Longest Increasing Subsequence: A Comprehensive Guide
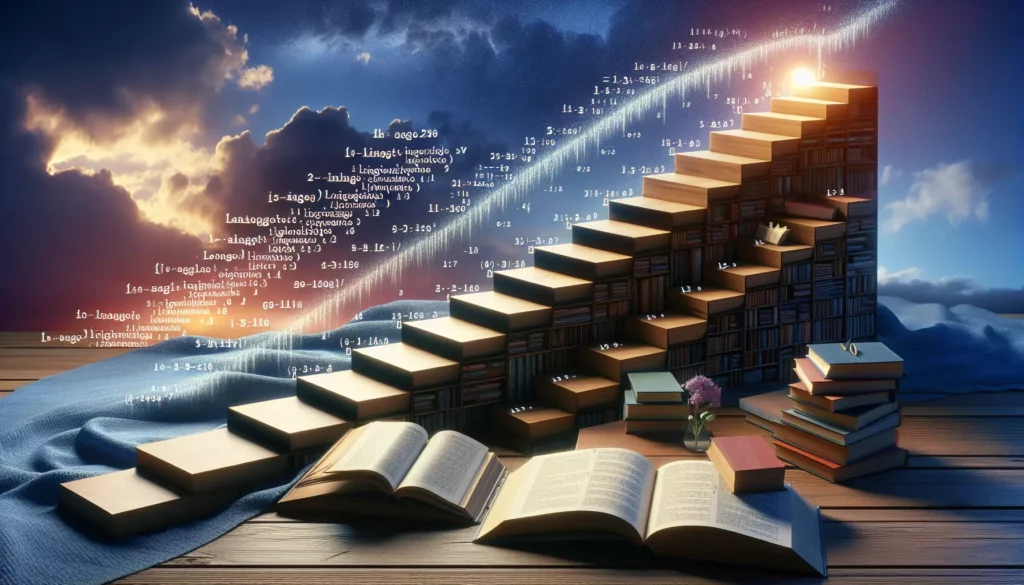
In the world of algorithmic problem-solving, the Longest Increasing Subsequence (LIS) problem stands out as a classic challenge that combines elements of dynamic programming and binary search. This problem not only serves as a great learning tool for aspiring programmers but also has practical applications in various fields, including bioinformatics and data analysis. In this comprehensive guide, we’ll dive deep into the Longest Increasing Subsequence problem, exploring its definition, various solution approaches, and real-world applications.
What is the Longest Increasing Subsequence?
Before we delve into the intricacies of solving the LIS problem, let’s first understand what it actually means:
The Longest Increasing Subsequence of a given sequence is the longest subsequence of the original sequence in which the subsequence’s elements are in strictly increasing order. This subsequence is not necessarily contiguous, or unique.
For example, consider the sequence [10, 9, 2, 5, 3, 7, 101, 18]:
- One possible longest increasing subsequence is [2, 5, 7, 101]
- Another valid LIS is [2, 5, 7, 18]
Both of these subsequences have a length of 4, which is the maximum possible for this given sequence.
Why is the LIS Problem Important?
The Longest Increasing Subsequence problem is significant for several reasons:
- Algorithmic Thinking: It’s an excellent problem for developing algorithmic thinking skills, as it can be solved using various approaches, each with its own trade-offs.
- Dynamic Programming: It serves as a classic example of dynamic programming, helping programmers understand this powerful problem-solving technique.
- Binary Search Application: The optimal solution involves a clever application of binary search, showcasing how this search algorithm can be used in unexpected contexts.
- Real-world Applications: The LIS problem has applications in various fields, including sequence alignment in bioinformatics, text analysis, and stock market prediction.
Approaches to Solving the LIS Problem
There are several approaches to solving the Longest Increasing Subsequence problem, each with its own time and space complexity. We’ll explore three main approaches: the brute force method, the dynamic programming solution, and the optimal solution using dynamic programming with binary search.
1. Brute Force Approach
The brute force approach involves generating all possible subsequences of the given sequence and checking which ones are in increasing order. While this method is straightforward, it’s highly inefficient with a time complexity of O(2^n), making it impractical for larger sequences.
2. Dynamic Programming Solution
The dynamic programming solution improves upon the brute force approach by breaking down the problem into smaller subproblems and storing their solutions to avoid redundant computations. Here’s how it works:
- Create an array
dp
of the same length as the input sequence, initialized with 1s (as each element is an increasing subsequence of length 1 by itself). - For each element at index i, look at all previous elements j where j < i:
- If the element at index i is greater than the element at index j, update dp[i] to be the maximum of its current value and dp[j] + 1.
- The maximum value in the dp array will be the length of the longest increasing subsequence.
Here’s a Python implementation of this approach:
def lengthOfLIS(nums):
if not nums:
return 0
n = len(nums)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if nums[i] > nums[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
# Example usage
sequence = [10, 9, 2, 5, 3, 7, 101, 18]
print(lengthOfLIS(sequence)) # Output: 4
This solution has a time complexity of O(n^2) and a space complexity of O(n), where n is the length of the input sequence. While this is a significant improvement over the brute force approach, we can do even better.
