Jump Game: Mastering Dynamic Programming for Coding Interviews
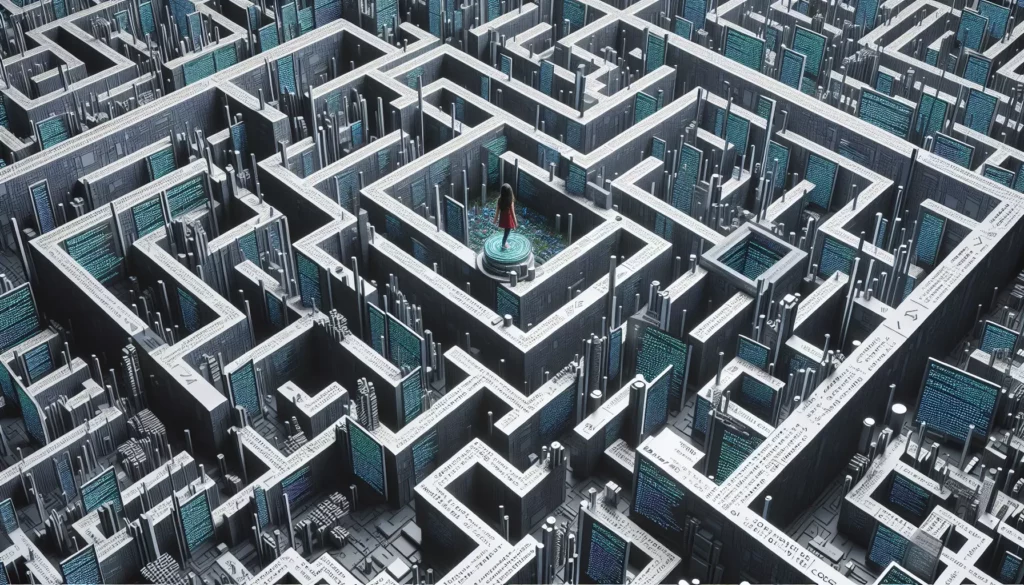
Welcome to AlgoCademy’s deep dive into the Jump Game problem! This classic algorithmic challenge is a favorite among tech interviewers, especially at FAANG companies. It’s an excellent example of how dynamic programming can be applied to solve seemingly complex problems efficiently. In this comprehensive guide, we’ll break down the problem, explore multiple solutions, and provide you with the tools to ace this question in your next coding interview.
Understanding the Jump Game Problem
Before we jump into solutions (pun intended), let’s clearly define the problem:
Given an array of non-negative integers
nums
, you are initially positioned at the first index of the array. Each element in the array represents your maximum jump length at that position. Your goal is to determine if you can reach the last index.
For example:
Input: nums = [2,3,1,1,4]
Output: true
Explanation: Jump 1 step from index 0 to 1, then 3 steps to the last index.
Input: nums = [3,2,1,0,4]
Output: false
Explanation: You will always arrive at index 3 no matter what. Its maximum jump length is 0, which makes it impossible to reach the last index.
This problem tests your ability to think dynamically and optimize your solution. It’s a perfect example of how a greedy approach can sometimes outperform a more intuitive dynamic programming solution.
Approach 1: Brute Force (Recursive)
Let’s start with the most straightforward approach – a recursive solution that explores all possible jumps.
class Solution {
public:
bool canJump(vector<int>& nums) {
return canJumpFromPosition(0, nums);
}
private:
bool canJumpFromPosition(int position, vector<int>& nums) {
if (position == nums.size() - 1) {
return true;
}
int furthestJump = min(position + nums[position], (int)nums.size() - 1);
for (int nextPosition = position + 1; nextPosition <= furthestJump; nextPosition++) {
if (canJumpFromPosition(nextPosition, nums)) {
return true;
}
}
return false;
}
};
This solution works by recursively trying all possible jumps from the current position. While it correctly solves the problem, it has a time complexity of O(2^n) in the worst case, making it impractical for large inputs.
Approach 2: Dynamic Programming (Top-down)
We can significantly improve our brute force approach by introducing memoization:
class Solution {
public:
bool canJump(vector<int>& nums) {
vector<int> memo(nums.size(), -1);
return canJumpFromPosition(0, nums, memo);
}
private:
bool canJumpFromPosition(int position, vector<int>& nums, vector<int>& memo) {
if (memo[position] != -1) {
return memo[position] == 1;
}
if (position == nums.size() - 1) {
memo[position] = 1;
return true;
}
int furthestJump = min(position + nums[position], (int)nums.size() - 1);
for (int nextPosition = position + 1; nextPosition <= furthestJump; nextPosition++) {
if (canJumpFromPosition(nextPosition, nums, memo)) {
memo[position] = 1;
return true;
}
}
memo[position] = 0;
return false;
}
};
This top-down dynamic programming approach uses memoization to avoid redundant calculations. It reduces the time complexity to O(n^2) in the worst case, which is a significant improvement over the brute force method.
