Java vs C++: Key Differences Every Programmer Should Know
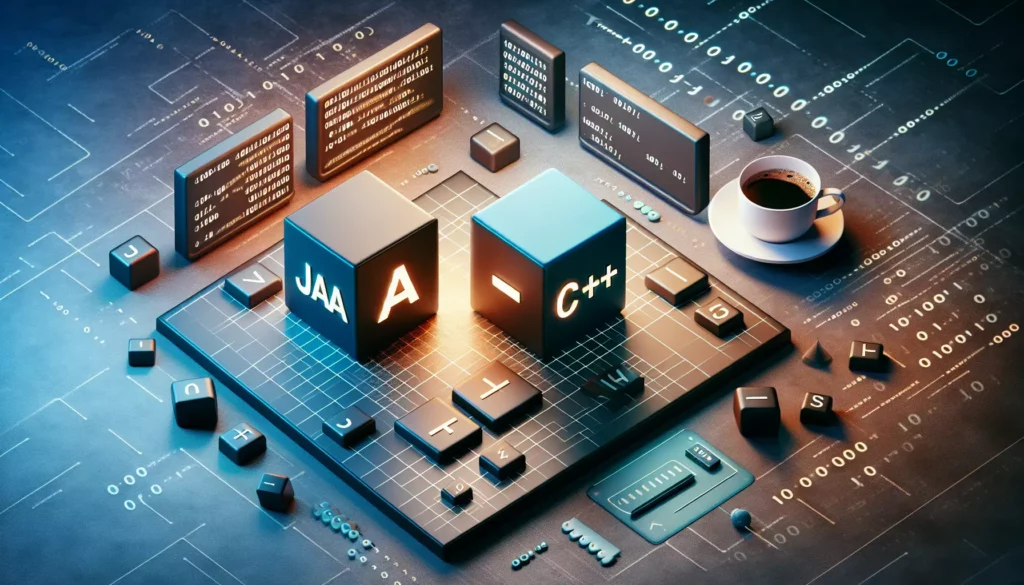
In the world of programming, Java and C++ stand as two of the most popular and influential languages. Both have their strengths, unique features, and specific use cases. Whether you’re a beginner looking to choose your first language or an experienced developer aiming to expand your skill set, understanding the key differences between Java and C++ is crucial. In this comprehensive guide, we’ll explore the main distinctions between these two powerhouse languages, helping you make informed decisions about which one to learn or use for your next project.
1. Language Type and Design Philosophy
Java: Pure Object-Oriented Programming
Java was designed from the ground up as a purely object-oriented programming (OOP) language. This means that in Java, everything (except for primitive data types) is an object. The language enforces OOP principles, making it easier for developers to write modular, reusable, and maintainable code.
C++: Multi-paradigm Programming
C++, on the other hand, is a multi-paradigm language. While it supports object-oriented programming, it also allows for procedural and generic programming. This flexibility gives developers more control over their coding style but can also lead to more complex and potentially error-prone code if not managed carefully.
2. Platform Independence
Java: Write Once, Run Anywhere
One of Java’s most significant advantages is its platform independence. Java code is compiled into bytecode, which can run on any device with a Java Virtual Machine (JVM). This “Write Once, Run Anywhere” (WORA) principle makes Java highly portable across different operating systems and devices.
C++: Platform-Dependent Compilation
C++ code is typically compiled directly into machine code for a specific platform. This means that C++ programs often need to be recompiled for different operating systems or architectures. While this can result in more optimized performance for specific platforms, it reduces portability compared to Java.
3. Memory Management
Java: Automatic Memory Management
Java uses automatic memory management through its garbage collection system. This means that developers don’t need to explicitly allocate or deallocate memory for objects. The JVM automatically handles memory management, which can significantly reduce memory-related errors and make programming easier, especially for beginners.
C++: Manual Memory Management
C++ gives programmers direct control over memory allocation and deallocation. While this allows for more fine-tuned memory management and potentially better performance, it also puts the responsibility of proper memory handling on the developer. This can lead to issues like memory leaks or dangling pointers if not managed correctly.
