Key Git Commands Every Developer Should Know
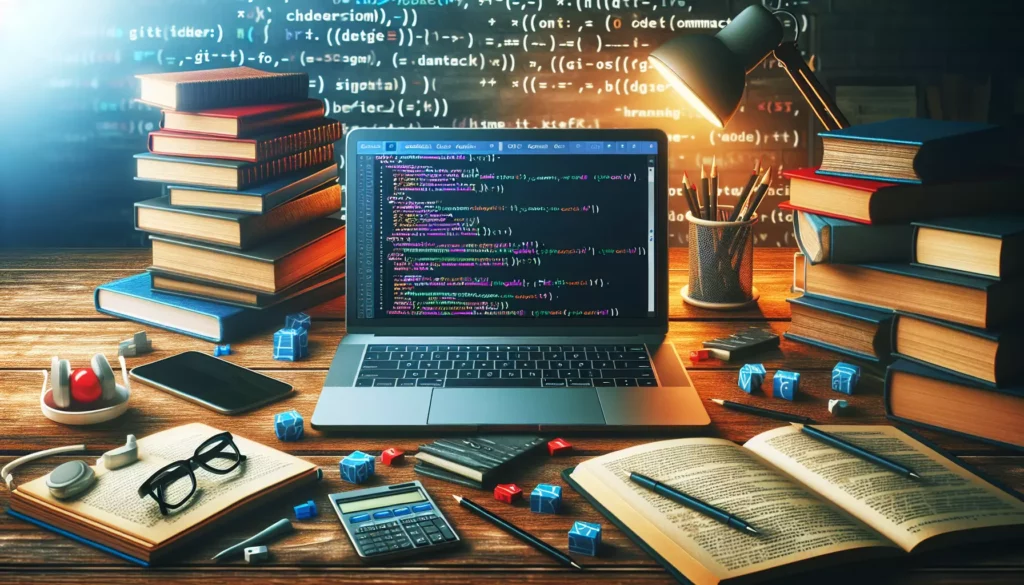
In the world of software development, version control is an essential skill that every programmer must master. Git, the distributed version control system created by Linus Torvalds, has become the industry standard for managing code repositories and collaborating on projects. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding and effectively using Git commands is crucial.
In this comprehensive guide, we’ll explore the most important Git commands that every developer should know. We’ll cover everything from basic operations to more advanced techniques, providing you with the knowledge you need to manage your projects efficiently and collaborate effectively with other developers.
1. Git Configuration
Before diving into Git commands, it’s essential to set up your Git environment. These configuration commands help you personalize your Git experience:
1.1. Set Your Name and Email
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
These commands set your name and email globally, which will be associated with all your Git commits.
1.2. Check Your Configuration
git config --list
This command displays all your current Git configuration settings.
2. Repository Initialization and Cloning
To start using Git, you need to either initialize a new repository or clone an existing one.
2.1. Initialize a New Repository
git init
This command creates a new Git repository in the current directory.
2.2. Clone an Existing Repository
git clone <repository-url>
Use this command to create a local copy of a remote repository.
3. Basic Git Workflow Commands
These commands form the core of the Git workflow and are used frequently in day-to-day development.
3.1. Check Status
git status
This command shows the current state of your working directory and staging area.
3.2. Add Files to Staging Area
git add <file>
Use this to add specific files to the staging area.
git add .
This command adds all modified and new files to the staging area.
3.3. Commit Changes
git commit -m "Your commit message"
This command creates a new commit with the changes in the staging area.
3.4. Push Changes to Remote Repository
git push origin <branch-name>
Use this command to upload your local commits to a remote repository.
3.5. Pull Changes from Remote Repository
git pull origin <branch-name>
This command fetches and merges changes from the remote repository to your local branch.
4. Branching and Merging
Branching is a powerful feature in Git that allows developers to work on different features or experiments without affecting the main codebase.
4.1. Create a New Branch
git branch <branch-name>
This command creates a new branch but doesn’t switch to it.
4.2. Switch to a Branch
git checkout <branch-name>
Use this command to switch to an existing branch.
4.3. Create and Switch to a New Branch
git checkout -b <branch-name>
This command creates a new branch and immediately switches to it.
4.4. List All Branches
git branch
This command shows a list of all local branches.
4.5. Merge Branches
git merge <branch-name>
Use this command to merge changes from the specified branch into the current branch.
4.6. Delete a Branch
git branch -d <branch-name>
This command deletes a local branch after it has been merged.
git branch -D <branch-name>
Use this command to force delete a branch, even if it hasn’t been merged.
5. Viewing and Comparing Changes
Git provides several commands to inspect and compare changes in your repository.
