Learning Basic Syntax of a Coding Language: A Comprehensive Guide for Beginners
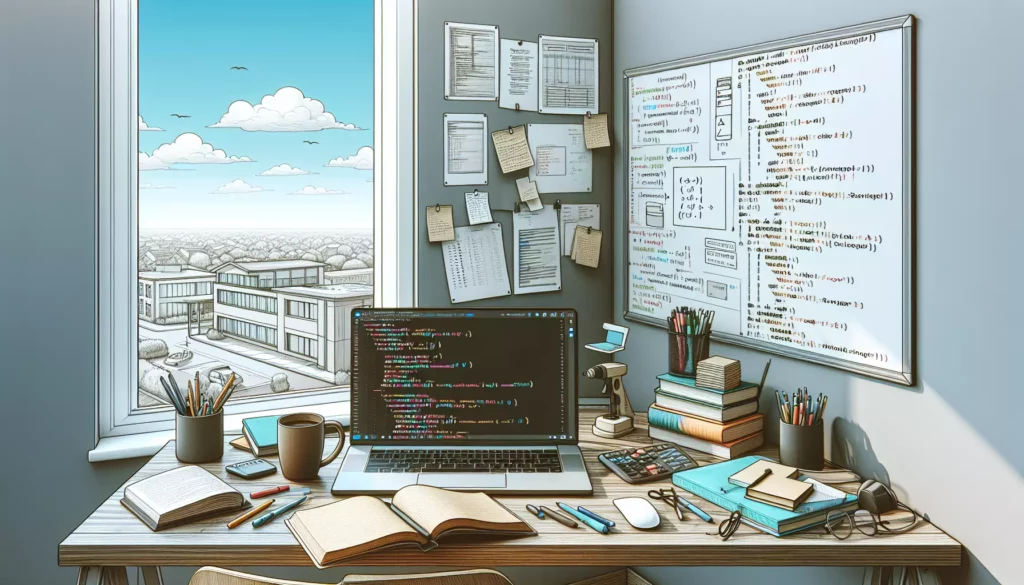
Embarking on your coding journey can be both exciting and daunting. One of the first hurdles you’ll encounter is learning the basic syntax of a programming language. Understanding syntax is crucial as it forms the foundation upon which you’ll build your coding skills. In this comprehensive guide, we’ll explore the fundamental concepts of coding syntax, using popular languages as examples, and provide you with practical tips to accelerate your learning process.
Table of Contents
- What is Syntax in Programming?
- The Importance of Proper Syntax
- Common Elements of Programming Syntax
- Syntax Examples in Popular Programming Languages
- Best Practices for Learning Syntax
- Common Syntax Mistakes and How to Avoid Them
- Tools and Resources for Mastering Syntax
- Moving Beyond the Basics
- Conclusion
What is Syntax in Programming?
In programming, syntax refers to the set of rules that define how programs written in a specific language should be structured. It’s akin to grammar in spoken languages – just as we need to follow grammatical rules to form coherent sentences, we must adhere to syntactical rules to write valid code that a computer can understand and execute.
Syntax encompasses various elements, including:
- Keywords and reserved words
- Operators
- Punctuation
- How to declare variables and functions
- How to structure control flow statements (if-else, loops, etc.)
- How to organize code into blocks
Each programming language has its own unique syntax, although many share similar concepts. Learning the syntax of one language can often make it easier to pick up others in the future.
The Importance of Proper Syntax
Mastering syntax is crucial for several reasons:
- Code Execution: Computers are precise machines. Even a small syntax error can prevent your entire program from running.
- Readability: Proper syntax makes your code easier to read and understand, both for yourself and for other developers who might work on your code.
- Efficiency: Understanding syntax allows you to write code more quickly and efficiently.
- Debugging: When you’re familiar with correct syntax, it becomes easier to spot and fix errors in your code.
- Foundation for Advanced Concepts: A solid grasp of basic syntax is essential for learning more complex programming concepts and techniques.
Common Elements of Programming Syntax
While syntax varies between languages, there are several common elements you’ll encounter in most programming languages:
1. Variables and Data Types
Variables are used to store data in your program. Most languages require you to declare variables before using them, often specifying the type of data they will hold.
// JavaScript example
let name = "John";
let age = 30;
let isStudent = true;
// Java example
String name = "John";
int age = 30;
boolean isStudent = true;
2. Operators
Operators are symbols that tell the compiler to perform specific mathematical or logical manipulations.
