Learning by Doing: How to Develop Projects That Solve Real Problems
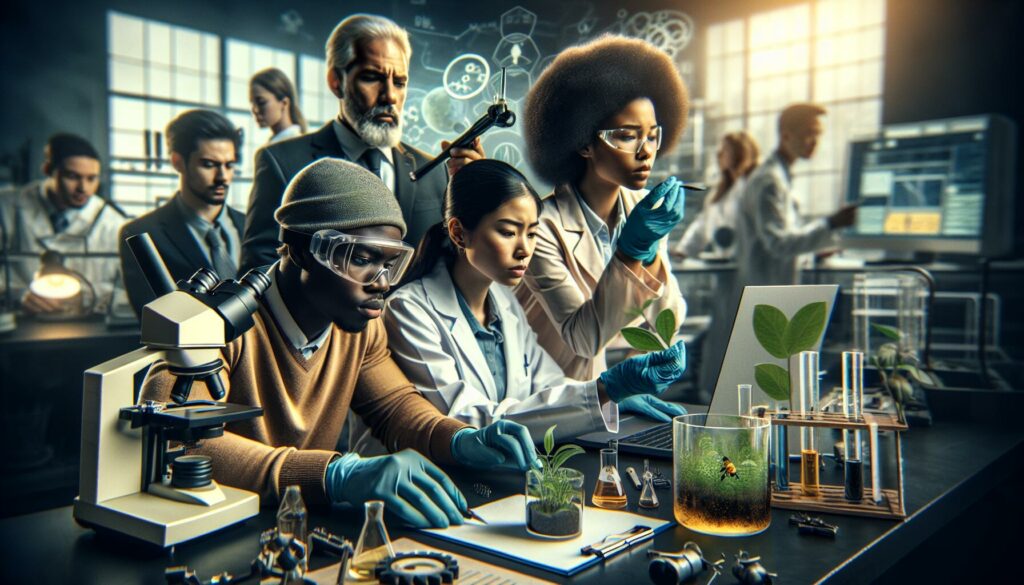
In the world of coding and software development, there’s a saying that rings true for learners at all levels: “The best way to learn is by doing.” This principle is at the heart of project-based learning, a powerful approach that not only enhances your coding skills but also prepares you for real-world challenges. In this comprehensive guide, we’ll explore how to develop projects that solve real problems, a method that aligns perfectly with AlgoCademy’s mission of nurturing algorithmic thinking and practical coding skills.
Why Project-Based Learning Matters
Before we dive into the how-to, let’s understand why project-based learning is so crucial:
- Practical Application: It bridges the gap between theory and practice, allowing you to apply what you’ve learned in real scenarios.
- Problem-Solving Skills: You develop critical thinking and problem-solving abilities that are essential in the tech industry.
- Portfolio Building: Each project becomes a tangible demonstration of your skills, perfect for showcasing to potential employers.
- Motivation: Solving real problems provides a sense of accomplishment and keeps you motivated to learn more.
- Industry Relevance: It prepares you for the type of work you’ll encounter in professional settings, especially in FAANG-level companies.
Step 1: Identify a Real Problem
The first step in developing a project that solves real problems is, naturally, to identify a problem worth solving. Here’s how you can go about it:
1. Observe Your Surroundings
Look around your daily life, work, or community. What inefficiencies or pain points do you notice? For example, you might observe that local restaurants struggle with managing takeout orders efficiently.
2. Research Industry Challenges
Dive into industry reports, tech blogs, or forums to understand current challenges in various sectors. This can give you insights into problems that businesses are actively trying to solve.
3. Engage with Communities
Join online forums, attend local meetups, or participate in hackathons. These platforms often discuss real-world problems that need solutions.
4. Analyze Existing Solutions
Look at popular apps or services and think about how they could be improved. Sometimes, the best projects come from enhancing existing solutions.
Step 2: Define the Scope of Your Project
Once you’ve identified a problem, it’s crucial to define the scope of your project. This helps in keeping your project manageable and focused.
1. Set Clear Objectives
What exactly do you want your project to achieve? For instance, if you’re addressing the restaurant takeout problem, your objective might be “To create a mobile app that streamlines the takeout order process for small restaurants.”
2. Identify Key Features
List the essential features your project needs to solve the problem effectively. For our takeout app example, key features might include:
- User authentication
- Menu display
- Order placement
- Payment integration
- Order tracking
3. Determine Technical Requirements
Based on your features, decide on the technical stack you’ll need. This might include:
- Frontend framework (e.g., React Native for cross-platform mobile development)
- Backend language and framework (e.g., Node.js with Express)
- Database (e.g., MongoDB for flexible data storage)
- APIs (e.g., Stripe for payment processing)
4. Set Realistic Timelines
Break down your project into phases and set deadlines for each. Be realistic about what you can achieve, especially if you’re learning new technologies along the way.
Step 3: Plan Your Project
With a clear scope in mind, it’s time to plan your project in detail. This step is crucial for keeping your development process organized and efficient.
1. Create a Project Roadmap
Outline the major milestones of your project. For our takeout app, it might look like this:
- Research and Requirements Gathering
- UI/UX Design
- Frontend Development
- Backend Development
- Database Setup and Integration
- API Integrations
- Testing and Debugging
- Deployment
2. Break Down Tasks
For each milestone, break down the specific tasks needed. For example, under “Frontend Development,” you might have tasks like:
- Set up React Native project
- Create login and registration screens
- Develop menu browsing interface
- Implement shopping cart functionality
3. Choose Project Management Tools
Select tools to help you manage your project effectively. Some popular options include:
- Trello for kanban-style task management
- GitHub Projects for code-centric project tracking
- Jira for more complex project management needs
4. Set Up Version Control
Use Git for version control and host your repository on platforms like GitHub or GitLab. This not only helps in managing your code but also showcases your project to potential employers.
