Linked Lists vs. Arrays: When and Why to Use Each Data Structure
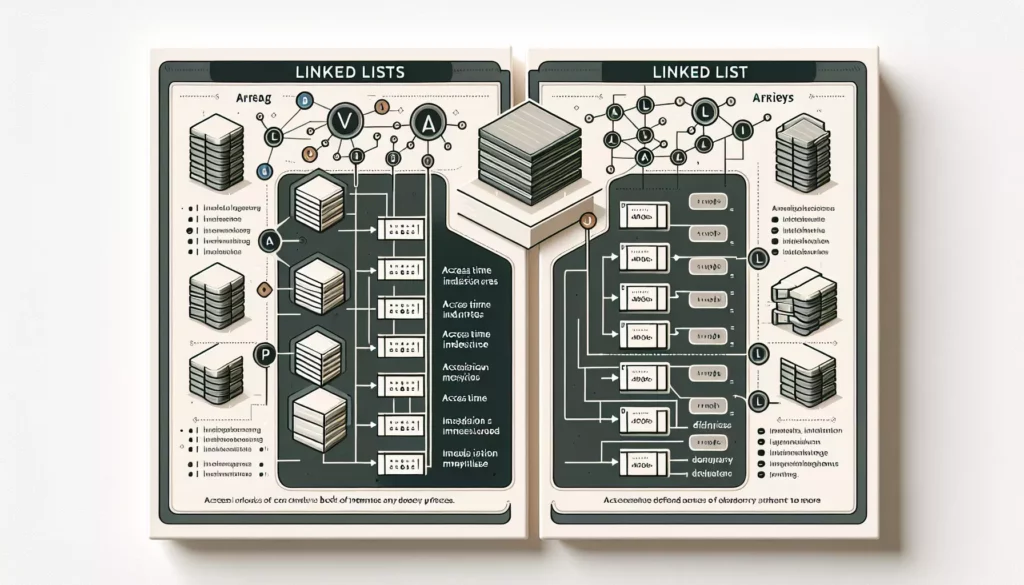
In the world of programming and computer science, data structures play a crucial role in organizing and managing information efficiently. Two fundamental data structures that often come up in discussions and technical interviews are linked lists and arrays. While both serve the purpose of storing collections of data, they have distinct characteristics that make them suitable for different scenarios. In this comprehensive guide, we’ll dive deep into the world of linked lists and arrays, exploring their strengths, weaknesses, and optimal use cases.
Understanding Linked Lists
A linked list is a linear data structure where elements are stored in nodes. Each node contains two main components: the data itself and a reference (or link) to the next node in the sequence. This structure allows for dynamic size allocation and efficient insertion and deletion operations.
Types of Linked Lists
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node has references to both the next and previous nodes.
- Circular Linked List: The last node points back to the first node, creating a circle.
Implementing a Basic Singly Linked List in Python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def display(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Usage
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
ll.display() # Output: 1 -> 2 -> 3 -> None
Advantages of Linked Lists
- Dynamic Size: Linked lists can grow or shrink in size during runtime without the need for reallocation.
- Efficient Insertions and Deletions: Adding or removing elements at the beginning or middle of the list is generally more efficient than with arrays.
- Memory Efficiency: Linked lists only use the memory they need, which can be advantageous when dealing with large datasets with unknown sizes.
- Implementation of Other Data Structures: Linked lists serve as the foundation for implementing other data structures like stacks, queues, and certain types of trees.
Disadvantages of Linked Lists
- Random Access: Accessing elements by index is slower compared to arrays, as you need to traverse the list from the beginning.
- Extra Memory Usage: Each node requires additional memory to store the reference to the next node.
- Cache Performance: Linked lists have poor cache locality, which can impact performance in certain scenarios.
Understanding Arrays
An array is a collection of elements stored in contiguous memory locations. Each element can be accessed directly using an index. Arrays are one of the most fundamental and widely used data structures in programming.
Types of Arrays
- Static Arrays: Fixed-size arrays where the size is determined at compile-time.
- Dynamic Arrays: Resizable arrays that can grow or shrink during runtime (e.g., ArrayList in Java, List in Python).
- Multidimensional Arrays: Arrays with two or more dimensions, often used to represent matrices or tables.
