Java vs JavaScript: Understanding the Key Differences Between These Popular Programming Languages
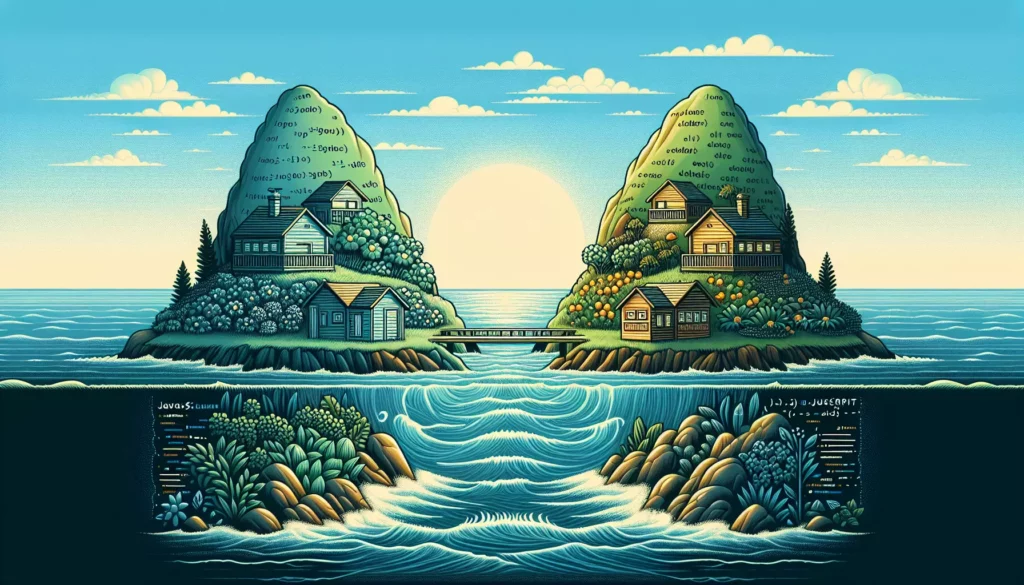
In the world of programming, Java and JavaScript are two of the most widely used languages. Despite their similar names, these languages have distinct characteristics, purposes, and applications. This comprehensive guide will explore the key differences between Java and JavaScript, helping you understand when and why to use each language in your development projects.
Table of Contents
- Introduction to Java and JavaScript
- Language Type and Execution
- Syntax and Structure
- Object-Oriented Programming Approach
- Type System
- Memory Management
- Platform Compatibility
- Common Use Cases
- Performance Considerations
- Learning Curve and Development Speed
- Ecosystem and Libraries
- Career Opportunities
- Conclusion
1. Introduction to Java and JavaScript
Before diving into the differences, let’s briefly introduce both languages:
Java
Java is a general-purpose, class-based, object-oriented programming language designed to be platform-independent. It was created by James Gosling at Sun Microsystems (now owned by Oracle Corporation) and released in 1995. Java follows the principle of “Write Once, Run Anywhere” (WORA), which means that compiled Java code can run on all platforms that support Java without the need for recompilation.
JavaScript
JavaScript, often abbreviated as JS, is a high-level, interpreted programming language that conforms to the ECMAScript specification. It was created by Brendan Eich at Netscape Communications and first appeared in 1995. Initially designed as a scripting language for web browsers, JavaScript has evolved into a versatile language used for both client-side and server-side development.
2. Language Type and Execution
Java
Java is a compiled language, which means that the source code is first compiled into bytecode. This bytecode is then executed by the Java Virtual Machine (JVM). The compilation process involves several steps:
- Writing the source code (.java files)
- Compiling the source code into bytecode (.class files) using the Java compiler (javac)
- Running the bytecode on the JVM
This process allows Java to achieve its “Write Once, Run Anywhere” capability, as the bytecode can be executed on any platform with a compatible JVM.