4. Performance
Java: Interpreted Language with JIT Compilation
Java is often considered slower than C++ due to its interpreted nature and the overhead of the JVM. However, modern Java implementations use Just-In-Time (JIT) compilation to optimize performance, narrowing the gap with C++. For most applications, Java’s performance is more than adequate, and the ease of development often outweighs minor performance differences.
C++: Compiled Language with Direct Hardware Access
C++ is generally faster than Java, especially for system-level programming or performance-critical applications. The direct compilation to machine code and the ability to manage memory manually allow for highly optimized code. This makes C++ a preferred choice for applications where every millisecond counts, such as game engines or high-frequency trading systems.
5. Syntax and Language Features
Java: Simplified Syntax
Java’s syntax is generally considered simpler and more readable than C++. It eliminates many complex features of C++, such as multiple inheritance and operator overloading, in favor of a cleaner, more straightforward syntax. This simplification makes Java easier to learn and less prone to certain types of errors.
C++: More Complex but Powerful Syntax
C++ offers a wider range of syntax features, including multiple inheritance, operator overloading, and templates. While these features provide more power and flexibility to experienced developers, they can also make the language more difficult to learn and increase the potential for complex, hard-to-maintain code.
6. Standard Library and Ecosystem
Java: Rich Standard Library and Extensive Ecosystem
Java comes with a comprehensive standard library that provides a wide range of built-in functionalities. Additionally, the Java ecosystem is vast, with numerous third-party libraries and frameworks available for various purposes. This rich ecosystem makes Java particularly well-suited for enterprise-level applications, web development, and Android app development.
C++: Smaller Standard Library, Focused on Performance
C++’s standard library is smaller compared to Java’s but is highly efficient. It focuses on providing essential functionalities while allowing developers to create custom implementations for more specific needs. The C++ ecosystem, while not as extensive as Java’s, still offers a wide range of libraries and tools, particularly for system-level programming and high-performance computing.
7. Pointers and References
Java: No Direct Pointer Manipulation
Java does not allow direct manipulation of pointers. Instead, it uses references to objects, which are managed by the JVM. This design choice enhances security and reduces the likelihood of memory-related errors, but it also limits low-level memory access and manipulation.
C++: Full Support for Pointers
C++ provides full support for pointers, allowing direct memory manipulation. This gives developers more control over memory usage and can lead to more efficient code. However, it also increases the risk of errors such as buffer overflows or null pointer dereferences if not handled carefully.
8. Inheritance and Polymorphism
Java: Single Inheritance with Interfaces
Java supports single inheritance for classes, meaning a class can only inherit from one superclass. To compensate for this limitation, Java introduces the concept of interfaces, which allow for a form of multiple inheritance of behavior. This approach simplifies the inheritance hierarchy and reduces the complexity often associated with multiple inheritance.
C++: Multiple Inheritance
C++ supports multiple inheritance, allowing a class to inherit from multiple base classes. While this provides more flexibility in designing class hierarchies, it can also lead to complexities such as the diamond problem. C++ developers need to be more careful when designing class hierarchies to avoid potential conflicts and ambiguities.
9. Exception Handling
Java: Checked Exceptions
Java introduces the concept of checked exceptions, which are exceptions that must be either caught or declared in the method signature. This forces developers to consider and handle potential exceptions, leading to more robust error handling. However, it can also result in more verbose code and has been a subject of debate in the programming community.
C++: Unchecked Exceptions
C++ uses unchecked exceptions, which do not need to be explicitly caught or declared. This provides more flexibility but puts the responsibility of proper exception handling entirely on the developer. C++ also allows for more granular control over exception handling, including the ability to specify which exceptions a function might throw.
10. Operator Overloading
Java: No Operator Overloading
Java does not support operator overloading (except for the + operator with strings). This design decision was made to keep the language simple and prevent potential abuse or confusion that can arise from overloaded operators. Instead, Java encourages the use of methods with descriptive names to perform operations on objects.
