Learning to Recognize Patterns in Problems: Building a Mental Algorithm Library
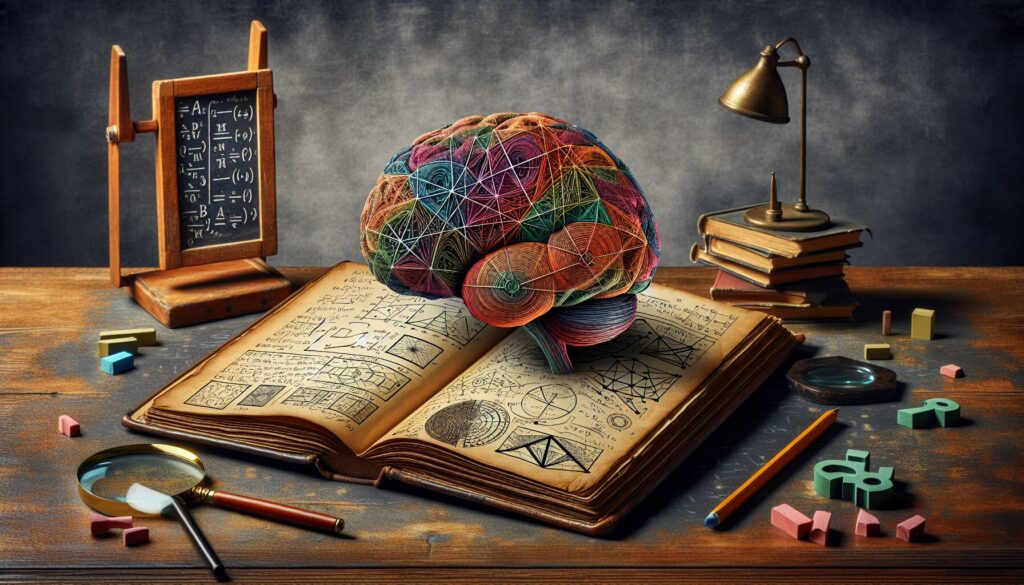
In the world of programming and computer science, the ability to recognize patterns in problems is a crucial skill that separates novice coders from seasoned professionals. This skill is not just about memorizing algorithms; it’s about developing a mental library of problem-solving techniques that can be applied to a wide range of challenges. In this comprehensive guide, we’ll explore the importance of pattern recognition in coding, strategies for building your mental algorithm library, and how this skill can significantly boost your performance in technical interviews and real-world programming tasks.
The Importance of Pattern Recognition in Coding
Pattern recognition is a fundamental cognitive skill that allows us to identify regularities, trends, and recurring themes in the problems we encounter. In the context of coding and algorithm design, this skill is invaluable for several reasons:
- Efficiency: Recognizing patterns allows you to quickly identify the most appropriate solution for a given problem, saving time and computational resources.
- Problem-Solving: It helps you break down complex problems into smaller, more manageable components that you’ve likely encountered before.
- Algorithmic Thinking: Pattern recognition fosters a deeper understanding of algorithmic principles, enabling you to design more elegant and optimized solutions.
- Interview Performance: Many technical interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), rely heavily on your ability to recognize and apply common algorithmic patterns.
Building Your Mental Algorithm Library
Developing a robust mental algorithm library is an ongoing process that requires dedication, practice, and a structured approach. Here are some strategies to help you build and expand your pattern recognition skills:
1. Study Classic Algorithms and Data Structures
Start by familiarizing yourself with fundamental algorithms and data structures. These form the building blocks of more complex solutions and are often the basis for recognizing patterns in new problems. Some essential topics to cover include:
- Sorting algorithms (e.g., Quicksort, Mergesort, Heapsort)
- Searching algorithms (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Data structures (e.g., Arrays, Linked Lists, Trees, Graphs, Hash Tables)
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
2. Practice Regularly with Diverse Problems
Consistent practice is key to developing pattern recognition skills. Solve a variety of problems from different sources to expose yourself to various problem types and difficulty levels. Some great resources include:
- LeetCode
- HackerRank
- CodeSignal
- Project Euler
- AlgoExpert
Aim to solve problems across different categories, such as arrays, strings, linked lists, trees, graphs, and dynamic programming. This diversity will help you recognize patterns across various problem domains.
