Learn Lua: A Comprehensive Guide for Beginners
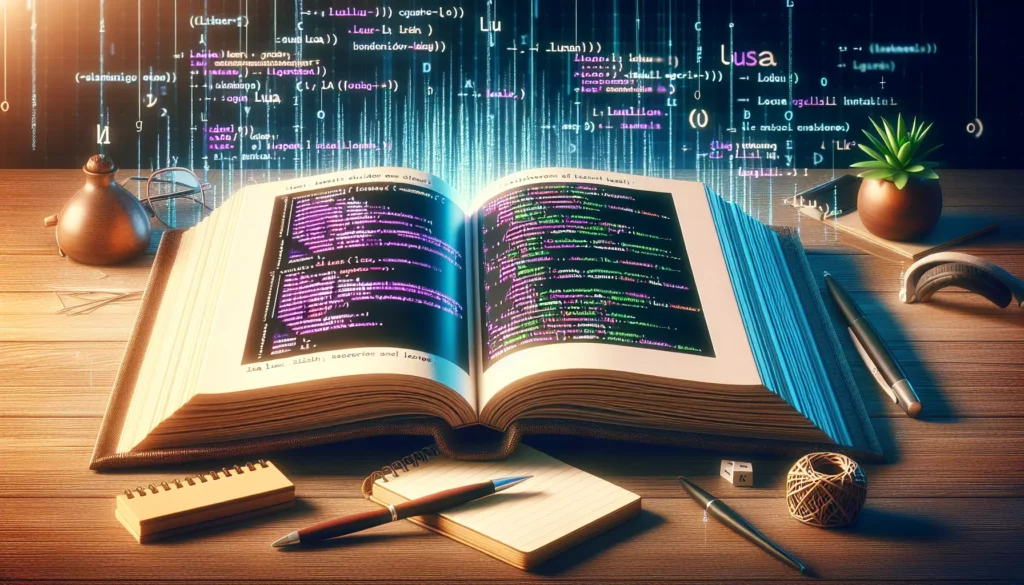
Introduction to Lua
Lua is a lightweight, high-level programming language designed for embedded use in applications. Created in 1993 by Roberto Ierusalimschy, Luiz Henrique de Figueiredo, and Waldemar Celes at the Pontifical Catholic University of Rio de Janeiro in Brazil, Lua has gained popularity due to its simplicity, efficiency, and ease of integration with other languages.
In this comprehensive guide, we’ll explore the fundamentals of Lua programming, its unique features, and how to get started with writing your first Lua scripts. Whether you’re a beginner programmer or an experienced developer looking to add another language to your toolkit, this article will provide you with a solid foundation in Lua.
Why Learn Lua?
Before diving into the language itself, let’s consider some compelling reasons to learn Lua:
- Simplicity: Lua has a clean and straightforward syntax, making it easy to learn and read.
- Portability: Lua can run on a wide range of platforms, from embedded systems to desktop computers.
- Efficiency: It’s known for its small footprint and fast execution, making it ideal for resource-constrained environments.
- Embeddability: Lua is designed to be embedded in other applications, allowing for powerful scripting capabilities.
- Versatility: It’s used in various domains, including game development, web applications, and scientific computing.
Setting Up Your Lua Environment
To start programming in Lua, you’ll need to set up your development environment. Here’s how to get started:
1. Installing Lua
You can download Lua from the official website (https://www.lua.org/download.html) or use package managers for your operating system:
- On Windows: Use a package manager like Chocolatey or download the binaries directly.
- On macOS: Use Homebrew with the command
brew install lua
. - On Linux: Use your distribution’s package manager, e.g.,
sudo apt-get install lua5.3
for Ubuntu.
2. Verifying the Installation
After installation, open a terminal or command prompt and type:
lua -v
This should display the version of Lua installed on your system.
3. Choosing an Editor
While you can write Lua code in any text editor, using an IDE or a code editor with Lua support can enhance your productivity. Some popular options include:
- Visual Studio Code with the Lua extension
- ZeroBrane Studio (a dedicated Lua IDE)
- Sublime Text with Lua packages
Lua Basics: Syntax and Data Types
Now that we have our environment set up, let’s explore the basic syntax and data types in Lua.