// Arithmetic operators
let sum = 5 + 3;
let difference = 10 - 4;
let product = 6 * 2;
let quotient = 15 / 3;
// Comparison operators
let isGreater = 5 > 3;
let isEqual = 4 == 4;
// Logical operators
let andResult = true && false;
let orResult = true || false;
3. Control Structures
Control structures allow you to control the flow of your program’s execution. Common structures include if-else statements, loops, and switch statements.
// If-else statement
if (age >= 18) {
console.log("You are an adult");
} else {
console.log("You are a minor");
}
// For loop
for (let i = 0; i < 5; i++) {
console.log(i);
}
// While loop
let count = 0;
while (count < 3) {
console.log(count);
count++;
}
4. Functions
Functions are reusable blocks of code that perform specific tasks. The syntax for declaring and calling functions varies between languages.
// JavaScript function declaration
function greet(name) {
return "Hello, " + name + "!";
}
// Calling the function
let message = greet("Alice");
console.log(message); // Outputs: Hello, Alice!
5. Comments
Comments are used to add explanations within your code. They are ignored by the compiler but can be very helpful for other developers (or yourself) when reading the code later.
// This is a single-line comment
/*
This is a
multi-line comment
*/
Syntax Examples in Popular Programming Languages
Let’s look at how basic syntax differs across some popular programming languages:
Python
Python is known for its clean, readable syntax. It uses indentation to define code blocks.
# Variable declaration
name = "John"
age = 30
# Function definition
def greet(name):
return f"Hello, {name}!"
# If statement
if age >= 18:
print("You are an adult")
else:
print("You are a minor")
# For loop
for i in range(5):
print(i)
# List comprehension
squares = [x**2 for x in range(10)]
JavaScript
JavaScript is widely used for web development. It uses curly braces to define code blocks.
// Variable declaration
let name = "John";
const age = 30;
// Function definition
function greet(name) {
return `Hello, ${name}!`;
}
// Arrow function
const square = (x) => x * x;
// If statement
if (age >= 18) {
console.log("You are an adult");
} else {
console.log("You are a minor");
}
// For loop
for (let i = 0; i < 5; i++) {
console.log(i);
}
// Array methods
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(x => x * 2);
Java
Java is an object-oriented language with a more verbose syntax. It requires explicit type declarations for variables.
public class Example {
public static void main(String[] args) {
// Variable declaration
String name = "John";
int age = 30;
// Method definition
public static String greet(String name) {
return "Hello, " + name + "!";
}
// If statement
if (age >= 18) {
System.out.println("You are an adult");
} else {
System.out.println("You are a minor");
}
// For loop
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
// ArrayList and lambda expression
ArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5));
numbers.forEach(n -> System.out.println(n * 2));
}
}
Best Practices for Learning Syntax
Learning the syntax of a programming language can be challenging, but there are several strategies you can employ to make the process smoother and more effective:
1. Start with One Language
While it’s tempting to try learning multiple languages at once, it’s generally more effective to focus on mastering the syntax of one language before moving on to others. This allows you to build a strong foundation and avoid confusion between different syntaxes.
2. Practice Consistently
Consistency is key when learning syntax. Set aside time each day to write code, even if it’s just for 15-30 minutes. Regular practice helps reinforce your understanding and builds muscle memory for common syntax patterns.
3. Use Interactive Tutorials
Many online platforms offer interactive coding tutorials that allow you to write and run code directly in your browser. These can be excellent for getting immediate feedback on your syntax and seeing the results of your code in real-time.
4. Read and Analyze Existing Code
Reading code written by experienced developers can help you understand how syntax is used in real-world applications. Look for open-source projects or code samples in documentation to study.
5. Use a Good Code Editor
Modern code editors and Integrated Development Environments (IDEs) often include features like syntax highlighting, auto-completion, and error detection. These tools can help you learn syntax more quickly and avoid common mistakes.
6. Write Comments
As you’re learning, get into the habit of writing comments that explain what your code is doing. This not only helps you understand your own code better but also reinforces your understanding of the syntax you’re using.
7. Build Small Projects
Apply your syntax knowledge by building small projects. This practical application helps solidify your understanding and shows you how different syntactical elements work together in a complete program.
Common Syntax Mistakes and How to Avoid Them
Even experienced programmers make syntax errors from time to time. Here are some common mistakes to watch out for:
1. Missing Semicolons
In languages that require semicolons at the end of statements (like JavaScript or Java), forgetting them is a common error.
