Making the Most of Coding Interview Hints: How to Use Hints Without Relying on Them
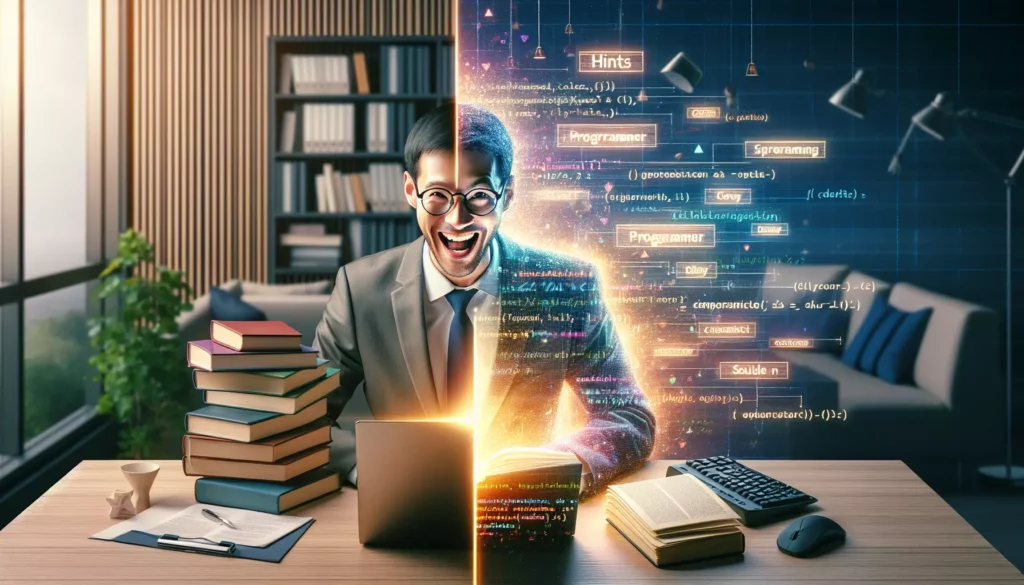
Coding interviews can be nerve-wracking experiences, especially when you’re faced with a challenging problem and the clock is ticking. In these situations, interviewers may offer hints to help guide you towards a solution. While these hints can be valuable, it’s crucial to use them effectively without becoming overly dependent on them. In this comprehensive guide, we’ll explore strategies for making the most of coding interview hints while still showcasing your problem-solving skills.
Understanding the Role of Hints in Coding Interviews
Before we dive into strategies for using hints effectively, it’s important to understand why interviewers provide hints in the first place:
- To assess your ability to take direction: Interviewers want to see how well you can incorporate new information and adjust your approach.
- To keep the interview moving: If you’re stuck, a hint can help prevent the interview from stalling.
- To evaluate your communication skills: How you respond to and discuss hints can reveal your ability to collaborate and think out loud.
- To test your problem-solving process: Hints allow interviewers to observe how you integrate new information into your existing thought process.
Strategies for Effectively Using Coding Interview Hints
1. Acknowledge the Hint
When an interviewer offers a hint, it’s crucial to acknowledge it explicitly. This demonstrates that you’re actively listening and engaged in the problem-solving process. Here’s how to do it effectively:
- Verbalize your understanding: Repeat the hint in your own words to ensure you’ve grasped it correctly.
- Thank the interviewer: A simple “Thank you for the hint” shows appreciation and professionalism.
- Relate it to your current approach: Briefly explain how the hint might fit into or change your current thinking.
Example response:
"Thank you for that hint. If I understand correctly, you're suggesting that I consider using a hash map to optimize the lookup process. That's an interesting approach that I hadn't considered. Let me think about how that might change my current strategy."
2. Incorporate the Hint Thoughtfully
Once you’ve acknowledged the hint, take a moment to consider how it fits into your problem-solving approach. Here are some tips for incorporating hints effectively:
- Don’t immediately abandon your current approach: Consider if the hint can be integrated into your existing solution.
- Think out loud: Verbalize your thought process as you consider how to use the hint.
