Introduction to Web Development Basics: HTML, CSS, and JavaScript
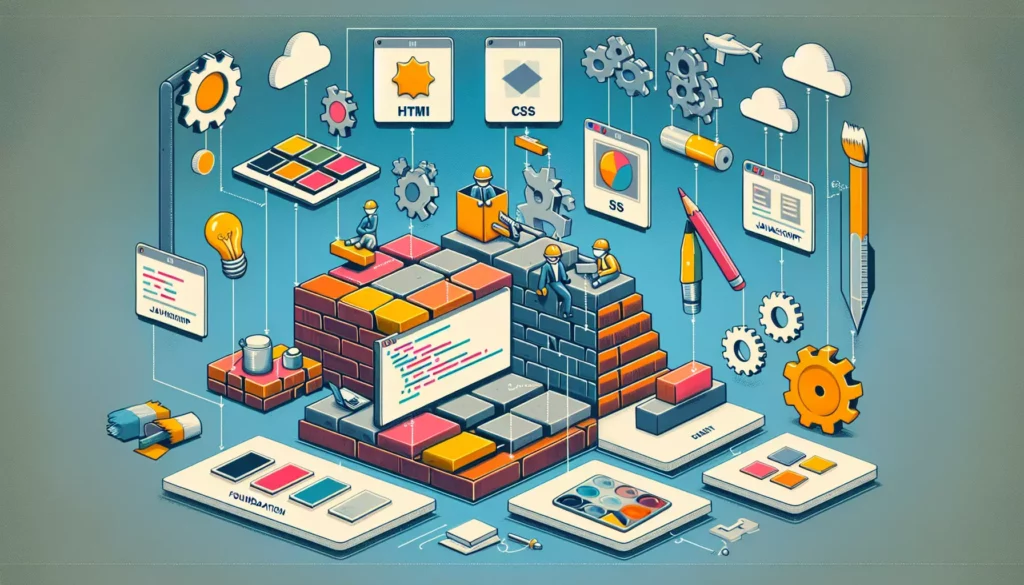
Welcome to the exciting world of web development! If you’re looking to start your journey in creating websites and web applications, you’ve come to the right place. In this comprehensive guide, we’ll explore the fundamental building blocks of web development: HTML, CSS, and JavaScript. These three technologies form the core of modern web development and are essential skills for any aspiring web developer.
Whether you’re a complete beginner or have some programming experience, this article will provide you with a solid foundation in web development basics. We’ll cover each technology in detail, explain how they work together, and provide practical examples to help you get started. By the end of this guide, you’ll have a clear understanding of how to create basic web pages and add interactivity to them.
Table of Contents
- HTML: The Structure of Web Pages
- CSS: Styling and Layout
- JavaScript: Adding Interactivity
- Putting It All Together
- Tools and Resources for Web Development
- Conclusion
1. HTML: The Structure of Web Pages
HTML (HyperText Markup Language) is the backbone of every web page. It provides the structure and content of a webpage, defining elements such as headings, paragraphs, images, links, and more. Let’s dive into the basics of HTML:
HTML Document Structure
Every HTML document follows a basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Web Page</title>
</head>
<body>
<!-- Your content goes here -->
</body>
</html>
Let’s break down this structure:
<!DOCTYPE html>
: Declares that this is an HTML5 document<html>
: The root element of the HTML page<head>
: Contains meta-information about the document<meta>
: Provides metadata about the HTML document<title>
: Specifies a title for the document<body>
: Defines the document’s body, which contains all the visible contents
Common HTML Elements
HTML uses tags to define elements. Here are some common elements you’ll use frequently:
- Headings:
<h1>
to<h6>
- Paragraphs:
<p>
- Links:
<a href="url">
- Images:
<img src="image.jpg" alt="description">
- Lists:
<ul>
(unordered) and<ol>
(ordered) with<li>
for list items - Div:
<div>
for grouping elements - Span:
<span>
for inline grouping
Example: A Simple HTML Page
Let’s create a simple HTML page with some common elements:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Web Page</title>
</head>
<body>
<h1>Welcome to My First Web Page</h1>
<p>This is a paragraph of text. HTML is fun to learn!</p>
<h2>My Favorite Foods</h2>
<ul>
<li>Pizza</li>
<li>Sushi</li>
<li>Ice Cream</li>
</ul>
<img src="https://example.com/image.jpg" alt="A delicious pizza">
<p>Learn more about HTML on <a href="https://developer.mozilla.org/en-US/docs/Web/HTML">MDN Web Docs</a>.</p>
</body>
</html>
This example demonstrates the use of headings, paragraphs, lists, images, and links. When you open this HTML file in a web browser, you’ll see a basic web page with structured content.
