Learning to Code is Like Learning to Walk: It’s Messy Before It’s Mastered
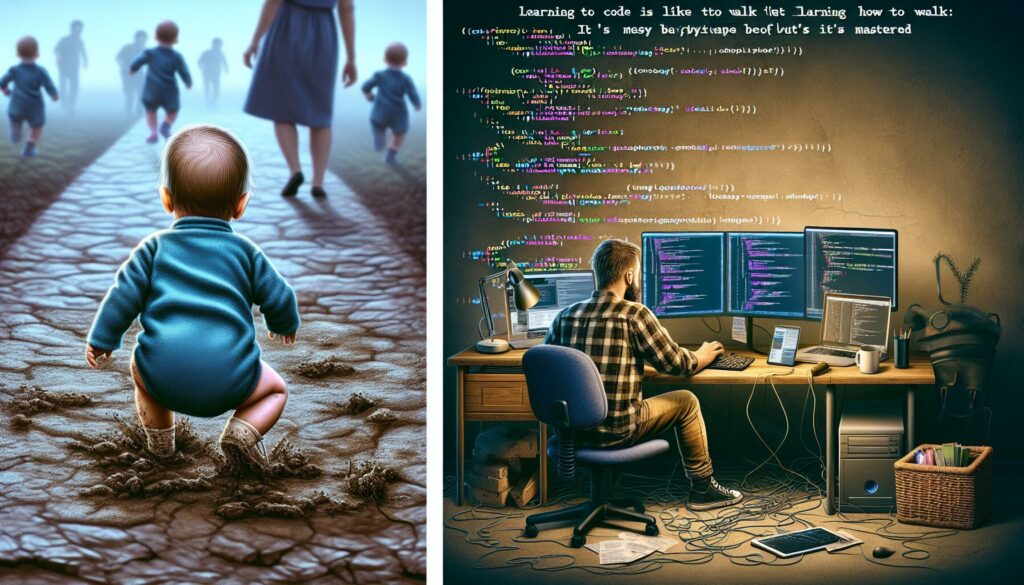
When you first start learning to code, it can feel like you’re a toddler taking your first steps. You’re wobbly, unsure, and prone to falling flat on your face. But just like learning to walk, mastering coding is a journey filled with stumbles, triumphs, and gradual improvement. In this post, we’ll explore why the process of learning to code is so similar to learning to walk, and how embracing the messiness can lead to true mastery.
The First Steps: Crawling Through Syntax
Just as babies start by crawling before they walk, new coders begin by grappling with the basics of syntax. You’re learning the alphabet of your chosen programming language, figuring out how to string together simple commands, and often feeling like you’re moving at a snail’s pace.
At AlgoCademy, we understand this phase intimately. Our interactive tutorials are designed to guide you through these early stages, providing a safe environment to experiment and make mistakes. Remember, every expert coder once struggled to print “Hello, World!” to the console.
Example: Your First Lines of Code
Let’s look at a simple Python example that might be one of your first coding experiences:
print("Hello, World!")
name = input("What's your name? ")
print("Nice to meet you, " + name + "!")
This basic interaction might seem trivial to experienced programmers, but for beginners, it’s a significant milestone. It’s your first step into the world of programming, akin to a baby’s first unsteady stand.
Stumbling and Getting Back Up: Debugging Your First Errors
As you progress from crawling to walking in your coding journey, you’ll inevitably encounter errors and bugs. This is where many beginners get frustrated and consider giving up. But remember, every error is an opportunity to learn and improve.
At AlgoCademy, we emphasize the importance of debugging skills from the very beginning. Our AI-powered assistance can help you understand and fix errors, much like a parent’s guiding hand steadying a toddler’s wobbly steps.
Common Beginner Errors and How to Fix Them
Let’s look at some common errors you might encounter and how to approach them:
-
Syntax Errors: These are like stumbling over your own feet. They occur when you’ve made a mistake in the basic structure of your code.
print("Hello World" # Missing closing parenthesis # Correct version: print("Hello World")
-
Logic Errors: These are trickier, like trying to walk in the wrong direction. Your code runs, but it doesn’t do what you expect.
def add_numbers(a, b): return a - b # Oops! We're subtracting instead of adding # Correct version: def add_numbers(a, b): return a + b
-
Runtime Errors: These occur when your code tries to do something impossible, like dividing by zero. It’s like trying to walk through a wall.
numbers = [1, 2, 3] print(numbers[3]) # Trying to access an index that doesn't exist # Correct version: print(numbers[2]) # Remember, indexing starts at 0!
Learning to identify and fix these errors is a crucial part of your coding journey. It’s frustrating at first, but each error you overcome makes you a stronger programmer.
Finding Your Balance: Building Your First Projects
As you become more comfortable with the basics, you’ll start to work on small projects. This is like a toddler gaining confidence and exploring their environment. Your projects might be simple at first – a basic calculator, a to-do list app, or a simple game – but they’re crucial for building your skills and confidence.
AlgoCademy provides a range of project-based learning experiences to help you apply your skills in practical scenarios. These projects are designed to challenge you just enough to promote growth without overwhelming you.
Project Idea: Building a Simple Guessing Game
Here’s an example of a simple project you might tackle as you’re finding your coding feet:
import random
def guessing_game():
number = random.randint(1, 100)
attempts = 0
print("I'm thinking of a number between 1 and 100. Can you guess it?")
while True:
guess = int(input("Enter your guess: "))
attempts += 1
if guess < number:
print("Too low! Try again.")
elif guess > number:
print("Too high! Try again.")
else:
print(f"Congratulations! You guessed the number in {attempts} attempts.")
break
guessing_game()
This project incorporates several important programming concepts: loops, conditionals, user input, and random number generation. It’s simple enough for a beginner to understand, but complex enough to be engaging and educational.