JavaScript
JavaScript, on the other hand, is an interpreted language. This means that the code is executed directly, line by line, by an interpreter. In web browsers, this interpreter is part of the JavaScript engine (e.g., V8 in Chrome, SpiderMonkey in Firefox). The execution process for JavaScript typically involves:
- Writing the source code (.js files)
- Loading the script in a web browser or Node.js environment
- The JavaScript engine interpreting and executing the code on the fly
This interpreted nature allows for more flexibility and faster development cycles, as changes can be made and immediately tested without a separate compilation step.
3. Syntax and Structure
While Java and JavaScript share some syntactical similarities due to their common C-style syntax heritage, they have distinct differences in their structure and coding conventions.
Java
Java has a more rigid structure and verbose syntax. Here are some key characteristics:
- Statically typed: Variables must be declared with their type before use.
- Class-based: All code must be written within classes.
- Strict syntax: Semicolons are required to end statements, and curly braces are used to define blocks of code.
- Strong typing: Implicit type conversion is limited, and explicit casting is often required.
Here’s a simple example of a Java class:
public class HelloWorld {
public static void main(String[] args) {
String message = "Hello, World!";
System.out.println(message);
}
}
JavaScript
JavaScript has a more flexible and concise syntax. Key features include:
- Dynamically typed: Variables can hold values of any type without explicit declaration.
- Prototype-based: Objects can be created and modified on the fly without strict class definitions.
- More lenient syntax: Semicolons are optional in many cases, and there’s more flexibility in code structure.
- Weak typing: Implicit type conversion is common, and variables can change types during runtime.
Here’s an equivalent example in JavaScript:
let message = "Hello, World!";
console.log(message);
4. Object-Oriented Programming Approach
Both Java and JavaScript support object-oriented programming (OOP), but they implement it in different ways.
Java
Java is a class-based OOP language, which means:
- Classes are the fundamental units of code organization.
- Objects are instances of classes.
- Inheritance is achieved through class extension.
- It supports encapsulation, inheritance, and polymorphism as core OOP concepts.
- Interfaces and abstract classes are used for defining contracts and achieving abstraction.
Example of a simple class hierarchy in Java:
public abstract class Animal {
protected String name;
public Animal(String name) {
this.name = name;
}
public abstract void makeSound();
}
public class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void makeSound() {
System.out.println(name + " says: Woof!");
}
}
JavaScript
JavaScript uses prototype-based OOP, which means:
- Objects can be created without defining a class.
- Prototypes are used for inheritance and sharing properties/methods.
- It supports dynamic addition or modification of properties and methods at runtime.
- Classes were introduced in ES6 as syntactic sugar over prototype-based OOP.
Example of object creation and prototype-based inheritance in JavaScript:
// Using ES6 class syntax
class Animal {
constructor(name) {
this.name = name;
}
makeSound() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
makeSound() {
console.log(`${this.name} says: Woof!`);
}
}
// Using prototype-based approach
function Animal(name) {
this.name = name;
}
Animal.prototype.makeSound = function() {
console.log(this.name + " makes a sound.");
};
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.makeSound = function() {
console.log(this.name + " says: Woof!");
};
5. Type System
The type system is one of the most significant differences between Java and JavaScript, affecting how variables are declared and used throughout the code.
Java
Java uses a static type system, which means:
- Variables must be declared with their type before use.
- Type checking is done at compile-time, catching many errors before the program runs.
- Explicit type casting is required when converting between types.
- Generics are supported for creating reusable, type-safe code.
Example of Java’s type system:
int number = 5;
String text = "Hello";
double decimal = 3.14;
// Type casting
int intValue = (int) decimal;
// Generics
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
JavaScript
JavaScript uses a dynamic type system, which means:
- Variables can hold values of any type without explicit declaration.
- Type checking is done at runtime.
- Variables can change types during program execution.
- Implicit type conversion (coercion) is common.
