Is “Fail Fast” a Recipe for Bad Coding Habits?
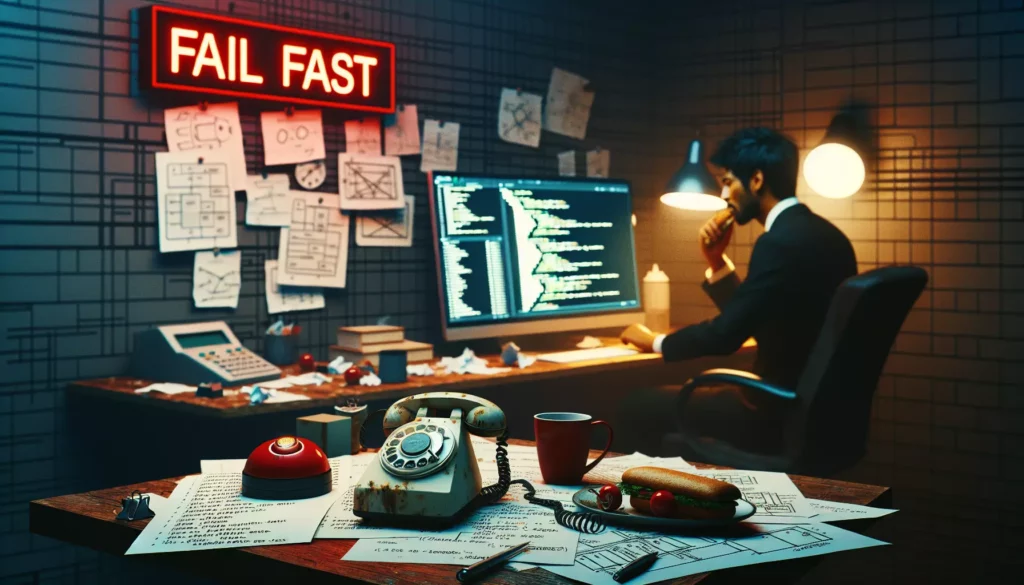
In the world of software development, the mantra “fail fast” has gained significant traction over the years. It’s a principle that encourages developers to quickly identify and address issues in their code. But as with many popular concepts in programming, it’s worth taking a closer look to understand its implications fully. Is “fail fast” truly a beneficial approach, or could it potentially lead to bad coding habits? Let’s dive deep into this topic and explore its various facets.
Understanding the “Fail Fast” Principle
Before we can evaluate whether “fail fast” might lead to bad coding habits, it’s crucial to understand what this principle actually means. The “fail fast” approach advocates for immediate and visible failure at the point where an error occurs. This is in contrast to systems that attempt to continue operating or return partial results when something goes wrong.
The core ideas behind “fail fast” include:
- Detecting errors as early as possible in the development process
- Making errors immediately visible and obvious
- Stopping execution at the point of failure rather than continuing with potentially corrupted state
- Providing clear and actionable error messages
Proponents of “fail fast” argue that this approach leads to more robust and reliable software by catching and addressing issues early in the development cycle.
The Benefits of Failing Fast
There are several compelling reasons why many developers and organizations have embraced the “fail fast” philosophy:
1. Early Problem Detection
By failing immediately when an error occurs, developers can identify and fix issues much earlier in the development process. This can save significant time and resources compared to discovering problems later, especially in production environments.
2. Improved Debugging
When a system fails fast, it’s often easier to pinpoint the exact location and cause of the error. This can greatly simplify the debugging process and reduce the time spent tracking down elusive bugs.
3. Enhanced Code Quality
The “fail fast” approach encourages developers to write more defensive code. By anticipating potential failure points and handling them explicitly, the overall quality and robustness of the codebase can improve.
4. Faster Development Cycles
By catching errors early and providing clear feedback, “fail fast” can lead to faster iteration cycles. Developers can quickly identify and fix issues, leading to more rapid progress and potentially shorter development timelines.
5. Increased Reliability
Systems that fail fast are often more reliable in the long run. By preventing the propagation of errors and invalid states, they can avoid more catastrophic failures that might occur if issues were allowed to persist undetected.
Potential Pitfalls of Failing Fast
While the benefits of “fail fast” are significant, there are potential downsides to consider:
1. Overemphasis on Errors
An excessive focus on failing fast might lead developers to become overly concerned with error handling at the expense of other important aspects of software design. This could result in code that’s more complex than necessary or that prioritizes error cases over normal operation.
2. Reduced Resilience
In some cases, a system that fails fast might be less resilient than one that attempts to recover or continue operation in the face of errors. This can be particularly problematic in mission-critical systems where any downtime is unacceptable.
3. User Experience Concerns
From a user perspective, a system that fails fast might appear less stable or user-friendly than one that gracefully handles errors and continues to provide partial functionality.
4. Potential for Overengineering
The desire to catch every possible error case can sometimes lead to overengineering, resulting in code that’s more complex and harder to maintain than necessary.
5. False Sense of Security
Relying too heavily on “fail fast” mechanisms might create a false sense of security, leading developers to neglect other important aspects of software quality such as thorough testing and code reviews.
Balancing “Fail Fast” with Good Coding Practices
The key to leveraging the benefits of “fail fast” while avoiding its potential pitfalls lies in balancing this approach with other good coding practices. Here are some strategies to consider:
1. Thoughtful Error Handling
Instead of blindly throwing exceptions or terminating execution at every potential error point, consider the context and criticality of each situation. Sometimes, logging an error and continuing execution might be more appropriate than failing fast.