Gaining Speed: Mastering Algorithms and Data Structures
As you become more proficient, you’ll start to tackle more complex topics like algorithms and data structures. This is akin to a child progressing from walking to running and jumping. You’re not just writing code anymore; you’re learning to write efficient, optimized code.
AlgoCademy’s curriculum is specifically designed to guide you through this crucial phase. We provide in-depth tutorials on common algorithms and data structures, along with practice problems to reinforce your learning.
Understanding Big O Notation
One key concept you’ll encounter is Big O notation, which is used to describe the performance or complexity of an algorithm. Here’s a quick overview:
- O(1): Constant time – the algorithm always takes the same amount of time, regardless of input size.
- O(n): Linear time – the time taken increases linearly with input size.
- O(n²): Quadratic time – the time taken increases quadratically with input size.
- O(log n): Logarithmic time – the time taken increases logarithmically with input size.
Understanding these concepts allows you to write more efficient code and solve problems more effectively.
Example: Implementing a Binary Search
Let’s look at an implementation of a binary search algorithm, which has a time complexity of O(log n):
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage:
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
This algorithm efficiently searches a sorted array by repeatedly dividing the search interval in half. Understanding and implementing algorithms like this is a significant step in your coding journey.
Running with Confidence: Tackling Complex Problems
As you continue to grow as a programmer, you’ll find yourself able to tackle increasingly complex problems. This is like a child who has mastered walking and running, now learning to play sports or dance. You’re not just writing code; you’re architecting solutions to real-world problems.
At AlgoCademy, we provide advanced tutorials and challenges that mirror the types of problems you might encounter in technical interviews at major tech companies. These problems often require you to combine multiple concepts and think creatively to find optimal solutions.
Example: Solving the Longest Palindromic Substring Problem
Here’s an example of a more complex problem you might encounter:
def longest_palindrome_substring(s):
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
length1 = expand_around_center(i, i)
length2 = expand_around_center(i, i + 1)
length = max(length1, length2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
# Example usage:
s = "babad"
result = longest_palindrome_substring(s)
print(f"Longest palindromic substring: {result}")
This problem requires a good understanding of string manipulation, dynamic programming concepts, and optimization techniques. Solving problems like this demonstrates a high level of coding proficiency.
The Journey Never Ends: Continuous Learning and Improvement
Even when you’ve “mastered” coding, the learning never stops. The tech world is constantly evolving, with new languages, frameworks, and paradigms emerging all the time. This is like an adult who has mastered walking and running, but still takes dance classes or learns new sports.
At AlgoCademy, we’re committed to helping you on this lifelong learning journey. Our platform is constantly updated with new content, challenges, and resources to keep you at the cutting edge of coding and software development.
Staying Current: Exploring New Technologies
Here are some ways to keep your skills sharp and stay current in the ever-changing world of technology:
- Learn a New Language: If you’re comfortable with Python, try learning JavaScript or Go.
- Explore Cloud Computing: Familiarize yourself with platforms like AWS, Google Cloud, or Azure.
- Dive into Machine Learning: Start with the basics of ML and work your way up to advanced topics like deep learning.
- Contribute to Open Source: This is a great way to work on real-world projects and collaborate with other developers.
- Attend Tech Conferences and Meetups: These events can expose you to new ideas and help you network with other professionals.
Embracing the Messiness: Why It’s Okay to Struggle
Throughout this post, we’ve drawn parallels between learning to code and learning to walk. One of the most important lessons from this analogy is that it’s okay – even necessary – to struggle and make mistakes.
When a toddler is learning to walk, they fall down countless times. Each fall is a learning experience, teaching them about balance, momentum, and the limits of their own body. Similarly, every bug you encounter, every error message you decipher, and every problem you solve is teaching you valuable lessons about programming.
The Growth Mindset in Coding
Adopting a growth mindset is crucial for success in coding. This means:
- Viewing challenges as opportunities for growth, not insurmountable obstacles
- Embracing the process of learning, not just the end result
- Seeing effort as the path to mastery, not something to be avoided
- Learning from criticism and the success of others
- Finding inspiration and lessons in the success of others
At AlgoCademy, we foster this growth mindset through our supportive community, constructive feedback mechanisms, and carefully designed learning pathways that challenge you appropriately at each stage of your journey.
Conclusion: Your Coding Journey is Unique
Just as every child learns to walk at their own pace, every coder’s journey is unique. Some may progress quickly through the basics, while others take more time. Some may excel at algorithmic thinking, while others shine in creative problem-solving or user interface design.
The key is to embrace your journey, celebrate your progress (no matter how small), and keep pushing forward. Remember, even the most accomplished programmers were once beginners, struggling with the same concepts you’re grappling with now.
At AlgoCademy, we’re here to support you every step of the way. From your first “Hello, World!” to tackling complex algorithmic challenges, we provide the resources, guidance, and community you need to grow as a programmer.
So, don’t be discouraged by the messiness of learning to code. Embrace it, learn from it, and use it as fuel for your growth. Before you know it, you’ll be running confidently through the world of programming, ready to take on whatever challenges come your way.
Happy coding, and remember – every expert was once a beginner. Your coding mastery is just a series of messy, beautiful steps away.