Loops Demystified: From ‘For’ to ‘While’
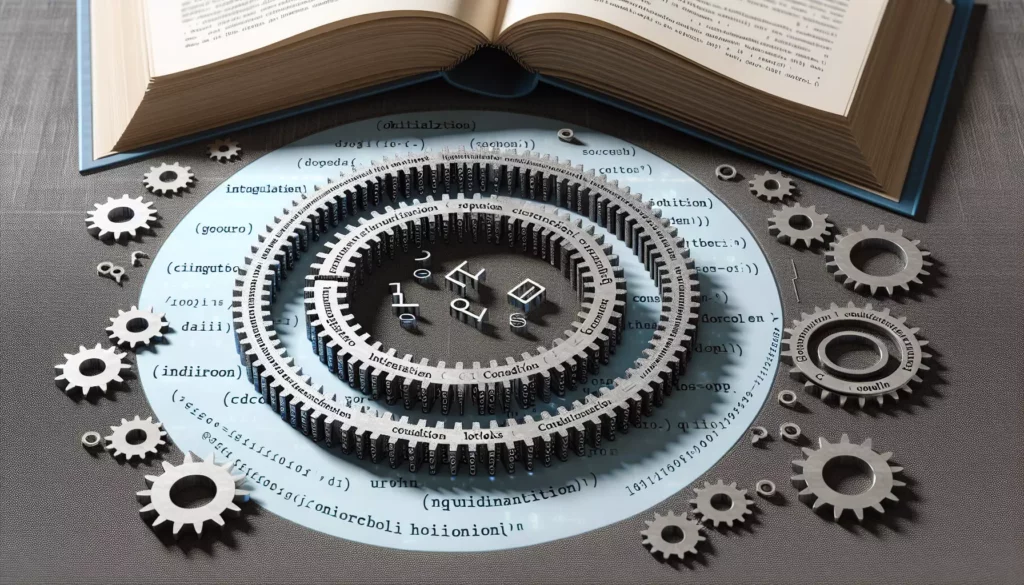
In the world of programming, loops are like the heartbeat of your code. They allow you to repeat actions, process data, and create dynamic behaviors in your programs. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, understanding loops is crucial. In this comprehensive guide, we’ll demystify loops, focusing on two of the most common types: ‘for’ loops and ‘while’ loops.
What Are Loops?
Before we dive into the specifics of ‘for’ and ‘while’ loops, let’s establish a solid foundation by understanding what loops are and why they’re so important in programming.
Loops are control structures that allow a set of instructions to be repeated multiple times. They’re essential for automating repetitive tasks, iterating over data structures, and creating efficient, scalable code. Without loops, many programming tasks would be tedious, error-prone, and impractical.
The ‘For’ Loop
The ‘for’ loop is one of the most commonly used loop structures in programming. It’s particularly useful when you know in advance how many times you want to execute a block of code.
Anatomy of a ‘For’ Loop
A typical ‘for’ loop consists of three main components:
- Initialization: This is where you set up the initial value of your loop counter.
- Condition: This is the test that determines whether the loop should continue or stop.
- Increment/Decrement: This is where you update the loop counter after each iteration.
Here’s a basic structure of a ‘for’ loop in Python:
for i in range(start, end, step):
# Code to be repeated
And here’s how it looks in JavaScript:
for (let i = start; i < end; i += step) {
// Code to be repeated
}
Example: Printing Numbers
Let’s look at a simple example where we use a ‘for’ loop to print numbers from 1 to 5:
for i in range(1, 6):
print(i)
This will output:
1
2
3
4
5
When to Use ‘For’ Loops
‘For’ loops are ideal in situations where:
- You know the exact number of iterations needed.
- You’re iterating over a sequence (like a list or array).
- You need to perform an action a specific number of times.
The ‘While’ Loop
The ‘while’ loop is another fundamental loop structure in programming. Unlike ‘for’ loops, ‘while’ loops are used when you don’t know in advance how many times the loop needs to run.
