Learning to Code Through Failure: Embracing Bugs as Your Best Teacher
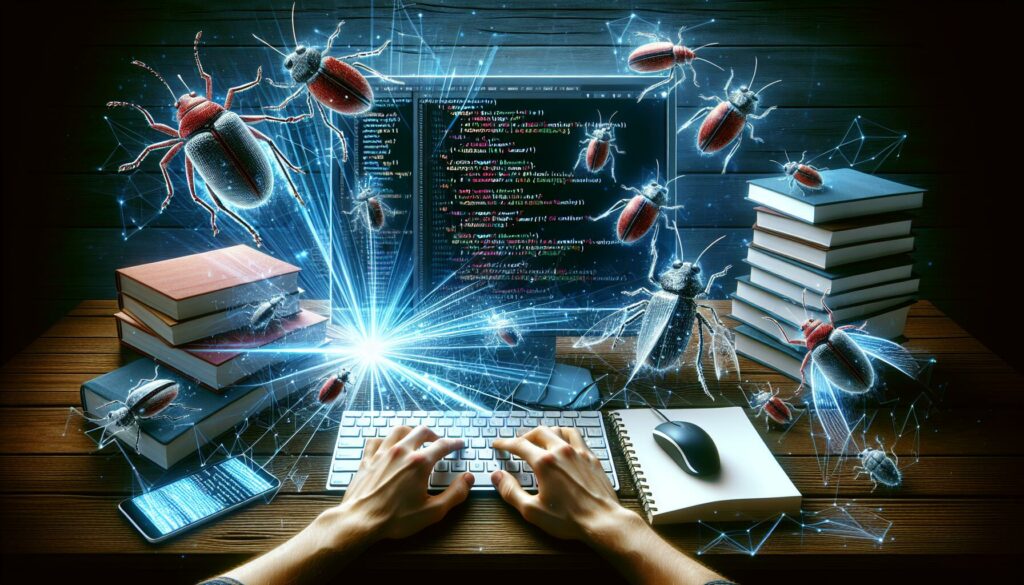
In the world of coding and software development, encountering bugs and errors is an inevitable part of the journey. While these roadblocks can be frustrating, they are also invaluable learning opportunities. At AlgoCademy, we believe that embracing failure and learning from bugs is a crucial aspect of becoming a proficient programmer. In this comprehensive guide, we’ll explore why bugs are your best teachers and how you can leverage them to enhance your coding skills.
The Importance of Bugs in the Learning Process
Bugs and errors are often seen as obstacles, but they play a vital role in the learning process for several reasons:
- They provide hands-on problem-solving experience
- They deepen your understanding of programming concepts
- They improve your debugging skills
- They teach you patience and persistence
- They prepare you for real-world programming challenges
Let’s dive deeper into each of these aspects and explore how you can make the most of your encounters with bugs.
Hands-on Problem-Solving Experience
When you encounter a bug in your code, you’re faced with a real-world problem that needs solving. This presents an opportunity to apply your knowledge and critical thinking skills in a practical context. Unlike theoretical exercises, debugging requires you to:
- Identify the problem
- Analyze the code and its behavior
- Formulate hypotheses about the cause
- Test different solutions
- Implement and verify the fix
This process not only reinforces your existing knowledge but also helps you develop problem-solving strategies that you can apply to future coding challenges.
Example: Debugging a Simple Function
Let’s consider a simple example of a function that’s supposed to calculate the average of an array of numbers:
function calculateAverage(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
console.log(calculateAverage([1, 2, 3, 4, 5])); // Expected output: 3
console.log(calculateAverage([])); // Expected output: NaN
This function works correctly for non-empty arrays, but it returns NaN (Not a Number) for empty arrays. To fix this, you might modify the function like this:
function calculateAverage(numbers) {
if (numbers.length === 0) {
return 0; // or throw an error, depending on requirements
}
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
By encountering and solving this bug, you’ve learned about edge cases and how to handle them, improving your overall coding skills.
Deepening Your Understanding of Programming Concepts
Bugs often arise from misunderstandings or oversights related to fundamental programming concepts. When you encounter and resolve these issues, you gain a deeper, more nuanced understanding of these concepts. This process can help clarify:
- Language-specific syntax and behavior
- Data types and type coercion
- Scope and variable lifetime
- Asynchronous programming concepts
- Memory management and garbage collection
Example: Understanding Scope
Consider the following code snippet:
function outer() {
let x = 10;
function inner() {
console.log(x);
let x = 20;
}
inner();
}
outer(); // Throws a ReferenceError: Cannot access 'x' before initialization
This code throws a ReferenceError, which might be surprising at first. By debugging this issue, you’ll learn about the temporal dead zone and how variable hoisting works in JavaScript, deepening your understanding of scope and variable declaration.