3. Analyze and Categorize Problems
As you solve problems, make a habit of analyzing and categorizing them based on the underlying patterns and techniques used to solve them. Create a personal database or notebook where you can record:
- Problem description
- The pattern or technique used to solve it
- Key insights or tricks that led to the solution
- Similar problems you’ve encountered
This practice will help you build connections between problems and reinforce your understanding of common patterns.
4. Study Problem-Solving Techniques
Familiarize yourself with general problem-solving techniques that can be applied across various types of coding challenges. Some important techniques include:
- Two-pointer technique
- Sliding window
- Binary search variations
- Backtracking
- Topological sorting
- Union-find (Disjoint Set)
Understanding these techniques will help you recognize when they can be applied to new problems you encounter.
5. Implement Algorithms from Scratch
Don’t just study algorithms theoretically; implement them from scratch in your preferred programming language. This hands-on approach will deepen your understanding of how these algorithms work and make it easier to recognize when they can be applied to new problems.
Here’s an example of implementing a basic binary search algorithm in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
6. Participate in Coding Competitions
Engaging in coding competitions can expose you to a wide range of problem types and help you practice recognizing patterns under time pressure. Platforms like Codeforces, TopCoder, and Google’s Coding Competitions offer regular contests that can sharpen your skills.
7. Collaborate and Discuss with Others
Join coding communities, participate in forums, or form study groups to discuss problems and solutions with other programmers. Explaining your approach to others and hearing different perspectives can enhance your pattern recognition abilities and expose you to new ways of thinking about problems.
Common Problem Patterns to Recognize
As you build your mental algorithm library, there are several common patterns that you’ll encounter frequently. Recognizing these patterns can significantly speed up your problem-solving process. Here are some key patterns to be aware of:
1. Two Pointer Technique
This pattern is often used for problems involving arrays or linked lists. It involves using two pointers that move through the data structure in a specific way to solve the problem efficiently.
Example problem: Find a pair of numbers in a sorted array that sum up to a target value.
def two_sum(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
# Example usage
sorted_array = [2, 7, 11, 15]
target = 9
result = two_sum(sorted_array, target)
print(f"Pair that sums to {target}: {result}")
2. Sliding Window
This pattern is useful for problems that involve subarrays or substrings. It maintains a “window” of elements and slides it through the data structure, updating the result as it moves.
Example problem: Find the maximum sum of a subarray of size k in an array.
def max_subarray_sum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
array = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
result = max_subarray_sum(array, k)
print(f"Maximum sum of subarray of size {k}: {result}")
3. Fast and Slow Pointers
This pattern is often used for cycle detection in linked lists or arrays. It involves two pointers moving at different speeds through the data structure.
Example problem: Detect a cycle in a linked list.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Example usage
# Create a linked list with a cycle
head = ListNode(3)
head.next = ListNode(2)
head.next.next = ListNode(0)
head.next.next.next = ListNode(-4)
head.next.next.next.next = head.next # Create cycle
result = has_cycle(head)
print(f"Linked list has cycle: {result}")
4. Merge Intervals
This pattern is used for problems involving intervals or ranges. It typically involves sorting the intervals and then merging overlapping ones.
Example problem: Merge overlapping intervals in a list of intervals.
def merge_intervals(intervals):
if not intervals:
return []
# Sort intervals based on start time
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for interval in intervals[1:]:
if interval[0] <= merged[-1][1]:
merged[-1][1] = max(merged[-1][1], interval[1])
else:
merged.append(interval)
return merged
# Example usage
intervals = [[1,3],[2,6],[8,10],[15,18]]
result = merge_intervals(intervals)
print(f"Merged intervals: {result}")
5. Binary Search Variations
While the basic binary search is straightforward, recognizing when and how to apply binary search to more complex problems is a valuable skill.