Example of JavaScript’s type system:
let number = 5;
let text = "Hello";
let decimal = 3.14;
// Dynamic typing
number = "Now I'm a string";
// Implicit type conversion
let result = 5 + "5"; // result is "55" (string)
let boolResult = 5 > "3"; // true (string "3" is converted to number)
6. Memory Management
Memory management is another area where Java and JavaScript differ significantly, affecting how developers handle resource allocation and deallocation.
Java
Java uses automatic memory management through garbage collection:
- Objects are allocated memory from the heap.
- The Java Virtual Machine (JVM) includes a garbage collector that automatically frees memory occupied by objects that are no longer in use.
- Developers don’t need to explicitly deallocate memory, reducing the risk of memory leaks.
- The JVM provides various garbage collection algorithms and tuning options for optimizing memory management.
Example of object creation in Java (memory is managed automatically):
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
}
// Creating objects
Person person1 = new Person("Alice");
Person person2 = new Person("Bob");
// When these objects are no longer referenced, the garbage collector will free the memory
JavaScript
JavaScript also uses automatic memory management, but with some differences:
- Memory is allocated when objects are created and freed automatically when they are no longer in use.
- The JavaScript engine (e.g., V8) includes a garbage collector that identifies and removes objects that are no longer reachable.
- Reference counting and mark-and-sweep algorithms are commonly used for garbage collection in JavaScript engines.
- Developers need to be aware of potential memory leaks, especially when dealing with closures and event listeners.
Example of object creation in JavaScript:
function Person(name) {
this.name = name;
}
// Creating objects
let person1 = new Person("Alice");
let person2 = new Person("Bob");
// When these variables go out of scope or are reassigned, the objects become eligible for garbage collection
7. Platform Compatibility
Java and JavaScript have different approaches to platform compatibility, which affects where and how they can be run.
Java
Java’s platform compatibility is based on its “Write Once, Run Anywhere” (WORA) principle:
- Java code is compiled into bytecode, which can run on any platform with a Java Virtual Machine (JVM).
- The JVM acts as an abstraction layer between the Java program and the underlying operating system.
- Java is commonly used for desktop applications, Android app development, server-side applications, and enterprise systems.
- Different editions of Java (e.g., Java SE, Java EE, Java ME) cater to various platforms and use cases.
JavaScript
JavaScript’s platform compatibility is primarily web-focused but has expanded to other environments:
- Originally designed for web browsers, JavaScript runs on all modern web browsers without additional plugins.
- Node.js allows JavaScript to run on servers and as a general-purpose programming language.
- JavaScript can be used for cross-platform mobile app development using frameworks like React Native or Ionic.
- Desktop applications can be built using JavaScript with frameworks like Electron.
8. Common Use Cases
Java and JavaScript have distinct strengths that make them suitable for different types of projects and applications.
Java
Common use cases for Java include:
- Enterprise-level applications
- Android mobile app development
- Large-scale distributed systems
- Scientific and financial applications requiring high precision
- Server-side web applications (using frameworks like Spring)
- Big data processing (with platforms like Hadoop)
- Embedded systems and IoT devices
JavaScript
Common use cases for JavaScript include:
- Front-end web development
- Single-page applications (SPAs)
- Server-side development with Node.js
- Full-stack web development (e.g., MEAN or MERN stack)
- Cross-platform mobile app development
- Desktop application development (using Electron)
- Browser extensions and add-ons
- Game development (using libraries like Phaser or Three.js)
9. Performance Considerations
Performance characteristics of Java and JavaScript can vary depending on the specific use case and implementation.
Java
Java’s performance characteristics include:
- Generally faster execution speed due to compilation to bytecode and Just-In-Time (JIT) compilation
- More predictable performance, especially for long-running applications
- Better suited for CPU-intensive tasks and multi-threaded applications
- Requires more memory due to the JVM overhead
- Longer startup time compared to interpreted languages
JavaScript
JavaScript’s performance characteristics include:
- Faster startup time due to its interpreted nature
- Modern JavaScript engines use Just-In-Time (JIT) compilation for improved performance
- Well-suited for I/O-bound tasks, especially in server-side environments like Node.js
- Performance can vary across different browsers and JavaScript engines
- May struggle with CPU-intensive tasks compared to compiled languages
10. Learning Curve and Development Speed
The learning curve and development speed for Java and JavaScript can differ significantly, affecting how quickly developers can become productive in each language.