Improving Your Debugging Skills
The more bugs you encounter and resolve, the better you become at debugging. This skill is crucial for any programmer, as it involves:
- Reading and understanding error messages
- Using debugging tools effectively
- Tracing code execution
- Isolating problems
- Implementing and testing fixes
At AlgoCademy, we provide interactive debugging tools and step-by-step guidance to help you develop these skills systematically.
Tips for Effective Debugging
- Read the error message carefully: Error messages often provide valuable information about what went wrong and where.
- Use console.log() strategically: Print out variable values at different points in your code to trace its execution.
- Utilize breakpoints: Set breakpoints in your code to pause execution and examine the state of your program at specific points.
- Isolate the problem: Try to reproduce the bug with the smallest possible code snippet.
- Use a debugger: Learn to use your IDE’s debugging tools to step through your code line by line.
Teaching Patience and Persistence
Debugging can be a time-consuming and sometimes frustrating process. However, it teaches valuable soft skills that are essential for any programmer:
- Patience: Some bugs can take hours or even days to resolve. Learning to stay calm and methodical is crucial.
- Persistence: The ability to keep trying different approaches and not give up is a hallmark of successful programmers.
- Attention to detail: Many bugs are caused by small oversights. Developing a keen eye for detail can help prevent and resolve issues more quickly.
- Resilience: Learning to bounce back from setbacks and view them as learning opportunities is essential for long-term success in programming.
Strategies for Maintaining Patience and Persistence
- Take breaks: If you’re stuck on a problem, step away for a while. A fresh perspective can often lead to breakthroughs.
- Break the problem down: Divide complex issues into smaller, more manageable parts.
- Seek help: Don’t hesitate to ask for help from peers, mentors, or online communities. Sometimes, explaining your problem to others can help you see it in a new light.
- Celebrate small wins: Acknowledge your progress, no matter how small. This can help maintain motivation during challenging debugging sessions.
Preparing for Real-World Programming Challenges
In professional software development, a significant portion of time is spent maintaining and debugging existing code. By embracing bugs as learning opportunities, you’re preparing yourself for the realities of professional programming. This includes:
- Working with legacy code
- Collaborating with other developers
- Handling production issues and hotfixes
- Optimizing code performance
- Ensuring code security
Example: Debugging in a Team Environment
Imagine you’re working on a team project, and you encounter a bug in a function written by a colleague:
function calculateTotalPrice(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price * items[i].quantity;
}
return total.toFixed(2);
}
The function seems to work correctly, but occasionally it returns incorrect results. After investigation, you discover that some item prices are stored as strings instead of numbers. To fix this, you might modify the function like this:
function calculateTotalPrice(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += parseFloat(items[i].price) * items[i].quantity;
}
return total.toFixed(2);
}
This experience teaches you about type coercion, the importance of data validation, and how to collaborate effectively with team members to resolve issues.
Leveraging AlgoCademy’s Resources for Bug-Driven Learning
At AlgoCademy, we’ve designed our platform to support and enhance your learning through bugs and errors. Here’s how you can make the most of our resources:
Interactive Coding Challenges
Our coding challenges are designed to expose you to common bugs and edge cases. As you work through these challenges, pay attention to the errors you encounter and use them as opportunities to deepen your understanding.
AI-Powered Assistance
Our AI assistant can help guide you through the debugging process, offering hints and explanations tailored to your specific errors. Use this feature to gain insights into the root causes of bugs and learn best practices for avoiding similar issues in the future.
Step-by-Step Tutorials
Our tutorials often include intentional bugs or common mistakes. By working through these examples, you’ll learn to identify and fix issues in a structured, educational environment.
Community Forums
Engage with other learners in our community forums. Discussing bugs and solutions with peers can provide new perspectives and reinforce your understanding of complex concepts.
Conclusion: Embracing the Learning Potential of Bugs
While encountering bugs and errors can be frustrating, they are an integral part of the learning process for any programmer. By embracing these challenges and viewing them as opportunities for growth, you can accelerate your learning and become a more proficient coder.
Remember that every bug you encounter and resolve is adding to your skillset and preparing you for real-world programming challenges. At AlgoCademy, we’re committed to supporting you through this journey, providing the tools, resources, and guidance you need to turn every bug into a valuable learning experience.
So the next time you face a perplexing error or an unexpected bug, take a deep breath and remind yourself: this is not just a problem to be solved, but an opportunity to become a better programmer. Happy coding, and may your bugs be ever instructive!