Key Differences Between Syntax in Popular Programming Languages
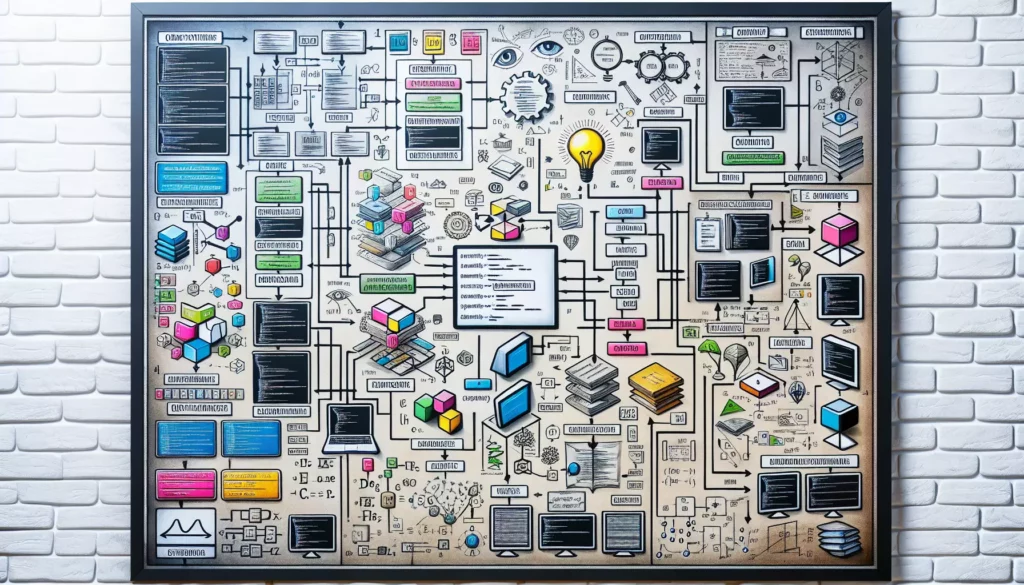
Programming languages are the backbone of software development, each with its unique syntax and features. Understanding the key differences between these languages is crucial for aspiring developers and those looking to expand their coding repertoire. In this comprehensive guide, we’ll explore the syntax variations among popular programming languages, helping you navigate the diverse landscape of coding.
1. Variable Declaration and Data Types
One of the fundamental aspects of any programming language is how it handles variable declarations and data types. Let’s compare some popular languages:
Python
Python uses dynamic typing, which means you don’t need to declare variable types explicitly:
x = 5 # Integer
y = "Hello" # String
z = 3.14 # Float
Java
Java, on the other hand, is statically typed and requires explicit type declarations:
int x = 5;
String y = "Hello";
double z = 3.14;
JavaScript
JavaScript is dynamically typed like Python, but uses different keywords for variable declaration:
let x = 5; // Can be reassigned
const y = "Hello"; // Cannot be reassigned
var z = 3.14; // Old-style declaration, function-scoped
C++
C++ is statically typed and requires explicit type declarations:
int x = 5;
std::string y = "Hello";
double z = 3.14;
2. Function Definitions
The syntax for defining functions varies significantly across languages:
Python
def greet(name):
return f"Hello, {name}!"
Java
public static String greet(String name) {
return "Hello, " + name + "!";
}
JavaScript
function greet(name) {
return `Hello, ${name}!`;
}
// Arrow function syntax
const greet = (name) => `Hello, ${name}!`;
C++
std::string greet(std::string name) {
return "Hello, " + name + "!";
}
3. Control Structures
Control structures like loops and conditionals are essential in programming. Let’s compare their syntax:
If-Else Statements
Python
if x > 0:
print("Positive")
elif x < 0:
print("Negative")
else:
print("Zero")
Java
if (x > 0) {
System.out.println("Positive");
} else if (x < 0) {
System.out.println("Negative");
} else {
System.out.println("Zero");
}
JavaScript
if (x > 0) {
console.log("Positive");
} else if (x < 0) {
console.log("Negative");
} else {
console.log("Zero");
}
C++
if (x > 0) {
std::cout << "Positive" << std::endl;
} else if (x < 0) {
std::cout << "Negative" << std::endl;
} else {
std::cout << "Zero" << std::endl;
}
For Loops
Python
for i in range(5):
print(i)
Java
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
JavaScript
for (let i = 0; i < 5; i++) {
console.log(i);
}
C++
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
4. Object-Oriented Programming (OOP)
OOP is a crucial paradigm in modern programming. Let’s see how different languages implement classes and objects:
Python
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says Woof!"
my_dog = Dog("Buddy")
print(my_dog.bark()) # Output: Buddy says Woof!
