The algorithm cleverly uses information from previously computed palindromes to avoid unnecessary comparisons.
- Maintaining a Center and Right Boundary: It keeps track of the center and right boundary of the currently largest palindrome encountered.
The Algorithm Step by Step
Let’s break down Manacher’s Algorithm into detailed steps:
- Preprocess the String:
- Insert ‘#’ between each character and at the start and end of the string.
- For example, “babad” becomes “#b#a#b#a#d#”.
- Initialize Variables:
- Create an array P to store the length of palindromes centered at each position.
- Initialize center C and right boundary R of the current palindrome.
- Iterate Through the String:
- For each position i in the transformed string:
- If i < R, set P[i] to the minimum of P[2*C – i] (mirror of i) and R – i.
- Expand around i while the characters match.
- Update C and R if a larger palindrome is found.
- Find the Longest Palindrome:
- Identify the maximum value in P and its corresponding index.
- Extract the original substring using this information.
Implementation in Python
Let’s implement Manacher’s Algorithm in Python:
def manacher_algorithm(s):
# Preprocess the string
t = "#" + "#".join(s) + "#"
n = len(t)
P = [0] * n
C = R = 0
for i in range(1, n-1):
mirror = 2*C - i
if i < R:
P[i] = min(R - i, P[mirror])
# Attempt to expand palindrome centered at i
while i + (1 + P[i]) < n and i - (1 + P[i]) >= 0 and t[i + (1 + P[i])] == t[i - (1 + P[i])]:
P[i] += 1
# If palindrome centered at i expands past R,
# adjust center based on expanded palindrome.
if i + P[i] > R:
C, R = i, i + P[i]
# Find the maximum element in P
max_len, center_index = max((n, i) for i, n in enumerate(P))
start = (center_index - max_len) // 2
return s[start : start + max_len]
# Example usage
print(manacher_algorithm("babad")) # Output: "bab" or "aba"
Time and Space Complexity Analysis
One of the most impressive aspects of Manacher’s Algorithm is its efficiency:
- Time Complexity: O(n), where n is the length of the input string. This linear time complexity is achieved because each character is expanded at most once.
- Space Complexity: O(n), as we need to store the transformed string and the P array.
This is a significant improvement over the brute force approach, especially for large strings.
Practical Applications and Variations
Manacher’s Algorithm isn’t just a theoretical concept; it has practical applications in various fields:
1. Bioinformatics
In DNA sequence analysis, palindromic sequences often indicate important biological structures. Manacher’s Algorithm can efficiently identify these sequences in large genomic datasets.
2. Text Processing
In natural language processing, identifying palindromes can be useful for tasks like text compression or stylistic analysis of literary works.
3. Data Compression
Some compression algorithms use palindrome detection as part of their strategy to reduce data size.
4. Cryptography
Palindromes play a role in certain cryptographic algorithms and can be used in code breaking techniques.
Variations and Extensions
The basic Manacher’s Algorithm can be extended or modified for various related problems:
- Counting All Palindromic Substrings: With slight modifications, the algorithm can count all palindromic substrings in linear time.
- Finding the Shortest Palindrome: By applying Manacher’s Algorithm in reverse, we can find the shortest string that needs to be prepended to make the entire string a palindrome.
- Palindromic Factorization: The algorithm can be adapted to find the minimum number of cuts needed to divide a string into palindromes.
Common Pitfalls and Optimization Tips
While Manacher’s Algorithm is powerful, there are some common pitfalls to avoid and optimizations to consider:
Pitfalls:
- Off-by-One Errors: Be careful with index calculations, especially when converting between the transformed and original string.
- Overflow in Large Strings: For very long strings, be mindful of potential integer overflow in languages with fixed-size integers.
- Handling Empty Strings: Ensure your implementation correctly handles edge cases like empty strings or single-character strings.
Optimization Tips:
- Memory Efficiency: In memory-constrained environments, you can avoid storing the transformed string explicitly by using clever indexing.
- Early Termination: If you’re only interested in palindromes above a certain length, you can add early termination conditions.
- Parallel Processing: For extremely large strings, consider parallelizing the algorithm by dividing the string into segments.
Comparing Manacher’s Algorithm with Other Approaches
To fully appreciate Manacher’s Algorithm, it’s worth comparing it with other approaches to the longest palindromic substring problem:
1. Dynamic Programming Approach
A common alternative is using dynamic programming:
def longest_palindrome_dp(s):
n = len(s)
dp = [[False] * n for _ in range(n)]
start, max_length = 0, 1
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for length in range(3, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
if length > max_length:
start = i
max_length = length
return s[start:start + max_length]
# Example usage
print(longest_palindrome_dp("babad")) # Output: "bab" or "aba"
This approach has a time complexity of O(n²) and space complexity of O(n²), making it less efficient than Manacher’s Algorithm for large inputs.
2. Expand Around Center Approach
Another approach is to expand around each possible center:
def expand_around_center(s, left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
def longest_palindrome_expand(s):
if not s:
return ""
start, end = 0, 0
for i in range(len(s)):
len1 = expand_around_center(s, i, i)
len2 = expand_around_center(s, i, i + 1)
max_len = max(len1, len2)
if max_len > end - start:
start = i - (max_len - 1) // 2
end = i + max_len // 2
return s[start:end+1]
# Example usage
print(longest_palindrome_expand("babad")) # Output: "bab" or "aba"
This approach has a time complexity of O(n²) in the worst case but can be faster in practice for many inputs. However, it’s still not as efficient as Manacher’s Algorithm for large or worst-case scenarios.
Preparing for Technical Interviews
Understanding Manacher’s Algorithm can be a significant asset in technical interviews, especially for positions at top tech companies. Here are some tips for leveraging this knowledge:
- Understand the Core Concept: Be able to explain the key idea behind Manacher’s Algorithm – how it uses symmetry to optimize palindrome detection.
- Implement from Scratch: Practice implementing the algorithm without referring to notes. This demonstrates a deep understanding of the concept.
- Analyze Time and Space Complexity: Be prepared to discuss why the algorithm achieves linear time complexity and how it compares to other approaches.
- Consider Edge Cases: Think about how the algorithm handles edge cases like empty strings, single-character strings, or strings with no palindromes longer than one character.
- Discuss Applications: Be ready to talk about real-world applications of the algorithm and how it might be used in practical scenarios.
- Optimize and Extend: Consider how you might optimize the algorithm for specific constraints or extend it to solve related problems.
Conclusion
Manacher’s Algorithm stands as a testament to the power of clever algorithmic thinking. By transforming a seemingly quadratic-time problem into a linear-time solution, it showcases the importance of understanding string properties and symmetry in algorithm design.
As you continue your journey in algorithmic problem-solving and prepare for technical interviews, remember that mastering algorithms like Manacher’s is not just about memorizing steps. It’s about understanding the underlying principles, recognizing patterns, and developing the ability to apply these concepts to new and varied problems.
Whether you’re aiming for a position at a top tech company or simply looking to enhance your coding skills, the insights gained from studying Manacher’s Algorithm will serve you well. It’s a powerful addition to your algorithmic toolkit, demonstrating the beauty and efficiency that can be achieved in computer science when approaching problems with creativity and mathematical insight.
Keep practicing, exploring variations, and most importantly, enjoy the process of unraveling these algorithmic puzzles. The skills you develop here will not only prepare you for interviews but will also make you a more effective and insightful programmer in your future endeavors.