Java vs. C++: Which Language Should You Learn for Your Career?
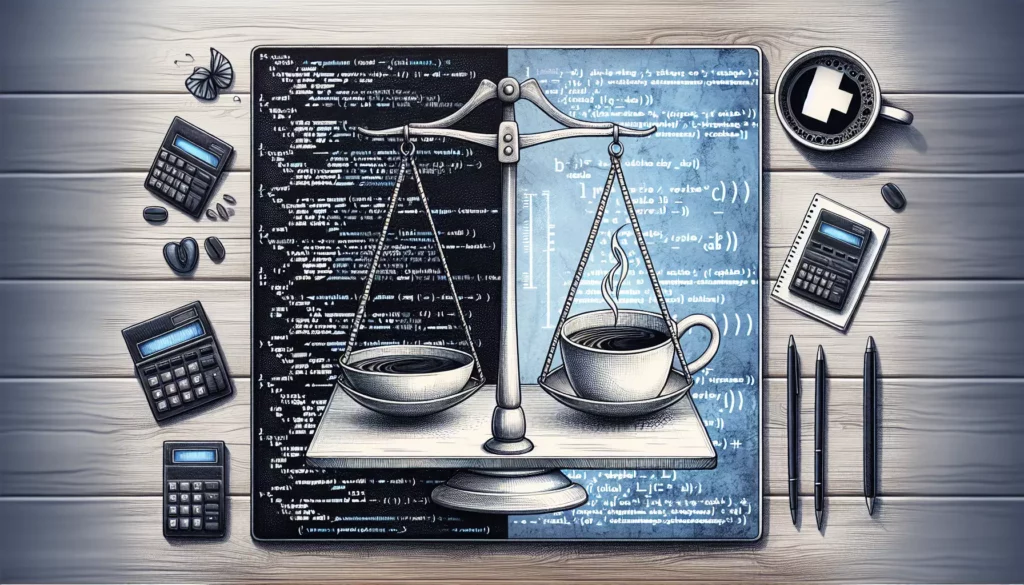
In the ever-evolving world of programming, two giants have consistently stood the test of time: Java and C++. Both languages have played crucial roles in shaping the software development landscape, and continue to be in high demand across various industries. As aspiring programmers or those looking to advance their careers, you might find yourself at a crossroads, wondering which language to invest your time and energy in. In this comprehensive guide, we’ll dive deep into the Java vs. C++ debate, exploring their strengths, weaknesses, and career prospects to help you make an informed decision.
Understanding Java and C++: A Brief Overview
Java: Write Once, Run Anywhere
Java, developed by Sun Microsystems (now owned by Oracle) in 1995, is an object-oriented, class-based programming language designed with the principle of “Write Once, Run Anywhere” (WORA). This means that Java code can run on any platform that supports the Java Virtual Machine (JVM) without needing to be recompiled.
Key features of Java include:
- Platform independence
- Strong memory management with automatic garbage collection
- Extensive standard library
- Strong focus on security
- Multi-threading capabilities
C++: Power and Performance
C++, an extension of the C programming language, was developed by Bjarne Stroustrup in 1979. It is known for its performance, efficiency, and close-to-hardware programming capabilities. C++ supports both procedural and object-oriented programming paradigms.
Key features of C++ include:
- High performance and efficiency
- Low-level memory manipulation
- Extensive control over system resources
- Support for both high-level and low-level programming
- Rich set of libraries and frameworks
Comparing Java and C++: Key Differences
1. Memory Management
Java: Java uses automatic memory management through its garbage collector. This means that developers don’t need to explicitly allocate or deallocate memory, reducing the chances of memory leaks and making development easier, especially for beginners.
C++: C++ gives programmers direct control over memory management. While this allows for more efficient memory usage, it also requires developers to manually allocate and deallocate memory, which can lead to issues like memory leaks if not handled properly.
2. Platform Independence
Java: Java’s “Write Once, Run Anywhere” philosophy means that Java bytecode can run on any platform with a Java Virtual Machine (JVM) installed. This makes Java highly portable across different operating systems and devices.
C++: C++ code needs to be compiled for specific platforms. While it’s possible to write portable C++ code, it often requires additional effort and consideration of platform-specific details.
3. Performance
Java: Java’s performance has improved significantly over the years, but it generally has more overhead due to the JVM and garbage collection. However, for most applications, this difference is negligible.
C++: C++ is known for its high performance and efficiency. It allows for low-level optimizations and direct hardware access, making it suitable for system-level programming and performance-critical applications.
4. Syntax and Complexity
Java: Java has a simpler syntax compared to C++ and eliminates certain complex features like multiple inheritance (of classes) and operator overloading. This can make Java easier to learn and read, especially for beginners.
C++: C++ offers more flexibility and control, but with that comes increased complexity. Features like multiple inheritance, operator overloading, and pointer arithmetic can be powerful but also more challenging to master.
5. Standard Library
Java: Java comes with a comprehensive standard library that provides a wide range of utilities, from data structures to networking and GUI development. This rich ecosystem can significantly speed up development time.
C++: While C++ also has a standard library, it’s generally considered less extensive than Java’s. However, C++ has a vast array of third-party libraries and frameworks available.
