Learning to Handle Uncertainty and Ambiguity in Code
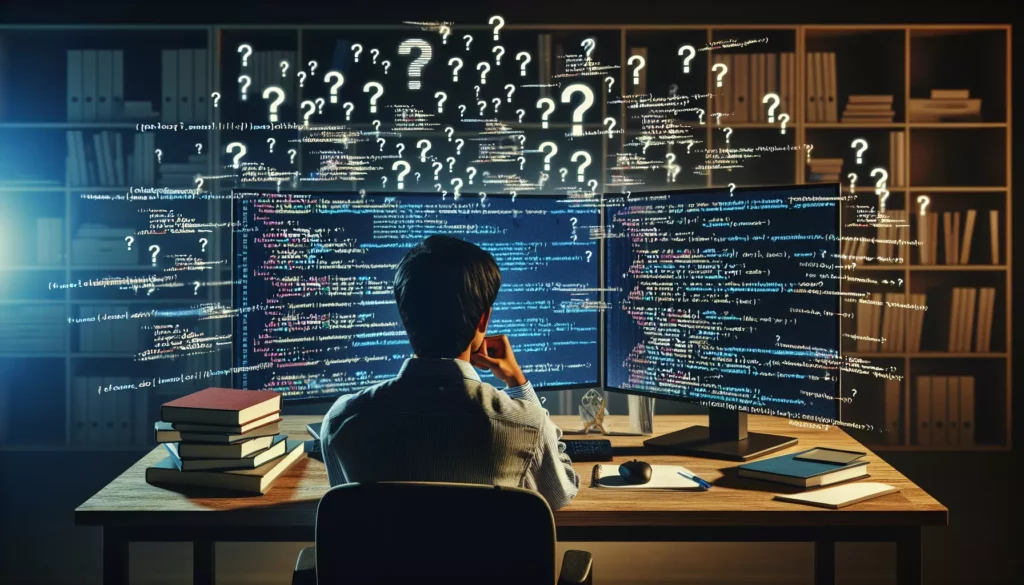
In the world of programming, uncertainty and ambiguity are constant companions. As developers, we often encounter situations where the requirements are unclear, the problem is not well-defined, or the solution is not immediately apparent. Learning to navigate these murky waters is a crucial skill that separates novice programmers from seasoned professionals. In this comprehensive guide, we’ll explore strategies and techniques to help you handle uncertainty and ambiguity in your coding journey, from beginner-level challenges to advanced problem-solving scenarios.
Understanding Uncertainty and Ambiguity in Programming
Before we dive into strategies for dealing with uncertainty and ambiguity, let’s first define what these terms mean in the context of programming:
- Uncertainty: This refers to situations where you’re not sure about the correct approach or solution to a problem. You might have multiple options but lack confidence in choosing the best one.
- Ambiguity: This occurs when the problem statement or requirements are not clearly defined, leaving room for multiple interpretations.
Both uncertainty and ambiguity can lead to challenges in writing effective code, designing robust systems, and delivering successful projects. However, they’re also opportunities for growth and innovation when approached with the right mindset and tools.
Strategies for Handling Uncertainty
1. Break Down Complex Problems
When faced with a complex problem that seems overwhelming, break it down into smaller, more manageable parts. This approach, often called “divide and conquer,” can help reduce uncertainty by allowing you to focus on solving one piece of the puzzle at a time.
For example, if you’re tasked with building a web application, you might break it down into these components:
- User authentication system
- Database design and implementation
- Frontend user interface
- API endpoints
- Integration with third-party services
By tackling each component separately, you can reduce the overall complexity and uncertainty of the project.
2. Use Pseudocode and Flowcharts
Before diving into writing actual code, use pseudocode or flowcharts to outline your approach. This can help you visualize the logic and structure of your solution without getting bogged down in syntax details.
Here’s an example of pseudocode for a simple sorting algorithm:
function sortArray(arr):
for i from 0 to length(arr) - 1:
for j from 0 to length(arr) - i - 1:
if arr[j] > arr[j+1]:
swap arr[j] and arr[j+1]
return arr
This high-level representation can help you clarify your thoughts and identify potential issues before you start coding.
3. Implement Prototype Solutions
When you’re unsure about which approach to take, try implementing multiple prototype solutions. This allows you to experiment with different ideas and compare their effectiveness before committing to a final implementation.
For instance, if you’re working on optimizing a search algorithm, you might implement both a binary search and a hash table-based solution to compare their performance:
// Binary Search Implementation
function binarySearch(arr, target):
left = 0
right = length(arr) - 1
while left <= right:
mid = (left + right) / 2
if arr[mid] == target:
return mid
else if arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
// Hash Table Implementation
function hashTableSearch(arr, target):
hashTable = {}
for i from 0 to length(arr) - 1:
hashTable[arr[i]] = i
if target in hashTable:
return hashTable[target]
else:
return -1
By implementing both solutions, you can test them with various inputs and determine which one performs better for your specific use case.