Comments
In Lua, you can add comments to your code using two dashes (–) for single-line comments or –[[ and ]] for multi-line comments:
-- This is a single-line comment
--[[
This is a
multi-line comment
]]
Variables and Data Types
Lua is dynamically typed, meaning you don’t need to declare the type of a variable explicitly. The basic data types in Lua are:
- nil: Represents the absence of a value
- boolean: true or false
- number: Represents both integer and floating-point numbers
- string: Sequence of characters
- function: First-class values in Lua
- table: The only data structuring mechanism in Lua
Here’s an example of declaring variables:
local name = "John Doe" -- string
local age = 30 -- number
local is_student = false -- boolean
local data = nil -- nil
print(type(name)) -- Output: string
print(type(age)) -- Output: number
print(type(is_student)) -- Output: boolean
print(type(data)) -- Output: nil
Basic Operators
Lua supports various operators for arithmetic, comparison, and logical operations:
-- Arithmetic operators
local sum = 5 + 3
local difference = 10 - 4
local product = 6 * 7
local quotient = 20 / 4
local exponent = 2 ^ 3
local modulo = 10 % 3
-- Comparison operators
local is_equal = (5 == 5)
local is_not_equal = (5 ~= 6)
local is_greater = (10 > 5)
local is_less = (3 < 7)
local is_greater_or_equal = (5 >= 5)
local is_less_or_equal = (4 <= 4)
-- Logical operators
local and_result = true and false
local or_result = true or false
local not_result = not true
Control Structures in Lua
Lua provides several control structures to manage the flow of your program.
If-Else Statements
The if-else statement allows you to execute code based on conditions:
local age = 18
if age >= 18 then
print("You are an adult")
elseif age >= 13 then
print("You are a teenager")
else
print("You are a child")
end
Loops
Lua offers several types of loops:
While Loop
local count = 0
while count < 5 do
print(count)
count = count + 1
end
Repeat-Until Loop
local count = 0
repeat
print(count)
count = count + 1
until count >= 5
Numeric For Loop
for i = 1, 5 do
print(i)
end
-- With step value
for i = 10, 1, -2 do
print(i)
end
Generic For Loop
This type of loop is used to iterate over tables:
local fruits = {"apple", "banana", "orange"}
for index, value in ipairs(fruits) do
print(index, value)
end
Functions in Lua
Functions are first-class citizens in Lua, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
Defining and Calling Functions
-- Basic function definition
function greet(name)
print("Hello, " .. name .. "!")
end
-- Calling the function
greet("Alice") -- Output: Hello, Alice!
-- Function with return value
function add(a, b)
return a + b
end
local result = add(3, 4)
print(result) -- Output: 7
-- Anonymous functions
local multiply = function(a, b)
return a * b
end
print(multiply(5, 6)) -- Output: 30
Variable Arguments
Lua functions can accept a variable number of arguments using the ...
syntax:
function sum(...)
local total = 0
for _, value in ipairs({...}) do
total = total + value
end
return total
end
print(sum(1, 2, 3, 4, 5)) -- Output: 15
Tables: Lua’s Versatile Data Structure
Tables are the only data structuring mechanism in Lua. They can be used to represent arrays, dictionaries, objects, and more.
Creating and Using Tables
-- Creating a simple table
local fruits = {"apple", "banana", "orange"}
-- Accessing elements
print(fruits[1]) -- Output: apple (Note: Lua arrays are 1-indexed)
-- Adding elements
fruits[4] = "grape"
-- Using tables as dictionaries
local person = {
name = "John Doe",
age = 30,
is_student = false
}
print(person.name) -- Output: John Doe
print(person["age"]) -- Output: 30
-- Nested tables
local matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
}
print(matrix[2][3]) -- Output: 6
Table Functions
Lua provides several built-in functions for working with tables:
local numbers = {10, 20, 30, 40, 50}
-- Insert an element
table.insert(numbers, 60)
-- Remove an element
table.remove(numbers, 1)
-- Get the length of a table
print(#numbers) -- Output: 5
-- Concatenate table elements into a string
print(table.concat(numbers, ", ")) -- Output: 20, 30, 40, 50, 60
-- Sort a table
table.sort(numbers)
print(table.concat(numbers, ", ")) -- Output: 20, 30, 40, 50, 60
Modules and Packages
Lua allows you to organize your code into modules, which can be reused across different scripts.