2. CSS: Styling and Layout
While HTML provides the structure and content of a web page, CSS (Cascading Style Sheets) is responsible for its presentation. CSS allows you to control the layout, colors, fonts, and other visual aspects of your HTML elements. Let’s explore the basics of CSS:
CSS Syntax
CSS uses a simple syntax to apply styles to HTML elements:
selector {
property: value;
}
- Selector: Targets the HTML element(s) to style
- Property: Specifies what aspect of the element to change
- Value: Defines the new style for the property
Ways to Include CSS
There are three ways to include CSS in your HTML document:
- Inline CSS: Applied directly to HTML elements using the
style
attribute - Internal CSS: Placed within a
<style>
tag in the HTML<head>
section - External CSS: Stored in a separate .css file and linked to the HTML using the
<link>
tag
Common CSS Properties
Here are some frequently used CSS properties:
color
: Sets the text colorbackground-color
: Sets the background colorfont-family
: Specifies the fontfont-size
: Sets the font sizemargin
: Controls the outer spacing of an elementpadding
: Controls the inner spacing of an elementborder
: Adds a border around an elementwidth
andheight
: Set the dimensions of an elementdisplay
: Controls how an element is displayed (block, inline, flex, etc.)
Example: Adding CSS to Our HTML Page
Let’s add some CSS to style our previous HTML example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Styled Web Page</title>
<style>
body {
font-family: Arial, sans-serif;
line-height: 1.6;
margin: 0;
padding: 20px;
background-color: #f4f4f4;
}
h1 {
color: #333;
text-align: center;
}
h2 {
color: #666;
}
p {
margin-bottom: 10px;
}
ul {
background-color: #fff;
padding: 20px;
border-radius: 5px;
}
li {
margin-bottom: 5px;
}
img {
max-width: 100%;
height: auto;
display: block;
margin: 20px auto;
}
a {
color: #0066cc;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
</style>
</head>
<body>
<h1>Welcome to My Styled Web Page</h1>
<p>This is a paragraph of text. HTML and CSS work great together!</p>
<h2>My Favorite Foods</h2>
<ul>
<li>Pizza</li>
<li>Sushi</li>
<li>Ice Cream</li>
</ul>
<img src="https://example.com/image.jpg" alt="A delicious pizza">
<p>Learn more about CSS on <a href="https://developer.mozilla.org/en-US/docs/Web/CSS">MDN Web Docs</a>.</p>
</body>
</html>
In this example, we’ve added an internal CSS section within the <style>
tag. This CSS applies various styles to our HTML elements, including fonts, colors, spacing, and more. When you view this page in a browser, you’ll see a much more visually appealing layout compared to the unstyled HTML.
3. JavaScript: Adding Interactivity
JavaScript is a powerful programming language that allows you to add interactivity and dynamic behavior to your web pages. It can manipulate HTML and CSS, handle events, make asynchronous requests, and much more. Let’s explore the basics of JavaScript:
JavaScript Syntax
JavaScript uses a C-style syntax. Here are some basic concepts:
- Variables: Declared using
let
,const
, orvar
- Functions: Blocks of reusable code
- Conditionals:
if
,else if
,else
statements - Loops:
for
,while
,do...while
- Objects: Collections of related data and/or functionality
- Arrays: Ordered lists of values
Including JavaScript in HTML
You can include JavaScript in your HTML document in two ways:
- Internal JavaScript: Place code within
<script>
tags in the HTML file - External JavaScript: Link to an external .js file using
<script src="script.js"></script>
DOM Manipulation
One of the most powerful features of JavaScript is its ability to manipulate the Document Object Model (DOM). The DOM is a programming interface for HTML documents, allowing you to change the document structure, style, and content dynamically.