Java
public class Dog {
private String name;
public Dog(String name) {
this.name = name;
}
public String bark() {
return this.name + " says Woof!";
}
}
Dog myDog = new Dog("Buddy");
System.out.println(myDog.bark()); // Output: Buddy says Woof!
JavaScript
class Dog {
constructor(name) {
this.name = name;
}
bark() {
return `${this.name} says Woof!`;
}
}
const myDog = new Dog("Buddy");
console.log(myDog.bark()); // Output: Buddy says Woof!
C++
class Dog {
private:
std::string name;
public:
Dog(std::string name) : name(name) {}
std::string bark() {
return name + " says Woof!";
}
};
Dog myDog("Buddy");
std::cout << myDog.bark() << std::endl; // Output: Buddy says Woof!
5. Error Handling
Error handling is crucial for writing robust code. Let’s compare exception handling across languages:
Python
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
finally:
print("This always executes")
Java
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
} finally {
System.out.println("This always executes");
}
JavaScript
try {
let result = 10 / 0;
} catch (error) {
console.log("An error occurred:", error.message);
} finally {
console.log("This always executes");
}
C++
#include <stdexcept>
try {
throw std::runtime_error("Cannot divide by zero!");
} catch (const std::exception& e) {
std::cout << "Caught exception: " << e.what() << std::endl;
}
6. Array and List Manipulation
Working with collections of data is a common task in programming. Let’s compare array and list syntax:
Python (Lists)
numbers = [1, 2, 3, 4, 5]
numbers.append(6)
print(len(numbers)) # Output: 6
print(numbers[2]) # Output: 3
Java (Arrays and ArrayLists)
// Array
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(numbers.length); // Output: 5
System.out.println(numbers[2]); // Output: 3
// ArrayList
import java.util.ArrayList;
ArrayList<Integer> numberList = new ArrayList<>();
numberList.add(1);
numberList.add(2);
System.out.println(numberList.size()); // Output: 2
System.out.println(numberList.get(1)); // Output: 2
JavaScript (Arrays)
let numbers = [1, 2, 3, 4, 5];
numbers.push(6);
console.log(numbers.length); // Output: 6
console.log(numbers[2]); // Output: 3
C++ (Arrays and Vectors)
#include <vector>
// Array
int numbers[] = {1, 2, 3, 4, 5};
std::cout << sizeof(numbers) / sizeof(numbers[0]) << std::endl; // Output: 5
std::cout << numbers[2] << std::endl; // Output: 3
// Vector
std::vector<int> numberVector = {1, 2, 3, 4, 5};
numberVector.push_back(6);
std::cout << numberVector.size() << std::endl; // Output: 6
std::cout << numberVector[2] << std::endl; // Output: 3
7. String Manipulation
String handling is another crucial aspect of programming. Let’s compare string operations:
Python
text = "Hello, World!"
print(text.upper()) # Output: HELLO, WORLD!
print(text.split(",")) # Output: ['Hello', ' World!']
print(f"The length is {len(text)}") # Output: The length is 13
Java
String text = "Hello, World!";
System.out.println(text.toUpperCase()); // Output: HELLO, WORLD!
System.out.println(Arrays.toString(text.split(","))); // Output: [Hello, World!]
System.out.println("The length is " + text.length()); // Output: The length is 13
JavaScript
let text = "Hello, World!";
console.log(text.toUpperCase()); // Output: HELLO, WORLD!
console.log(text.split(",")); // Output: ['Hello', ' World!']
console.log(`The length is ${text.length}`); // Output: The length is 13
C++
#include <string>
#include <algorithm>
#include <sstream>
#include <vector>
std::string text = "Hello, World!";
std::transform(text.begin(), text.end(), text.begin(), ::toupper);
std::cout << text << std::endl; // Output: HELLO, WORLD!
std::vector<std::string> result;
std::istringstream iss(text);
std::string token;
while (std::getline(iss, token, ',')) {
result.push_back(token);
}
// result now contains ["HELLO", " WORLD!"]
std::cout << "The length is " << text.length() << std::endl; // Output: The length is 13
8. File I/O
File input/output operations are essential for data persistence and processing. Let’s compare file handling:
Python
# Writing to a file
with open("example.txt", "w") as file:
file.write("Hello, World!")
# Reading from a file
with open("example.txt", "r") as file:
content = file.read()
print(content) # Output: Hello, World!
Java
import java.io.*;
// Writing to a file
try (FileWriter writer = new FileWriter("example.txt")) {
writer.write("Hello, World!");
} catch (IOException e) {
e.printStackTrace();
}
// Reading from a file
try (BufferedReader reader = new BufferedReader(new FileReader("example.txt"))) {
String line = reader.readLine();
System.out.println(line); // Output: Hello, World!
