Is JavaScript Losing Its Relevance in 2024?
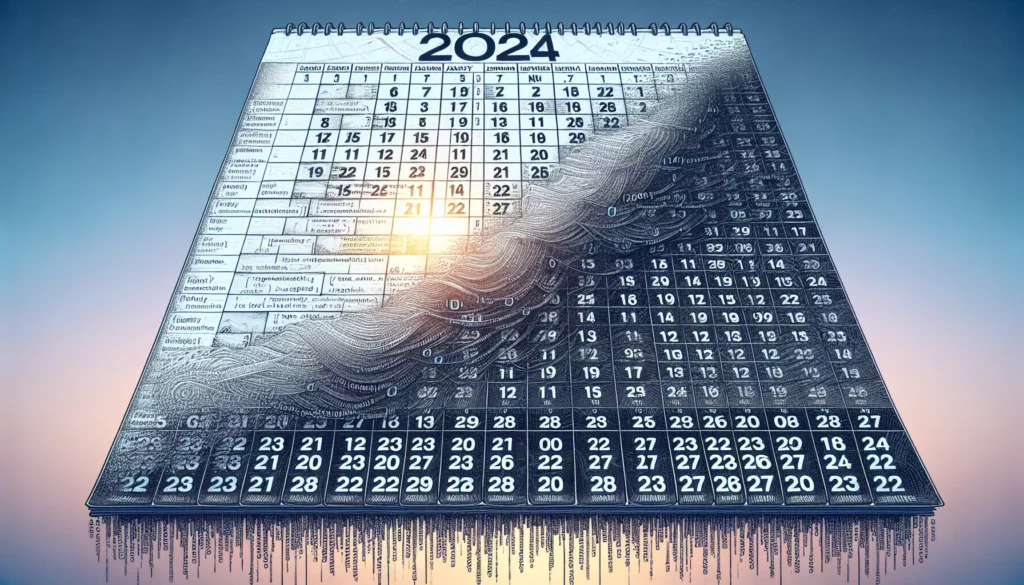
As we dive into 2024, a question that’s been circulating in tech circles is whether JavaScript, one of the most popular programming languages of the past decades, is losing its relevance. This question is particularly pertinent for aspiring developers and those looking to enhance their coding skills. At AlgoCademy, where we focus on providing comprehensive coding education and preparing learners for technical interviews at top tech companies, we believe it’s crucial to address this topic head-on.
The Rise of JavaScript
Before we can assess JavaScript’s current standing, it’s important to understand its journey. JavaScript was created in 1995 by Brendan Eich and has since become an integral part of web development. Its ability to make web pages interactive and dynamic revolutionized the internet, transforming static HTML pages into rich, responsive applications.
Over the years, JavaScript has evolved significantly:
- The introduction of frameworks like jQuery, Angular, React, and Vue.js expanded its capabilities.
- Node.js brought JavaScript to the server-side, allowing full-stack development with a single language.
- ECMAScript updates have continually added new features and improved the language’s syntax and functionality.
This evolution has kept JavaScript at the forefront of web development for nearly three decades. But as we enter 2024, the tech landscape is changing rapidly. New technologies and programming paradigms are emerging, leading some to question JavaScript’s continued relevance.
The Current State of JavaScript
As of 2024, JavaScript still holds a dominant position in web development. According to the latest Stack Overflow Developer Survey, it remains one of the most widely used programming languages. Its ecosystem is vast, with a plethora of libraries and frameworks that cater to various development needs.
Key strengths that maintain JavaScript’s relevance include:
- Ubiquity: JavaScript is supported by all modern web browsers, making it a universal language for client-side scripting.
- Versatility: From front-end to back-end, desktop to mobile apps, JavaScript can be used across various platforms.
- Rich Ecosystem: The npm registry, JavaScript’s package manager, hosts millions of packages, providing solutions for almost any development need.
- Community Support: A large, active community contributes to ongoing development and problem-solving.
- Constant Evolution: Regular updates to the ECMAScript specification keep the language modern and relevant.