Step 4: Start Coding
Now comes the exciting part – bringing your project to life through code. Here’s how to approach the development phase:
1. Set Up Your Development Environment
Ensure you have all necessary tools and frameworks installed. For our takeout app example, you might need:
- Node.js and npm
- React Native CLI
- Android Studio or Xcode (depending on your target platform)
- A code editor like Visual Studio Code
2. Start with a Minimum Viable Product (MVP)
Begin by implementing the core features that solve the primary problem. For the takeout app, this might be:
- Basic user authentication
- Menu display
- Simple order placement
3. Follow Best Practices
As you code, keep these best practices in mind:
- Write clean, readable code with proper comments
- Follow the DRY (Don’t Repeat Yourself) principle
- Implement error handling and logging
- Write unit tests for critical functions
4. Leverage AlgoCademy Resources
Use AlgoCademy’s interactive tutorials and AI-powered assistance to overcome coding challenges. The platform’s focus on algorithmic thinking can be particularly helpful when implementing complex features.
5. Regular Commits and Pushes
Make frequent commits with meaningful messages and regularly push your code to your remote repository. This habit helps in tracking progress and reverting changes if needed.
Step 5: Test and Debug
Testing is a crucial phase that ensures your project functions as intended and solves the problem effectively.
1. Implement Different Types of Testing
- Unit Testing: Test individual components or functions. For example, test the function that calculates the total order price.
- Integration Testing: Ensure different parts of your application work together correctly, like the interaction between the order placement and payment processing.
- User Acceptance Testing (UAT): Have potential users test your app and provide feedback.
2. Use Testing Frameworks
Leverage testing frameworks appropriate for your tech stack. For a React Native app, you might use:
- Jest for unit and integration testing
- React Native Testing Library for component testing
- Detox for end-to-end testing
3. Debug Methodically
When you encounter bugs:
- Reproduce the issue consistently
- Use debugging tools (like Chrome DevTools for web or React Native Debugger)
- Log relevant information
- Isolate the problem
- Fix and verify the solution
4. Performance Testing
Ensure your application performs well under various conditions:
- Test with different network speeds
- Check memory usage and CPU load
- Verify responsiveness on different devices
Step 6: Deploy and Launch
With a well-tested project, you’re ready to deploy and launch your solution to the world.
1. Choose a Hosting Solution
Select an appropriate hosting platform. For a mobile app backend, you might consider:
- Heroku for easy deployment
- AWS Elastic Beanstalk for scalability
- Google Cloud Platform for its suite of tools
2. Set Up Continuous Integration/Continuous Deployment (CI/CD)
Implement CI/CD pipelines to automate testing and deployment. Tools like Jenkins, GitLab CI, or GitHub Actions can be invaluable here.
3. Prepare for App Store Submission
If you’re developing a mobile app:
- Prepare necessary assets (icons, screenshots)
- Write a compelling app description
- Ensure compliance with app store guidelines
4. Monitor and Gather Feedback
After launch:
- Use analytics tools to monitor usage and performance
- Gather user feedback through reviews or in-app surveys
- Be prepared to quickly address critical issues
Step 7: Iterate and Improve
Launching your project is just the beginning. Continuous improvement is key to long-term success.
1. Analyze User Feedback
Regularly review user feedback and analytics to understand:
- Which features are most used
- Common pain points or frustrations
- Suggestions for new features
2. Prioritize Enhancements
Based on feedback and your project goals, prioritize future enhancements. You might use a framework like the MoSCoW method (Must have, Should have, Could have, Won’t have) to categorize potential improvements.
3. Stay Updated with Technology
Keep your project up-to-date with the latest technologies and best practices. This might involve:
- Upgrading dependencies
- Refactoring code for better performance
- Implementing new security measures
4. Scale Your Solution
As your project grows, consider how to scale it effectively:
- Optimize database queries
- Implement caching mechanisms
- Consider microservices architecture for larger applications
Conclusion: The Journey of Continuous Learning
Developing projects that solve real problems is not just about creating a functional piece of software; it’s about embarking on a journey of continuous learning and improvement. Each project you undertake enhances your skills, challenges your thinking, and prepares you for the complex problems you’ll face in your career, especially if you’re aiming for positions at top tech companies.
Remember, the process of building such projects aligns perfectly with AlgoCademy’s approach to coding education. By focusing on algorithmic thinking and practical problem-solving, you’re not just learning to code – you’re learning to innovate and create solutions that can make a real difference.
As you progress through your projects, don’t hesitate to leverage AlgoCademy’s resources. The platform’s interactive tutorials, AI-powered assistance, and focus on preparing for technical interviews can be invaluable as you tackle complex coding challenges and aim to elevate your skills to the level expected by major tech companies.
Finally, celebrate your achievements along the way. Each problem you solve, each feature you implement, and each user you help is a testament to your growing skills and the real-world impact of your work. Keep pushing boundaries, stay curious, and remember that in the world of coding, learning truly never stops.
Now, armed with this guide and your coding skills, go forth and build something amazing that solves a real problem. The world is waiting for your innovations!