Junior Engineer Technical Interview Prep: Your Ultimate Guide to Success
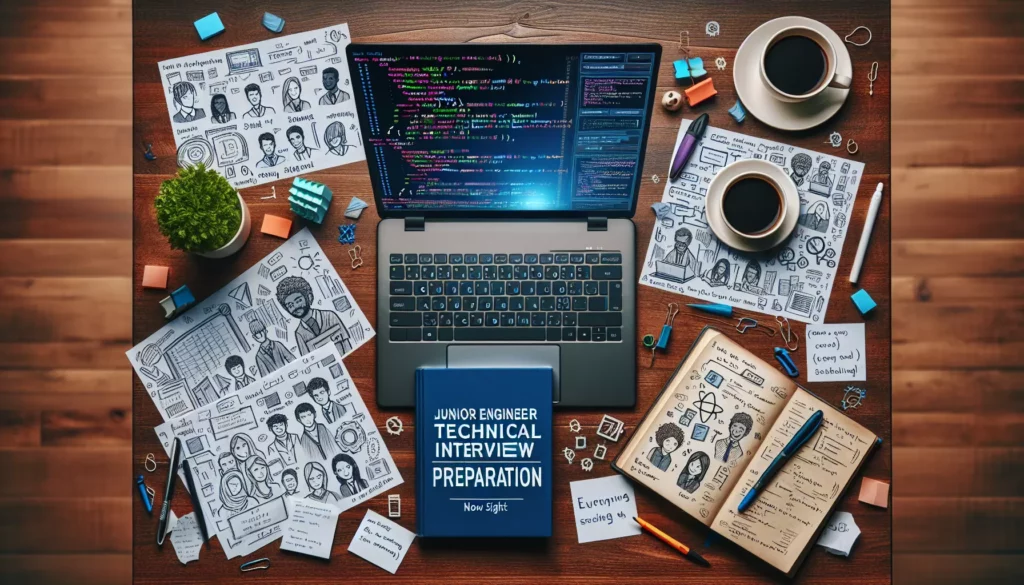
Are you a junior engineer gearing up for your first technical interview? Or perhaps you’re a recent graduate looking to break into the world of software development? Whatever your situation, preparing for a technical interview can be a daunting task. But fear not! This comprehensive guide will walk you through everything you need to know to ace your junior engineer technical interview.
Table of Contents
- Understanding the Technical Interview Process
- Core Concepts to Master
- Essential Data Structures
- Key Algorithms to Know
- Tackling Coding Challenges
- Basic System Design Concepts
- Soft Skills and Communication
- Tools and Resources for Preparation
- The Importance of Mock Interviews
- What to Expect on the Day of the Interview
- Post-Interview Follow-up
1. Understanding the Technical Interview Process
Before diving into the specifics of what to study, it’s crucial to understand the structure of a typical technical interview for junior engineers. While processes may vary between companies, most technical interviews follow a similar pattern:
- Phone Screening: An initial call to assess your background and interest in the position.
- Coding Challenge: Often a take-home assignment or a timed online coding test.
- Technical Phone Interview: A remote interview focusing on your problem-solving skills and basic coding ability.
- On-site Interview: A series of face-to-face interviews that may include whiteboarding sessions, pair programming, and discussions about your past projects.
As a junior engineer, the emphasis will likely be on your problem-solving skills, basic coding proficiency, and ability to learn quickly rather than deep expertise in specific technologies.
2. Core Concepts to Master
To prepare effectively, focus on mastering these fundamental concepts:
Object-Oriented Programming (OOP)
Understand the four pillars of OOP:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Be prepared to explain these concepts and provide simple examples of how you’ve used them in your projects.
Time and Space Complexity
Familiarize yourself with Big O notation and be able to analyze the time and space complexity of simple algorithms. This knowledge is crucial for optimizing your code and discussing trade-offs in your solutions.
Basic Design Patterns
While you’re not expected to be an expert, having a basic understanding of common design patterns can be beneficial. Focus on patterns like:
- Singleton
- Factory
- Observer
- Strategy
Version Control
Demonstrate proficiency with Git. Understand basic commands and concepts like branching, merging, and resolving conflicts.