Challenges to JavaScript’s Dominance
Despite its strong position, JavaScript faces several challenges that could potentially affect its relevance:
1. The Rise of WebAssembly
WebAssembly (Wasm) is a binary instruction format for a stack-based virtual machine that runs in web browsers. It’s designed to be faster to load and execute than JavaScript, and it allows languages like C, C++, and Rust to run in the browser at near-native speed.
Example of a simple WebAssembly module in JavaScript:
(async () => {
const response = await fetch("simple.wasm");
const bytes = await response.arrayBuffer();
const { instance } = await WebAssembly.instantiate(bytes);
console.log(instance.exports.add(1, 2));
})();
While WebAssembly isn’t designed to replace JavaScript entirely, it does provide an alternative for performance-critical parts of web applications.
2. TypeScript’s Growing Popularity
TypeScript, a statically typed superset of JavaScript, has gained significant traction. It addresses some of JavaScript’s shortcomings, particularly in large-scale applications, by adding optional static typing and other features that enhance code quality and maintainability.
Example of TypeScript code:
interface User {
id: number;
name: string;
email: string;
}
function greetUser(user: User): string {
return `Hello, ${user.name}!`;
}
const user: User = { id: 1, name: "Alice", email: "alice@example.com" };
console.log(greetUser(user));
While TypeScript compiles to JavaScript, its growing adoption could be seen as a shift away from pure JavaScript development.
3. The Complexity of the JavaScript Ecosystem
The vast JavaScript ecosystem, once a strength, is now sometimes criticized for its complexity. The multitude of frameworks, libraries, and tools can be overwhelming for newcomers and can lead to decision fatigue for experienced developers.
4. Performance Concerns
Despite significant improvements in JavaScript engines, there are still scenarios where JavaScript’s performance lags behind compiled languages. This is particularly noticeable in computationally intensive tasks or when dealing with large amounts of data.
5. The Push for Native Development
For mobile and desktop applications, there’s been a renewed interest in native development using languages like Swift, Kotlin, or C#. While JavaScript frameworks like React Native and Electron remain popular for cross-platform development, they sometimes face criticism for performance and user experience compared to native apps.
JavaScript’s Adaptation and Evolution
In response to these challenges, JavaScript and its ecosystem continue to evolve:
1. ECMAScript Advancements
The language itself is continuously improving. Recent ECMAScript versions have introduced features like async/await, optional chaining, and nullish coalescing, making the language more expressive and easier to use.
Example of modern JavaScript features:
// Async/Await
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log(data);
} catch (error) {
console.error("Failed to fetch data:", error);
}
}
// Optional Chaining and Nullish Coalescing
const user = {
name: "Bob",
address: {
street: "123 Main St"
}
};
const zipCode = user.address?.zipCode ?? "Unknown";
console.log(zipCode); // Output: "Unknown"
2. Performance Optimizations
JavaScript engines like V8 (used in Chrome and Node.js) continue to implement optimizations that improve JavaScript’s performance. Just-in-Time (JIT) compilation and other techniques have significantly narrowed the performance gap between JavaScript and compiled languages in many scenarios.
3. Integration with WebAssembly
Rather than competing with WebAssembly, the JavaScript community is embracing it. Many projects now use WebAssembly for performance-critical parts while using JavaScript for the rest of the application, leveraging the strengths of both technologies.
4. Framework Innovation
JavaScript frameworks continue to innovate. For example, React has introduced features like Hooks and Concurrent Mode, while Vue.js 3 brought improved performance and the Composition API. These innovations keep JavaScript development fresh and efficient.
Example of React Hooks:
import React, { useState, useEffect } from "react";
function ExampleComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
5. Serverless and Edge Computing
JavaScript’s role in serverless computing and edge functions is growing. Platforms like Cloudflare Workers and Vercel Edge Functions allow JavaScript to run closer to the user, enabling new architectures for web applications.
The Role of JavaScript in Modern Web Development
As we assess JavaScript’s relevance in 2024, it’s clear that while the language faces challenges, it remains a cornerstone of web development. Its role, however, is evolving:
1. Full-Stack Development
With Node.js on the server-side and various frameworks on the client-side, JavaScript continues to be a go-to choice for full-stack development. This allows developers to use a single language across the entire stack, streamlining development processes.