3. Optimal Solution: Dynamic Programming with Binary Search
The optimal solution to the LIS problem combines dynamic programming with binary search, achieving a time complexity of O(n log n). This approach maintains a separate array that represents the smallest tail of all increasing subsequences of each length.
Here’s how it works:
- Initialize an empty array
tails
to store the smallest tail of all increasing subsequences. - For each number in the input sequence:
- If it’s larger than all tails, append it to tails.
- If it’s smaller than all tails, it will become the new smallest tail of length 1.
- If it’s in between, use binary search to find the smallest tail that’s larger than or equal to the current number, and update that tail.
- The length of the tails array at the end is the length of the longest increasing subsequence.
Here’s a Python implementation of this optimal approach:
def lengthOfLIS(nums):
tails = []
for num in nums:
if not tails or num > tails[-1]:
tails.append(num)
else:
idx = bisect_left(tails, num)
tails[idx] = num
return len(tails)
from bisect import bisect_left
# Example usage
sequence = [10, 9, 2, 5, 3, 7, 101, 18]
print(lengthOfLIS(sequence)) # Output: 4
This solution achieves a time complexity of O(n log n) and a space complexity of O(n), making it much more efficient for larger sequences.
Variations and Extensions of the LIS Problem
The Longest Increasing Subsequence problem has several interesting variations and extensions that are worth exploring:
1. Longest Non-decreasing Subsequence
This variation allows for equal elements in the subsequence. The solution is similar to the LIS problem, but the comparison changes from strictly greater than (>) to greater than or equal to (>=).
2. Longest Decreasing Subsequence
This is essentially the same problem as LIS, but looking for a decreasing sequence instead. It can be solved by either reversing the input array or changing the comparison operator.
3. Longest Bitonic Subsequence
A bitonic subsequence is one that first increases and then decreases. This problem can be solved by running the LIS algorithm twice – once from left to right and once from right to left – and then finding the maximum sum of the two results minus 1 (to avoid counting the peak twice).
4. Minimum Number of Removals to Make Mountain Array
This leetcode problem (1671) is an interesting application of the LIS concept. A mountain array is an array that is strictly increasing to some peak element, then strictly decreasing afterwards.
5. Building Bridges
This is a 2D variation of the LIS problem where you need to find the maximum number of bridges that can be built between two parallel rivers without crossing.
Real-world Applications of LIS
The Longest Increasing Subsequence problem and its variations have several practical applications in various fields:
1. Bioinformatics
In bioinformatics, the LIS algorithm is used for sequence alignment and finding common subsequences in DNA or protein sequences. This helps in understanding evolutionary relationships between species and identifying conserved regions in genomes.
2. Text Analysis and Compression
LIS can be used in text analysis to find patterns and in text compression algorithms to identify redundant sequences that can be compressed.
3. Computer Graphics
In computer graphics, particularly in the field of ray tracing, LIS-like algorithms are used to optimize the rendering process by identifying and processing similar rays together.
4. Stock Market Analysis
LIS can be applied to stock price data to identify trends and potential investment opportunities. For example, finding the longest increasing subsequence in stock prices could indicate a strong upward trend.
5. Network Routing
In network routing algorithms, concepts similar to LIS are used to find optimal paths through a network while satisfying certain constraints.
Tips for Mastering the LIS Problem
To truly master the Longest Increasing Subsequence problem and its variations, consider the following tips:
- Understand the core concept: Make sure you fully grasp the idea of a subsequence versus a subarray, and what makes a subsequence increasing.
- Practice multiple approaches: Implement both the O(n^2) dynamic programming solution and the O(n log n) solution with binary search. Understanding both will give you a better grasp of the problem.
- Visualize the problem: Try to visualize how the LIS is formed as you process each element. This can help in understanding why certain approaches work.
- Solve variations: Once you’re comfortable with the basic LIS problem, try solving its variations like the Longest Bitonic Subsequence or the Building Bridges problem.
- Optimize space: For the dynamic programming solution, try to optimize the space usage. Can you solve it using only O(n) space instead of O(n^2)?
- Implement binary search: For the optimal solution, implement your own binary search function instead of relying on built-in functions. This will deepen your understanding of how it works in this context.
- Consider edge cases: Always think about edge cases like an empty input array, an array with all equal elements, or an array in descending order.
Conclusion
The Longest Increasing Subsequence problem is a fascinating algorithmic challenge that offers valuable insights into dynamic programming, binary search, and algorithmic optimization. By mastering this problem and its variations, you’ll not only improve your problem-solving skills but also gain techniques that are applicable to a wide range of real-world scenarios.
As you continue your journey in algorithmic problem-solving, remember that understanding the LIS problem is just one step. The key is to apply these concepts to new problems, always looking for opportunities to optimize and improve your solutions. Happy coding!