Implementing and Using Arrays in Python
# Static-like array (list with a fixed size)
static_array = [0] * 5
static_array[2] = 42
print(static_array) # Output: [0, 0, 42, 0, 0]
# Dynamic array (standard Python list)
dynamic_array = []
dynamic_array.append(1)
dynamic_array.append(2)
dynamic_array.append(3)
print(dynamic_array) # Output: [1, 2, 3]
# Multidimensional array
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(matrix[1][1]) # Output: 5
Advantages of Arrays
- Fast Random Access: Elements can be accessed directly using their index in O(1) time.
- Memory Efficiency: Arrays use a contiguous block of memory, which can be more efficient for certain operations.
- Cache Friendliness: The contiguous memory layout of arrays leads to better cache performance.
- Simplicity: Arrays are straightforward to use and understand, making them a good choice for simple data storage needs.
Disadvantages of Arrays
- Fixed Size (for static arrays): Once declared, the size of a static array cannot be changed.
- Inefficient Insertions and Deletions: Adding or removing elements from the middle of an array requires shifting other elements, which can be costly for large arrays.
- Wasted Space: If not all elements are used, there might be unused memory allocated to the array.
Comparing Linked Lists and Arrays
Now that we’ve explored the characteristics of both linked lists and arrays, let’s compare them directly across various aspects:
Aspect | Linked Lists | Arrays |
---|---|---|
Memory Allocation | Dynamic | Static (for fixed arrays) or Dynamic (for resizable arrays) |
Element Access | O(n) – Sequential access | O(1) – Random access |
Insertion at Beginning | O(1) | O(n) – Requires shifting elements |
Insertion at End | O(n) for singly linked list, O(1) for doubly linked list with tail pointer | O(1) amortized for dynamic arrays, O(n) if resizing is needed |
Deletion at Beginning | O(1) | O(n) – Requires shifting elements |
Deletion at End | O(n) for singly linked list, O(1) for doubly linked list | O(1) |
Memory Overhead | Higher (due to storage of next/prev pointers) | Lower |
Cache Performance | Poor | Good |
When to Use Linked Lists
Linked lists are particularly useful in the following scenarios:
- Dynamic Size Requirements: When you need a data structure that can easily grow or shrink without reallocation.
- Frequent Insertions/Deletions: If your application requires frequent insertions or deletions, especially at the beginning or middle of the list.
- Implementation of Other Data Structures: When building data structures like stacks, queues, or certain types of trees.
- Memory Management: In situations where memory is a concern and you want to allocate memory as needed.
- Polynomial Manipulation: Linked lists are often used to represent polynomials, where each node represents a term.
Real-World Applications of Linked Lists
- Music Player Playlists: Linked lists can be used to implement the “next” and “previous” functionality in music players.
- Undo Functionality in Applications: A stack implemented using a linked list can efficiently manage undo operations.
- Hash Tables with Chaining: Linked lists are used in the implementation of hash tables to handle collisions.
- Memory Management in Operating Systems: Linked lists help manage free memory blocks in operating systems.
When to Use Arrays
Arrays are preferable in the following situations:
- Random Access Needs: When you need fast, constant-time access to elements by their index.
- Fixed Size Requirements: If you know the size of your data structure in advance and it won’t change.
- Cache-Sensitive Operations: For applications where cache performance is critical.
- Mathematical Operations: Arrays are well-suited for mathematical operations, especially in linear algebra.
- Sorting Algorithms: Many efficient sorting algorithms are designed to work with arrays.
Real-World Applications of Arrays
- Image Processing: Images are often represented as 2D arrays of pixels.
- Spreadsheets: The grid structure of spreadsheets is typically implemented using 2D arrays.
- Sensor Data Collection: Arrays are used to store time-series data from sensors.
- Database Indexing: Some database systems use arrays for efficient indexing and searching.
Hybrid Approaches and Advanced Considerations
In practice, the choice between linked lists and arrays isn’t always binary. There are scenarios where hybrid approaches or more advanced data structures might be more appropriate:
1. Array of Linked Lists
This hybrid structure combines the random access capability of arrays with the dynamic nature of linked lists. It’s commonly used in hash table implementations to handle collisions.