2. Single-Page Applications (SPAs)
JavaScript frameworks like React, Vue, and Angular remain the primary tools for building complex, interactive single-page applications. These frameworks have matured, offering robust solutions for state management, routing, and performance optimization.
3. Progressive Web Apps (PWAs)
JavaScript is crucial in developing Progressive Web Apps, which offer native-like experiences on the web. Service Workers, a key component of PWAs, are implemented in JavaScript.
Example of a simple Service Worker:
self.addEventListener("install", (event) => {
event.waitUntil(
caches.open("v1").then((cache) => {
return cache.addAll([
"/",
"/index.html",
"/styles.css",
"/script.js"
]);
})
);
});
self.addEventListener("fetch", (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
4. Server-Side Rendering (SSR) and Static Site Generation (SSG)
Frameworks like Next.js and Gatsby use JavaScript for server-side rendering and static site generation, improving initial load times and SEO while maintaining the benefits of client-side JavaScript applications.
5. WebAssembly Integration
As mentioned earlier, JavaScript is increasingly used in conjunction with WebAssembly. This allows developers to use JavaScript for the bulk of their application while leveraging WebAssembly for performance-critical sections.
6. Cross-Platform Development
Frameworks like React Native for mobile apps and Electron for desktop apps continue to use JavaScript as a means of building cross-platform applications with a single codebase.
The Future of JavaScript
Looking ahead, several trends suggest that JavaScript will continue to evolve and maintain its relevance:
1. AI and Machine Learning Integration
Libraries like TensorFlow.js are bringing machine learning capabilities to JavaScript, opening up new possibilities for AI-powered web applications.
2. Improved Type Systems
While TypeScript has gained popularity, there are ongoing discussions about adding optional static typing to JavaScript itself. This could address one of the primary criticisms of the language without losing its dynamic nature.
3. Continued ECMAScript Evolution
The ECMAScript committee continues to work on new features and improvements. Proposals like the Pipeline Operator and Pattern Matching could further enhance JavaScript’s expressiveness and capabilities.
4. WebGPU and Advanced Graphics
As browsers implement WebGPU, JavaScript will gain access to more powerful graphics capabilities, potentially opening up new use cases in gaming and data visualization.
5. Quantum Computing
While still in its early stages, there are already efforts to bring quantum computing concepts to JavaScript, such as the QISKit.js library. As quantum computing advances, JavaScript may play a role in making it accessible to web developers.
Conclusion: JavaScript’s Ongoing Relevance
As we’ve explored throughout this article, while JavaScript faces challenges and competition from new technologies, it’s far from losing its relevance in 2024. Instead, it’s adapting and evolving to meet the changing needs of web development.
JavaScript’s strengths – its ubiquity, versatility, and vast ecosystem – continue to make it a valuable skill for developers. Its ability to work across the full stack, from front-end to back-end, serverless functions to desktop applications, ensures its continued importance in the development world.
However, the landscape is changing. Developers today need to be aware of complementary technologies like WebAssembly and TypeScript, and be prepared to use JavaScript in conjunction with these tools. The most successful developers will be those who can leverage JavaScript’s strengths while also understanding when and how to use other technologies to overcome its limitations.
At AlgoCademy, we believe in preparing developers not just for today’s technology landscape, but for the future as well. That’s why our curriculum includes JavaScript alongside other important languages and concepts. We focus on teaching fundamental programming principles and problem-solving skills that transcend any single language or framework.
Whether you’re just starting your coding journey or looking to advance your skills for technical interviews at top tech companies, understanding JavaScript and its ecosystem remains crucial. Its ongoing evolution and central role in web development make it a key language to master.
In conclusion, while the way we use JavaScript may be changing, its relevance in 2024 and beyond remains strong. As with any technology, the key is to stay curious, keep learning, and be ready to adapt to new developments. JavaScript’s journey is far from over, and it continues to offer exciting opportunities for developers willing to grow and evolve with it.