Kadane’s Algorithm: Mastering the Maximum Subarray Problem
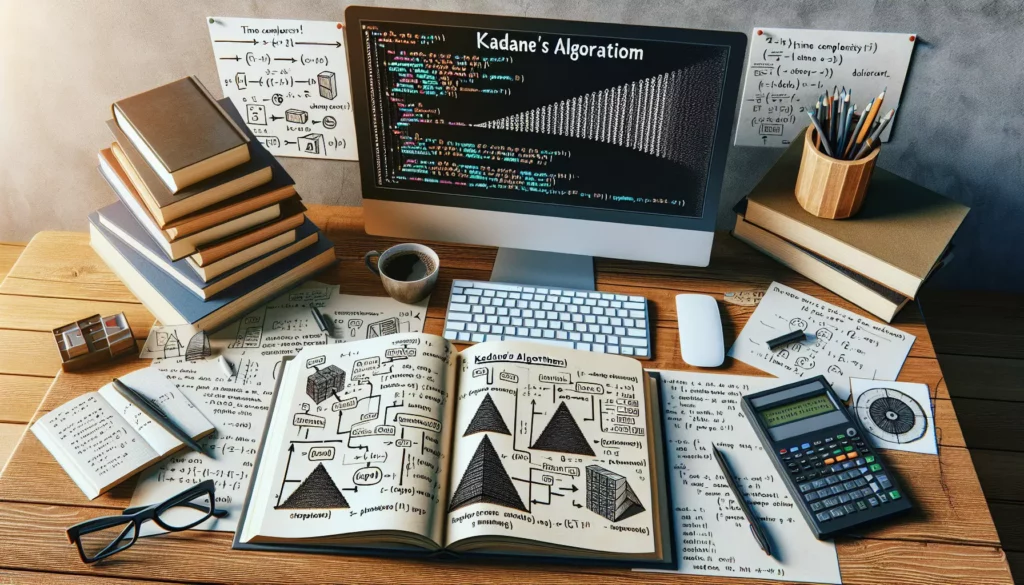
In the world of competitive programming and technical interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), certain algorithmic problems stand out as classics. One such problem is the Maximum Subarray Problem, which can be elegantly solved using Kadane’s Algorithm. This blog post will dive deep into Kadane’s Algorithm, exploring its implementation, time complexity, and various applications.
What is the Maximum Subarray Problem?
Before we delve into Kadane’s Algorithm, let’s first understand the problem it solves. The Maximum Subarray Problem is defined as follows:
Given an array of integers, find the contiguous subarray with the largest sum.
For example, given the array [-2, 1, -3, 4, -1, 2, 1, -5, 4], the contiguous subarray with the largest sum is [4, -1, 2, 1], which sums to 6.
This problem has applications in various fields, including:
- Financial analysis for finding the most profitable period
- Image processing for identifying areas of interest
- Bioinformatics for analyzing DNA sequences
Naive Approach: Brute Force
Before introducing Kadane’s Algorithm, let’s consider a brute force approach to solve this problem. The naive solution would involve checking all possible subarrays and keeping track of the maximum sum encountered. Here’s a Python implementation of this approach:
def max_subarray_brute_force(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result = max_subarray_brute_force(arr)
print(f"Maximum subarray sum: {result}")
While this approach works, it has a time complexity of O(n³), making it inefficient for large arrays. This is where Kadane’s Algorithm comes in, offering a much more efficient solution.
Enter Kadane’s Algorithm
Kadane’s Algorithm, named after its creator Joseph Born Kadane, is a dynamic programming approach to solve the Maximum Subarray Problem. The algorithm works by maintaining two variables:
- max_ending_here: The maximum sum contiguous subarray ending at the current position.
- max_so_far: The maximum sum of contiguous subarray found so far.
The key insight of Kadane’s Algorithm is that the maximum subarray ending at each position is either:
- The current element itself, or
- The current element plus the maximum subarray ending at the previous position
This insight allows us to solve the problem in a single pass through the array, resulting in a time complexity of O(n).
Implementation of Kadane’s Algorithm
Here’s a Python implementation of Kadane’s Algorithm:
def kadanes_algorithm(arr):
max_ending_here = max_so_far = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result = kadanes_algorithm(arr)
print(f"Maximum subarray sum: {result}")
Step-by-Step Explanation
Let’s break down how Kadane’s Algorithm works step by step:
- Initialize both
max_ending_here
andmax_so_far
with the first element of the array. - Iterate through the array starting from the second element:
- For each element, update
max_ending_here
:- If adding the current element to
max_ending_here
results in a larger sum, keep the sum. - Otherwise, start a new subarray from the current element.