Anatomy of a ‘While’ Loop
A ‘while’ loop consists of two main components:
- Condition: This is the test that determines whether the loop should continue or stop.
- Loop Body: This is the code that gets executed as long as the condition is true.
Here’s the basic structure of a ‘while’ loop in Python:
while condition:
# Code to be repeated
And in JavaScript:
while (condition) {
// Code to be repeated
}
Example: Counting Down
Let’s look at an example where we use a ‘while’ loop to count down from 5 to 1:
count = 5
while count > 0:
print(count)
count -= 1
This will output:
5
4
3
2
1
When to Use ‘While’ Loops
‘While’ loops are ideal in situations where:
- You don’t know how many iterations are needed in advance.
- The loop should continue until a certain condition is met.
- You’re dealing with user input or external data that determines when to stop.
Comparing ‘For’ and ‘While’ Loops
Now that we’ve explored both ‘for’ and ‘while’ loops, let’s compare them to understand their strengths and use cases better.
Clarity and Readability
‘For’ loops are often more readable when dealing with a known number of iterations or when iterating over a sequence. They clearly express the start, end, and step of the loop in a single line.
‘While’ loops, on the other hand, are more flexible and can be more readable when the termination condition is complex or when the number of iterations is unknown.
Flexibility
‘While’ loops offer more flexibility in terms of the conditions that can control the loop. They can use complex boolean expressions or even functions to determine whether to continue or stop.
‘For’ loops are more structured and are typically used for simpler, more predictable iteration patterns.
Performance
In most cases, the performance difference between ‘for’ and ‘while’ loops is negligible. However, ‘for’ loops can sometimes be slightly more efficient in certain languages because the compiler can optimize them more easily.
Risk of Infinite Loops
‘While’ loops carry a higher risk of creating infinite loops if the termination condition is not properly managed. It’s crucial to ensure that the condition will eventually become false.
‘For’ loops are less prone to infinite loops because the iteration count is typically predetermined.
Advanced Loop Concepts
As you become more comfortable with basic loops, it’s important to explore some advanced concepts that can make your code more efficient and expressive.
Nested Loops
Nested loops are loops within loops. They’re useful for working with multi-dimensional data structures or when you need to perform complex iterations. Here’s an example of nested ‘for’ loops to create a multiplication table:
for i in range(1, 6):
for j in range(1, 6):
print(f"{i * j:2}", end=" ")
print() # Move to the next line after each row
This will output:
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
Loop Control Statements
Loop control statements allow you to alter the flow of a loop. The two most common are:
- break: Exits the loop immediately.
- continue: Skips the rest of the current iteration and moves to the next one.
Here’s an example using both:
for i in range(10):
if i == 3:
continue # Skip 3
if i == 7:
break # Stop at 7
print(i)
This will output:
0
1
2
4
5
6
List Comprehensions (Python)
In Python, list comprehensions provide a concise way to create lists based on existing lists. They’re essentially a compact form of a ‘for’ loop. Here’s an example:
squares = [x**2 for x in range(10)]
print(squares)
This will output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Iterators and Generators
Iterators and generators are advanced concepts that allow for more memory-efficient looping, especially when dealing with large datasets. They provide a way to generate values on-the-fly rather than storing them all in memory.
Here’s a simple generator function in Python:
def countdown(n):
while n > 0:
yield n
n -= 1
for num in countdown(5):
print(num)
This will output:
5
4
3
2
1
Common Pitfalls and Best Practices
As you work with loops, it’s important to be aware of common pitfalls and follow best practices to write efficient, bug-free code.
Infinite Loops
One of the most common mistakes is creating an infinite loop. This happens when the loop condition never becomes false. Always ensure that your loop has a way to terminate.
Example of an infinite loop (avoid this!):
while True:
print("This will never end!")
Off-by-One Errors
Off-by-one errors occur when you iterate one too many or one too few times. Be careful with your loop conditions, especially when using < vs <= or > vs >=.
Modifying Loop Variables
Be cautious when modifying loop variables within the loop body, especially in ‘for’ loops. This can lead to unexpected behavior.