3. Essential Data Structures
A solid grasp of data structures is crucial for solving coding problems efficiently. Focus on understanding these key data structures:
Arrays and Strings
Master operations like traversal, insertion, deletion, and searching. Understand the differences between static and dynamic arrays.
Linked Lists
Know how to implement singly and doubly linked lists. Be familiar with common operations and their time complexities.
Stacks and Queues
Understand the LIFO (Last In, First Out) nature of stacks and the FIFO (First In, First Out) nature of queues. Be able to implement them using arrays or linked lists.
Hash Tables
Grasp the concept of hashing and collision resolution. Understand when to use hash tables and their average time complexities for various operations.
Trees
Focus on binary trees and binary search trees. Understand tree traversal algorithms (in-order, pre-order, post-order) and basic operations like insertion and deletion.
Graphs
Know the basics of graph representation (adjacency list vs. adjacency matrix) and fundamental algorithms like breadth-first search (BFS) and depth-first search (DFS).
4. Key Algorithms to Know
While you’re not expected to memorize complex algorithms, understanding these fundamental algorithms will greatly boost your problem-solving capabilities:
Sorting Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
Understand the basic implementation and time complexity of each. Be prepared to implement at least one of these from scratch.
Searching Algorithms
- Linear Search
- Binary Search
Know how to implement binary search on sorted arrays and when to use it for optimization.
Graph Algorithms
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
Understand their applications and basic implementations.
Dynamic Programming
While not always expected for junior positions, having a basic understanding of dynamic programming concepts can be impressive. Start with simple problems like the Fibonacci sequence or the coin change problem.
5. Tackling Coding Challenges
Coding challenges are a crucial part of the technical interview process. Here’s how to approach them effectively:
Problem-Solving Approach
- Understand the problem: Read the question carefully and ask clarifying questions if needed.
- Plan your approach: Think about the algorithm and data structures you’ll use before starting to code.
- Write pseudocode: Outline your solution in plain English or pseudocode.
- Implement the solution: Write clean, readable code.
- Test your code: Consider edge cases and test your solution thoroughly.
- Optimize: If time allows, think about ways to improve the efficiency of your solution.
Common Types of Coding Challenges
- String manipulation
- Array operations
- Linked list operations
- Tree traversal and manipulation
- Basic graph problems
- Simple dynamic programming
Practice, Practice, Practice
Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Start with easy problems and gradually move to medium difficulty as you improve.
6. Basic System Design Concepts
While in-depth system design questions are typically reserved for more senior positions, having a basic understanding of system design principles can set you apart as a junior candidate:
Key Concepts to Understand
- Client-Server Architecture: Understand the basics of how web applications are structured.
- RESTful API Design: Know the principles of RESTful APIs and how to design simple endpoints.
- Databases: Understand the difference between relational and non-relational databases and when to use each.
- Caching: Grasp the basic concept of caching and its benefits in improving application performance.
- Load Balancing: Have a high-level understanding of what load balancers do and why they’re important for scalable systems.
Practice Explaining Simple Systems
Be prepared to explain the high-level architecture of systems you’re familiar with, such as a simple e-commerce website or a social media application. Focus on the main components and how they interact.
7. Soft Skills and Communication
Technical skills are crucial, but soft skills can often be the deciding factor in hiring decisions. Here’s what to focus on:
Clear Communication
Practice explaining your thought process clearly. When solving problems, talk through your approach out loud. This helps interviewers understand your reasoning and problem-solving skills.
Teamwork and Collaboration
Be prepared to discuss experiences where you’ve worked in a team. Highlight your ability to collaborate, share ideas, and receive feedback constructively.
Enthusiasm and Willingness to Learn
Show genuine interest in the role and the company. Demonstrate your eagerness to learn new technologies and tackle challenges.
Handling Uncertainty
It’s okay not to know everything. If you’re unsure about something, be honest and explain how you would go about finding the answer or solving the problem.
8. Tools and Resources for Preparation
Leverage these tools and resources to enhance your preparation:
Online Platforms
- LeetCode: Offers a wide range of coding problems with solutions and discussions.
- HackerRank: Provides coding challenges and has a specific interview preparation kit.