4. Leverage Version Control
When dealing with uncertainty, it’s crucial to have a safety net that allows you to experiment freely without fear of breaking your codebase. Version control systems like Git provide this safety net by allowing you to create branches, commit changes incrementally, and easily revert to previous states if needed.
Here’s a basic Git workflow that can help you manage uncertainty:
// Create a new branch for experimenting
git checkout -b experimental-feature
// Make changes and commit them
git add .
git commit -m "Implement experimental feature"
// If the changes work well, merge them into the main branch
git checkout main
git merge experimental-feature
// If the changes don't work out, simply delete the branch
git branch -D experimental-feature
This approach allows you to explore different solutions without risking the stability of your main codebase.
Techniques for Dealing with Ambiguity
1. Ask Clarifying Questions
When faced with ambiguous requirements or specifications, don’t hesitate to ask clarifying questions. This is especially important in professional settings where miscommunication can lead to wasted time and resources.
Some examples of clarifying questions include:
- “Can you provide an example of the expected input and output for this function?”
- “What should happen if the user enters invalid data?”
- “Are there any performance requirements or constraints we need to consider?”
- “How should the system behave in edge cases, such as when the database is unavailable?”
By asking these questions, you can reduce ambiguity and gain a clearer understanding of the problem you’re trying to solve.
2. Document Assumptions and Decisions
When working with ambiguous requirements, it’s important to document your assumptions and decisions. This not only helps you keep track of your thought process but also provides a reference for future discussions or revisions.
Consider creating a document or comments in your code that outline:
- Assumptions made about the problem or requirements
- Decisions made regarding implementation details
- Rationale behind those decisions
- Potential areas of concern or future improvements
Here’s an example of how you might document assumptions in your code:
/**
* Processes user input for a search query.
*
* Assumptions:
* - Input is always a string
* - Empty strings are treated as valid inputs
* - Maximum query length is 100 characters
*
* @param {string} query - The user's search query
* @returns {string} - Processed query ready for search
*/
function processSearchQuery(query) {
// Implementation details...
}
This documentation helps clarify your understanding of the problem and can serve as a starting point for discussions with team members or stakeholders.
3. Use Flexible and Extensible Design Patterns
When dealing with ambiguous requirements that may change in the future, it’s wise to use flexible and extensible design patterns. These patterns allow you to create code that can adapt to changing needs without requiring a complete rewrite.
Some useful design patterns for handling ambiguity include:
- Strategy Pattern: Allows you to define a family of algorithms, encapsulate each one, and make them interchangeable.
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- Factory Method Pattern: Provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
Here’s an example of the Strategy Pattern in JavaScript:
// Define strategies
const strategies = {
add: (a, b) => a + b,
subtract: (a, b) => a - b,
multiply: (a, b) => a * b,
divide: (a, b) => a / b
};
// Context class
class Calculator {
constructor(strategy) {
this.strategy = strategy;
}
setStrategy(strategy) {
this.strategy = strategy;
}
calculate(a, b) {
return this.strategy(a, b);
}
}
// Usage
const calculator = new Calculator(strategies.add);
console.log(calculator.calculate(5, 3)); // Output: 8
calculator.setStrategy(strategies.multiply);
console.log(calculator.calculate(5, 3)); // Output: 15
This pattern allows you to easily add new operations or change the behavior of the calculator without modifying the existing code, making it more adaptable to ambiguous or changing requirements.
4. Implement Feature Flags
Feature flags (also known as feature toggles) are a powerful technique for managing ambiguity in software development. They allow you to enable or disable features at runtime, which can be particularly useful when requirements are unclear or subject to change.
Here’s a simple example of how you might implement feature flags in Python:
class FeatureFlags:
def __init__(self):
self.flags = {
"new_user_interface": False,
"advanced_search": True,
"beta_feature": False
}
def is_enabled(self, feature_name):
return self.flags.get(feature_name, False)
# Usage
feature_flags = FeatureFlags()
if feature_flags.is_enabled("new_user_interface"):
# Implement new UI
pass
else:
# Use old UI
pass
if feature_flags.is_enabled("advanced_search"):
# Implement advanced search functionality
pass
else:
# Use basic search
pass
This approach allows you to develop and deploy features incrementally, test different variations, and easily roll back changes if needed, all of which can help manage ambiguity in the development process.
Advanced Techniques for Seasoned Developers
1. Embrace Test-Driven Development (TDD)
Test-Driven Development is a powerful approach for dealing with both uncertainty and ambiguity. By writing tests before implementing the actual code, you force yourself to clarify requirements and consider various scenarios upfront.
