Learning to Code: Python vs Java – Which is Easier for Beginners?
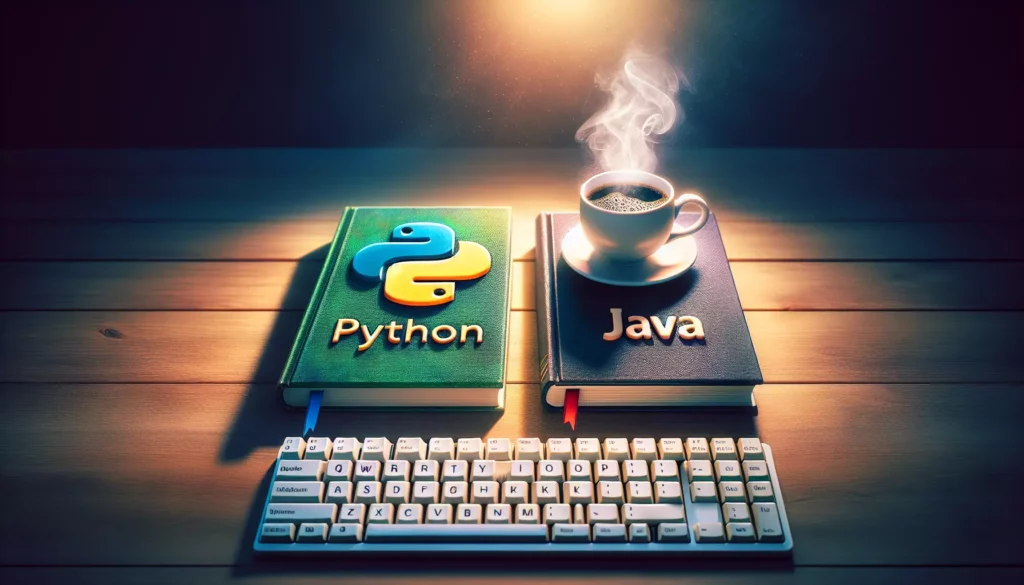
In the ever-evolving world of programming, choosing the right language to start your coding journey can be a daunting task. Two popular contenders that often come up in this discussion are Python and Java. Both are widely used in various domains, from web development to data science, but they have distinct characteristics that can make one more suitable than the other for beginners. In this comprehensive guide, we’ll dive deep into the comparison between Python and Java, focusing on their ease of learning for newcomers to the programming world.
Understanding the Basics: Python and Java at a Glance
Before we delve into the specifics of learning each language, let’s briefly introduce Python and Java:
Python: The Friendly Giant
Python is often touted as one of the most beginner-friendly programming languages. Created by Guido van Rossum and first released in 1991, Python has gained immense popularity due to its simplicity and readability. It’s an interpreted, high-level language known for its clean syntax and extensive standard library.
Java: The Enterprise Workhorse
Java, developed by James Gosling at Sun Microsystems and released in 1995, is a class-based, object-oriented programming language designed to be “write once, run anywhere” (WORA). It’s known for its robustness, security, and platform independence, making it a favorite in enterprise environments.
Syntax Simplicity: The First Hurdle for Beginners
When it comes to ease of learning, syntax plays a crucial role. Let’s compare how Python and Java handle basic programming concepts:
Python’s Intuitive Syntax
Python is renowned for its clean and readable syntax. It uses indentation to define code blocks, which enforces a consistent and visually appealing structure. Here’s a simple “Hello, World!” program in Python:
print("Hello, World!")
As you can see, it’s straightforward and almost reads like plain English. This simplicity extends to more complex structures as well. For example, here’s a simple function in Python:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Java’s Structured Approach
Java, on the other hand, has a more verbose syntax. Here’s the equivalent “Hello, World!” program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
As you can see, Java requires more boilerplate code. Even for this simple program, you need to define a class and a main method. Here’s a similar greeting function in Java:
public class Greeter {
public static String greet(String name) {
return "Hello, " + name + "!";
}
public static void main(String[] args) {
System.out.println(greet("Alice"));
}
}
While Java’s syntax is more verbose, it does provide a clear structure that can be beneficial for larger projects. However, for beginners, this additional complexity can be a stumbling block.