C++: Full Support for Operator Overloading
C++ allows for operator overloading, giving developers the ability to define custom behaviors for operators when used with user-defined types. This can lead to more intuitive and expressive code, especially when working with mathematical or domain-specific types. However, it can also lead to confusion if overloaded operators are used in non-intuitive ways.
11. Generics/Templates
Java: Generics with Type Erasure
Java implements generics using type erasure, which means that generic type information is removed at compile-time. This approach ensures backward compatibility but can lead to limitations in how generics can be used. For example, you cannot create an instance of a generic type or use primitive types directly with generics.
C++: Templates with Full Type Information
C++ uses templates, which preserve full type information at compile-time. This allows for more powerful metaprogramming capabilities and can lead to more efficient code. However, it can also result in longer compilation times and larger binary sizes due to template instantiation.
12. Community and Industry Usage
Java: Widespread Enterprise Adoption
Java has a massive community and is widely used in enterprise environments. It’s the primary language for Android development and is extensively used in web development (especially backend systems), big data processing, and financial applications. The “write once, run anywhere” philosophy has made Java a popular choice for cross-platform development.
C++: Dominant in System-Level and Performance-Critical Applications
C++ has a strong presence in system-level programming, game development, and performance-critical applications. It’s widely used in operating systems, device drivers, embedded systems, and high-performance computing. The gaming industry, in particular, heavily relies on C++ for its performance benefits.
13. Learning Curve
Java: Generally Easier to Learn
Java is often considered easier to learn, especially for beginners. Its simplified syntax, automatic memory management, and extensive documentation make it more accessible. The enforced object-oriented approach also helps in understanding OOP concepts from the start.
C++: Steeper Learning Curve
C++ has a steeper learning curve due to its more complex features like manual memory management, pointers, and multiple inheritance. It requires a deeper understanding of how computers work at a lower level. However, this complexity also provides a solid foundation for understanding many programming concepts.
14. Concurrency and Multithreading
Java: Built-in Support for Concurrency
Java provides built-in support for concurrency through its java.util.concurrent package. It offers high-level concurrency APIs that make it easier to write multi-threaded applications. Java’s memory model also provides strong guarantees for thread safety when used correctly.
C++: Lower-Level Concurrency Support
C++ has traditionally relied on platform-specific libraries for concurrency. However, modern C++ (C++11 and later) introduced standardized threading support. While powerful, C++’s concurrency features are generally lower-level compared to Java’s, requiring more careful management by the developer.
15. Development Tools and IDEs
Java: Rich IDE Support
Java has excellent IDE support with powerful tools like IntelliJ IDEA, Eclipse, and NetBeans. These IDEs offer advanced features like code completion, refactoring, and debugging, which can significantly boost developer productivity.
C++: Varied IDE Options
C++ also has strong IDE support with options like Visual Studio, CLion, and Code::Blocks. However, the setup and configuration of C++ development environments can be more complex, especially when dealing with different compilers and build systems.
Conclusion: Choosing Between Java and C++
Choosing between Java and C++ depends on various factors, including the specific requirements of your project, your personal preferences, and your career goals. Here are some general guidelines:
- Choose Java if:
- You’re new to programming and want an easier learning curve
- You’re developing cross-platform applications
- You’re working on enterprise-level software or web applications
- You’re interested in Android app development
- You prefer automatic memory management and a simpler syntax
- Choose C++ if:
- You need maximum performance and low-level hardware control
- You’re working on system-level programming or embedded systems
- You’re developing games or high-performance applications
- You want more control over memory management and system resources
- You’re interested in template metaprogramming and generic programming
Remember, many successful developers are proficient in both languages, as they each have their strengths and ideal use cases. Understanding the differences between Java and C++ not only helps in choosing the right tool for the job but also broadens your perspective as a programmer, making you more versatile and adaptable in the ever-evolving world of software development.
As you continue your journey in programming, whether you choose Java, C++, or both, remember that the key to success lies in continuous learning, practice, and application of your skills to real-world problems. Happy coding!