The TDD cycle typically follows these steps:
- Write a failing test that defines a desired improvement or new function
- Write the minimum amount of code to make the test pass
- Refactor the code to meet quality standards
Here’s an example of TDD in Python using the pytest framework:
import pytest
# Step 1: Write a failing test
def test_add_numbers():
assert add_numbers(2, 3) == 5
assert add_numbers(-1, 1) == 0
assert add_numbers(0, 0) == 0
# Step 2: Write the minimum code to make the test pass
def add_numbers(a, b):
return a + b
# Step 3: Refactor if necessary
# In this case, no refactoring is needed as the function is simple
# Run the test
pytest.main([__file__])
By following TDD, you can incrementally build your understanding of the problem and solution, reducing uncertainty and ambiguity along the way.
2. Utilize Domain-Driven Design (DDD)
Domain-Driven Design is an approach to software development that focuses on creating a shared understanding of the problem domain between developers and domain experts. This can be particularly useful when dealing with complex or ambiguous business requirements.
Key concepts in DDD include:
- Ubiquitous Language: A common, precise language used by both developers and domain experts.
- Bounded Contexts: Clearly defined boundaries between different parts of the domain model.
- Entities and Value Objects: Distinguishing between objects with identity and those defined by their attributes.
- Aggregates: Clusters of domain objects that can be treated as a single unit.
Here’s a simplified example of how you might structure code using DDD principles:
// Bounded Context: Order Management
// Entity
class Order {
constructor(id, customerId, items) {
this.id = id;
this.customerId = customerId;
this.items = items;
this.status = "pending";
}
addItem(item) {
this.items.push(item);
}
removeItem(itemId) {
this.items = this.items.filter(item => item.id !== itemId);
}
submit() {
// Business logic for submitting an order
this.status = "submitted";
}
}
// Value Object
class OrderItem {
constructor(id, productId, quantity, price) {
this.id = id;
this.productId = productId;
this.quantity = quantity;
this.price = price;
}
get total() {
return this.quantity * this.price;
}
}
// Aggregate Root
class OrderAggregate {
constructor(order) {
this.order = order;
}
submitOrder() {
// Additional business logic, e.g., validation
if (this.order.items.length === 0) {
throw new Error("Cannot submit an empty order");
}
this.order.submit();
}
}
// Usage
const order = new Order("ORD-001", "CUST-001", []);
order.addItem(new OrderItem("ITEM-001", "PROD-001", 2, 10.99));
order.addItem(new OrderItem("ITEM-002", "PROD-002", 1, 24.99));
const orderAggregate = new OrderAggregate(order);
orderAggregate.submitOrder();
By using DDD, you can create a more precise and shared understanding of the problem domain, which helps reduce ambiguity and uncertainty in the development process.
3. Implement Continuous Integration and Continuous Deployment (CI/CD)
CI/CD practices can help manage uncertainty and ambiguity by providing rapid feedback and enabling frequent, small releases. This approach allows you to validate assumptions quickly and adapt to changing requirements more easily.
Key components of a CI/CD pipeline include:
- Automated testing (unit tests, integration tests, end-to-end tests)
- Code quality checks (linting, static analysis)
- Automated builds
- Deployment to staging and production environments
Here’s an example of a simple CI/CD configuration using GitHub Actions:
name: CI/CD Pipeline
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm ci
- name: Run linter
run: npm run lint
- name: Run tests
run: npm test
- name: Build
run: npm run build
deploy:
needs: build-and-test
runs-on: ubuntu-latest
if: github.ref == 'refs/heads/main'
steps:
- uses: actions/checkout@v2
- name: Deploy to production
run: |
# Add your deployment script here
echo "Deploying to production..."
By implementing CI/CD, you can reduce the risk associated with uncertain or ambiguous requirements by validating changes quickly and frequently.
Conclusion
Handling uncertainty and ambiguity in code is a crucial skill for developers at all levels. By employing strategies such as breaking down problems, using pseudocode, implementing prototypes, and leveraging version control, you can navigate uncertain situations more effectively. For dealing with ambiguity, techniques like asking clarifying questions, documenting assumptions, using flexible design patterns, and implementing feature flags can help you create more robust and adaptable code.
As you progress in your development career, advanced techniques like Test-Driven Development, Domain-Driven Design, and CI/CD practices can further enhance your ability to handle complex, uncertain, and ambiguous scenarios.
Remember that dealing with uncertainty and ambiguity is an ongoing process. As you gain experience, you’ll develop a toolkit of strategies and techniques that work best for you and your projects. Embrace these challenges as opportunities for growth and learning, and you’ll become a more effective and confident developer in the face of uncertainty and ambiguity.