- Ask clarifying questions: If you’re unsure about how to apply the hint, don’t hesitate to ask for more information.
- Explain your reasoning: As you incorporate the hint, explain why you’re making certain decisions.
Example thought process:
"I see how using a hash map could improve the time complexity of my solution. Currently, I'm using a nested loop to find pairs, which has O(n^2) time complexity. By using a hash map, I could potentially reduce that to O(n). Let me walk through how I might implement this..."
3. Demonstrate Independent Problem-Solving
While it’s important to use hints when offered, you should also demonstrate your ability to solve problems independently. Here’s how to strike a balance:
- Continue to generate your own ideas: Don’t rely solely on the interviewer’s hints. Keep brainstorming and suggesting your own approaches.
- Show your work: Even if a hint leads you to the correct solution, explain each step of your implementation process.
- Discuss trade-offs: Compare the hinted approach with your original idea, discussing the pros and cons of each.
- Extend the solution: Once you’ve implemented the hint, consider how you might optimize or expand upon it.
Example of demonstrating independent thinking:
"Now that I've implemented the solution using a hash map as you suggested, I'm wondering if we could further optimize this for space complexity. One idea I have is to use a two-pointer approach instead, which would reduce our space complexity to O(1). Would you like me to explore that option as well?"
4. Use Hints as Learning Opportunities
Hints can be valuable learning experiences, even beyond the scope of the current problem. Here’s how to maximize their educational value:
- Reflect on the underlying concept: Consider why the hinted approach is effective and how it might apply to other problems.
- Ask about related problems: Inquire if there are similar problems where this technique would be useful.
- Discuss variations: Consider how the hinted approach might change if the problem constraints were different.
- Connect to your existing knowledge: Try to relate the hint to concepts or problems you’re already familiar with.
Example of using a hint as a learning opportunity:
"I see how using a stack solves this particular problem efficiently. Are there other types of problems where a stack would be the optimal data structure? I'm curious about how I might recognize when to use this approach in the future."
Common Pitfalls to Avoid When Using Coding Interview Hints
While hints can be helpful, there are several pitfalls to be aware of when using them during a coding interview:
1. Over-reliance on Hints
One of the most common mistakes candidates make is becoming too dependent on hints. This can manifest in several ways:
- Waiting for hints instead of attempting the problem: Some candidates may hesitate to start solving the problem, hoping the interviewer will provide hints.
- Asking for hints too frequently: Constantly asking for hints can give the impression that you lack problem-solving skills.
- Failing to generate your own ideas: If you only pursue ideas suggested by the interviewer, you miss opportunities to demonstrate your creativity and independent thinking.
To avoid this pitfall, always start by attempting the problem on your own. Only use hints as a last resort when you’re truly stuck or to verify your approach.
2. Misinterpreting Hints
Sometimes, candidates may misunderstand the hint provided or apply it incorrectly. This can lead to confusion and wasted time. To avoid this:
- Clarify your understanding: Always restate the hint in your own words to ensure you’ve interpreted it correctly.
- Ask follow-up questions: If you’re unsure about how to apply the hint, don’t hesitate to ask for clarification.
- Consider multiple interpretations: A hint may have more than one possible application. Consider different ways it could be relevant to the problem.
Example of clarifying a hint:
"You mentioned using a sliding window approach. Just to make sure I understand, are you suggesting I maintain a window of fixed size, or should the window size be variable based on certain conditions?"
3. Neglecting to Explain Your Reasoning
Even when using a hint, it’s crucial to articulate your thought process. Some candidates make the mistake of simply implementing the hinted solution without explaining their reasoning. To avoid this:
- Verbalize your thoughts: Explain why you think the hint is helpful and how it fits into your overall approach.
- Discuss alternatives: Compare the hinted approach with other methods you’ve considered.
- Explain each step: As you implement the solution, describe what you’re doing and why.
Example of explaining reasoning:
"The hint about using a binary search tree makes sense because it would allow us to maintain a sorted structure while providing efficient insertion and lookup operations. This could be particularly useful for this problem because..."