Example: Adding JavaScript to Our Web Page
Let’s enhance our previous example with some JavaScript functionality:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Web Page</title>
<style>
/* Previous CSS styles here */
.highlight {
background-color: yellow;
}
</style>
</head>
<body>
<h1>Welcome to My Interactive Web Page</h1>
<p>This is a paragraph of text. HTML, CSS, and JavaScript work great together!</p>
<h2>My Favorite Foods</h2>
<ul id="foodList">
<li>Pizza</li>
<li>Sushi</li>
<li>Ice Cream</li>
</ul>
<button id="addFood">Add Food</button>
<img src="https://example.com/image.jpg" alt="A delicious pizza">
<p>Learn more about JavaScript on <a href="https://developer.mozilla.org/en-US/docs/Web/JavaScript">MDN Web Docs</a>.</p>
<script>
// Function to add a new food item
function addFoodItem() {
const foodList = document.getElementById('foodList');
const newFood = prompt('Enter a new food:');
if (newFood) {
const li = document.createElement('li');
li.textContent = newFood;
foodList.appendChild(li);
}
}
// Add click event listener to the button
document.getElementById('addFood').addEventListener('click', addFoodItem);
// Function to highlight list items on hover
function highlightItem(event) {
if (event.target.tagName === 'LI') {
event.target.classList.toggle('highlight');
}
}
// Add mouseover and mouseout event listeners to the list
const foodList = document.getElementById('foodList');
foodList.addEventListener('mouseover', highlightItem);
foodList.addEventListener('mouseout', highlightItem);
</script>
</body>
</html>
In this example, we’ve added JavaScript to make our web page interactive:
- A button that allows users to add new food items to the list
- A hover effect that highlights list items when the mouse is over them
These features demonstrate how JavaScript can manipulate the DOM and respond to user events, creating a more dynamic and engaging web page.
4. Putting It All Together
Now that we’ve covered the basics of HTML, CSS, and JavaScript, let’s look at how these technologies work together to create modern web pages and applications:
- HTML provides the structure and content of the web page.
- CSS styles the HTML elements, controlling layout and appearance.
- JavaScript adds interactivity and dynamic behavior to the page.
This separation of concerns allows for more maintainable and scalable web development. Each technology has its specific role, but they work together seamlessly to create the full web experience.
Best Practices
As you continue your journey in web development, keep these best practices in mind:
- Use semantic HTML to improve accessibility and SEO
- Keep your CSS organized and use a consistent naming convention
- Write clean, commented JavaScript code
- Optimize your web pages for performance
- Ensure your designs are responsive and work well on various devices
- Stay updated with the latest web standards and best practices
5. Tools and Resources for Web Development
To help you on your web development journey, here are some essential tools and resources:
Text Editors and IDEs
- Visual Studio Code: A popular, free, and feature-rich code editor
- Sublime Text: A sophisticated text editor for code and markup
- WebStorm: A powerful IDE specifically designed for web development
Browser Developer Tools
Modern web browsers come with built-in developer tools that allow you to inspect and debug your web pages. Familiarize yourself with these tools in browsers like Chrome, Firefox, or Safari.
Online Learning Platforms
- MDN Web Docs: Comprehensive documentation and tutorials for web technologies
- freeCodeCamp: Free coding bootcamp with interactive lessons
- Codecademy: Interactive coding courses for various web technologies
- AlgoCademy: Interactive coding tutorials and resources for learners, with a focus on algorithmic thinking and problem-solving
Version Control
Learn to use Git and GitHub to manage your code and collaborate with others.
Package Managers
Familiarize yourself with npm (Node Package Manager) for managing JavaScript libraries and tools.
Frameworks and Libraries
As you advance, explore popular frameworks and libraries like React, Vue.js, or Angular for building more complex web applications.
6. Conclusion
Congratulations! You’ve taken your first steps into the world of web development. By understanding the basics of HTML, CSS, and JavaScript, you’ve laid a solid foundation for creating web pages and applications. Remember, web development is a vast field with constantly evolving technologies and best practices. The key to success is continuous learning and practice.
As you continue your journey, don’t be afraid to experiment, build projects, and seek out new challenges. Join coding communities, contribute to open-source projects, and stay curious about new web technologies. With dedication and persistence, you’ll be well on your way to becoming a skilled web developer.
Happy coding, and may your web development journey be exciting and rewarding!