- If adding the current element to
- Update
max_so_far
ifmax_ending_here
is greater. - After the iteration,
max_so_far
will contain the maximum subarray sum.
Time and Space Complexity
Kadane’s Algorithm has the following complexities:
- Time Complexity: O(n), where n is the length of the input array. We only need to iterate through the array once.
- Space Complexity: O(1), as we only use two variables regardless of the input size.
This significant improvement over the brute force approach makes Kadane’s Algorithm an excellent choice for solving the Maximum Subarray Problem, especially when dealing with large datasets.
Variations and Extensions of Kadane’s Algorithm
While the basic version of Kadane’s Algorithm solves the Maximum Subarray Problem efficiently, there are several variations and extensions worth exploring. These variations can help solve related problems or handle special cases.
1. Handling All Negative Numbers
The basic implementation of Kadane’s Algorithm assumes that there is at least one positive number in the array. If all numbers are negative, it will return the largest negative number. To handle this case, we can modify the algorithm slightly:
def kadanes_algorithm_all_negative(arr):
max_ending_here = max_so_far = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far if max_so_far > 0 else max(arr)
# Example usage
arr = [-2, -1, -3, -4, -1, -2, -1, -5, -4]
result = kadanes_algorithm_all_negative(arr)
print(f"Maximum subarray sum: {result}")
2. Finding the Subarray Indices
Sometimes, we not only want to know the maximum sum but also the start and end indices of the maximum subarray. Here’s how we can modify Kadane’s Algorithm to return this information:
def kadanes_algorithm_with_indices(arr):
max_ending_here = max_so_far = arr[0]
start = end = 0
temp_start = 0
for i, num in enumerate(arr[1:], 1):
if num > max_ending_here + num:
max_ending_here = num
temp_start = i
else:
max_ending_here += num
if max_ending_here > max_so_far:
max_so_far = max_ending_here
start = temp_start
end = i
return max_so_far, start, end
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result, start, end = kadanes_algorithm_with_indices(arr)
print(f"Maximum subarray sum: {result}")
print(f"Subarray indices: [{start}, {end}]")
3. Circular Maximum Subarray
A variation of the Maximum Subarray Problem is finding the maximum subarray sum in a circular array. This means the subarray can wrap around the end of the array. We can solve this problem using Kadane’s Algorithm twice:
def kadanes_circular(arr):
def kadane(arr):
max_ending_here = max_so_far = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far
max_linear = kadane(arr)
total_sum = sum(arr)
inverted_arr = [-num for num in arr]
max_circular = total_sum + kadane(inverted_arr)
return max(max_linear, max_circular) if max_linear > 0 else max_linear
# Example usage
arr = [1, -2, 3, -2]
result = kadanes_circular(arr)
print(f"Maximum circular subarray sum: {result}")
Applications of Kadane’s Algorithm
Kadane’s Algorithm and its variations have numerous practical applications across various domains. Let’s explore some of these applications:
1. Stock Market Analysis
In financial analysis, Kadane’s Algorithm can be used to find the best time to buy and sell stocks to maximize profit. By treating daily price changes as the input array, the algorithm can identify the most profitable period for holding a stock.
def max_profit(prices):
if not prices:
return 0
max_profit = 0
min_price = float('inf')
for price in prices:
min_price = min(min_price, price)
current_profit = price - min_price
max_profit = max(max_profit, current_profit)
return max_profit
# Example usage
stock_prices = [7, 1, 5, 3, 6, 4]
result = max_profit(stock_prices)
print(f"Maximum profit: {result}")
2. Image Processing
In image processing, a 2D version of Kadane’s Algorithm can be used to find the largest sum rectangular submatrix in a given matrix. This can be useful for identifying regions of interest in images or for optimizing certain image processing operations.
def max_sum_submatrix(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
max_sum = float('-inf')
for left in range(cols):
temp = [0] * rows
for right in range(left, cols):
for i in range(rows):
temp[i] += matrix[i][right]
current_sum = kadanes_algorithm(temp)
max_sum = max(max_sum, current_sum)
return max_sum
def kadanes_algorithm(arr):
max_ending_here = max_so_far = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far
# Example usage
matrix = [
[1, 2, -1, -4, -20],
[-8, -3, 4, 2, 1],
[3, 8, 10, 1, 3],
[-4, -1, 1, 7, -6]
]
result = max_sum_submatrix(matrix)
print(f"Maximum sum submatrix: {result}")
3. Bioinformatics
In bioinformatics, Kadane’s Algorithm can be applied to DNA sequence analysis. For instance, it can be used to find regions of high GC content in a DNA sequence, which are often associated with gene-rich areas.
