Learning Graph Databases: An Introduction to Neo4j
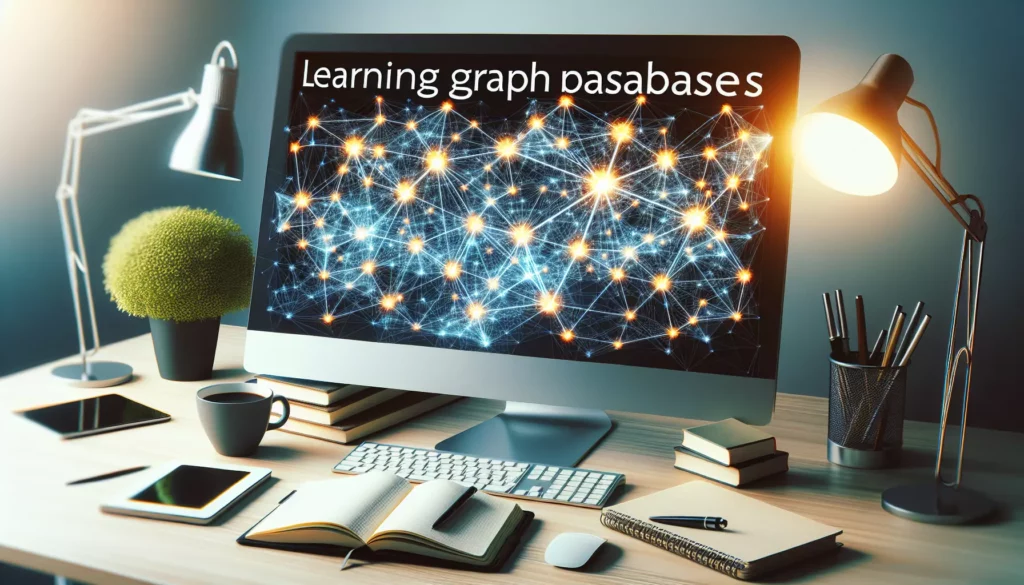
In the ever-evolving landscape of database technologies, graph databases have emerged as a powerful tool for managing complex, interconnected data. Among the various graph database solutions available, Neo4j stands out as a popular and robust option. This comprehensive guide will introduce you to graph databases and provide an in-depth look at Neo4j, helping you understand its concepts, features, and practical applications.
Table of Contents
- Understanding Graph Databases
- Introduction to Neo4j
- Key Concepts in Neo4j
- Setting Up Neo4j
- Cypher Query Language
- CRUD Operations in Neo4j
- Advanced Querying and Data Modeling
- Neo4j Use Cases
- Best Practices and Optimization
- Integrating Neo4j with Other Technologies
- Conclusion
1. Understanding Graph Databases
Before diving into Neo4j, it’s essential to understand what graph databases are and how they differ from traditional relational databases.
What is a Graph Database?
A graph database is a type of NoSQL database that uses graph structures to represent and store data. Unlike relational databases that use tables, rows, and columns, graph databases use nodes, edges, and properties to organize and connect data.
Key Components of Graph Databases
- Nodes: Represent entities or objects in the data model.
- Edges: Represent relationships between nodes.
- Properties: Attributes that describe nodes and edges.
Advantages of Graph Databases
- Flexibility: Easily adapt to changing data structures and relationships.
- Performance: Efficient for querying highly connected data.
- Intuitive Data Modeling: Represent complex relationships naturally.
- Scalability: Scale horizontally to handle large datasets and complex queries.
2. Introduction to Neo4j
Neo4j is an open-source, native graph database management system developed by Neo4j, Inc. It is designed to leverage the power of graph structures for intuitive modeling, storage, and querying of connected data.
Key Features of Neo4j
- ACID (Atomicity, Consistency, Isolation, Durability) compliant
- Native graph storage and processing
- Scalable architecture
- Cypher query language
- Built-in web interface (Neo4j Browser)
- Rich ecosystem of drivers and integrations
Neo4j Editions
- Community Edition: Free, open-source version for individual developers and small projects.