Example problem: Find the smallest number in a rotated sorted array.
def find_minimum(nums):
left, right = 0, len(nums) - 1
while left < right:
mid = (left + right) // 2
if nums[mid] > nums[right]:
left = mid + 1
else:
right = mid
return nums[left]
# Example usage
rotated_array = [4, 5, 6, 7, 0, 1, 2]
result = find_minimum(rotated_array)
print(f"Minimum element in rotated array: {result}")
6. Depth-First Search (DFS) and Breadth-First Search (BFS)
These fundamental graph traversal algorithms are applicable to a wide range of problems involving trees, graphs, and even matrices.
Example problem: Implement DFS to find if a path exists between two nodes in a graph.
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def dfs_util(self, v, visited, destination):
visited.add(v)
if v == destination:
return True
for neighbor in self.graph[v]:
if neighbor not in visited:
if self.dfs_util(neighbor, visited, destination):
return True
return False
def has_path(self, start, end):
visited = set()
return self.dfs_util(start, visited, end)
# Example usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
start, end = 1, 3
result = g.has_path(start, end)
print(f"Path exists from {start} to {end}: {result}")
Applying Pattern Recognition in Technical Interviews
When facing a problem in a technical interview, especially at major tech companies like FAANG, the ability to quickly recognize patterns can give you a significant advantage. Here’s a step-by-step approach to apply your pattern recognition skills:
- Listen carefully and ask clarifying questions: Ensure you fully understand the problem before jumping to a solution.
- Identify the problem type: Is it a string manipulation problem? A graph problem? An optimization problem?
- Look for familiar elements: Are there sorted arrays involved? Is there a need to find cycles? Are you dealing with intervals?
- Consider common patterns: Based on the problem type and familiar elements, think about which patterns might be applicable (e.g., two pointers, sliding window, DFS/BFS).
- Propose a high-level approach: Explain your thought process to the interviewer, mentioning the pattern you’ve recognized and why you think it’s applicable.
- Refine and implement: Work with the interviewer to refine your approach and then implement the solution.
- Analyze and optimize: Discuss the time and space complexity of your solution and consider if there are any optimizations you can make.
Continuous Improvement and Learning
Building a mental algorithm library and honing your pattern recognition skills is an ongoing process. Here are some tips for continuous improvement:
- Review and reflect: After solving a problem, take time to reflect on the patterns you used and how they might apply to other problems.
- Study multiple solutions: For each problem you solve, look at different approaches and understand the trade-offs between them.
- Read code: Study well-written code and algorithms implemented by experienced developers to learn new patterns and coding styles.
- Teach others: Explaining concepts and patterns to others can deepen your own understanding and reveal gaps in your knowledge.
- Stay updated: Keep up with new algorithmic techniques and problem-solving strategies by following relevant blogs, research papers, and coding competitions.
Conclusion
Learning to recognize patterns in coding problems is a powerful skill that can significantly enhance your problem-solving abilities and performance in technical interviews. By building a comprehensive mental algorithm library, you’ll be better equipped to tackle a wide range of coding challenges efficiently and elegantly.
Remember that developing this skill takes time and consistent practice. Don’t get discouraged if you don’t immediately recognize patterns in every problem you encounter. With persistence and dedication, you’ll gradually build a robust set of problem-solving tools that will serve you well throughout your programming career.
As you continue your journey in coding education and skills development, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to help you refine your pattern recognition skills and prepare for technical interviews at top tech companies. Embrace the learning process, stay curious, and keep challenging yourself with diverse problems to expand your mental algorithm library and become a more effective problem solver.