def max_gc_content(dna_sequence):
gc_array = [1 if base in 'GC' else -1 for base in dna_sequence]
max_sum, start, end = kadanes_algorithm_with_indices(gc_array)
return max_sum, dna_sequence[start:end+1]
def kadanes_algorithm_with_indices(arr):
max_ending_here = max_so_far = arr[0]
start = end = 0
temp_start = 0
for i, num in enumerate(arr[1:], 1):
if num > max_ending_here + num:
max_ending_here = num
temp_start = i
else:
max_ending_here += num
if max_ending_here > max_so_far:
max_so_far = max_ending_here
start = temp_start
end = i
return max_so_far, start, end
# Example usage
dna_sequence = "ATGCATGCATGCGCGCGCGCATGCATGC"
gc_score, gc_rich_region = max_gc_content(dna_sequence)
print(f"Maximum GC content score: {gc_score}")
print(f"GC-rich region: {gc_rich_region}")
Common Pitfalls and How to Avoid Them
While Kadane’s Algorithm is relatively straightforward, there are some common pitfalls that programmers might encounter. Here are a few to watch out for:
1. Handling Empty Arrays
The basic implementation of Kadane’s Algorithm assumes that the input array is non-empty. To handle empty arrays, you should add a check at the beginning of your function:
def kadanes_algorithm_safe(arr):
if not arr:
return 0 # or raise an exception, depending on your requirements
max_ending_here = max_so_far = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far
2. Overflow in Languages with Fixed-Size Integers
In languages like C or Java where integers have a fixed size, you need to be careful about integer overflow. If you’re dealing with large numbers, consider using long integers or implementing a check for overflow:
public static int kadanesAlgorithmSafe(int[] arr) {
if (arr == null || arr.length == 0) {
throw new IllegalArgumentException("Array must not be empty");
}
long maxEndingHere = arr[0];
long maxSoFar = arr[0];
for (int i = 1; i < arr.length; i++) {
maxEndingHere = Math.max(arr[i], maxEndingHere + arr[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
if (maxSoFar > Integer.MAX_VALUE) {
return Integer.MAX_VALUE; // or handle overflow as needed
}
}
return (int) maxSoFar;
}
3. Mishandling All-Negative Arrays
As mentioned earlier, the basic Kadane’s Algorithm doesn’t handle all-negative arrays correctly. Make sure to use the modified version we discussed earlier if your input might contain all negative numbers.
Practice Problems
To really master Kadane’s Algorithm, it’s essential to practice solving various problems that can be tackled using this technique. Here are some problems you can try:
- Maximum Product Subarray: Similar to the Maximum Subarray Problem, but you need to find the contiguous subarray with the largest product.
- Flip Bits: Given a binary array, find the maximum number of zeroes that can be flipped to ones to create the longest contiguous sequence of ones.
- Maximum Sum Rectangle: Given a 2D matrix, find the submatrix with the largest sum.
- Maximum Circular Sum Subarray: Find the maximum sum subarray in a circular array.
- Largest Sum Contiguous Subarray with at least K numbers: Find the largest sum of a contiguous subarray that contains at least K elements.
These problems will help you apply Kadane’s Algorithm in different contexts and deepen your understanding of its principles.
Conclusion
Kadane’s Algorithm is a powerful technique for solving the Maximum Subarray Problem and its variations. Its elegance lies in its simplicity and efficiency, making it a favorite among competitive programmers and a common topic in technical interviews, especially at major tech companies.
By mastering Kadane’s Algorithm, you’ll not only be better prepared for coding interviews but also gain insights into dynamic programming and efficient algorithm design. The principles behind this algorithm can be applied to a wide range of problems beyond just finding maximum subarrays.
Remember, the key to truly understanding and internalizing Kadane’s Algorithm is practice. Try implementing it in different programming languages, solve the practice problems we’ve suggested, and look for opportunities to apply it in real-world scenarios. With time and practice, you’ll find that this algorithm becomes a valuable tool in your programming toolkit.
Happy coding, and may your subarrays always be maximum!