Is Competitive Programming Necessary for Software Engineering Jobs?
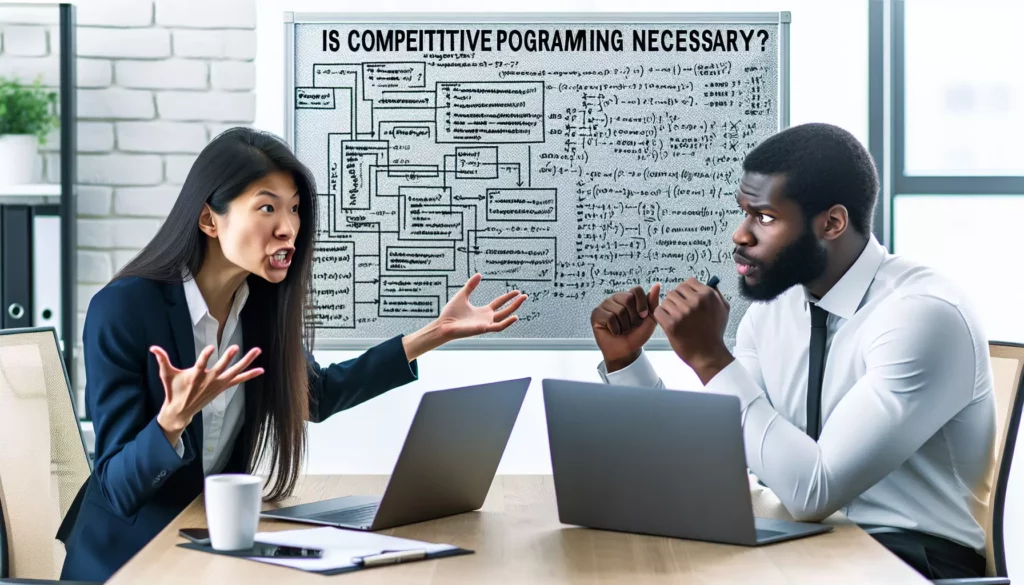
In the ever-evolving landscape of software engineering, aspiring developers often find themselves pondering the importance of competitive programming. As the tech industry continues to grow and job markets become increasingly competitive, many wonder if honing skills through coding competitions is a prerequisite for landing coveted software engineering positions. This article aims to explore the relationship between competitive programming and software engineering careers, weighing the benefits and potential drawbacks to help you make an informed decision about your learning path.
Understanding Competitive Programming
Before diving into its necessity for software engineering jobs, let’s first define what competitive programming entails:
Competitive programming is a mind sport that involves participants solving well-defined computational problems within a limited time frame. These competitions typically focus on algorithmic and mathematical challenges, requiring contestants to write efficient code that solves complex problems quickly and accurately.
Some popular competitive programming platforms include:
- Codeforces
- LeetCode
- HackerRank
- TopCoder
- AtCoder
These platforms host regular contests and provide a vast array of practice problems for programmers to sharpen their skills.
The Benefits of Competitive Programming
While opinions on the necessity of competitive programming for software engineering jobs may vary, there are undeniable benefits to engaging in this practice:
1. Enhanced Problem-Solving Skills
Competitive programming challenges participants to think critically and approach problems from multiple angles. This cultivates a problem-solving mindset that is invaluable in real-world software development scenarios.
2. Improved Algorithmic Thinking
Regular participation in coding competitions exposes programmers to a wide variety of algorithmic concepts and data structures. This knowledge can be directly applied to optimize code in professional settings.
3. Code Efficiency and Optimization
The time constraints in competitive programming force participants to write not just correct code, but highly efficient code. This skill translates well to industry scenarios where performance optimization is crucial.
4. Faster Coding Speed
Practice makes perfect, and competitive programming provides ample opportunity to code frequently. This naturally leads to increased coding speed, which can be beneficial in time-sensitive work environments.
5. Preparation for Technical Interviews
Many tech companies, especially larger ones like Google, Facebook, and Amazon, incorporate algorithmic problems in their interview processes. Experience with competitive programming can give candidates an edge in these situations.
The Limitations of Competitive Programming
While competitive programming offers numerous benefits, it’s essential to recognize its limitations in the context of software engineering careers:
1. Narrow Focus
Competitive programming primarily emphasizes algorithms and data structures, which are just a subset of the skills required in software engineering. It doesn’t cover other crucial aspects such as:
- Software design and architecture
- Version control systems (e.g., Git)
- Collaborative coding practices
- Testing and debugging in large-scale systems
- DevOps and deployment processes
2. Unrealistic Time Constraints
The pressure to solve problems quickly in competitive programming doesn’t always align with real-world development scenarios, where thoughtful planning and careful implementation are often more valuable than speed.
3. Lack of Software Engineering Best Practices
Competitive programming solutions often prioritize brevity and speed over code readability, maintainability, and scalability – qualities that are essential in professional software development.
4. Limited Scope of Technologies
Most competitive programming platforms focus on a handful of programming languages and don’t cover the wide array of frameworks, libraries, and tools used in modern software development.
5. Potential for Burnout
The intense nature of competitive programming can lead to burnout if not balanced with other aspects of learning and development.
The Role of Platforms Like AlgoCademy
In the context of this discussion, it’s worth mentioning platforms like AlgoCademy that aim to bridge the gap between competitive programming skills and practical software engineering knowledge. AlgoCademy offers a more holistic approach to coding education by:
- Providing interactive coding tutorials that cover both algorithmic thinking and real-world programming scenarios
- Offering resources for learners at various skill levels, from beginners to those preparing for technical interviews
- Incorporating AI-powered assistance to guide learners through problem-solving processes
- Emphasizing the development of practical coding skills alongside algorithmic proficiency
Such platforms recognize the value of competitive programming skills while also addressing the broader needs of aspiring software engineers.