2. Comprehensive Testing
While “fail fast” can help catch errors early, it’s not a substitute for thorough testing. Implement a robust testing strategy that includes unit tests, integration tests, and end-to-end tests to catch issues before they reach production.
3. Clear Error Messages
When implementing “fail fast” mechanisms, ensure that error messages are clear, informative, and actionable. This can greatly aid in debugging and improve the overall developer experience.
4. Graceful Degradation
Consider implementing graceful degradation strategies alongside “fail fast” approaches. This allows your system to maintain some level of functionality even when errors occur, improving user experience and system resilience.
5. Code Reviews and Best Practices
Regular code reviews and adherence to established best practices can help ensure that “fail fast” mechanisms are implemented appropriately and don’t lead to overly complex or brittle code.
Implementing “Fail Fast” in Practice
Let’s look at some practical examples of how to implement “fail fast” principles in your code without falling into bad habits:
1. Input Validation
One of the most common applications of “fail fast” is in input validation. Here’s an example in Python:
def process_user_input(age):
if not isinstance(age, int) or age < 0 or age > 120:
raise ValueError("Age must be a positive integer between 0 and 120")
# Process the age...
return f"User is {age} years old"
try:
result = process_user_input(25)
print(result)
except ValueError as e:
print(f"Error: {e}")
In this example, we’re failing fast by immediately raising an exception if the input doesn’t meet our criteria. This prevents invalid data from propagating through our system.
2. Null Checks
Another common use of “fail fast” is in null checks. Here’s an example in Java:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = Objects.requireNonNull(name, "Name cannot be null");
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
this.age = age;
}
// Other methods...
}
Here, we’re using Java’s Objects.requireNonNull()
method to fail fast if a null value is passed for the name. We’re also checking for negative age values and failing fast in that case as well.
3. Early Returns
Sometimes, “fail fast” can be implemented using early returns rather than exceptions. Here’s an example in JavaScript:
function processOrder(order) {
if (!order) {
console.error("Order is null or undefined");
return false;
}
if (!order.items || order.items.length === 0) {
console.error("Order has no items");
return false;
}
if (order.total <= 0) {
console.error("Order total must be positive");
return false;
}
// Process the order...
return true;
}
In this example, we’re checking for various error conditions and returning early if any of them are met. This prevents the function from continuing with invalid data.
When Not to Fail Fast
While “fail fast” is a useful principle in many situations, there are times when it might not be the best approach:
1. User-Facing Applications
In user-facing applications, it’s often better to handle errors gracefully and provide a smooth user experience rather than failing immediately. For example, if a user enters invalid data in a form, it’s usually better to display an error message and allow them to correct the input rather than crashing the entire application.
2. Long-Running Processes
For long-running processes or batch jobs, failing fast on every minor error might not be practical. In these cases, it might be better to log errors, skip problematic items, and continue processing.
3. Distributed Systems
In distributed systems, network partitions and temporary failures are common. Designing these systems to fail fast on every communication error could lead to unnecessary downtime. Instead, techniques like retries, circuit breakers, and eventual consistency are often more appropriate.
4. Non-Critical Functionality
For non-critical parts of an application, it might be overkill to fail fast on every possible error. For instance, if a non-essential feature fails to load, it might be better to simply disable that feature and allow the rest of the application to function normally.
Alternatives to Failing Fast
While “fail fast” is a valuable approach in many scenarios, it’s worth considering alternative strategies that might be more appropriate in certain situations:
1. Graceful Degradation
Instead of failing completely, a system can be designed to degrade gracefully when errors occur. This might involve disabling certain features or falling back to a simpler mode of operation.
2. Retry Mechanisms
For transient errors, implementing retry mechanisms with exponential backoff can often resolve issues without the need for immediate failure.
3. Circuit Breakers
In distributed systems, circuit breakers can be used to prevent cascading failures by temporarily disabling problematic components.
4. Asynchronous Error Handling
In some cases, errors can be handled asynchronously, allowing the main execution path to continue while error processing happens in the background.
Conclusion: Finding the Right Balance
The “fail fast” principle, when applied judiciously, can indeed lead to more robust, reliable, and maintainable code. It encourages developers to think critically about potential failure points and handle them explicitly, which can prevent subtle bugs and improve overall system stability.
However, like any programming principle, “fail fast” should not be treated as a universal solution. Blindly applying it without consideration for the specific context and requirements of your system can indeed lead to bad coding habits, such as overly complex error handling, reduced system resilience, or poor user experience.
The key is to find the right balance. Use “fail fast” where it makes sense – typically in core logic, data processing, and areas where catching errors early can prevent more serious issues down the line. But also be prepared to use other error handling strategies where appropriate, especially in user-facing components or distributed systems where some level of fault tolerance is necessary.
Remember that “fail fast” is just one tool in a developer’s toolkit. It should be used in conjunction with other good coding practices such as:
- Comprehensive testing
- Clear and consistent error messaging
- Regular code reviews
- Thoughtful system design that considers both happy paths and error scenarios
- Continuous monitoring and logging in production environments
By combining “fail fast” with these practices, you can create systems that are both robust in the face of errors and provide a good experience for both developers and end-users.
Ultimately, the goal is not to fail fast for the sake of failing fast, but to create software that behaves predictably, fails gracefully when necessary, and provides clear feedback when things go wrong. This approach leads to systems that are easier to develop, maintain, and debug – which is the true measure of good coding habits.