Performance Considerations
When working with large datasets or performance-critical code, consider:
- Using appropriate data structures (e.g., sets for faster lookups).
- Moving invariant computations outside the loop.
- Using built-in functions or library methods that are optimized for performance.
Readability and Maintainability
Write clear, readable loop code:
- Use meaningful variable names for loop counters and iterators.
- Keep loop bodies concise. If a loop body is too long, consider extracting it into a separate function.
- Use comments to explain complex loop logic.
Real-World Applications of Loops
Understanding loops is crucial because they’re used extensively in real-world programming scenarios. Let’s explore some common applications:
Data Processing
Loops are fundamental in data processing tasks. For example, when working with large datasets, you might use loops to:
- Clean and normalize data
- Perform calculations on each data point
- Filter data based on certain conditions
Here’s a simple example of using a loop to calculate the average of a list of numbers:
numbers = [10, 20, 30, 40, 50]
total = 0
for num in numbers:
total += num
average = total / len(numbers)
print(f"The average is: {average}")
Web Scraping
When scraping data from websites, loops are used to iterate over multiple pages or elements. Here’s a conceptual example using Python’s requests library:
import requests
for page_num in range(1, 11):
url = f"https://example.com/page/{page_num}"
response = requests.get(url)
# Process the response here
Game Development
In game development, loops are crucial for creating game loops that continuously update the game state and render graphics. Here’s a simple conceptual game loop:
while game_is_running:
process_user_input()
update_game_state()
render_graphics()
check_for_game_over()
File Operations
When working with files, loops are often used to process data line by line. Here’s an example of reading a file and counting the number of lines:
line_count = 0
with open('example.txt', 'r') as file:
for line in file:
line_count += 1
print(f"The file has {line_count} lines.")
API Interactions
When working with APIs, loops can be used to paginate through results or to process multiple API endpoints. Here’s a conceptual example:
import requests
api_key = "your_api_key"
base_url = "https://api.example.com/data"
page = 1
while True:
response = requests.get(f"{base_url}?page={page}&api_key={api_key}")
data = response.json()
if not data: # No more data to process
break
process_data(data)
page += 1
Loops in Different Programming Paradigms
As you advance in your programming journey, you’ll encounter different programming paradigms. Let’s briefly explore how loops are used (or avoided) in different paradigms:
Functional Programming
In functional programming, traditional loops are often replaced by recursive functions or higher-order functions like map, filter, and reduce. This approach emphasizes immutability and avoids side effects.
Example of using map instead of a loop in Python:
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, numbers))
print(squared) # Output: [1, 4, 9, 16, 25]
Object-Oriented Programming (OOP)
In OOP, loops are often encapsulated within methods of objects. This allows for better organization and reusability of code.
Example of a class using a loop in its method:
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, item):
self.items.append(item)
def calculate_total(self):
total = 0
for item in self.items:
total += item.price
return total
Declarative Programming
In declarative programming, the focus is on describing what you want to achieve, rather than specifying the exact steps. SQL is a good example of a declarative language where loops are often implicit.
Example of an SQL query that implicitly loops over data:
SELECT name, AVG(score) as average_score
FROM students
GROUP BY name;
Conclusion
Loops are a fundamental concept in programming, serving as the backbone for many algorithms and processes. Whether you’re using a ‘for’ loop to iterate over a known sequence or a ‘while’ loop to continue until a condition is met, understanding loops is crucial for writing efficient and effective code.
As you progress in your coding journey, you’ll find that mastering loops opens up a world of possibilities. From simple data processing tasks to complex game development, loops are everywhere in programming. By understanding when and how to use different types of loops, you’ll be better equipped to solve a wide range of programming challenges.
Remember, practice is key. Experiment with different types of loops, try solving problems using both ‘for’ and ‘while’ loops, and don’t be afraid to explore more advanced concepts like list comprehensions or generators. With time and experience, you’ll develop an intuition for when to use each type of loop and how to write loop-based code that is both efficient and readable.
Happy coding, and may your loops always terminate!