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.next = None
class HashTable:
def __init__(self, size):
self.size = size
self.table = [None] * size
def hash_function(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self.hash_function(key)
if not self.table[index]:
self.table[index] = Node(key, value)
else:
current = self.table[index]
while current.next:
if current.key == key:
current.value = value
return
current = current.next
current.next = Node(key, value)
def get(self, key):
index = self.hash_function(key)
current = self.table[index]
while current:
if current.key == key:
return current.value
current = current.next
return None
# Usage
ht = HashTable(10)
ht.insert("apple", 5)
ht.insert("banana", 7)
print(ht.get("apple")) # Output: 5
2. Unrolled Linked Lists
An unrolled linked list is a variation where each node contains an array of elements instead of a single element. This structure aims to combine the benefits of both linked lists and arrays.
class Node:
def __init__(self, capacity):
self.capacity = capacity
self.size = 0
self.elements = [None] * capacity
self.next = None
class UnrolledLinkedList:
def __init__(self, node_capacity):
self.head = Node(node_capacity)
self.node_capacity = node_capacity
def insert(self, data):
current = self.head
while current.size == current.capacity:
if not current.next:
current.next = Node(self.node_capacity)
current = current.next
current.elements[current.size] = data
current.size += 1
def display(self):
current = self.head
while current:
print(current.elements[:current.size], end=" -> ")
current = current.next
print("None")
# Usage
ull = UnrolledLinkedList(3)
for i in range(10):
ull.insert(i)
ull.display() # Output: [0, 1, 2] -> [3, 4, 5] -> [6, 7, 8] -> [9] -> None
3. Dynamic Arrays (e.g., ArrayList in Java, List in Python)
Dynamic arrays combine the benefits of static arrays (random access) with the ability to resize. They’re implemented in many programming languages as the default “list” type.
class DynamicArray:
def __init__(self):
self.array = [None] * 2
self.size = 0
self.capacity = 2
def append(self, element):
if self.size == self.capacity:
self._resize()
self.array[self.size] = element
self.size += 1
def _resize(self):
new_array = [None] * (self.capacity * 2)
for i in range(self.size):
new_array[i] = self.array[i]
self.array = new_array
self.capacity *= 2
def __getitem__(self, index):
if 0 <= index < self.size:
return self.array[index]
raise IndexError("Index out of range")
def __str__(self):
return str(self.array[:self.size])
# Usage
da = DynamicArray()
for i in range(10):
da.append(i)
print(da) # Output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(da[5]) # Output: 5
Performance Considerations and Big O Notation
When choosing between linked lists and arrays, it’s crucial to consider the time complexity of various operations. Big O notation is used to describe the upper bound of the time complexity of an algorithm. Here’s a comparison of common operations:
Operation | Linked List | Array |
---|---|---|
Access by Index | O(n) | O(1) |
Search | O(n) | O(n) |
Insertion at Beginning | O(1) | O(n) |
Insertion at End | O(n) or O(1) with tail pointer | O(1) amortized |
Insertion in Middle | O(n) | O(n) |
Deletion at Beginning | O(1) | O(n) |
Deletion at End | O(n) or O(1) with tail pointer | O(1) |
Deletion in Middle | O(n) | O(n) |
Making the Right Choice: Factors to Consider
When deciding between linked lists and arrays, consider the following factors:
- Access Pattern: If you need frequent random access, arrays are generally better. If you mostly access elements sequentially, linked lists might be suitable.
- Size Flexibility: If the size of your data structure needs to change frequently, linked lists or dynamic arrays are preferable.
- Insertion/Deletion Frequency: If you often insert or delete elements at the beginning or middle of the structure, linked lists have an advantage.
- Memory Constraints: Consider the available memory and the overhead of each structure.
- Performance Requirements: Analyze the time complexity of the operations you’ll perform most frequently.
- Cache Considerations: If cache performance is critical, arrays typically have better cache locality.
- Implementation Complexity: Arrays are generally simpler to implement and use.
Conclusion
Choosing between linked lists and arrays is not always a straightforward decision. Both data structures have their strengths and weaknesses, and the best choice depends on the specific requirements of your application. By understanding the characteristics, performance implications, and use cases of each structure, you can make informed decisions that lead to more efficient and effective code.
Remember that in many real-world scenarios, you might not need to implement these data structures from scratch. Most programming languages and frameworks provide optimized implementations of both arrays and list-like structures. However, understanding the underlying principles is crucial for making the right choices and optimizing your code when necessary.
As you continue to develop your programming skills, practice implementing and using both linked lists and arrays. Experiment with different scenarios and analyze how each structure performs. This hands-on experience, combined with theoretical knowledge, will help you become a more proficient and versatile programmer, capable of choosing the right tool for each job.