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “The Algorithm Design Manual” by Steven S. Skiena
- “Clean Code” by Robert C. Martin
Online Courses
- Coursera’s “Data Structures and Algorithms” specialization
- edX’s “Introduction to Computer Science” courses
- Udacity’s “Data Structures and Algorithms in Python” course
IDEs and Code Editors
Familiarize yourself with popular IDEs and code editors like Visual Studio Code, PyCharm, or Eclipse. Being comfortable with your coding environment can help reduce stress during the interview.
9. The Importance of Mock Interviews
Mock interviews are one of the most effective ways to prepare for the real thing. Here’s how to make the most of them:
Find a Practice Partner
Team up with a friend, classmate, or mentor who can play the role of the interviewer. If possible, find someone with experience in technical interviews.
Simulate Real Interview Conditions
Conduct mock interviews in a quiet space, use a whiteboard or shared document for coding, and set a timer to mimic the time constraints of a real interview.
Practice Different Types of Questions
Cover a range of question types, including coding challenges, system design discussions, and behavioral questions.
Seek Feedback
After each mock interview, ask for honest feedback on your performance. Focus on areas like problem-solving approach, code quality, communication skills, and overall impression.
Online Mock Interview Platforms
Consider using platforms like Pramp or InterviewBit, which connect you with other candidates for peer-to-peer mock interviews.
10. What to Expect on the Day of the Interview
Being prepared for the logistics and format of the interview day can help reduce anxiety and improve your performance:
For On-site Interviews
- Arrive early to allow time for security check-in and to compose yourself.
- Bring multiple copies of your resume, a notebook, and a pen.
- Dress appropriately – usually business casual is suitable for tech companies.
- Be prepared for multiple rounds of interviews, potentially with different team members.
For Remote Interviews
- Test your internet connection, camera, and microphone in advance.
- Choose a quiet, well-lit space for the interview.
- Have a backup plan (like a phone number) in case of technical issues.
- Keep a glass of water nearby.
Common Interview Formats
- Whiteboarding: You may be asked to solve problems on a whiteboard or shared online document.
- Pair Programming: Some companies use pair programming exercises to assess your coding and collaboration skills.
- Take-home Projects: You might be given a small project to complete within a specified timeframe.
Be Ready for Various Question Types
- Technical questions about your preferred programming languages and technologies
- Coding challenges
- Behavioral questions about your past experiences and how you handle various situations
- Questions about your resume and past projects
11. Post-Interview Follow-up
The interview process doesn’t end when you walk out of the room or log off the video call. Proper follow-up can leave a lasting positive impression:
Send a Thank You Email
Within 24 hours of your interview, send a brief thank you email to your interviewer or the HR contact. Express your appreciation for their time and reiterate your interest in the position.
Reflect on Your Performance
Take some time to think about what went well and what you could improve. This reflection will be valuable for future interviews, regardless of the outcome.
Follow Up on Next Steps
If you haven’t been given a timeline for the next steps, it’s appropriate to ask about the expected timeframe for a decision.
Be Patient
The hiring process can take time, especially for larger companies. While it’s okay to follow up if you haven’t heard back within the stated timeframe, avoid excessive follow-ups.
Learn from the Experience
Whether you get the job or not, each interview is a learning opportunity. If you don’t get an offer, don’t be afraid to ask for feedback on how you can improve for future interviews.
Conclusion
Preparing for a junior engineer technical interview may seem overwhelming, but with the right approach and consistent practice, you can significantly improve your chances of success. Remember, the goal is not just to memorize information but to develop problem-solving skills and the ability to apply your knowledge in various scenarios.
Focus on building a strong foundation in core computer science concepts, data structures, and algorithms. Practice coding regularly and work on explaining your thought process clearly. Don’t forget the importance of soft skills and the ability to work well in a team.
Leverage the wealth of resources available, from online platforms like AlgoCademy to books and mock interview practice. Each interview, whether successful or not, is an opportunity to learn and improve.
Most importantly, stay confident in your abilities and show enthusiasm for the role and the company. Your passion for technology and willingness to learn can often be just as important as your current skill set.
Good luck with your preparation, and remember – with dedication and the right mindset, you’re well on your way to landing your dream job as a junior engineer!