Learning from Failure: The Programmer’s Growth Mindset
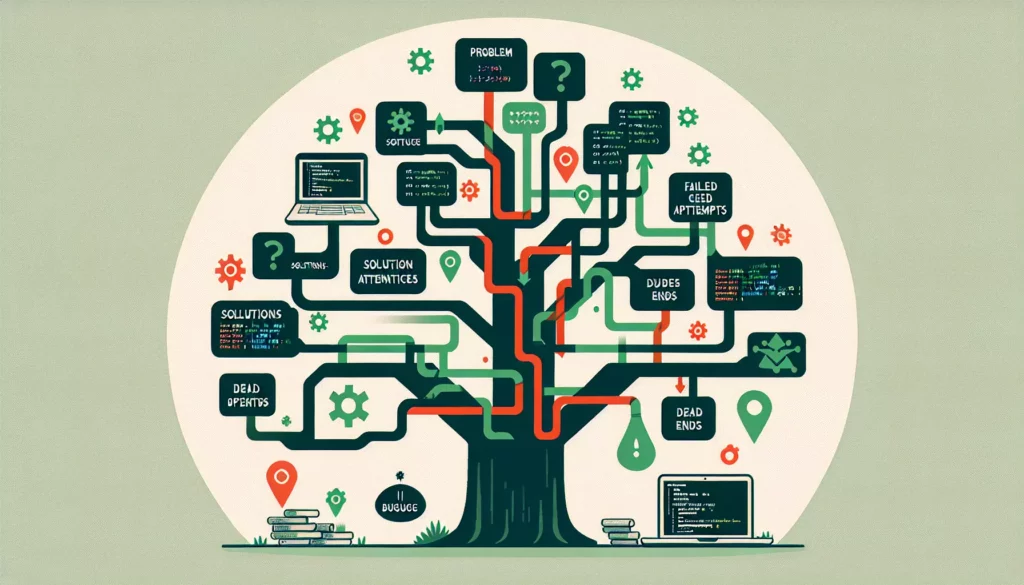
In the world of programming, failure is not just a possibility—it’s an inevitability. From syntax errors to logical flaws, from buggy code to failed deployments, every programmer encounters setbacks on their journey. However, what separates successful developers from those who struggle is not the absence of failure, but rather how they respond to it. This is where the concept of a growth mindset becomes crucial in a programmer’s career.
Understanding the Growth Mindset
The term “growth mindset” was coined by psychologist Carol Dweck and refers to the belief that abilities and intelligence can be developed through dedication, hard work, and learning from failures. In contrast, a fixed mindset assumes that our character, intelligence, and creative ability are static givens that we can’t change in any meaningful way.
For programmers, adopting a growth mindset is particularly important because the field of technology is constantly evolving. New languages, frameworks, and methodologies emerge regularly, and those who believe in their ability to learn and adapt are more likely to thrive in this dynamic environment.
The Role of Failure in Programming
Failure in programming takes many forms:
- Compilation errors
- Runtime exceptions
- Logical errors in algorithms
- Performance issues
- Security vulnerabilities
- Failed project deadlines
- Rejected pull requests
Each of these failures presents an opportunity for growth. Let’s explore how programmers can leverage these experiences to become better developers.
Strategies for Learning from Failure
1. Embrace Debugging as a Learning Tool
Debugging is often seen as a chore, but it’s one of the most valuable learning experiences in programming. When you encounter a bug, instead of getting frustrated, approach it with curiosity. Ask yourself:
- What exactly is going wrong?
- What was my initial assumption about how this code would work?
- How does the actual behavior differ from what I expected?
- What can I learn about the language or system from this unexpected behavior?
By treating debugging as an investigative process, you’ll not only solve the immediate problem but also deepen your understanding of the programming language and environment you’re working in.
