Leveraging Two-Pointer Techniques in Array Problems
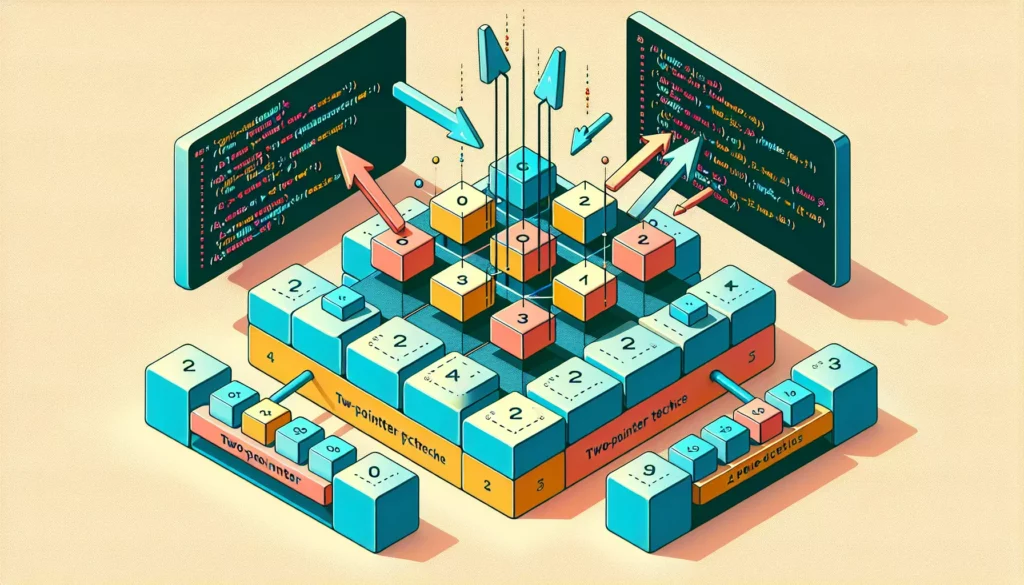
In the world of coding interviews and algorithmic problem-solving, mastering array manipulation is crucial. One powerful technique that often comes in handy when dealing with array problems is the two-pointer approach. This method can significantly improve the efficiency of your solutions, especially when working with sorted arrays or when you need to find pairs of elements that satisfy certain conditions. In this comprehensive guide, we’ll explore the two-pointer technique, its applications, and how to leverage it effectively in various array problems.
What is the Two-Pointer Technique?
The two-pointer technique is an algorithm design pattern that involves using two pointers to traverse an array or sequence. These pointers can move towards each other, in the same direction, or maintain a specific relationship based on the problem at hand. The primary goal of using two pointers is to reduce the time complexity of the solution, often from O(n²) to O(n), by avoiding nested loops.
Common Two-Pointer Patterns
There are several patterns within the two-pointer technique that you should be familiar with:
1. Opposite Directional Pointers
In this pattern, one pointer starts at the beginning of the array, and the other starts at the end. The pointers move towards each other until they meet or until a specific condition is met.
2. Same Directional Pointers
Both pointers start from the beginning of the array and move in the same direction, but at different speeds or based on different conditions.
3. Sliding Window
This is a variation where two pointers define the boundaries of a subarray or substring, and they move to expand or contract this window based on certain criteria.
Applications of the Two-Pointer Technique
Let’s dive into some common problems where the two-pointer technique can be effectively applied:
1. Reversing an Array
One of the simplest applications of the opposite directional pointers pattern is reversing an array in-place.
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
# Example usage
print(reverse_array([1, 2, 3, 4, 5])) # Output: [5, 4, 3, 2, 1]
Time Complexity: O(n), where n is the length of the array.
Space Complexity: O(1), as we’re reversing in-place.
2. Two Sum Problem
Given a sorted array of integers and a target sum, find two numbers such that they add up to the target number.
def two_sum(numbers, target):
left, right = 0, len(numbers) - 1
while left < right:
current_sum = numbers[left] + numbers[right]
if current_sum == target:
return [left + 1, right + 1] # Adding 1 for 1-indexed output
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
# Example usage
print(two_sum([2, 7, 11, 15], 9)) # Output: [1, 2]
Time Complexity: O(n), where n is the length of the array.
Space Complexity: O(1), as we’re using constant extra space.
3. Container With Most Water
Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it can trap.
def max_area(height):
left, right = 0, len(height) - 1
max_water = 0
while left < right:
water = min(height[left], height[right]) * (right - left)
max_water = max(max_water, water)
if height[left] < height[right]:
left += 1
else:
right -= 1
return max_water
# Example usage
print(max_area([1, 8, 6, 2, 5, 4, 8, 3, 7])) # Output: 49
Time Complexity: O(n), where n is the length of the array.
Space Complexity: O(1), as we’re using constant extra space.