- Enterprise Edition: Commercial version with advanced features for large-scale deployments.
3. Key Concepts in Neo4j
To effectively work with Neo4j, it’s crucial to understand its fundamental concepts and data model.
Nodes
Nodes are the fundamental entities in a Neo4j graph. They can represent any object or concept and can have multiple labels and properties.
Labels
Labels are used to group nodes into sets. A node can have multiple labels, allowing for flexible categorization.
Relationships
Relationships connect nodes and represent the associations between them. They are directional and can have properties.
Properties
Properties are key-value pairs that can be attached to both nodes and relationships, providing additional information about the entities and their connections.
Traversal
Traversal is the process of exploring the graph by following relationships between nodes. It’s a fundamental operation in graph databases and is crucial for efficient querying.
4. Setting Up Neo4j
To get started with Neo4j, you’ll need to set up the environment. Here’s a step-by-step guide to help you get Neo4j up and running.
Installing Neo4j
- Visit the official Neo4j website (https://neo4j.com/download/).
- Choose the appropriate version for your operating system.
- Download and run the installer.
- Follow the installation wizard instructions.
Starting Neo4j
After installation, you can start Neo4j using the following methods:
- Use the Neo4j Desktop application (recommended for beginners).
- Start Neo4j from the command line (for advanced users).
Accessing Neo4j Browser
Neo4j Browser is a web-based interface for interacting with your Neo4j database. To access it:
- Open a web browser.
- Navigate to
http://localhost:7474
(default address). - Log in using the default credentials (username: neo4j, password: neo4j).
- Change the default password when prompted.
5. Cypher Query Language
Cypher is Neo4j’s declarative query language, designed specifically for working with graph data. It allows you to describe what you want to select, insert, update, or delete from your graph database without requiring you to describe exactly how to do it.
Basic Cypher Syntax
Cypher uses ASCII-Art to represent patterns. Here are some basic elements:
()
– Represents a node[]
– Represents a relationship->
– Indicates relationship direction:
– Assigns a label to a node or relationship type{}
– Defines properties
Common Cypher Clauses
MATCH
: Pattern matching to find dataWHERE
: Filtering resultsRETURN
: Specifying what to include in the query resultsCREATE
: Creating new nodes and relationshipsMERGE
: Creating unique nodes and relationshipsSET
: Updating propertiesDELETE
: Removing nodes, relationships, and properties
Example Cypher Query
Here’s a simple Cypher query to find all people who are friends with someone named “Alice”:
MATCH (p:Person)-[:FRIENDS_WITH]->(alice:Person {name: "Alice"})
RETURN p.name
6. CRUD Operations in Neo4j
Let’s explore how to perform basic CRUD (Create, Read, Update, Delete) operations in Neo4j using Cypher.
Create
To create a new node:
CREATE (p:Person {name: "John Doe", age: 30})
RETURN p
To create a relationship between two nodes:
MATCH (a:Person {name: "John Doe"}), (b:Person {name: "Jane Smith"})
CREATE (a)-[:FRIENDS_WITH]->(b)
RETURN a, b
Read
To retrieve all nodes with a specific label:
MATCH (p:Person)
RETURN p
To find nodes with specific properties:
MATCH (p:Person {name: "John Doe"})
RETURN p
Update
To update a node’s properties:
MATCH (p:Person {name: "John Doe"})
SET p.age = 31
RETURN p
Delete
To delete a node:
MATCH (p:Person {name: "John Doe"})
DELETE p
To delete a relationship:
MATCH (a:Person {name: "John Doe"})-[r:FRIENDS_WITH]->(b:Person {name: "Jane Smith"})
DELETE r
7. Advanced Querying and Data Modeling
As you become more comfortable with Neo4j, you’ll want to explore more advanced querying techniques and data modeling strategies.