What Do Employers Really Want?
To determine whether competitive programming is necessary for software engineering jobs, it’s crucial to understand what employers are looking for. While requirements can vary depending on the company and specific role, some common desirable traits include:
1. Strong Foundational Knowledge
Employers value candidates with a solid understanding of computer science fundamentals, including data structures, algorithms, and system design principles.
2. Problem-Solving Abilities
The capacity to approach complex problems methodically and devise effective solutions is highly prized in the software engineering field.
3. Practical Coding Skills
Proficiency in relevant programming languages and the ability to write clean, maintainable code are essential for most software engineering positions.
4. Collaboration and Communication
Software engineering often involves working in teams, so strong interpersonal skills and the ability to communicate technical concepts clearly are important.
5. Continuous Learning
The tech industry evolves rapidly, and employers look for candidates who demonstrate a commitment to ongoing learning and adaptation to new technologies.
6. Project Experience
Many employers value candidates who have worked on real-world projects, either through internships, open-source contributions, or personal endeavors.
Striking a Balance: Integrating Competitive Programming into Your Learning Journey
Given the benefits and limitations of competitive programming, as well as the diverse requirements of software engineering roles, a balanced approach to skill development is often the most effective strategy. Here are some ways to incorporate competitive programming into your learning journey without neglecting other crucial areas:
1. Use Competitive Programming as a Supplement
Rather than making it the sole focus of your studies, use competitive programming as a tool to enhance your problem-solving skills and algorithmic thinking. Dedicate a portion of your learning time to solving coding challenges, but ensure it’s part of a broader curriculum.
2. Apply Learnings to Real-World Projects
Take the algorithmic concepts and optimization techniques you learn from competitive programming and apply them to practical projects. This helps bridge the gap between theoretical knowledge and real-world application.
3. Balance Speed with Best Practices
While practicing competitive programming, also focus on writing clean, well-documented code. This dual approach will prepare you for both technical interviews and professional coding standards.
4. Explore Different Types of Problems
Don’t limit yourself to the types of problems commonly found in coding competitions. Explore a wide range of programming challenges, including those that mimic real-world scenarios you might encounter in a software engineering role.
5. Collaborate and Learn from Others
Participate in team coding competitions or join coding communities where you can collaborate with others. This helps develop the teamwork and communication skills valued in professional settings.
6. Stay Updated with Industry Trends
While honing your competitive programming skills, keep abreast of current software development practices, tools, and frameworks. This rounded knowledge will make you a more attractive candidate to potential employers.
Code Example: Applying Competitive Programming Skills to Real-World Scenarios
To illustrate how competitive programming skills can be applied in a more practical context, let’s consider an example. Here’s a common competitive programming problem and how it might be adapted for a real-world scenario:
Competitive Programming Version: Two Sum
Problem: Given an array of integers and a target sum, return indices of two numbers such that they add up to the target.
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int, int> map;
for (int i = 0; i < nums.size(); i++) {
int complement = target - nums[i];
if (map.find(complement) != map.end()) {
return {map[complement], i};
}
map[nums[i]] = i;
}
return {};
}
};
Real-World Adaptation: Product Recommendation System
In a real-world scenario, we might adapt this concept to create a simple product recommendation system:
class RecommendationSystem {
private:
unordered_map<int, vector<Product>> priceToProducts;
public:
void addProduct(const Product& product) {
priceToProducts[product.getPrice()].push_back(product);
}
vector<pair<Product, Product>> findComplementaryProducts(int targetPrice) {
vector<pair<Product, Product>> recommendations;
for (const auto& entry : priceToProducts) {
int price1 = entry.first;
int price2 = targetPrice - price1;
if (priceToProducts.find(price2) != priceToProducts.end()) {
for (const Product& prod1 : entry.second) {
for (const Product& prod2 : priceToProducts[price2]) {
if (prod1.getId() != prod2.getId()) {
recommendations.push_back({prod1, prod2});
}
}
}
}
}
return recommendations;
}
};
This example demonstrates how the core concept from a competitive programming problem (finding two numbers that sum to a target) can be adapted to solve a practical business problem (recommending complementary products that fit a target price range).
Conclusion: Is Competitive Programming Necessary?
In conclusion, while competitive programming is not strictly necessary for all software engineering jobs, it can be a valuable tool in a programmer’s skill development arsenal. The problem-solving skills, algorithmic thinking, and coding efficiency gained from competitive programming can certainly give candidates an edge, especially in technical interviews for companies that emphasize these skills.
However, it’s crucial to recognize that competitive programming alone is not sufficient preparation for a career in software engineering. A well-rounded approach that combines algorithmic proficiency with practical coding experience, software design knowledge, and strong soft skills is likely to be the most effective strategy for aspiring software engineers.
Platforms like AlgoCademy recognize this need for balance and aim to provide a more comprehensive learning experience. By combining elements of competitive programming with practical, real-world coding scenarios, these platforms can help learners develop a diverse skill set that’s well-suited to the demands of the software engineering industry.
Ultimately, the decision to engage in competitive programming should be based on your personal career goals, learning style, and the specific requirements of the roles you’re targeting. Whether you choose to dive deep into competitive programming or opt for a more balanced approach, the key is to maintain a commitment to continuous learning and improvement in this dynamic and exciting field.