Learning Through Feedback Loops: How to Iterate on Your Code Solutions
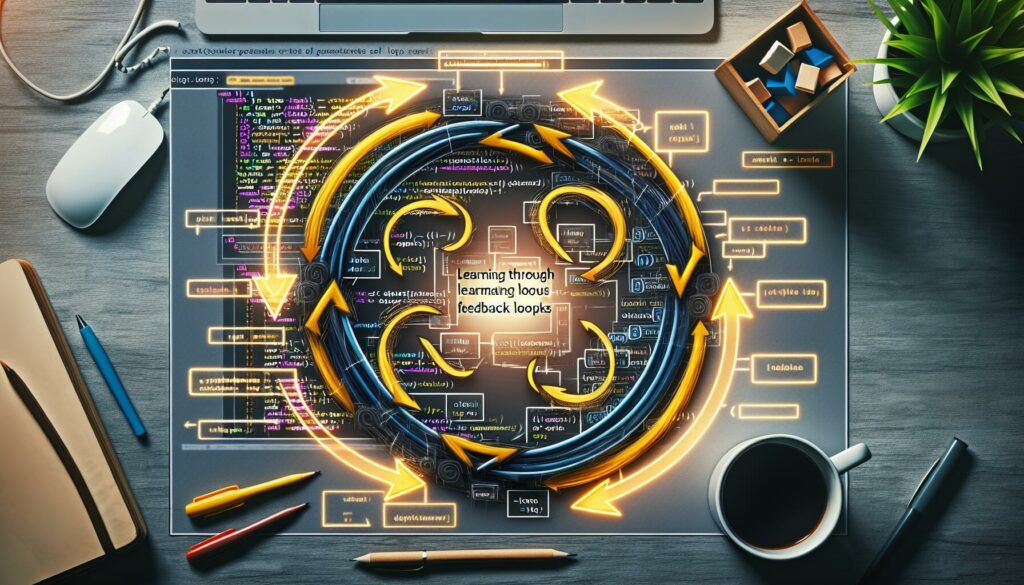
In the world of coding and software development, the ability to continuously improve and refine your solutions is a crucial skill. One of the most effective ways to achieve this is through the use of feedback loops. These iterative cycles of implementation, evaluation, and improvement can significantly enhance your coding abilities and help you create more efficient, robust, and elegant solutions. In this comprehensive guide, we’ll explore the concept of feedback loops in coding, their importance, and how you can leverage them to become a better programmer.
Understanding Feedback Loops in Coding
A feedback loop in coding is a process where the output of a system is used as input to make improvements or adjustments to that same system. In the context of programming, this typically involves writing code, testing it, analyzing the results, and then making changes based on those results. This cycle repeats, allowing for continuous improvement and refinement of your code.
The key components of a coding feedback loop include:
- Implementation: Writing the initial code or making changes to existing code
- Execution: Running the code to see how it performs
- Evaluation: Analyzing the results and identifying areas for improvement
- Iteration: Making changes based on the evaluation and starting the cycle again
The Importance of Feedback Loops in Coding Education
Feedback loops are particularly valuable in the context of coding education and skill development. Here’s why they’re so important:
- Accelerated Learning: By quickly implementing, testing, and refining your code, you can learn from your mistakes and successes more rapidly than with a linear approach.
- Practical Skill Development: Feedback loops encourage hands-on practice, which is essential for developing real-world coding skills.
- Problem-Solving Enhancement: The iterative nature of feedback loops helps you develop better problem-solving strategies over time.
- Adaptation to Different Scenarios: As you encounter various coding challenges, feedback loops help you adapt your solutions more effectively.
- Preparation for Professional Development: In professional settings, the ability to iterate and improve code is highly valued, making this skill crucial for career advancement.
Implementing Effective Feedback Loops in Your Coding Practice
To make the most of feedback loops in your coding journey, consider the following strategies:
1. Start with Clear Objectives
Before you begin coding, clearly define what you want to achieve. This could be solving a specific problem, implementing a particular feature, or optimizing an existing piece of code. Having a clear objective helps you focus your efforts and provides a benchmark for evaluating your progress.