5.1. View Commit History
git log
This command displays the commit history for the current branch.
5.2. View Changes in Working Directory
git diff
Use this command to see unstaged changes in your working directory.
5.3. View Changes in Staging Area
git diff --staged
This command shows the changes that have been staged and are ready to be committed.
5.4. View Changes Between Commits
git diff <commit1> <commit2>
Use this command to compare changes between two specific commits.
6. Undoing Changes
Sometimes you need to undo changes or revert to a previous state. Git provides several commands for this purpose.
6.1. Discard Changes in Working Directory
git checkout -- <file>
This command discards changes to a specific file in the working directory.
6.2. Unstage Changes
git reset HEAD <file>
Use this command to remove a file from the staging area while keeping the changes in your working directory.
6.3. Amend the Last Commit
git commit --amend
This command allows you to modify the most recent commit, adding new changes or updating the commit message.
6.4. Revert a Commit
git revert <commit-hash>
Use this command to create a new commit that undoes the changes made in a specific commit.
6.5. Reset to a Previous Commit
git reset --hard <commit-hash>
This command resets your branch to a specific commit, discarding all subsequent commits. Use with caution!
7. Remote Repository Operations
Working with remote repositories is crucial for collaboration and project management.
7.1. Add a Remote Repository
git remote add <name> <url>
This command adds a new remote repository to your local Git configuration.
7.2. List Remote Repositories
git remote -v
Use this command to list all remote repositories associated with your local repository.
7.3. Fetch Changes from Remote
git fetch <remote>
This command downloads objects and refs from the remote repository without merging them.
7.4. Push to Remote Repository
git push <remote> <branch>
Use this command to upload your local branch commits to a remote repository.
7.5. Remove a Remote Repository
git remote remove <name>
This command removes a remote repository from your local Git configuration.
8. Stashing Changes
Stashing allows you to temporarily save changes that you’re not ready to commit.
8.1. Stash Changes
git stash
This command saves your current changes and reverts your working directory to the last commit.
8.2. List Stashes
git stash list
Use this command to see all your saved stashes.
8.3. Apply a Stash
git stash apply
This command applies the most recent stash to your working directory.
8.4. Apply a Specific Stash
git stash apply stash@{n}
Use this command to apply a specific stash, where n is the stash index.
8.5. Remove a Stash
git stash drop stash@{n}
This command removes a specific stash from your stash list.
9. Tagging
Tags are used to mark specific points in Git history, typically for release versions.
9.1. Create a Lightweight Tag
git tag <tag-name>
This command creates a lightweight tag at the current commit.
9.2. Create an Annotated Tag
git tag -a <tag-name> -m "Tag message"
Use this command to create an annotated tag with a message.
9.3. List Tags
git tag
This command lists all tags in the repository.
9.4. Push Tags to Remote
git push origin --tags
Use this command to push all tags to the remote repository.
10. Advanced Git Commands
These advanced commands are useful for more complex Git operations and can significantly enhance your workflow.
10.1. Interactive Rebase
git rebase -i <commit-hash>
This command allows you to modify, squash, or reorder commits interactively.
10.2. Cherry-pick
git cherry-pick <commit-hash>
Use this command to apply the changes from a specific commit to your current branch.
10.3. Reflog
git reflog
This command shows a log of all reference updates in the repository, useful for recovering lost commits.
10.4. Bisect
git bisect start
git bisect bad
git bisect good <commit-hash>
These commands start a binary search to find the commit that introduced a bug.
Conclusion
Mastering these Git commands is essential for any developer looking to excel in their career, especially when preparing for technical interviews at top tech companies. Git’s power lies not just in its ability to track changes, but in how it facilitates collaboration, experimentation, and code management at scale.
As you progress in your coding journey, make it a habit to use these commands regularly. Practice creating branches for new features, merging changes, and resolving conflicts. Experiment with more advanced features like rebasing and cherry-picking to understand how they can streamline your workflow.
Remember, Git is a powerful tool, and with great power comes great responsibility. Always be cautious when using commands that can alter history or discard changes. It’s a good practice to regularly push your changes to a remote repository and to create backups before performing major operations.
By incorporating these Git commands into your daily development routine, you’ll not only improve your version control skills but also enhance your overall productivity as a developer. Whether you’re working on personal projects, contributing to open-source initiatives, or collaborating in a professional setting, a solid understanding of Git will serve you well throughout your programming career.
Keep practicing, stay curious, and don’t be afraid to explore Git’s more advanced features. With time and experience, you’ll find that Git becomes an indispensable tool in your development arsenal, helping you manage your code with confidence and efficiency.