// Incorrect
let x = 5
console.log(x)
// Correct
let x = 5;
console.log(x);
2. Mismatched Parentheses, Brackets, or Braces
Ensure that every opening parenthesis, bracket, or brace has a corresponding closing one.
// Incorrect
if (x == 5 {
console.log("x is 5");
// Correct
if (x == 5) {
console.log("x is 5");
}
3. Incorrect Indentation
In languages like Python where indentation is significant, incorrect indentation can change the meaning of your code or cause errors.
# Incorrect
if x > 5:
print("x is greater than 5")
# Correct
if x > 5:
print("x is greater than 5")
4. Case Sensitivity
Many programming languages are case-sensitive. ‘Variable’ and ‘variable’ are considered different identifiers.
// Incorrect
let myVariable = 10;
console.log(MyVariable);
// Correct
let myVariable = 10;
console.log(myVariable);
5. Using Assignment (=) Instead of Comparison (==)
This is a subtle but common mistake in conditional statements.
// Incorrect (assigns 5 to x)
if (x = 5) {
console.log("x is 5");
}
// Correct (compares x to 5)
if (x == 5) {
console.log("x is 5");
}
Tools and Resources for Mastering Syntax
There are numerous tools and resources available to help you master programming syntax:
1. Online Coding Platforms
- Codecademy: Offers interactive courses in various programming languages.
- freeCodeCamp: Provides free coding challenges and projects.
- LeetCode: Focuses on coding challenges, great for practicing syntax and problem-solving.
2. Documentation and Reference Guides
- Official language documentation (e.g., Python docs, MDN for JavaScript)
- W3Schools: Offers tutorials and references for various web technologies.
- DevDocs: Combines multiple API documentations in a fast, organized, and searchable interface.
3. Code Editors and IDEs
- Visual Studio Code: A popular, free, and highly customizable code editor.
- PyCharm: An IDE specifically designed for Python development.
- IntelliJ IDEA: A powerful IDE for Java development.
4. Version Control Systems
- Git: Essential for tracking changes in your code and collaborating with others.
- GitHub: A platform for hosting and sharing Git repositories.
5. Syntax Checkers and Linters
- ESLint: A pluggable linting utility for JavaScript.
- Pylint: A Python static code analysis tool.
6. Online Communities and Forums
- Stack Overflow: A question and answer site for programmers.
- Reddit programming communities: Subreddits like r/learnprogramming can be valuable resources.
Moving Beyond the Basics
Once you’ve grasped the basic syntax of a programming language, you’re ready to dive deeper into more advanced concepts. Here are some areas to explore:
1. Object-Oriented Programming (OOP)
Many modern programming languages support OOP, which is a programming paradigm based on the concept of “objects” that contain data and code. Understanding OOP concepts like classes, inheritance, and polymorphism can greatly enhance your coding skills.
2. Functional Programming
Functional programming is another paradigm that treats computation as the evaluation of mathematical functions. Languages like JavaScript, Python, and Scala support functional programming concepts.
3. Data Structures and Algorithms
Learning about data structures (like arrays, linked lists, trees) and algorithms (sorting, searching, graph algorithms) is crucial for writing efficient and scalable code.
4. API Integration
Many modern applications interact with external services through APIs. Learning how to work with APIs can open up a world of possibilities in your programming projects.
5. Database Management
Understanding how to interact with databases is essential for many types of applications. This includes learning SQL for relational databases and understanding NoSQL databases.
6. Web Development Frameworks
If you’re interested in web development, learning frameworks like React, Angular, or Django can help you build more complex and feature-rich web applications.
7. Version Control
While not strictly a programming concept, version control systems like Git are essential tools for any serious programmer. They allow you to track changes in your code, collaborate with others, and manage different versions of your project.
Conclusion
Learning the basic syntax of a programming language is the first step in your coding journey. It lays the foundation for everything else you’ll learn as a programmer. Remember that becoming proficient in a programming language takes time and practice. Don’t be discouraged if you find it challenging at first – every experienced programmer was once a beginner.
As you progress in your learning, you’ll find that understanding syntax becomes second nature, allowing you to focus more on problem-solving and creating innovative solutions. Keep practicing, stay curious, and don’t hesitate to seek help when you need it. The programming community is vast and generally very supportive of newcomers.
Remember, the goal isn’t just to memorize syntax, but to understand how to use it effectively to create functional and efficient programs. As you become more comfortable with the basics, challenge yourself with more complex projects and continue to explore new concepts and technologies.
Happy coding!