Complex Queries
Neo4j excels at handling complex queries involving multiple relationships and conditions. Here’s an example of a more advanced query:
MATCH (person:Person)-[:WORKS_AT]->(company:Company)
WHERE company.industry = "Technology"
AND person.age > 30
WITH person, company
MATCH (person)-[:LIVES_IN]->(city:City)
WHERE city.population > 1000000
RETURN person.name, company.name, city.name
Aggregations and Functions
Cypher supports various aggregation functions and mathematical operations:
COUNT()
: Count the number of resultsSUM()
: Calculate the sum of a set of valuesAVG()
: Calculate the average of a set of valuesMAX()
andMIN()
: Find the maximum and minimum valuesCOLLECT()
: Gather results into a list
Path Finding and Graph Algorithms
Neo4j provides built-in algorithms for common graph operations:
- Shortest path
- All paths
- Centrality algorithms
- Community detection
Data Modeling Best Practices
- Model for queries: Design your graph structure based on the queries you need to perform.
- Use meaningful relationship types: Choose descriptive names for relationships to improve clarity.
- Avoid overly complex nodes: Break down complex entities into multiple connected nodes.
- Consider bidirectional relationships: Sometimes, it’s useful to create relationships in both directions for easier querying.
8. Neo4j Use Cases
Neo4j’s graph structure makes it particularly well-suited for certain types of applications and industries:
Social Networks
Graph databases excel at modeling and querying social connections, making them ideal for social networking applications, recommendation engines, and influencer analysis.
Fraud Detection
By analyzing patterns and connections in financial transactions, Neo4j can help identify potential fraudulent activities more effectively than traditional relational databases.
Knowledge Graphs
Neo4j is excellent for building and querying knowledge graphs, which can be used in various applications such as semantic search, AI assistants, and content recommendation systems.
Network and IT Operations
Graph databases can model complex network topologies and dependencies, making them useful for IT infrastructure management, impact analysis, and root cause analysis.
Supply Chain Management
Neo4j can model and analyze complex supply chain networks, helping optimize logistics, identify bottlenecks, and improve overall efficiency.
9. Best Practices and Optimization
To ensure optimal performance and maintainability of your Neo4j database, consider the following best practices:
Indexing
Create indexes on frequently queried properties to improve query performance:
CREATE INDEX ON :Person(name)
Query Optimization
- Use parameters instead of hard-coded values in queries for better caching and security.
- Limit the number of returned results when possible.
- Use
EXPLAIN
andPROFILE
to analyze query performance.
Data Model Optimization
- Denormalize data when appropriate to reduce the number of relationships traversed.
- Use labels effectively to partition your graph and improve query performance.
- Consider using relationship properties instead of intermediate nodes for simple attributes.
Backup and Recovery
Regularly backup your Neo4j database to prevent data loss. Neo4j Enterprise Edition provides built-in backup and restore functionality.
10. Integrating Neo4j with Other Technologies
Neo4j can be integrated with various programming languages and frameworks to build robust applications:
Programming Language Drivers
Neo4j provides official drivers for several popular programming languages:
- Java
- Python
- JavaScript
- .NET
- Go
Web Frameworks
Neo4j can be easily integrated with web frameworks such as:
- Spring (Java)
- Django (Python)
- Express.js (Node.js)
- ASP.NET (C#)
Data Integration Tools
Neo4j can be connected to various data integration and ETL tools:
- Apache Kafka
- Apache Spark
- Talend
- Pentaho
Visualization Tools
Several visualization tools can be used to create interactive graph visualizations from Neo4j data:
- Neovis.js
- Bloom (Neo4j’s visualization tool)
- Linkurious
- KeyLines
11. Conclusion
Neo4j is a powerful and flexible graph database that offers a unique approach to storing and querying connected data. Its intuitive data model, expressive query language, and robust ecosystem make it an excellent choice for applications that deal with complex relationships and interconnected information.
As you continue your journey with Neo4j, remember that graph thinking is a skill that develops over time. Practice modeling different scenarios as graphs, experiment with various querying techniques, and stay updated with the latest developments in the Neo4j ecosystem.
Whether you’re building a social network, optimizing a supply chain, or developing a recommendation engine, Neo4j provides the tools and capabilities to handle your graph data efficiently and effectively. By mastering Neo4j, you’ll add a valuable skill to your repertoire and open up new possibilities for solving complex data problems in your projects and career.