2. Write Testable Code
Structure your code in a way that makes it easy to test. This often involves breaking your solution down into smaller, modular components. Each component should have a clear purpose and be testable in isolation. Consider using test-driven development (TDD) approaches where you write tests before implementing the actual code.
Here’s an example of a testable function in Python:
def is_palindrome(s):
s = ''.join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
# Test cases
assert is_palindrome("A man, a plan, a canal: Panama") == True
assert is_palindrome("race a car") == False
assert is_palindrome("") == True
3. Leverage Automated Testing
Implement automated tests to quickly verify your code’s correctness. This could include unit tests, integration tests, and end-to-end tests. Automated testing allows you to catch errors early and ensures that your changes don’t introduce new bugs.
Here’s an example using Python’s unittest framework:
import unittest
class TestPalindrome(unittest.TestCase):
def test_is_palindrome(self):
self.assertTrue(is_palindrome("A man, a plan, a canal: Panama"))
self.assertFalse(is_palindrome("race a car"))
self.assertTrue(is_palindrome(""))
if __name__ == '__main__':
unittest.main()
4. Use Version Control
Implement a version control system like Git to track changes in your code over time. This allows you to experiment with different approaches, revert to previous versions if needed, and maintain a history of your iterations. It’s also an essential tool for collaboration in professional settings.
5. Analyze Performance Metrics
Use profiling tools and benchmarks to measure your code’s performance. This can help you identify bottlenecks and areas for optimization. Many programming languages have built-in profiling tools or third-party libraries that can help with this.
For example, in Python, you can use the cProfile module:
import cProfile
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
cProfile.run('fibonacci(30)')
6. Seek External Feedback
Don’t rely solely on your own evaluation. Seek feedback from peers, mentors, or online communities. Code reviews can provide valuable insights and alternative perspectives on your solutions. Platforms like GitHub or Stack Overflow can be great resources for this.
7. Reflect on Your Process
After each iteration, take time to reflect on what you’ve learned. Consider questions like:
- What worked well in this iteration?
- What challenges did I face, and how did I overcome them?
- How can I apply these lessons to future coding tasks?
This reflection helps reinforce your learning and improves your problem-solving strategies over time.
Advanced Techniques for Leveraging Feedback Loops
As you become more comfortable with basic feedback loops, consider incorporating these advanced techniques:
1. A/B Testing
When faced with multiple potential solutions, implement A/B testing to compare their performance. This involves running two or more versions of your code and comparing their outcomes based on predefined metrics.
import random
def solution_a(data):
# Implementation of solution A
pass
def solution_b(data):
# Implementation of solution B
pass
def ab_test(data, num_trials=1000):
a_results = []
b_results = []
for _ in range(num_trials):
if random.choice([True, False]):
a_results.append(solution_a(data))
else:
b_results.append(solution_b(data))
# Compare results and determine which solution performs better
# based on your defined metrics
pass
2. Continuous Integration (CI)
Implement a CI system that automatically runs tests and checks code quality whenever you push changes to your version control system. This ensures that your feedback loop is consistently applied and helps catch issues early in the development process.
3. Fuzzing
Use fuzzing techniques to generate random or semi-random input data for your code. This can help uncover edge cases and potential vulnerabilities that you might not have considered in your initial test cases.
import random
import string
def generate_random_string(length):
return ''.join(random.choice(string.ascii_letters + string.digits) for _ in range(length))
def fuzz_test(func, num_tests=1000):
for _ in range(num_tests):
input_length = random.randint(0, 100)
random_input = generate_random_string(input_length)
try:
func(random_input)
except Exception as e:
print(f"Error with input: {random_input}")
print(f"Exception: {e}")
# Example usage
fuzz_test(is_palindrome)
4. Performance Profiling
Use advanced profiling tools to dive deep into your code’s performance characteristics. This can help you identify micro-optimizations and understand the impact of your changes on overall system performance.