Learning Curve: Gradual vs. Steep
The learning curve is another crucial factor to consider when choosing between Python and Java. Let’s examine how each language fares in this aspect:
Python’s Gentle Slope
Python is often described as having a gentle learning curve. This is due to several factors:
- Readability: Python’s syntax is designed to be easily readable, which helps beginners understand code more quickly.
- Less Boilerplate: Python requires minimal setup code, allowing beginners to focus on core programming concepts.
- Dynamic Typing: Python uses dynamic typing, which means you don’t need to declare variable types explicitly. This can be easier for beginners to grasp initially.
- Interactive Shell: Python comes with an interactive shell (REPL – Read-Eval-Print Loop) that allows for immediate code execution and experimentation.
These features combine to create an environment where beginners can start writing meaningful code quickly, which can be highly motivating.
Java’s Steeper Climb
Java, while powerful and widely used, presents a steeper learning curve for beginners:
- Verbose Syntax: Java requires more code to accomplish simple tasks, which can be overwhelming for newcomers.
- Static Typing: Java uses static typing, requiring explicit declaration of variable types. While this prevents certain types of errors, it adds complexity for beginners.
- Object-Oriented Programming (OOP) Focus: Java is heavily focused on OOP concepts from the start, which can be challenging for those new to programming.
- Compilation Process: Unlike Python, Java code needs to be compiled before it can be run, adding an extra step to the coding process.
These characteristics make Java more challenging to pick up initially, but they also provide a solid foundation for understanding important programming concepts.
Development Environment: Getting Started
The ease of setting up a development environment can significantly impact a beginner’s experience. Let’s compare Python and Java in this aspect:
Python: Quick and Easy Setup
Setting up Python is generally straightforward:
- Download Python from the official website.
- Run the installer (on Windows) or use package managers (on macOS or Linux).
- Python comes with its own IDE (IDLE) for simple projects.
- For more advanced development, popular IDEs like PyCharm or VS Code are easy to set up.
Once installed, you can start coding immediately using the Python interpreter or by creating .py files.
Java: More Steps Involved
Setting up Java requires a few more steps:
- Download and install the Java Development Kit (JDK).
- Set up environment variables (on Windows).
- Choose and install an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA.
- Create a new project in the IDE to start coding.
While not overly complex, this process involves more steps and potential for configuration issues, which can be frustrating for beginners.
Community and Resources: Learning Support
The availability of learning resources and community support can greatly influence how easy it is to learn a programming language. Both Python and Java have strong communities, but there are some differences:
Python’s Vibrant Community
Python boasts a large and active community known for being welcoming to beginners. This translates to:
- Extensive documentation and tutorials tailored for different skill levels.
- A wealth of online courses and interactive learning platforms.
- Active forums and Q&A sites like Stack Overflow with quick response times.
- Numerous libraries and frameworks with good documentation.
The Python community’s focus on readability and simplicity extends to its documentation and learning resources, making it easier for beginners to find help and solutions.
Java’s Established Ecosystem
Java also has a robust community and resource base:
- Comprehensive official documentation from Oracle.
- A wide range of books and online courses, many geared towards professional development.
- Strong presence on developer forums and Q&A sites.
- Extensive libraries and frameworks, although documentation can sometimes be more technical.
While Java’s resources are plentiful, they can sometimes be more oriented towards professional developers, which might be challenging for absolute beginners.
Practical Applications: Motivating Factors
Being able to create practical projects early in the learning process can be a significant motivator. Let’s see how Python and Java fare in this aspect:
Python: Quick Results
Python allows beginners to create useful programs relatively quickly:
- Web scraping scripts
- Simple data analysis projects
- Basic games (e.g., text-based adventures)
- Automation scripts for everyday tasks
The ability to see tangible results early on can be highly motivating for new programmers. For example, here’s a simple Python script that fetches and prints the current weather:
import requests
def get_weather(city):
api_key = "your_api_key_here"
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {"q": city, "appid": api_key, "units": "metric"}
response = requests.get(base_url, params=params)
data = response.json()
if response.status_code == 200:
temp = data["main"]["temp"]
description = data["weather"][0]["description"]
return f"The weather in {city} is {description} with a temperature of {temp}°C."