2. Document Your Failures and Solutions
Keep a “failure log” or a personal wiki where you document the problems you encounter and how you solve them. This practice serves multiple purposes:
- It helps reinforce the lessons learned from each failure
- It creates a personal knowledge base you can refer back to
- It allows you to track your progress and see how you’ve grown over time
Here’s an example of how you might structure an entry in your failure log:
Date: 2023-06-15
Problem: IndexOutOfBoundsException in Java array iteration
Code snippet:
for (int i = 0; i <= myArray.length; i++) {
System.out.println(myArray[i]);
}
Error message: java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
Root cause: Loop condition used <= instead of <
Solution: Changed loop condition to i < myArray.length
Lesson learned: Always use < when iterating through arrays to avoid accessing an index that doesn't exist.
3. Seek Feedback and Code Reviews
One of the best ways to learn from failure is to get input from others. Actively seek out code reviews from more experienced developers. When your code is critiqued, don’t take it personally. Instead:
- Ask questions to understand the reasoning behind suggested changes
- Look for patterns in the feedback you receive
- Implement the suggestions and observe how they improve your code
Remember, even senior developers have their code reviewed. It’s a normal part of the development process and a valuable learning opportunity.
4. Practice Deliberate Learning
When you encounter a failure, use it as a springboard for deliberate learning. If a particular concept or technology is giving you trouble, create a small project focused on mastering that specific area. For example, if you’re struggling with asynchronous programming in JavaScript, you might create a simple application that fetches data from multiple APIs concurrently.
Here’s a basic example of asynchronous programming using JavaScript Promises:
function fetchUserData(userId) {
return fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.catch(error => console.error('Error fetching user data:', error));
}
function fetchUserPosts(userId) {
return fetch(`https://api.example.com/users/${userId}/posts`)
.then(response => response.json())
.catch(error => console.error('Error fetching user posts:', error));
}
Promise.all([fetchUserData(123), fetchUserPosts(123)])
.then(([userData, userPosts]) => {
console.log('User Data:', userData);
console.log('User Posts:', userPosts);
})
.catch(error => console.error('Error:', error));
By working through examples like this, you can turn a point of failure into an opportunity for focused improvement.
5. Reframe Your Self-Talk
The way you talk to yourself about failure can significantly impact your ability to learn from it. Instead of negative self-talk, try reframing your thoughts:
- Instead of “I’m not good at this,” think “I’m still learning this.”
- Replace “I made a mistake” with “I discovered an area for improvement.”
- Change “This is too hard” to “This is challenging, but I can figure it out.”
This positive reframing helps maintain motivation and reinforces the growth mindset.
Real-World Examples of Learning from Failure
Let’s look at some real-world scenarios where programmers turned failures into valuable learning experiences:
The Case of the Billion-Dollar Bug
In 1962, NASA’s Mariner 1 space probe was destroyed shortly after takeoff due to a software error. The cause? A missing hyphen in the coded mathematical instructions. This costly mistake led to significantly improved code review processes and the development of more robust programming languages designed to catch such errors.
Lesson learned: Always double-check your code, especially in critical systems. This incident spurred the development of better testing and verification processes in software engineering.
Amazon’s Database Migration Mishap
In 2019, Amazon attempted to migrate its user database from Oracle to its own AWS database services. The process encountered unexpected issues, causing delays in Prime Day preparations. Instead of seeing this as a failure, Amazon used the experience to improve its migration tools and processes, ultimately completing the migration successfully.
Lesson learned: Large-scale system changes often come with unforeseen challenges. Proper planning, testing, and the ability to adapt are crucial in such projects.
The Birth of Ruby on Rails
David Heinemeier Hansson created Ruby on Rails out of frustration with the complexity of existing web development frameworks. His “failure” to find a satisfactory solution led him to create one of the most influential web frameworks of the past two decades.
Lesson learned: Sometimes, the best way to learn is to build something yourself. Your frustrations and failures can be the catalyst for innovation.