Java
Learning and development considerations for Java:
- Steeper learning curve due to its more complex syntax and concepts
- Requires understanding of object-oriented programming principles
- More verbose code, which can lead to slower initial development
- Strong typing and compile-time checks can catch errors early in the development process
- Extensive standard library and well-established design patterns
- Integrated Development Environments (IDEs) like IntelliJ IDEA and Eclipse provide powerful tools for Java development
JavaScript
Learning and development considerations for JavaScript:
- Generally considered easier to learn, especially for beginners
- More flexible syntax allows for quicker prototyping and experimentation
- Dynamic typing can lead to faster initial development but may introduce runtime errors
- Asynchronous programming concepts (e.g., callbacks, promises, async/await) can be challenging for newcomers
- Large ecosystem of libraries and frameworks that can accelerate development
- Browser developer tools provide built-in debugging capabilities
11. Ecosystem and Libraries
Both Java and JavaScript have rich ecosystems with a wide variety of libraries, frameworks, and tools to support development.
Java
Java’s ecosystem includes:
- A vast standard library (Java Class Library) covering a wide range of functionalities
- Popular frameworks for web development (e.g., Spring, JavaServer Faces)
- Build tools like Maven and Gradle
- Testing frameworks such as JUnit and TestNG
- ORM (Object-Relational Mapping) tools like Hibernate
- Big data processing frameworks (e.g., Apache Hadoop, Apache Spark)
- Android SDK for mobile app development
JavaScript
JavaScript’s ecosystem includes:
- npm (Node Package Manager) for managing dependencies
- Front-end frameworks and libraries (e.g., React, Angular, Vue.js)
- Back-end frameworks for Node.js (e.g., Express.js, Koa, Nest.js)
- Testing frameworks like Jest and Mocha
- Build tools and module bundlers (e.g., Webpack, Rollup)
- Task runners like Gulp and Grunt
- Static type checkers (e.g., TypeScript, Flow)
12. Career Opportunities
Both Java and JavaScript offer numerous career opportunities in the software development industry.
Java
Career opportunities for Java developers include:
- Enterprise software developer
- Android app developer
- Back-end web developer
- Big data engineer
- DevOps engineer
- Systems architect
- Embedded systems developer
JavaScript
Career opportunities for JavaScript developers include:
- Front-end web developer
- Full-stack developer
- Node.js developer
- Mobile app developer (using frameworks like React Native)
- UI/UX developer
- JavaScript framework specialist (e.g., React, Angular, Vue.js)
- DevOps engineer (using JavaScript for automation and scripting)
13. Conclusion
Java and JavaScript are both powerful and widely-used programming languages, each with its own strengths and ideal use cases. Java excels in building large-scale, enterprise-level applications, Android development, and scenarios requiring strong typing and high performance. JavaScript, on the other hand, dominates web development, offers flexibility for full-stack development, and enables rapid prototyping and cross-platform applications.
When choosing between Java and JavaScript, consider factors such as:
- The specific requirements of your project
- The target platform or environment
- Your team’s expertise and preferences
- Performance and scalability needs
- Development speed and maintainability
- Available resources and ecosystem support
In many cases, modern development projects may utilize both languages, leveraging Java’s robustness for backend services and JavaScript’s flexibility for frontend interfaces. Understanding the key differences between these languages will help you make informed decisions and choose the right tool for the job in your software development journey.
Remember, the best language to learn or use often depends on your goals, the project requirements, and the ecosystem you’re working in. Both Java and JavaScript continue to evolve and maintain their relevance in the ever-changing landscape of software development.