5. Code Complexity Analysis
Incorporate tools that analyze code complexity, such as cyclomatic complexity or cognitive complexity. This can help you identify areas of your code that may be difficult to maintain or prone to errors.
import ast
import mccabe
def analyze_complexity(code):
tree = ast.parse(code)
visitor = mccabe.McCabeVisitor()
visitor.preorder(tree)
return visitor.complexity()
# Example usage
code = """
def complex_function(x, y):
if x > 0:
if y > 0:
return x + y
else:
return x - y
else:
if y > 0:
return -x + y
else:
return -x - y
"""
complexity = analyze_complexity(code)
print(f"Cyclomatic complexity: {complexity}")
Overcoming Common Challenges in Implementing Feedback Loops
While feedback loops are powerful tools for improvement, you may encounter some challenges when implementing them. Here are some common issues and strategies to overcome them:
1. Overcoming Analysis Paralysis
Sometimes, the wealth of feedback and potential improvements can lead to decision paralysis. To combat this:
- Set clear priorities for each iteration
- Implement time-boxing techniques to limit the time spent on each improvement cycle
- Focus on making small, incremental improvements rather than trying to perfect everything at once
2. Dealing with Conflicting Feedback
When receiving feedback from multiple sources, you may encounter conflicting opinions. To handle this:
- Evaluate feedback based on the credibility and expertise of the source
- Test conflicting suggestions to see which performs better in practice
- Seek consensus among multiple expert opinions when possible
3. Maintaining Motivation Through Multiple Iterations
The iterative nature of feedback loops can sometimes feel tedious. To stay motivated:
- Celebrate small wins and improvements
- Set intermediate goals and milestones
- Vary your focus between different aspects of your code (e.g., functionality, performance, readability) to keep things interesting
4. Balancing Perfection and Progress
It’s easy to fall into the trap of endlessly refining code without making meaningful progress. To find the right balance:
- Define “good enough” criteria for each iteration
- Use the concept of MVP (Minimum Viable Product) to ensure you’re delivering functional solutions
- Schedule dedicated time for refactoring and optimization separate from feature development
Integrating Feedback Loops into Your Long-Term Learning Strategy
To truly benefit from feedback loops, it’s important to integrate them into your overall learning strategy. Here are some ways to do this:
1. Create a Personal Learning Roadmap
Develop a roadmap that outlines your learning goals and the skills you want to develop. Use feedback loops to track your progress and adjust your learning path as needed.
2. Build a Portfolio of Iterative Projects
Work on long-term projects that you can continuously improve over time. This allows you to apply feedback loops on a larger scale and see how your skills develop across multiple iterations.
3. Participate in Coding Challenges and Hackathons
These events provide opportunities to apply feedback loops in a time-constrained environment, helping you develop the ability to iterate quickly and efficiently.
4. Contribute to Open Source Projects
Open source contributions expose you to feedback from experienced developers and allow you to see how feedback loops work in large-scale, collaborative projects.
5. Teach Others
Explaining your code and thought processes to others can provide valuable insights and help you identify areas for improvement in your own understanding and approach.
Conclusion
Learning through feedback loops is a powerful approach to improving your coding skills and creating better software solutions. By implementing, evaluating, and iterating on your code, you can accelerate your learning, enhance your problem-solving abilities, and develop the skills necessary for success in the ever-evolving world of software development.
Remember that the key to effective feedback loops is consistency and openness to change. Embrace the iterative process, be willing to critically evaluate your own work, and always seek opportunities for improvement. With practice, you’ll find that feedback loops become an integral part of your coding workflow, leading to more efficient, robust, and elegant solutions.
As you continue your coding journey, keep exploring new tools, techniques, and resources that can enhance your feedback loops. Stay curious, stay persistent, and most importantly, enjoy the process of continuous improvement. Happy coding!