Implementing a Growth Mindset in Your Coding Journey
Now that we’ve explored the importance of learning from failure and seen some real-world examples, let’s discuss how you can implement a growth mindset in your own coding journey:
1. Set Learning Goals, Not Just Performance Goals
Instead of focusing solely on completing projects or passing tests, set goals that emphasize learning and skill development. For example:
- “I will learn and implement three new design patterns this month.”
- “I will contribute to an open-source project to improve my collaboration skills.”
- “I will deep dive into asynchronous programming concepts and create a project showcasing them.”
2. Celebrate Small Wins and Incremental Progress
Acknowledge and celebrate your progress, no matter how small. Did you finally understand that tricky concept? Solve a challenging problem? Fixed a bug that’s been bothering you for days? Take a moment to recognize these achievements. They’re all steps forward in your growth as a programmer.
3. Engage in Reflective Practice
Regularly reflect on your coding experiences. You might keep a coding journal where you answer questions like:
- What did I learn this week?
- What challenges did I face, and how did I overcome them?
- What areas do I need to focus on improving?
- How can I apply what I’ve learned to future projects?
This reflective practice helps reinforce learning and identifies areas for future growth.
4. Embrace Challenges and Step Out of Your Comfort Zone
Actively seek out challenges that push your boundaries. This might involve:
- Taking on a project using a new programming language or framework
- Participating in coding competitions or hackathons
- Tackling complex algorithmic problems on platforms like LeetCode or HackerRank
Remember, feeling challenged is a sign that you’re growing and learning.
5. Foster a Learning Community
Surround yourself with others who share a growth mindset. This could involve:
- Joining a local coding meetup or study group
- Participating in online forums and communities
- Finding a coding mentor or becoming a mentor yourself
Learning from and with others can provide new perspectives and accelerate your growth.
Tools and Resources for Continuous Learning
To support your journey of learning from failure and maintaining a growth mindset, consider using these tools and resources:
1. Version Control Systems
Tools like Git allow you to track changes in your code over time. This can be invaluable for understanding how your code evolves and for learning from past mistakes. Here’s a basic Git workflow:
git init # Initialize a new Git repository
git add . # Stage all changes
git commit -m "Initial commit" # Commit changes with a descriptive message
git log # View commit history
2. Code Analysis Tools
Static code analysis tools can help identify potential issues in your code before they become runtime errors. Some popular options include:
- ESLint for JavaScript
- Pylint for Python
- SonarQube for multiple languages
3. Online Learning Platforms
Platforms like Codecademy, freeCodeCamp, and Coursera offer structured learning paths and hands-on coding exercises. These can be great for filling knowledge gaps and learning new technologies.
4. Coding Challenge Websites
Sites like LeetCode, HackerRank, and Project Euler provide algorithmic challenges that can help you improve your problem-solving skills and learn from your mistakes in a controlled environment.
5. Documentation and Learning Wikis
Create your own personal wiki or use tools like Notion or Obsidian to document your learning journey, including challenges faced and solutions found.
Conclusion: Embracing Failure as a Path to Success
In the ever-evolving world of programming, failure is not just inevitable—it’s essential. Every bug you encounter, every project that doesn’t go as planned, and every concept you struggle to grasp is an opportunity for growth. By adopting a growth mindset and viewing failures as learning experiences, you transform these setbacks into stepping stones towards becoming a better programmer.
Remember, even the most successful developers faced countless failures on their journey. What sets them apart is their ability to learn from these experiences, adapt, and keep pushing forward. As you continue your coding journey, embrace your failures, celebrate your progress, and maintain a curiosity for learning. With this mindset, every line of code you write—whether it works perfectly or fails spectacularly—becomes a valuable part of your growth as a programmer.
In the words of Thomas Edison, “I have not failed. I’ve just found 10,000 ways that won’t work.” So, the next time you encounter a failure in your coding journey, remember: it’s not a setback, but a setup for your next breakthrough. Happy coding, and may your failures be as enlightening as your successes!