4. Ignoring the Broader Context
Sometimes, candidates become so focused on implementing the hint that they lose sight of the broader problem or fail to consider edge cases. To avoid this:
- Keep the problem requirements in mind: Make sure your solution still addresses all aspects of the original problem.
- Consider edge cases: Think about how the hinted approach handles various input scenarios, including edge cases.
- Discuss time and space complexity: Analyze how the hint affects the efficiency of your solution.
Example of considering the broader context:
"Now that we've implemented the solution using a hash set as hinted, let's consider how this handles our edge cases. For instance, what happens if we have duplicate elements in the input? And how does this affect our space complexity compared to our original approach?"
Balancing Hint Usage with Independent Problem-Solving
The key to successfully using hints in a coding interview is to strike a balance between leveraging the interviewer’s guidance and demonstrating your own problem-solving abilities. Here are some strategies to help you maintain this balance:
1. Start with Your Own Approach
Always begin by attempting to solve the problem on your own. This shows initiative and allows you to demonstrate your problem-solving process. Even if your initial approach isn’t optimal, it provides a starting point for discussion and improvement.
Example of starting with your own approach:
"My initial thought is to approach this using a brute force method. I know it might not be the most efficient, but it will help me understand the problem better. I'll start by implementing this, and then we can discuss potential optimizations."
2. Use Hints to Overcome Obstacles
When you encounter a significant roadblock, it’s appropriate to use a hint. However, try to overcome smaller hurdles on your own. This shows perseverance and problem-solving skills.
Example of using a hint to overcome an obstacle:
"I'm having trouble figuring out how to efficiently update all affected elements after each operation. The hint about using a segment tree is helpful here. Let me think through how I can implement that..."
3. Incorporate Hints into Your Thinking Process
When you receive a hint, don’t simply implement it verbatim. Instead, integrate it into your thought process and explain how it changes or enhances your approach.
Example of incorporating a hint:
"The suggestion to use dynamic programming is interesting. I can see how we could build up our solution incrementally. Let me walk you through how I'm thinking about restructuring my approach to incorporate this idea..."
4. Extend Beyond the Hint
After successfully implementing a hint, take the initiative to extend the solution or discuss potential improvements. This demonstrates that you can build upon given information and think critically about optimizations.
Example of extending beyond the hint:
"Now that we've implemented the solution using a trie as suggested, I'm wondering if we could further optimize the space usage. One idea I have is to compress the trie by merging nodes with only one child. Would you like me to explore that optimization?"
5. Reflect on Lessons Learned
At the end of the problem or interview, take a moment to reflect on what you’ve learned from the hints provided. This shows a growth mindset and the ability to learn from the interview experience.
Example of reflecting on lessons learned:
"The hint about using a monotonic stack was really insightful. It's made me realize I need to practice more problems involving stack-based solutions. Are there any resources you'd recommend for improving in this area?"
Conclusion: Mastering the Art of Using Coding Interview Hints
Effectively using hints in a coding interview is a skill that can significantly impact your performance and the interviewer’s perception of your abilities. By acknowledging hints graciously, incorporating them thoughtfully, and continuing to demonstrate independent problem-solving skills, you can make the most of the guidance offered while still showcasing your own capabilities.
Remember, the goal is not just to solve the problem, but to demonstrate your thought process, communication skills, and ability to learn and adapt. Hints should be viewed as tools to enhance your problem-solving approach, not crutches to rely on entirely.
As you prepare for coding interviews, practice integrating hints into your problem-solving process. Work on problems with a study partner who can provide hints, and focus on verbalizing your thoughts as you incorporate these suggestions. With practice, you’ll become more adept at balancing hint usage with independent thinking, ultimately presenting yourself as a strong, adaptable problem solver in your coding interviews.
Remember, every hint is an opportunity – not just to solve the current problem, but to learn something new that you can apply to future challenges in your programming career. Approach coding interviews with this mindset, and you’ll be well-equipped to handle whatever problems come your way, with or without hints.