else:
return "Unable to fetch weather data."
print(get_weather("London"))
Java: Building Foundations
Java projects often require more setup and code, but they provide a solid foundation for larger applications:
- Desktop applications with graphical user interfaces
- Android mobile apps
- Server-side web applications
- Enterprise software systems
While these projects might take longer to get off the ground, they often align more closely with professional software development practices. Here’s a simple Java program that creates a basic GUI window:
import javax.swing.*;
public class SimpleGUI {
public static void main(String[] args) {
JFrame frame = new JFrame("Simple GUI");
JButton button = new JButton("Click Me!");
button.addActionListener(e -> JOptionPane.showMessageDialog(frame, "Hello, World!"));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(button);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Career Prospects: Looking Ahead
While immediate learnability is important, it’s also worth considering the long-term career prospects associated with each language:
Python: Versatility and Growth
Python’s career prospects are excellent:
- High demand in data science, machine learning, and AI
- Popular for web development (especially backend)
- Widely used in scientific computing and research
- Growing adoption in fields like finance and healthcare
Python’s versatility means that learning it opens doors to various career paths, which can be appealing for those unsure about their specific direction in tech.
Java: Stability and Enterprise Focus
Java offers strong career prospects, particularly in certain sectors:
- Dominant in enterprise software development
- Essential for Android mobile app development
- Widely used in financial services and large-scale systems
- Strong presence in backend web development
Java’s long-standing presence in the industry means it’s likely to remain relevant for years to come, providing job stability for those who master it.
The Verdict: Which is Easier to Learn?
After considering various factors, we can conclude that Python is generally easier to learn for beginners compared to Java. Here’s a summary of the key points:
- Syntax: Python’s clean and readable syntax is more accessible for newcomers.
- Learning Curve: Python offers a gentler learning curve with fewer concepts to grasp initially.
- Development Environment: Setting up Python is typically quicker and involves fewer steps.
- Community and Resources: While both have strong communities, Python’s resources are often more beginner-friendly.
- Practical Applications: Python allows for quicker development of simple, practical projects, which can be motivating for beginners.
However, it’s important to note that “easier” doesn’t necessarily mean “better” for everyone. Java’s complexity comes with benefits:
- It provides a strong foundation in programming concepts, especially OOP.
- The skills learned are directly applicable to many professional development environments.
- It can lead to a deeper understanding of programming principles in the long run.
Making Your Choice: Factors to Consider
When deciding between Python and Java, consider the following:
- Your Goals: If you want to get into data science or AI, Python might be the better choice. For enterprise or Android development, Java could be more suitable.
- Learning Style: If you prefer a more gradual learning curve, Python might suit you better. If you enjoy diving deep into concepts from the start, Java could be rewarding.
- Time Commitment: If you’re looking to create simple projects quickly, Python allows for faster progress. Java might require more time investment initially but can pay off in complex project development.
- Career Aspirations: Research the job market in your area or desired field to see which language is more in demand.
Conclusion: The Path Forward
In the debate of Python vs Java for beginners, Python emerges as the easier language to learn due to its simplicity, readability, and gentle learning curve. However, Java’s complexity offers its own advantages, particularly for those aiming for a career in enterprise software development or Android app creation.
Ultimately, the “easier” language to learn is the one that aligns best with your goals, learning style, and career aspirations. Both Python and Java are powerful languages with bright futures in the tech industry. Whichever you choose, remember that learning to code is a journey, and the most important factor is your dedication and practice.
If you’re still unsure, consider starting with Python to get a feel for programming concepts, and then explore Java later if your interests or career path require it. Many programmers find that learning multiple languages broadens their understanding and makes them more versatile developers.
Platforms like AlgoCademy can be invaluable resources in your coding journey, offering interactive tutorials, AI-powered assistance, and a structured path from beginner-level coding to advanced algorithmic thinking. Whether you choose Python, Java, or both, remember that consistent practice and hands-on project work are key to mastering programming skills.
Happy coding, and may your programming journey be rewarding, whichever language you choose to start with!