Career Prospects: Java vs. C++
Job Market Demand
Both Java and C++ continue to be in high demand in the job market. However, the types of roles and industries can differ:
Java Job Opportunities:
- Web Development (especially backend)
- Android App Development
- Enterprise Software Development
- Big Data and Analytics
- Cloud Computing
- Financial Services
C++ Job Opportunities:
- Game Development
- Systems Programming
- Embedded Systems
- High-Performance Computing
- Graphics and Simulation
- Financial Trading Systems
Salary Prospects
Salaries for Java and C++ developers can vary based on factors like experience, location, and specific industry. However, both languages generally offer competitive salaries:
- According to Glassdoor, the average base salary for a Java Developer in the United States is around $79,000 per year.
- For C++ Developers, Glassdoor reports an average base salary of about $95,000 per year in the United States.
It’s important to note that these figures can vary significantly based on factors like location, experience, and specific job roles. Senior developers and those with specialized skills in either language can command much higher salaries.
Industry Trends
Java Trends:
- Continued dominance in enterprise software development
- Growing use in cloud-native applications and microservices architectures
- Increasing adoption in big data technologies (e.g., Hadoop, Spark)
- Ongoing popularity in Android app development
C++ Trends:
- Resurgence in systems programming with the rise of IoT and edge computing
- Continued importance in game development and graphics programming
- Growing use in machine learning and AI, especially for performance-critical components
- Ongoing evolution of the language with modern features in recent standards (C++11, C++14, C++17, C++20)
Making Your Decision: Factors to Consider
When deciding between Java and C++, consider the following factors:
1. Your Career Goals
If you’re interested in web development, enterprise software, or Android app development, Java might be the better choice. If you’re passionate about game development, systems programming, or working close to the hardware, C++ could be more suitable.
2. Learning Curve
Java is generally considered easier to learn, especially for beginners. Its simpler syntax and automatic memory management can help you focus on core programming concepts. C++, while more complex, offers a deeper understanding of how computers work and can be an excellent foundation for learning other languages.
3. Project Requirements
Consider the types of projects you want to work on. Java’s “Write Once, Run Anywhere” philosophy makes it ideal for cross-platform development. C++’s performance advantages make it suitable for resource-intensive applications.
4. Industry Demand
Research the job market in your area or the industry you want to work in. Some sectors may have a higher demand for Java developers, while others might prefer C++ expertise.
5. Personal Interest
Your personal interest and enjoyment in working with a language can significantly impact your learning and career progression. Try out both languages and see which one you find more engaging and enjoyable to work with.
Learning Strategies: Mastering Java or C++
Regardless of which language you choose, here are some strategies to help you master it:
1. Start with the Basics
Begin by understanding the fundamental concepts of programming and the specific syntax of your chosen language. Online resources like AlgoCademy can provide structured learning paths for both Java and C++.
2. Practice Regularly
Consistent practice is key to mastering any programming language. Set aside time each day to write code, even if it’s just for a small project or coding challenge.
3. Work on Projects
Apply your knowledge to real-world projects. This could be personal projects, open-source contributions, or internships. Practical experience is invaluable in solidifying your skills.
4. Learn Data Structures and Algorithms
A strong foundation in data structures and algorithms is crucial for both Java and C++ development. Platforms like AlgoCademy offer specialized courses and practice problems in this area.
5. Join Communities
Engage with the developer community for your chosen language. Join forums, attend meetups, and participate in online discussions. This can provide valuable insights, learning resources, and networking opportunities.
6. Stay Updated
Both Java and C++ continue to evolve. Stay updated with the latest language features, best practices, and industry trends.
Code Examples: Java vs. C++
To give you a taste of both languages, let’s look at a simple example implemented in both Java and C++. We’ll create a basic class representing a Person with a name and age, and a method to print their details.
Java Example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void printDetails() {
System.out.println("Name: " + name + ", Age: " + age);
}
public static void main(String[] args) {
Person person = new Person("Alice", 30);
person.printDetails();
}
}
C++ Example:
#include <iostream>
#include <string>
class Person {
private:
std::string name;
int age;
public:
Person(std::string name, int age) : name(name), age(age) {}
void printDetails() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
int main() {
Person person("Alice", 30);
person.printDetails();
return 0;
}
As you can see, while the overall structure is similar, there are differences in syntax and how certain features are implemented. Java uses the System.out.println()
for output, while C++ uses std::cout
. C++ also requires explicit inclusion of the <iostream>
and <string>
libraries.
Conclusion: The Best Choice for Your Career
In the Java vs. C++ debate, there’s no one-size-fits-all answer. Both languages have their strengths and are valuable in different contexts. Your choice should depend on your career goals, interests, and the type of projects you want to work on.
Java might be the better choice if you’re interested in:
- Web and enterprise application development
- Android app development
- Big data and cloud computing
- A gentler learning curve, especially for beginners
C++ could be more suitable if you’re passionate about:
- Game development and graphics programming
- Systems programming and embedded systems
- High-performance computing and applications
- Gaining a deeper understanding of computer systems
Remember, many successful developers are proficient in both Java and C++, along with other languages. The skills you learn in one language often transfer to others, and being versatile can open up more career opportunities.
Whichever language you choose, the key to success is dedication, consistent practice, and a passion for problem-solving. Platforms like AlgoCademy can provide you with the resources, structured learning paths, and practice problems you need to master either Java or C++, preparing you for a successful career in software development.
Start your journey today, whether it’s with Java’s “Write Once, Run Anywhere” philosophy or C++’s powerful performance capabilities. The world of programming is vast and exciting, and both Java and C++ offer rewarding paths to explore.