4. Remove Duplicates from Sorted Array
Given a sorted array, remove the duplicates in-place such that each element appears only once and return the new length.
def remove_duplicates(nums):
if not nums:
return 0
# Initialize the pointer for unique elements
unique_pointer = 0
# Iterate through the array
for i in range(1, len(nums)):
if nums[i] != nums[unique_pointer]:
unique_pointer += 1
nums[unique_pointer] = nums[i]
return unique_pointer + 1
# Example usage
nums = [1, 1, 2, 2, 3, 4, 4, 5]
new_length = remove_duplicates(nums)
print(f"New length: {new_length}, Modified array: {nums[:new_length]}")
# Output: New length: 5, Modified array: [1, 2, 3, 4, 5]
Time Complexity: O(n), where n is the length of the array.
Space Complexity: O(1), as we’re modifying the array in-place.
5. Three Sum Problem
Given an array of integers, find all unique triplets in the array which gives the sum of zero.
def three_sum(nums):
nums.sort()
result = []
n = len(nums)
for i in range(n - 2):
if i > 0 and nums[i] == nums[i - 1]:
continue
left, right = i + 1, n - 1
while left < right:
current_sum = nums[i] + nums[left] + nums[right]
if current_sum == 0:
result.append([nums[i], nums[left], nums[right]])
while left < right and nums[left] == nums[left + 1]:
left += 1
while left < right and nums[right] == nums[right - 1]:
right -= 1
left += 1
right -= 1
elif current_sum < 0:
left += 1
else:
right -= 1
return result
# Example usage
print(three_sum([-1, 0, 1, 2, -1, -4]))
# Output: [[-1, -1, 2], [-1, 0, 1]]
Time Complexity: O(n²), where n is the length of the array.
Space Complexity: O(1) if we don’t count the space required to store the output.
Advanced Two-Pointer Techniques
As you become more comfortable with the basic two-pointer patterns, you can explore more advanced applications:
1. Sliding Window Technique
The sliding window technique is a variation of the two-pointer method that is particularly useful for problems involving subarrays or substrings.
Example: Longest Substring Without Repeating Characters
def length_of_longest_substring(s):
char_index = {}
max_length = 0
start = 0
for end, char in enumerate(s):
if char in char_index and char_index[char] >= start:
start = char_index[char] + 1
else:
max_length = max(max_length, end - start + 1)
char_index[char] = end
return max_length
# Example usage
print(length_of_longest_substring("abcabcbb")) # Output: 3
Time Complexity: O(n), where n is the length of the string.
Space Complexity: O(min(m, n)), where m is the size of the character set.
2. Fast and Slow Pointers
This technique is often used in linked list problems but can also be applied to arrays in certain scenarios.
Example: Find the Duplicate Number
def find_duplicate(nums):
slow = fast = nums[0]
# Find the intersection point of the two pointers
while True:
slow = nums[slow]
fast = nums[nums[fast]]
if slow == fast:
break
# Find the entrance to the cycle
slow = nums[0]
while slow != fast:
slow = nums[slow]
fast = nums[fast]
return slow
# Example usage
print(find_duplicate([1, 3, 4, 2, 2])) # Output: 2
Time Complexity: O(n), where n is the length of the array.
Space Complexity: O(1), as we’re using constant extra space.
Tips for Mastering Two-Pointer Techniques
- Identify the Pattern: Practice recognizing problems that can benefit from the two-pointer approach. Often, these problems involve searching, reversing, or finding pairs in arrays or strings.
- Understand the Array Properties: Many two-pointer solutions rely on the array being sorted. If the array isn’t sorted, consider whether sorting it first might simplify the problem.
- Practice Visualization: Draw out the array and the movement of the pointers. This can help you understand how the algorithm works and spot edge cases.
- Handle Edge Cases: Always consider empty arrays, arrays with one element, or other special cases that might break your algorithm.
- Optimize Space Usage: Two-pointer techniques often allow for in-place modifications, which can be a significant advantage in space-constrained environments.
- Combine with Other Techniques: Two-pointer methods can often be combined with other algorithmic techniques like binary search or dynamic programming for more complex problems.
Common Pitfalls and How to Avoid Them
- Infinite Loops: Ensure that your pointers are always moving towards each other or in a way that guarantees termination.
- Off-by-One Errors: Be careful with your loop conditions and pointer increments/decrements, especially when dealing with array indices.
- Overlooking Duplicates: In problems where uniqueness matters, make sure to handle duplicate elements correctly.
- Unnecessary Sorting: While many two-pointer problems require sorted arrays, some don’t. Avoid the unnecessary O(n log n) cost of sorting if it’s not required.
- Ignoring Problem Constraints: Pay attention to any specific constraints mentioned in the problem statement, such as memory limitations or runtime requirements.
Conclusion
The two-pointer technique is a powerful tool in any programmer’s arsenal, especially when tackling array-based problems in coding interviews. By reducing time complexity and often allowing for in-place operations, this method can significantly optimize your solutions. As with any algorithmic technique, mastery comes with practice. Try implementing the examples provided here, and then challenge yourself with more complex problems that can benefit from the two-pointer approach.
Remember, the key to success in coding interviews is not just knowing the techniques but understanding when and how to apply them effectively. As you practice, focus on pattern recognition and problem-solving strategies. With time and dedication, you’ll find that the two-pointer technique becomes an intuitive part of your problem-solving toolkit, helping you tackle a wide range of array and string manipulation challenges with confidence and efficiency.