Creating a Module
Create a file named mymodule.lua
:
local mymodule = {}
function mymodule.greet(name)
return "Hello, " .. name .. "!"
end
function mymodule.farewell(name)
return "Goodbye, " .. name .. "!"
end
return mymodule
Using a Module
In another Lua script, you can use the module like this:
local mymodule = require("mymodule")
print(mymodule.greet("Alice")) -- Output: Hello, Alice!
print(mymodule.farewell("Bob")) -- Output: Goodbye, Bob!
Error Handling in Lua
Lua provides mechanisms for handling errors and exceptions in your code.
pcall (Protected Call)
The pcall
function calls a given function in protected mode, catching any errors that occur:
local function divide(a, b)
if b == 0 then
error("Division by zero")
end
return a / b
end
local success, result = pcall(divide, 10, 2)
if success then
print("Result:", result) -- Output: Result: 5
else
print("Error:", result)
end
success, result = pcall(divide, 10, 0)
if success then
print("Result:", result)
else
print("Error:", result) -- Output: Error: Division by zero
end
xpcall and Debug Library
For more advanced error handling, you can use xpcall
along with the debug library:
local function error_handler(err)
print("Error occurred: " .. err)
print(debug.traceback())
end
local function problematic_function()
error("Something went wrong")
end
xpcall(problematic_function, error_handler)
Object-Oriented Programming in Lua
While Lua doesn’t have built-in support for classes and objects, you can implement object-oriented programming using tables and metatables.
Creating a Simple Class
local Person = {}
Person.__index = Person
function Person.new(name, age)
local self = setmetatable({}, Person)
self.name = name
self.age = age
return self
end
function Person:introduce()
print("Hello, my name is " .. self.name .. " and I'm " .. self.age .. " years old.")
end
-- Creating an instance
local john = Person.new("John Doe", 30)
john:introduce() -- Output: Hello, my name is John Doe and I'm 30 years old.
Inheritance
You can implement inheritance using Lua’s prototype-based approach:
local Student = {}
setmetatable(Student, {__index = Person})
function Student.new(name, age, school)
local self = Person.new(name, age)
setmetatable(self, {__index = Student})
self.school = school
return self
end
function Student:introduce()
Person.introduce(self)
print("I'm a student at " .. self.school .. ".")
end
-- Creating a Student instance
local alice = Student.new("Alice Smith", 20, "University of Lua")
alice:introduce()
-- Output:
-- Hello, my name is Alice Smith and I'm 20 years old.
-- I'm a student at University of Lua.
Lua Standard Libraries
Lua comes with several standard libraries that provide useful functions for common tasks:
String Manipulation
local str = "Hello, World!"
print(string.upper(str)) -- Output: HELLO, WORLD!
print(string.lower(str)) -- Output: hello, world!
print(string.sub(str, 1, 5)) -- Output: Hello
print(string.find(str, "World")) -- Output: 8 12
print(string.gsub(str, "World", "Lua")) -- Output: Hello, Lua! 1
Math Functions
print(math.abs(-5)) -- Output: 5
print(math.ceil(3.2)) -- Output: 4
print(math.floor(3.8)) -- Output: 3
print(math.max(1, 2, 3, 4, 5)) -- Output: 5
print(math.min(1, 2, 3, 4, 5)) -- Output: 1
print(math.pi) -- Output: 3.1415926535898
Input/Output Operations
-- Writing to a file
local file = io.open("example.txt", "w")
file:write("Hello, Lua!")
file:close()
-- Reading from a file
file = io.open("example.txt", "r")
local content = file:read("*all")
file:close()
print(content) -- Output: Hello, Lua!
-- Reading user input
print("Enter your name:")
local name = io.read()
print("Hello, " .. name .. "!")
Conclusion
This comprehensive guide has introduced you to the fundamental concepts of Lua programming. We’ve covered everything from basic syntax and data types to more advanced topics like object-oriented programming and error handling. Lua’s simplicity, efficiency, and versatility make it an excellent choice for various applications, from game development to embedded systems.
As you continue your journey with Lua, consider exploring these additional resources:
- The official Lua documentation (https://www.lua.org/docs.html)
- Programming in Lua, a book by Roberto Ierusalimschy, one of Lua’s creators
- Lua users community and mailing list (https://www.lua.org/community.html)
- LuaRocks, a package manager for Lua modules
Remember that the best way to learn programming is through practice. Start working on small projects, experiment with different features, and don’t hesitate to consult the documentation and community resources when you encounter challenges. Happy coding with Lua!