Approach 3: Dynamic Programming (Bottom-up)
We can further optimize our solution by using a bottom-up dynamic programming approach:
class Solution {
public:
bool canJump(vector<int>& nums) {
int n = nums.size();
vector<bool> dp(n, false);
dp[n-1] = true;
for (int i = n - 2; i >= 0; i--) {
for (int j = 0; j <= nums[i] && i + j < n; j++) {
if (dp[i + j]) {
dp[i] = true;
break;
}
}
}
return dp[0];
}
};
This bottom-up approach builds the solution iteratively, starting from the last index. It has a time complexity of O(n^2) in the worst case, but it’s generally faster than the top-down approach due to less function call overhead.
Approach 4: Greedy
The most efficient solution to this problem is actually a greedy approach:
class Solution {
public:
bool canJump(vector<int>& nums) {
int lastPos = nums.size() - 1;
for (int i = nums.size() - 1; i >= 0; i--) {
if (i + nums[i] >= lastPos) {
lastPos = i;
}
}
return lastPos == 0;
}
};
This greedy approach works backwards from the last index, keeping track of the leftmost position from which we can reach the last index. If we can reach the start (index 0), then we can jump to the end. This solution has a time complexity of O(n) and space complexity of O(1), making it the most efficient approach.
Understanding the Greedy Approach
The greedy approach might not be immediately intuitive, so let’s break it down:
- We start from the last index, which is always reachable from itself.
- We then move backwards, for each position checking if we can reach the last known reachable position (initially the last index).
- If we can reach the last known reachable position from our current position, we update the last known reachable position to our current position.
- We continue this process until we reach the start of the array.
- If the last known reachable position ends up being the start of the array (index 0), we know we can reach the end from the start.
This approach works because we’re essentially building a chain of reachable positions from the end to the start. If this chain reaches the start, we know we can make it to the end.
Time and Space Complexity Analysis
Let’s compare the time and space complexities of our different approaches:
Approach | Time Complexity | Space Complexity |
---|---|---|
Brute Force | O(2^n) | O(n) |
DP (Top-down) | O(n^2) | O(n) |
DP (Bottom-up) | O(n^2) | O(n) |
Greedy | O(n) | O(1) |
As we can see, the greedy approach is significantly more efficient in both time and space complexity. This is why it’s often the preferred solution in coding interviews.
Common Pitfalls and Edge Cases
When tackling the Jump Game problem, be aware of these common pitfalls and edge cases:
- Single Element Array: Don’t forget to handle the case where the array has only one element. This is always true as you’re already at the last index.
- Zero Jump: Be careful with zeros in the array. A zero means you can’t jump from that position, which might trap you.
- Overflowing the Array: Ensure your jumps don’t go beyond the array bounds.
- Maximum Jumps: Remember that each number represents the maximum jump from that position, not the exact jump you must make.
Related Problems and Variations
Once you’ve mastered the Jump Game, you might want to try these related problems:
- Jump Game II: Instead of determining if you can reach the last index, find the minimum number of jumps to reach the last index.
- Jump Game III: You can move forward or backward by the value at the current index. Determine if you can reach an index with value 0.
- Jump Game IV: You can jump to i+1, i-1, or any index with the same value as the current index. Find the minimum number of steps to reach the last index.
- Jump Game V: You can jump up to a certain distance, but only to lower heights. Count the maximum number of indices you can visit.
These variations test similar concepts but add additional constraints or objectives, helping you further develop your problem-solving skills.
Interview Tips for the Jump Game
When faced with the Jump Game in an interview, keep these tips in mind:
- Start Simple: Begin with the brute force approach to show you understand the problem.
- Optimize Step-by-Step: Move from brute force to dynamic programming, explaining your thought process.
- Consider Greedy: If you see a pattern, propose the greedy approach and explain why it works.
- Analyze Complexity: Always discuss the time and space complexity of your solutions.
- Test Your Code: Use example inputs and edge cases to verify your solution.
- Communicate: Explain your thinking throughout the process. Interviewers value clear communication as much as correct solutions.
Conclusion
The Jump Game is a fantastic problem that showcases the power of dynamic programming and greedy algorithms. By understanding this problem deeply, you’re not just preparing for a potential interview question – you’re developing crucial problem-solving skills that will serve you well throughout your programming career.
Remember, the key to mastering algorithmic problems like the Jump Game is practice. Try implementing each approach we’ve discussed, analyze their performance, and understand why the greedy solution works so well for this particular problem. As you encounter variations and related problems, you’ll find that the insights you’ve gained here will help you tackle a wide range of challenges.
Keep jumping, keep coding, and keep pushing your skills to new heights. With persistence and practice, you’ll be well-prepared to ace your coding interviews and take on complex programming challenges in your future career. Happy coding!