} catch (IOException e) {
e.printStackTrace();
}
JavaScript (Node.js)
const fs = require('fs');
// Writing to a file
fs.writeFile('example.txt', 'Hello, World!', (err) => {
if (err) throw err;
console.log('File has been saved!');
});
// Reading from a file
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data); // Output: Hello, World!
});
C++
#include <fstream>
#include <string>
// Writing to a file
std::ofstream outFile("example.txt");
if (outFile.is_open()) {
outFile << "Hello, World!";
outFile.close();
}
// Reading from a file
std::string line;
std::ifstream inFile("example.txt");
if (inFile.is_open()) {
std::getline(inFile, line);
std::cout << line << std::endl; // Output: Hello, World!
inFile.close();
}
9. Lambda Functions (Anonymous Functions)
Lambda functions provide a concise way to create small, anonymous functions. Let’s compare their syntax:
Python
square = lambda x: x ** 2
print(square(5)) # Output: 25
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Java (Java 8+)
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
interface Square {
int calculate(int x);
}
public class LambdaExample {
public static void main(String[] args) {
Square square = (int x) -> x * x;
System.out.println(square.calculate(5)); // Output: 25
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = numbers.stream()
.map(x -> x * x)
.collect(Collectors.toList());
System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
}
}
JavaScript
const square = x => x ** 2;
console.log(square(5)); // Output: 25
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(x => x ** 2);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
C++ (C++11 and later)
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
auto square = [](int x) { return x * x; };
std::cout << square(5) << std::endl; // Output: 25
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::vector<int> squaredNumbers;
std::transform(numbers.begin(), numbers.end(), std::back_inserter(squaredNumbers),
[](int x) { return x * x; });
for (int num : squaredNumbers) {
std::cout << num << " "; // Output: 1 4 9 16 25
}
std::cout << std::endl;
return 0;
}
10. Modules and Imports
Organizing code into modules and importing functionality is crucial for maintaining large codebases. Let’s compare how different languages handle this:
Python
# In math_operations.py
def add(a, b):
return a + b
def multiply(a, b):
return a * b
# In main.py
import math_operations
result = math_operations.add(5, 3)
print(result) # Output: 8
# Alternatively, you can import specific functions
from math_operations import multiply
result = multiply(4, 2)
print(result) # Output: 8
Java
// In MathOperations.java
package com.example;
public class MathOperations {
public static int add(int a, int b) {
return a + b;
}
public static int multiply(int a, int b) {
return a * b;
}
}
// In Main.java
import com.example.MathOperations;
public class Main {
public static void main(String[] args) {
int result = MathOperations.add(5, 3);
System.out.println(result); // Output: 8
}
}
JavaScript (Node.js)
// In math_operations.js
exports.add = function(a, b) {
return a + b;
};
exports.multiply = function(a, b) {
return a * b;
};
// In main.js
const mathOps = require('./math_operations');
let result = mathOps.add(5, 3);
console.log(result); // Output: 8
// Using ES6 modules (needs to be supported by your environment)
// In math_operations.mjs
export function add(a, b) {
return a + b;
}
export function multiply(a, b) {
return a * b;
}
// In main.mjs
import { add, multiply } from './math_operations.mjs';
let result = add(5, 3);
console.log(result); // Output: 8
C++
// In math_operations.h
#ifndef MATH_OPERATIONS_H
#define MATH_OPERATIONS_H
namespace math_operations {
int add(int a, int b);
int multiply(int a, int b);
}
#endif
// In math_operations.cpp
#include "math_operations.h"
namespace math_operations {
int add(int a, int b) {
return a + b;
}
int multiply(int a, int b) {
return a * b;
}
}
// In main.cpp
#include <iostream>
#include "math_operations.h"
int main() {
int result = math_operations::add(5, 3);
std::cout << result << std::endl; // Output: 8
return 0;
}
Conclusion
Understanding the syntax differences between popular programming languages is crucial for developers, especially those working in multi-language environments or transitioning between languages. While this guide covers many key aspects, it’s important to note that each language has its own unique features, idioms, and best practices that go beyond basic syntax.
As you continue your journey in programming, remember that mastering a language involves more than just syntax. It requires understanding the language’s philosophy, ecosystem, and common design patterns. Platforms like AlgoCademy can be invaluable in this learning process, offering interactive tutorials and resources to help you deepen your understanding of various programming languages and prepare for technical interviews.
Whether you’re a beginner starting with your first language or an experienced developer expanding your skill set, the ability to compare and contrast different programming languages will make you a more versatile and effective programmer. Keep practicing, stay curious, and don’t hesitate to explore the unique strengths of each language you encounter.